Struggling with GraalVM Performance? Here’s the Fix!
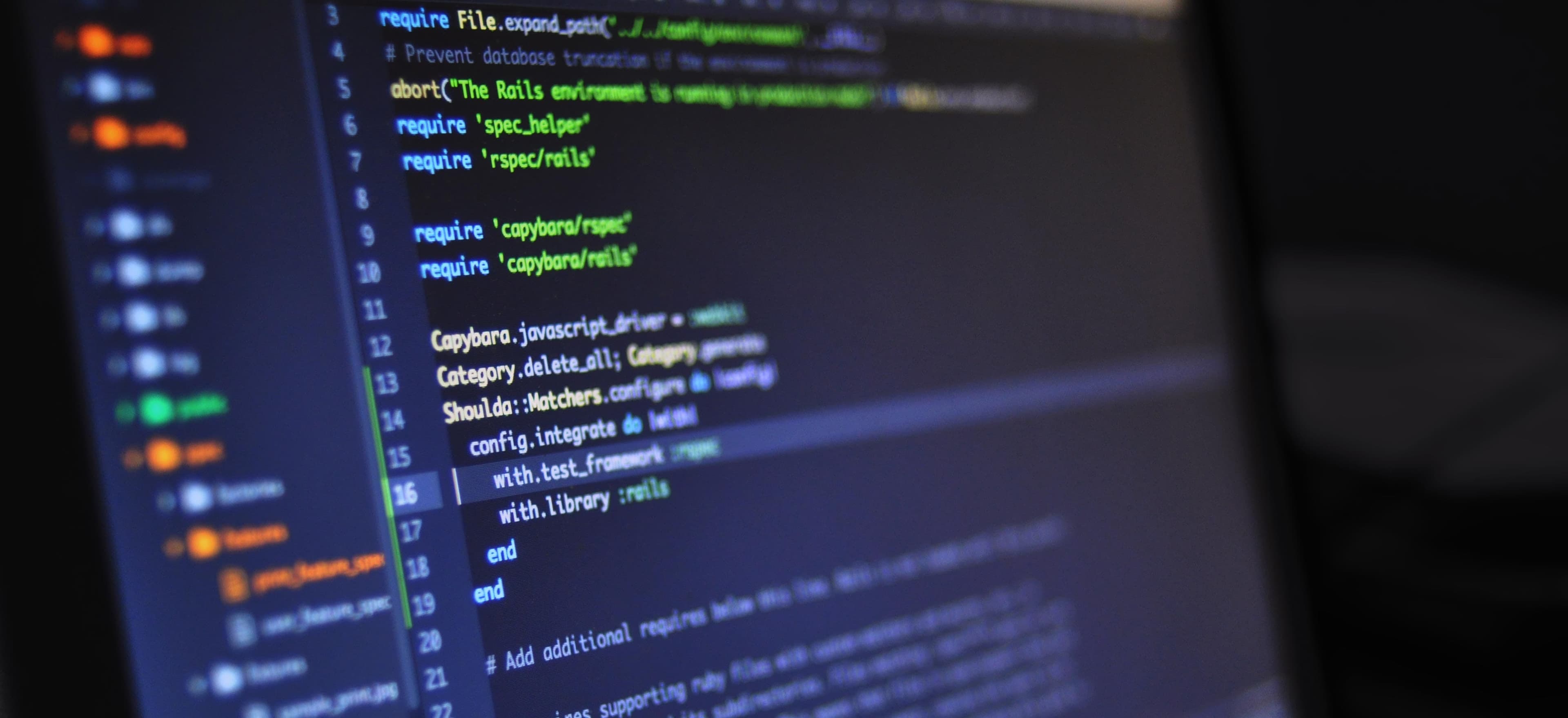
- Published on
Struggling with GraalVM Performance? Here’s the Fix!
GraalVM is an advanced virtual machine that supports various languages and execution modes, promising remarkable performance improvements when compared to traditional JVMs. However, improving performance often involves understanding its features and optimizing your application accordingly. In this blog post, we will dive deep into the performance issues you might face when using GraalVM and provide actionable solutions.
Understanding GraalVM and Its Performance Capabilities
Before we discuss performance fixes, it's important to understand what makes GraalVM special. GraalVM offers several remarkable features including:
- Polyglot Capabilities: GraalVM can execute code written in different languages (Java, JavaScript, Python, etc.) seamlessly.
