Mastering JAXB: Common Pitfalls and How to Avoid Them
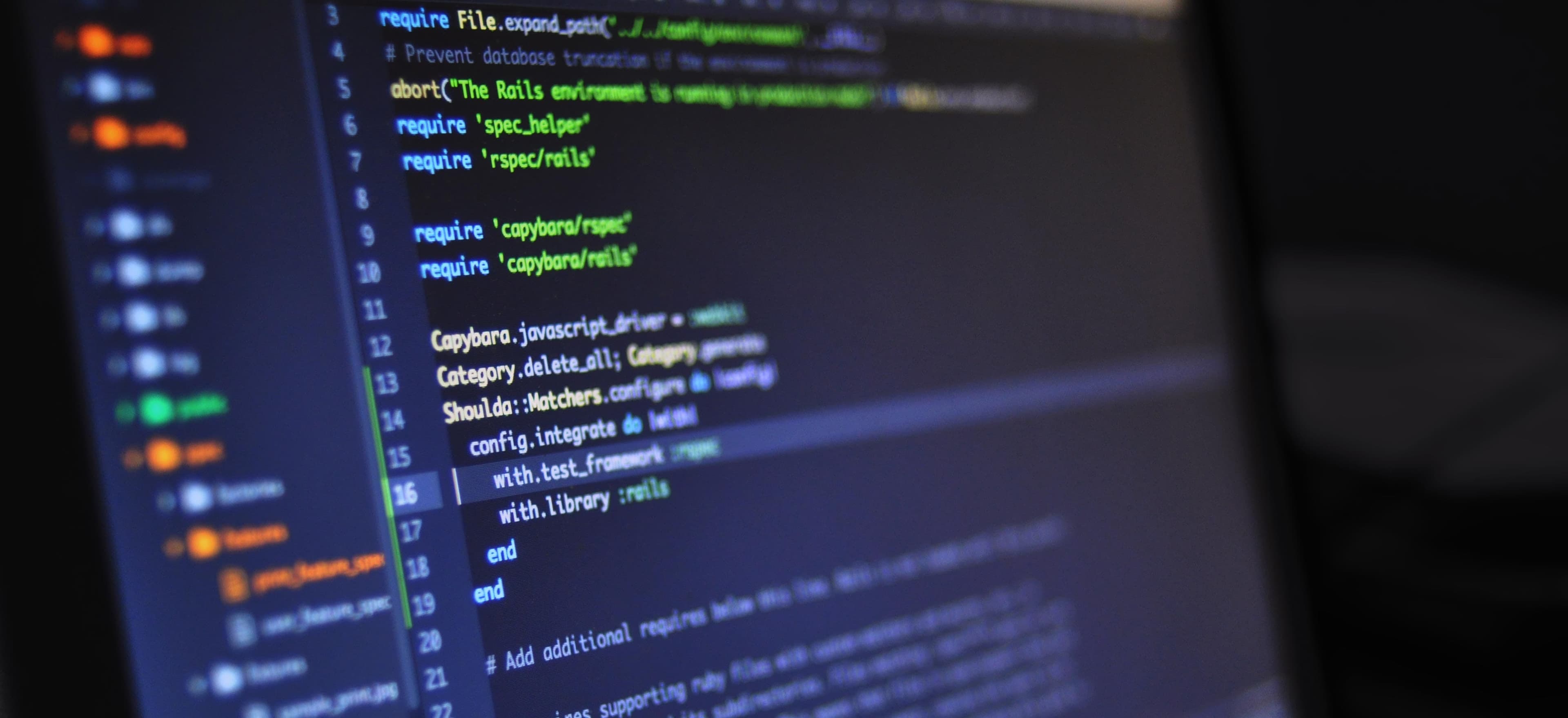
- Published on
Mastering JAXB: Common Pitfalls and How to Avoid Them
Java Architecture for XML Binding (JAXB) is a powerful framework used to convert Java classes into XML and vice versa. Despite its convenience, many developers encounter common pitfalls when using JAXB. This blog post aims to highlight these challenges and provide effective solutions, helping you master JAXB like a pro.
What is JAXB?
JAXB stands for Java Architecture for XML Binding. It simplifies the interaction between XML data and Java applications through the use of annotations. With JAXB, developers can easily marshal (convert Java objects to XML) and unmarshal (convert XML back to Java objects) their data.
Example of JAXB in Action
Before diving into the pitfalls, let's look at a basic example of JAXB in action.
