Common SLF4J Mistakes to Avoid in Java Development
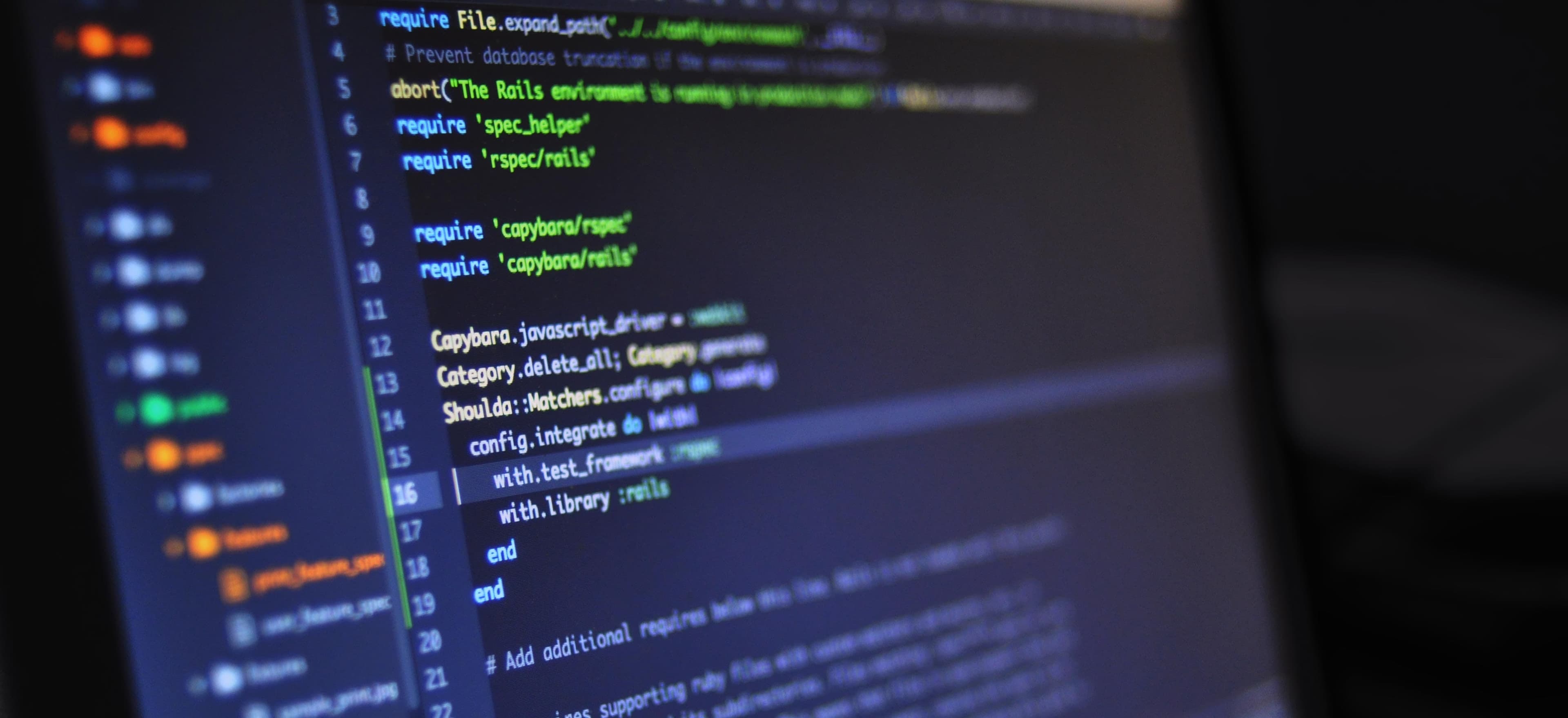
- Published on
Common SLF4J Mistakes to Avoid in Java Development
In the world of Java development, logging plays a crucial role in monitoring applications, debugging issues, and capturing usage metrics. SLF4J, or Simple Logging Facade for Java, simplifies the usage of different logging frameworks by providing a common API. However, developers often run into several common pitfalls when using SLF4J. In this blog post, we’ll discuss these mistakes and offer solutions to help you avoid them.
