Troubleshooting Common Issues with Spring Annotations
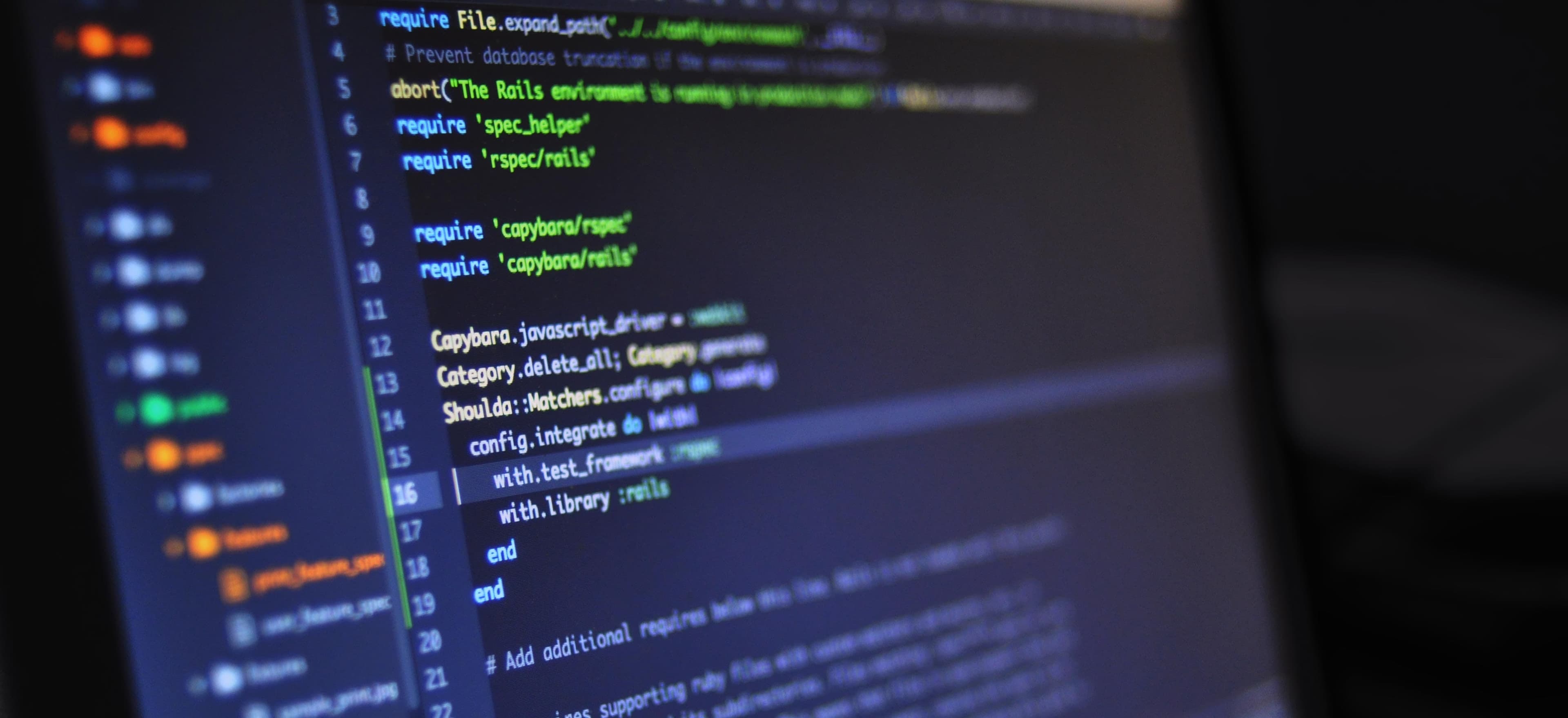
- Published on
Troubleshooting Common Issues with Spring Annotations
Spring Framework is a robust and powerful framework that simplifies enterprise Java application development. With the advent of Spring Annotations, the complexity of the configuration has significantly decreased. However, improper usage or understanding of these annotations can lead to common issues that can be a headache for developers. In this blog post, we will dive into troubleshooting these issues, understanding their causes, and providing effective solutions.
Understanding Spring Annotations
Spring Annotations provide a way to configure your Spring beans without the need for extensive XML configuration files. They promote cleaner code and make applications more maintainable. Here are some of the most commonly used annotations:
- @Component: Indicates that a class is a Spring-managed component.
