Transforming 2D Arrays: The Java Flattening Challenge
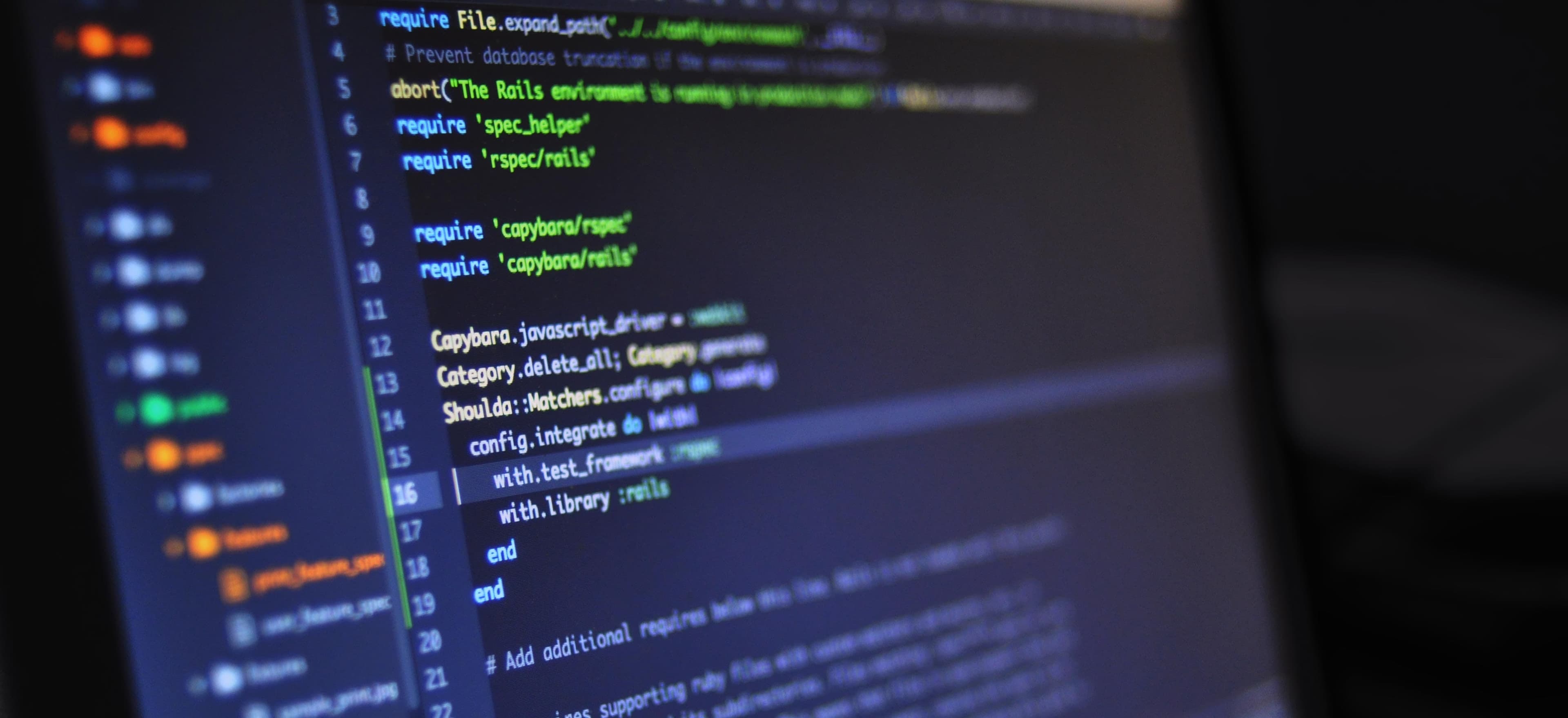
- Published on
Transforming 2D Arrays: The Java Flattening Challenge
Java is a powerful programming language that allows developers to manipulate data structures with ease. One common challenge that developers often face is transforming 2D arrays into 1D arrays. This process is known as "flattening." In this blog post, we will explore how to flatten a 2D array in Java, its applications, and best practices to ensure the implementation is both efficient and clean.