- Native Image: This feature compiles Java applications ahead-of-time into native binaries, which can lead to faster startup times and reduced memory consumption.
- Just-In-Time Compilation: GraalVM optimizes performance using advanced techniques like partial evaluation and escape analysis.
While these features are designed to boost performance, developers often face challenges, ranging from slow startup times to higher memory consumption. Let’s explore common issues and solutions.
Common Performance Issues
- Slow Startup Times: One of the key shortcomings of GraalVM is that it might have longer startup times compared to other JVMs.
- Higher Memory Usage: While GraalVM can lead to memory savings in certain scenarios, improper configuration can lead to excessive memory consumption.
- Suboptimal Native Builds: The native image feature, if not configured correctly, can result in missing optimizations and greater binary sizes.
Solutions to Boost GraalVM Performance
1. Optimize Your Native Image Build
The native image feature is a powerful tool for improving startup time and reducing memory usage. However, improper usage can lead to missed optimization opportunities.
Example Code Snippet
import com.oracle.svm.core.annotation.*;
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, GraalVM!");
}
}
To generate an optimized native image, the command line should include options for garbage collection settings, reflection configuration, etc.
native-image --no-fallback --initialize-at-build-time=HelloWorld HelloWorld
Why This Matters: The --no-fallback
option prevents a fallback image from being created, which uses the JVM and may negate some benefits of the native image. The --initialize-at-build-time
flag helps optimize the application further by executing initialization at build time, rather than runtime.
2. Reduce Native Image Size
A common issue with native images is their larger footprint. You can address this by excluding unused parts of your application.
Code Example
import com.oracle.svm.core.annotation.*;
@NativeImageHint
public class MyService {
// Only methods that you will use in your application should be included.
public void usefulMethod() {
// Implementation
}
}
To generate a smaller image, you can use the -H:ExcludeClasses
parameter:
native-image MyService -H:ExcludeClasses=SomeUnneededClass
Why This Matters: Reducing the number of classes and resources included in the native image can lead to better startup times and lower memory usage.
3. Control Garbage Collection
GraalVM provides options for tuning garbage collection for improved performance. In many scenarios, utilizing alternative garbage collectors can provide better behavior suited to your application.
Example Code Snippet
java -XX:+UseZGC -jar YourApp.jar
Why This Matters: The Z Garbage Collector (ZGC) is designed for low-latency applications with massive heaps and can significantly improve performance under certain workloads.
4. Perform Profiling and Benchmarking
Before making changes, assess your application’s performance. Tools like Java Flight Recorder (JFR) and GraalVM Profiler allow you to identify performance bottlenecks.
Code Example
// Run the application with Java Flight Recorder enabled
java -XX:StartFlightRecording=duration=60s,filename=my_recording.jfr -jar YourApp.jar
Why This Matters: By profiling your application, you can identify slow methods and memory leaks, allowing for targeted optimizations rather than guesswork.
5. Optimize Java Code
Sometimes, the bottleneck lies within the Java code itself. Even in a high-performance environment like GraalVM, inefficient code practices can lead to performance issues.
Example Code Snippet
public class Fibonacci {
public int compute(int n) {
return (n <= 1) ? n : compute(n - 1) + compute(n - 2);
}
}
For better performance, consider implementing memoization or iterative approaches:
public class Fibonacci {
public int compute(int n) {
if (n <= 1) return n;
int a = 0, b = 1;
for (int i = 2; i <= n; i++) {
int temp = a + b;
a = b;
b = temp;
}
return b;
}
}
Why This Matters: Iterative solutions generally outperform recursive ones in Java due to reduced stack depth and memory allocation.
To Wrap Things Up
GraalVM offers a powerful set of features that can take your Java applications to the next level in terms of performance. However, realizing its full capabilities requires proper configuration and optimizations in both build settings and Java code.
As you experiment with these solutions, don’t forget to benchmark your results. Performance tuning is a continuous cycle: measure, optimize, and then measure again.
For further reading, you can check out GraalVM Documentation for best practices and Java Performance Tuning for additional optimization techniques.
By implementing the strategies outlined in this post, you will be well on your way to achieving stellar performance using GraalVM. If you have your own tips or encounters with performance issues, feel free to share in the comments below!
Checkout our other articles