Parsing Errors: Handling Spaces in ANTLR 4 Grammar
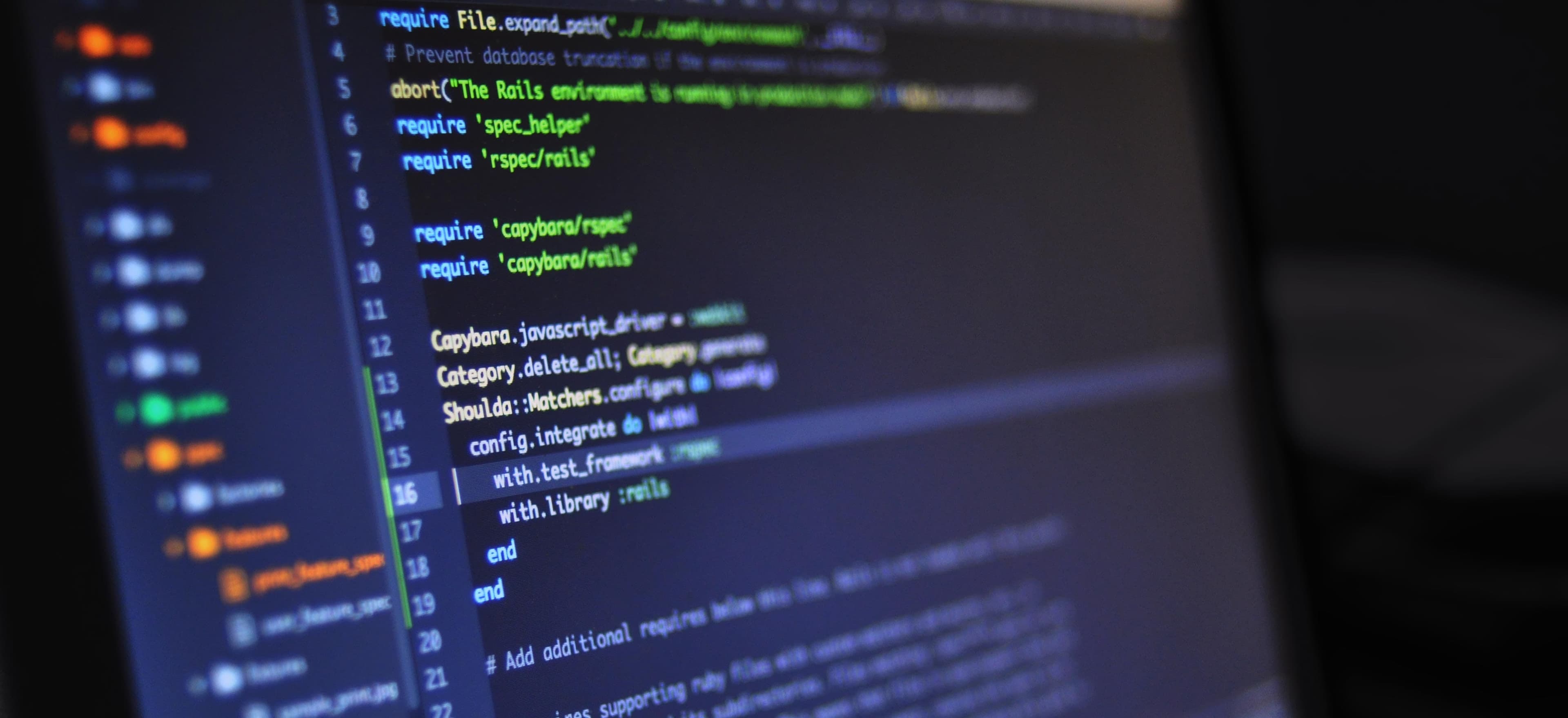
- Published on
Parsing Errors: Handling Spaces in ANTLR 4 Grammar
When developing a parser using ANTLR 4, one of the common challenges developers face is handling white spaces and parsing errors that can arise from unexpected spaces in the input. In this blog post, we'll explore how to manage spaces effectively in your ANTLR grammars, address parsing errors, and ensure your parser produces the intended results. By the end, you will understand how to create robust grammars that can gracefully handle mistakes and whitespace.
What is ANTLR 4?
ANTLR (ANother Tool for Language Recognition) is a popular parser generator that can be used to create parsers for reading, processing, translating, or executing programming languages. ANTLR 4 comes with numerous features, allowing it to generate parsers for a variety of programming languages using simple and expressive grammar syntax.