1. Not Choosing the Right Binding
SLF4J functions as a facade, meaning that while it provides a common interface for logging, it does not perform the actual logging. To enable logging, you must choose an appropriate binding.
Mistake:
Forgetting to include a logging implementation can lead to runtime errors.
Example Solution:
Ensure you include a binding for the SLF4J API in your build file. Here’s an example using Maven:
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.3</version>
</dependency>
Why: Logback is a reliable choice and works seamlessly with SLF4J. You may also explore other options such as Log4j or java.util.logging, but ensure that you have included the appropriate dependency in your project setup.
2. Using the Wrong Logger Level
Java developers sometimes misuse logging levels, leading to either too much information being logged or essential data being missed.
Mistake:
Confusing debug
, info
, warn
, error
, and trace
levels can clutter log files.
What to Use:
- DEBUG: Use for detailed technical information. This level is essential during development but should be minimized in production.
- INFO: Use for high-level application events such as startup notifications or significant actions like user registrations.
- WARN: This level highlights potential issues that are not yet errors.
- ERROR: When exceptions occur or the application does not function as intended, log an error.
Code Example:
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
public void process() {
logger.debug("Starting process");
try {
// Simulate processing
logger.info("Processing data");
} catch (Exception e) {
logger.error("An error occurred: {}", e.getMessage(), e);
}
}
Why: Properly categorizing logging events makes it easier to filter and analyze logs later. Application performance can also be improved if lower levels of logging such as DEBUG are disabled in production.
3. Logging Exception Stack Traces Incorrectly
Developers may often log exception stack traces without providing enough context, which can make troubleshooting difficult.
Mistake:
Logging errors without relevant context or just using printStackTrace()
.
Correct Approach:
Utilize the logging framework for your error messages.
Code Example:
public void handleRequest() {
try {
// Logic that might throw an exception
} catch (SpecificException e) {
logger.error("Failed to handle request for user ID {}: {}", userId, e.getMessage());
}
}
Why: Providing contextual information, such as input parameters or user IDs, makes it easier to diagnose issues from logs.
4. Not Using Parameterized Logging
String concatenation in logging statements can lead to performance issues, especially if the logging level is set at a higher level (e.g., WARN or ERROR).
Mistake:
Using plain string concatenation with logging.
Example:
// Incorrect
logger.debug("Processing user with ID " + userId);
Correct Approach:
Utilize SLF4J's parameterized logging.
Code Example:
// Correct
logger.debug("Processing user with ID {}", userId);
Why: Parameterized messages are only constructed if the logging level is active, which can offer performance benefits. This is particularly important in performance-sensitive applications.
5. Ignoring the Configuration of Logback or Other Logging Frameworks
The configuration of loggers is essential for effective logging. Developers sometimes overlook the configuration, leading to undesired log outputs.
Mistake:
Default configurations often do not suit production environments.
Solution:
Customize the logback.xml
(for Logback) or equivalent configuration to adjust the log levels and appenders.
Example Configuration:
<configuration>
<appender name="console" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<logger name="com.myapp" level="DEBUG"/>
<root level="INFO">
<appender-ref ref="console" />
</root>
</configuration>
Why: Properly configuring the logging framework allows you to control where logs are written (console, file, etc.) and at what level, ensuring that your logging infrastructure provides the necessary insights without overwhelming noise.
6. Failing to Log Entry and Exit Points
Many developers focus on error handling but neglect to log entry and exit points within critical methods.
Mistake:
Not tracking method entrances and exits can create challenges in debugging.
Example Solution:
Add logging at the beginning and end of methods.
Code Example:
public void performTask() {
logger.debug("Entering performTask");
try {
// Task implementation
} finally {
logger.debug("Exiting performTask");
}
}
Why: This practice improves traceability and helps in understanding how information flows through your application.
My Closing Thoughts on the Matter
Logging is a fundamental aspect of Java application development, and SLF4J provides a powerful yet flexible framework for managing it. By avoiding these common mistakes, you not only improve the quality of your logging but also ensure that your system logs provide valuable insights for debugging and monitoring.
For more in-depth reading on logging best practices, consider checking out the SLF4J official documentation and Logback.
By implementing the suggestions outlined in this article, you will not only enhance your logging strategies but also contribute to the overall robustness and reliability of your Java applications. Happy coding!