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import java.io.File;
public class JaxbExample {
public static void main(String[] args) {
try {
// Create a new person object
Person person = new Person("John", "Doe");
// Marshal the Java object to XML
JAXBContext context = JAXBContext.newInstance(Person.class);
Marshaller marshaller = context.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
marshaller.marshal(person, new File("person.xml"));
// Unmarshal XML back to Java object
Unmarshaller unmarshaller = context.createUnmarshaller();
Person unmarshalPerson = (Person) unmarshaller.unmarshal(new File("person.xml"));
System.out.println(unmarshalPerson);
} catch (JAXBException e) {
e.printStackTrace();
}
}
}
class Person {
public String firstName;
public String lastName;
// Default constructor is necessary for JAXB
public Person() {}
public Person(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
@Override
public String toString() {
return "Person{firstName='" + firstName + '\'' + ", lastName='" + lastName + '\'' + '}';
}
}
Why Use JAXB?
The main appeal of JAXB lies in its ability to simplify code complexity. Marshalling and unmarshalling are handled in just a few lines of code, allowing developers to focus on other aspects of their application.
However, while JAXB is indeed powerful, its advantages come with challenges. Here are some common pitfalls and how you can avoid them.
Common Pitfalls in JAXB
1. Not Using Annotations Correctly
One of the most common issues is the improper use of JAXB annotations. Annotations such as @XmlRootElement
, @XmlElement
, and @XmlAttribute
are crucial for mapping Java classes to XML elements accurately.
Solution: Always use appropriate JAXB annotations. A typical JAXB annotated class should look like this:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "person")
public class Person {
private String firstName;
private String lastName;
public Person() {}
@XmlElement(name = "first-name")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
@XmlElement(name = "last-name")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
}
2. Missing No-Argument Constructor
JAXB requires a no-argument constructor for marshalling and unmarshalling processes. Failure to include one often leads to exceptions during runtime.
Solution: Ensure every JAXB class implements a public no-argument constructor.
3. Ignoring Default Values
When converting Java objects to XML, JAXB won't include fields with default values. This can be misleading, especially if those fields are essential for your application logic.
Solution: Use @XmlAccessorType(XmlAccessType.FIELD)
to map fields explicitly. Below is an example:
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
@XmlAccessorType(XmlAccessType.FIELD)
public class Person {
private String firstName = "John";
private String lastName = "Doe";
}
4. Inconsistencies in XML Schema and Java Classes
Mismatch between XML schema (XSD) definitions and your Java classes can result in runtime exceptions. This is especially problematic when the XML data structure evolves, but the Java model remains unchanged.
Solution: Always regenerate your Java classes from the XML schema when changes occur. Tools like xjc
can help generate Java objects from XSD files, ensuring consistency between your schema and code.
5. Handling Collections Incorrectly
JAXB has special requirements for collections. Simply marking a list or set as a field does not work automatically.
Solution: Use @XmlElement
properly when working with collections. Here’s an example of how to manage a list of Person
objects:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import java.util.ArrayList;
import java.util.List;
@XmlRootElement(name = "company")
public class Company {
private List<Person> employees = new ArrayList<>();
@XmlElement(name = "employee")
public List<Person> getEmployees() {
return employees;
}
public void setEmployees(List<Person> employees) {
this.employees = employees;
}
}
6. Exception Handling
Many developers underestimate the importance of exception handling with JAXB. Unmarshalling operations can throw exceptions that indicate malformed XML.
Solution: Wrap your JAXB operations within try-catch blocks to catch JAXBException
and provide meaningful error messages.
7. XML Namespace Issues
Ignoring XML namespaces can lead to unexpected results, especially when your XML interacts with systems or APIs that require specific namespaces.
Solution: Use the @XmlSchema
annotation to set the default namespace for your JAXB classes. Here’s how:
import javax.xml.bind.annotation.XmlSchema;
@XmlSchema(namespace = "http://www.example.com/schema")
package mypackage;
The Last Word
JAXB is a fantastic tool that simplifies the complexity of XML handling in Java applications. However, avoiding common pitfalls is crucial to harnessing its full potential. By understanding the key issues that arise and employing the solutions provided in this post, you can ensure your JAXB implementation is robust and effective.
For more in-depth knowledge about XML processing in Java, consider checking out the official JAXB documentation and the Java XML Binding (JAXB) tutorial at Baeldung.
Remember, the right knowledge equips you to tackle the challenges of JAXB head-on. Happy coding!
Checkout our other articles