Simplifying Method Parameters Using the Builder Pattern
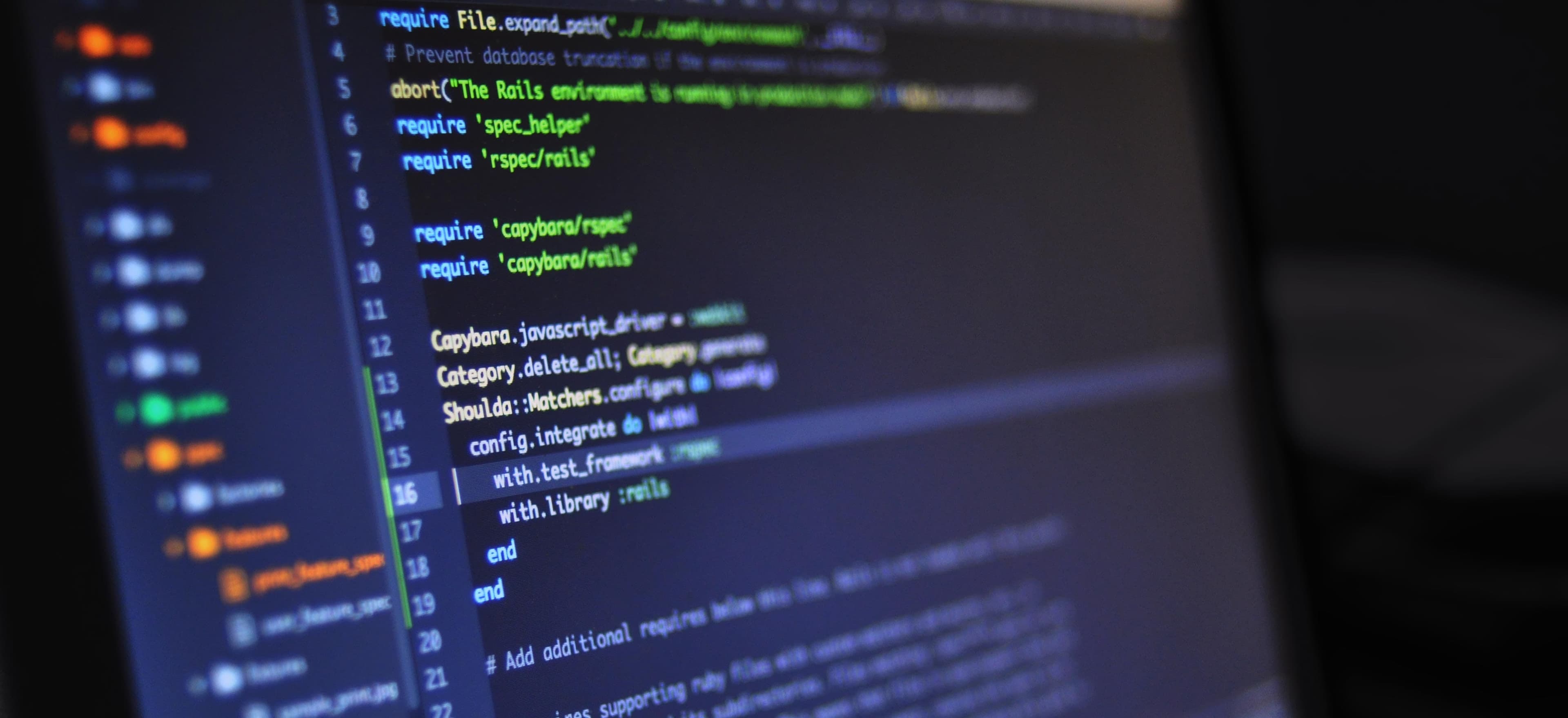
- Published on
Simplifying Method Parameters Using the Builder Pattern
In software development, dealing with method parameters can often become a daunting task, especially as complexity increases. If you've ever found yourself in a situation where a method requires numerous parameters, you understand the headache of managing them. Enter the Builder Pattern! This design pattern not only simplifies method parameters but also enhances code readability and maintainability. In this blog post, we'll explore the Builder Pattern, its advantages, and how to implement it in Java.