Understanding Whitespace in ANTLR
In ANTLR, the treatment of whitespace can be crucial. By default, ANTLR ignores whitespace and comments, but there are situations where you might need to be more explicit about how whitespace is handled. Here is why whitespace handling is vital:
- Code Readability: Spaces enhance human readability; hence, developers often use them liberally.
- Parsing Failures: Incorrectly handled whitespace can lead to parsing errors, resulting in unexpected behavior or output.
- Input Flexibility: By managing spaces intelligently, your parser can accept a variety of formatted input.
Let’s create a small example grammar to illustrate our points.
Example Grammar
Here’s a simple grammar that recognizes expressions involving addition and subtraction.
grammar Expr;
// Entry point for parsing
expr: term (('+'|'-') term)*;
// Define a term
term: INT;
// Define the integer
INT: [0-9]+;
// Ignore spaces
WS: [ \t\n\r]+ -> skip;
Breakdown of the Grammar
-
Continuity: The
expr
rule allows for multiple terms separated by '+' or '-'.expr: term (('+'|'-') term)*;
This line expresses that an expression consists of one term and can be followed by more terms, separated by operators.
-
The Integer Token: The
term
rule uses theINT
lexer rule defined below it. TheINT
rule captures numeric input.term: INT; INT: [0-9]+;
-
Whitespace Handling: The
WS
rule captures spaces, tabs, and newline characters and instructs ANTLR to skip them.WS: [ \t\n\r]+ -> skip;
This approach ensures that whitespace does not interfere with parsing while leaving flexibility for the user.
Testing the Grammar
To see how this works in practice, we can create a small Java program that uses this grammar to parse an input string.
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.tree.*;
public class ExprTest {
public static void main(String[] args) {
String expression = "3 + 5 - 2"; // Whitespace variations
// Create the lexer
ExprLexer lexer = new ExprLexer(CharStreams.fromString(expression));
// Create the parser
CommonTokenStream tokens = new CommonTokenStream(lexer);
ExprParser parser = new ExprParser(tokens);
// Parse the expression
ParseTree tree = parser.expr();
// Print the parse tree
System.out.println(tree.toStringTree(parser));
}
}
Code Explanation
- Lexer & Parser: This code initializes the lexer and parser to handle the input expression.
- Whitespace Handling: The grammar set up above allows for whitespace between numbers and operators, demonstrating how flexible input can lead to a valid parsed structure.
- Parse Tree Display: Finally, it prints the parse tree representation. If the grammar captures the expression correctly, the output will reflect the structure of the analyzed expression.
Dealing with Parsing Errors
Now that we know how whitespace is handled, let's focus on managing parsing errors. This area can be complex because input deviations can often lead to runtime exceptions or incorrect parsing states.
Using Error Handling in ANTLR
ANTLR provides several ways to customize error-handling behavior. Depending on the complexity of your input and needs, you may want to adopt different strategies for error recovery.
- Custom Error Listener: By creating your custom error listener, you can manage how your parser responds to errors more effectively.
class CustomErrorListener extends BaseErrorListener {
@Override
public void syntaxError(Recognizer<?, ?> recognizer,
Object offendingSymbol,
int line,
int charPositionInLine,
String msg,
RecognitionException e) {
System.err.println("Syntax error at line " + line + ":" + charPositionInLine + " - " + msg);
}
}
- Attach the Listener: To use this custom error listener, attach it to your parser.
parser.removeErrorListeners(); // Remove default listener
parser.addErrorListener(new CustomErrorListener());
- Testing Error Handling: Now, you can test how your parser handles erroneous input with unexpected spacing, such as:
String errorExpression = "3 + - 5"; // Extra operator
// Attach the error listener and execute...
Example Error Handling
When the example parser encounters an erroneous expression, our custom error listener can provide specific feedback on the nature of the syntax error, allowing developers to debug their code effectively.
Lessons Learned
In this blog post, we’ve covered how to handle spaces in ANTLR 4 grammars effectively and the strategies to manage parsing errors that may arise. We illustrated a basic expression grammar, provided sample Java code for parsing, and detailed custom error handling mechanisms.
Working with ANTLR allows for great flexibility in parsing input, but it requires careful planning in grammar design, especially when dealing with whitespace and potential parsing errors. For more information on ANTLR, you can check ANTLR's official documentation and ANTLR 4 in Action.
With these strategies, you should be able to handle spaces in your grammars and provide clear error messages that enhance the development experience. Happy parsing!