How to Flatten a 2D Array in Java
The objective of flattening a 2D array is to convert all of its elements into a single-dimensional array. This might seem simple, but understanding the logic and structure is crucial for writing effective code.
Understanding 2D Arrays
Before we dive into code, let’s clarify what a 2D array is. In Java, a 2D array is essentially an array of arrays. Each element in a 2D array can be accessed using two indices.
For instance, consider the following 2D array:
int[][] twoDArray = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
Here, twoDArray[0][0]
would return 1
, while twoDArray[1][2]
would return 6
.
Basic Flattening Approach
To flatten this array, we need to loop through each row and then through each column, adding the element to a new 1D array.
Here is a sample implementation:
public class ArrayFlattener {
public static void main(String[] args) {
int[][] twoDArray = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
int[] flatArray = flatten2DArray(twoDArray);
// Print flattened array
for (int num : flatArray) {
System.out.print(num + " ");
}
}
public static int[] flatten2DArray(int[][] array) {
int totalElements = 0;
// First, calculate the total number of elements
for (int[] row : array) {
totalElements += row.length;
}
// Create a new 1D array to hold the flattened values
int[] flatArray = new int[totalElements];
int index = 0;
// Now, populate the 1D array
for (int[] row : array) {
// Copy elements from the 2D array to the 1D array
System.arraycopy(row, 0, flatArray, index, row.length);
index += row.length; // Move index forward
}
return flatArray;
}
}
Explanation of the Code
-
Calculating Total Elements: The first for-loop counts the number of elements across all rows. This allows us to dynamically allocate the size of the resulting 1D array, which is a good practice to prevent wasting memory.
-
Creating a Flattened Array: Once we know the total number of elements, we create the new 1D array.
-
Copying the Elements: In the second loop, we use
System.arraycopy()
to copy elements from the current row to the flat array. This method is efficient because it minimizes the overhead of repeated assignments.
Alternative Approach: Using Streams
With Java 8 and above, we can also take advantage of the Stream API for a more concise implementation. Here’s how:
import java.util.Arrays;
public class StreamArrayFlattener {
public static void main(String[] args) {
int[][] twoDArray = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
int[] flatArray = flattenWithStreams(twoDArray);
// Print the flattened array
Arrays.stream(flatArray).forEach(num -> System.out.print(num + " "));
}
public static int[] flattenWithStreams(int[][] array) {
return Arrays.stream(array) // Convert 2D array to Stream<int[]>
.flatMapToInt(Arrays::stream) // Flatten the Stream<int[]> to IntStream
.toArray(); // Convert to int[]
}
}
Explanation of the Stream Code
-
Converting to Stream: First, the 2D array is converted to a stream of arrays (
Stream<int[]>
). -
Flattening the Stream:
flatMapToInt(Arrays::stream)
takes each array within the stream and flattens it down to a stream of integers. -
Creating the Final Array: Finally, we convert the resulting stream back into an integer array.
This approach minimizes the amount of code you have to write and makes it easier to read. Streams are also beneficial in terms of performance for large datasets.
When to Use Flattened Arrays
Flattening arrays can be useful in various scenarios:
- Data Processing: Converting grids of data (such as images) into a one-dimensional format for easier manipulation.
- Database Interaction: When fetching rows from a database, you might want a simple list of elements.
- Algorithms: Certain algorithms work more efficiently with one-dimensional data, particularly in sorting and searching.
In Conclusion, Here is What Matters
In summary, flattening a 2D array in Java is a relatively straightforward process. Whether you choose to use traditional loops or the Stream API depends on your specific use case and preference. Both methods we discussed have their advantages—clarity and performance, respectively.
Understanding how to handle arrays is fundamental in Java and serves as an excellent exercise for honing your algorithmic thinking. Implementing this concept not only improves your skills but can also make your codebase more efficient and adaptable.
For more resources on arrays and their manipulation in Java, you can check out the official Java documentation.
Feel free to ask any questions or share your own experiences with flattening arrays in Java in the comments below!
Checkout our other articles