What is the Builder Pattern?
The Builder Pattern is a creational design pattern which allows you to construct complex objects step by step. Unlike traditional constructors that might require numerous parameters, a builder provides a clear and concise way to create an object. This is particularly useful when:
- An object has many parameters.
- Many of those parameters are optional.
- You want to enforce immutability of the created object.
Benefits of the Builder Pattern
-
Improved Readability: With the Builder Pattern, the intent of the code becomes clearer. With named methods, it’s easier to understand what each part is doing.
-
Fluency: It promotes a fluent interface, allowing for a more readable code where methods can be chained together.
-
Immutability: Once an object is created, its state does not change, promoting safety and reliability.
-
No Constructor Overloading: Constructor overloading can lead to confusion; the Builder Pattern alleviates this problem by using a single builder class.
Basic Structure of the Builder Pattern
Here's a common way to implement the Builder Pattern in Java:
public class Person {
private final String name;
private final int age;
private final String address;
private Person(Builder builder) {
this.name = builder.name;
this.age = builder.age;
this.address = builder.address;
}
public static class Builder {
private final String name; // Required parameter
private int age; // Optional parameter
private String address; // Optional parameter
// Constructor for required parameters
public Builder(String name) {
this.name = name;
}
// Method for optional parameters
public Builder age(int age) {
this.age = age;
return this;
}
public Builder address(String address) {
this.address = address;
return this;
}
public Person build() {
return new Person(this);
}
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
", address='" + address + '\'' +
'}';
}
}
Breakdown of the Code
- Final Fields: By declaring the fields as
final
, you ensure that the fields are set once during the object's construction and never changed. - Nested Builder Class: The
Builder
class is defined inside thePerson
class, providing a clear relationship between the two. - Chaining Methods: Each method in the
Builder
returnsthis
, enabling chained calls. This does not just improve readability but also makes the creation process more expressive.
Using the Builder Pattern
Now let's see how to utilize our Person
builder to create person objects efficiently.
public class Main {
public static void main(String[] args) {
Person person1 = new Person.Builder("John Doe")
.age(30)
.address("123 Elm Street")
.build();
Person person2 = new Person.Builder("Jane Doe")
.address("456 Oak Avenue")
.build();
System.out.println(person1);
System.out.println(person2);
}
}
Explanation of Usage
In this example, you create two Person
objects. The first one has all parameters set, while the second one only sets the required parameter and one optional. This exemplifies how you can control the complexity of object creation while keeping your code clean and easy to read.
When to Use Builder Pattern?
The Builder Pattern is particularly useful when:
- Complex Objects: If an object requires many parameters or has a complicated initialization process.
- Optional Parameters: When most parameters have sensible defaults and only a few need to be specified.
- Immutability: When the created object should not change state after its creation.
Performance Considerations
While the Builder Pattern offers numerous advantages, it does come with a performance overhead due to the creation of an additional builder object. In cases where performance is a critical concern, the benefits of increased readability and maintainability must be weighed against potential inefficiencies.
Bringing It All Together
The Builder Pattern is a highly effective design strategy for simplifying method parameters and enhancing code maintainability in Java. When creating complex objects with multiple optional parameters, the Builder Pattern shines by promoting a clear and fluent construction process.
If you're interested in deepening your understanding of design patterns, consider exploring the Gang of Four's Design Patterns Book), which offers numerous insights about various design patterns, including the Builder Pattern.
By employing the Builder Pattern in your Java applications, you not only improve the readability of your code but also foster a culture of cleaner, more maintainable, and robust code. Start using the Builder Pattern today and witness the transformation of how you handle method parameters!