- @Service: A specialization of @Component, used in service layer classes.
- @Repository: A specialization of @Component, used for persistence layer classes.
- @Controller: Marks a class as a web controller.
- @Autowired: Automatically injects the required dependencies.
- @Configuration: Indicates that a class can be used by the Spring IoC container as a source of bean definitions.
Common Issues and Troubleshooting Steps
1. BeanNotFoundException
Issue: One of the common issues when using Spring annotations is the BeanNotFoundException
, which occurs when the Spring container cannot find a required bean.
Cause: This can happen because you may not have annotated your class with @Component
, or the component scanning may not be set up correctly.
Solution:
- Ensure that the class is annotated with the appropriate stereotype annotations (e.g.,
@Service
,@Repository
, or@Component
). - Check the component scan configuration.
@Configuration
@ComponentScan(basePackages = "com.example")
public class AppConfig {
// Configuration details
}
2. NoSuchBeanDefinitionException
Issue: The NoSuchBeanDefinitionException
arises when the Spring context does not contain a bean definition for the required type.
Cause: This often occurs if you forget to create a Spring-managed bean for the specified dependency.
Solution:
- Ensure that the class you are trying to inject is correctly annotated.
- Verify that the package containing the bean is included in the component scanning.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
3. Circular Dependency Issues
Issue: Circular dependencies can arise when two or more beans depend on one another, resulting in a BeanCurrentlyInCreationException
.
Cause: This problem typically occurs when two beans reference each other directly through @Autowired
.
Solution:
- Refactor the code to eliminate circular dependencies.
- Use
@Lazy
annotation to solve issues temporarily, but it is not the best practice for long-term solutions. For example:
@Component
public class A {
@Autowired
@Lazy
private B b;
}
@Component
public class B {
@Autowired
private A a;
}
4. @Autowired Not Working
Issue: Sometimes @Autowired
may not inject the required beans, which might leave you with a NullPointerException
.
Cause: This can happen due to several reasons, including Spring context not being properly configured, or if the beans were not registered in the context.
Solution:
- Ensure your classes are being scanned correctly.
- Check for
@Autowired
annotations in classes and their visibility (public/private). - It’s also a good practice to specify the required attribute.
@Autowired(required = false)
private SomeService someService; // Won't throw exception if not found.
5. Context Not Loaded
Issue: When running tests, you may encounter issues where the Spring context does not load.
Cause: This usually happens due to incorrect configuration in your test classes.
Solution:
- Use the right annotations like
@RunWith(SpringJUnit4ClassRunner.class)
and@ContextConfiguration(classes = AppConfig.class)
. - Ensure your test classes are in the correct package and can access the application context.
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = {AppConfig.class})
public class UserServiceTest {
@Autowired
private UserService userService;
@Test
public void testUserService() {
// Your test code here
}
}
6. Missing @Transactional
Issue: When database operations don’t reflect changes as expected.
Cause: This typically occurs when the transaction management is not properly set up, particularly when using repositories.
Solution:
- Ensure your service methods that require transaction management are annotated with
@Transactional
.
@Service
public class AccountService {
@Autowired
private AccountRepository accountRepository;
@Transactional // This will ensure a transaction is created
public void transferMoney(Account from, Account to, double amount) {
accountRepository.debit(from, amount);
accountRepository.credit(to, amount);
}
}
Additional Best Practices
-
Use Profiles: When developing applications that need different configurations for different environments (development, testing, production), utilize Spring Profiles.
-
Stay Updated: Ensure you are using the latest version of Spring to benefit from bug fixes and improvements.
-
Read the Documentation: The official Spring Documentation is an excellent resource for understanding the nuances of Spring annotations.
-
Testing: Always write comprehensive tests to catch issues early. Utilize Spring’s testing framework to help mock your context and dependencies properly.
Final Thoughts
Even though Spring annotations simplify configuration and make it more intuitive, they can introduce complexities, especially when misused. However, understanding these common issues and their solutions can significantly reduce the downtime during development. By following the best practices shared in this post, you can prevent many of these pitfalls and ensure a smooth Spring development experience. Happy coding!
For more information on Spring Annotations, check out the Spring Annotations Reference and dive deeper into their functionalities.