Optimizing Cache: Avoiding Stale Data Issues
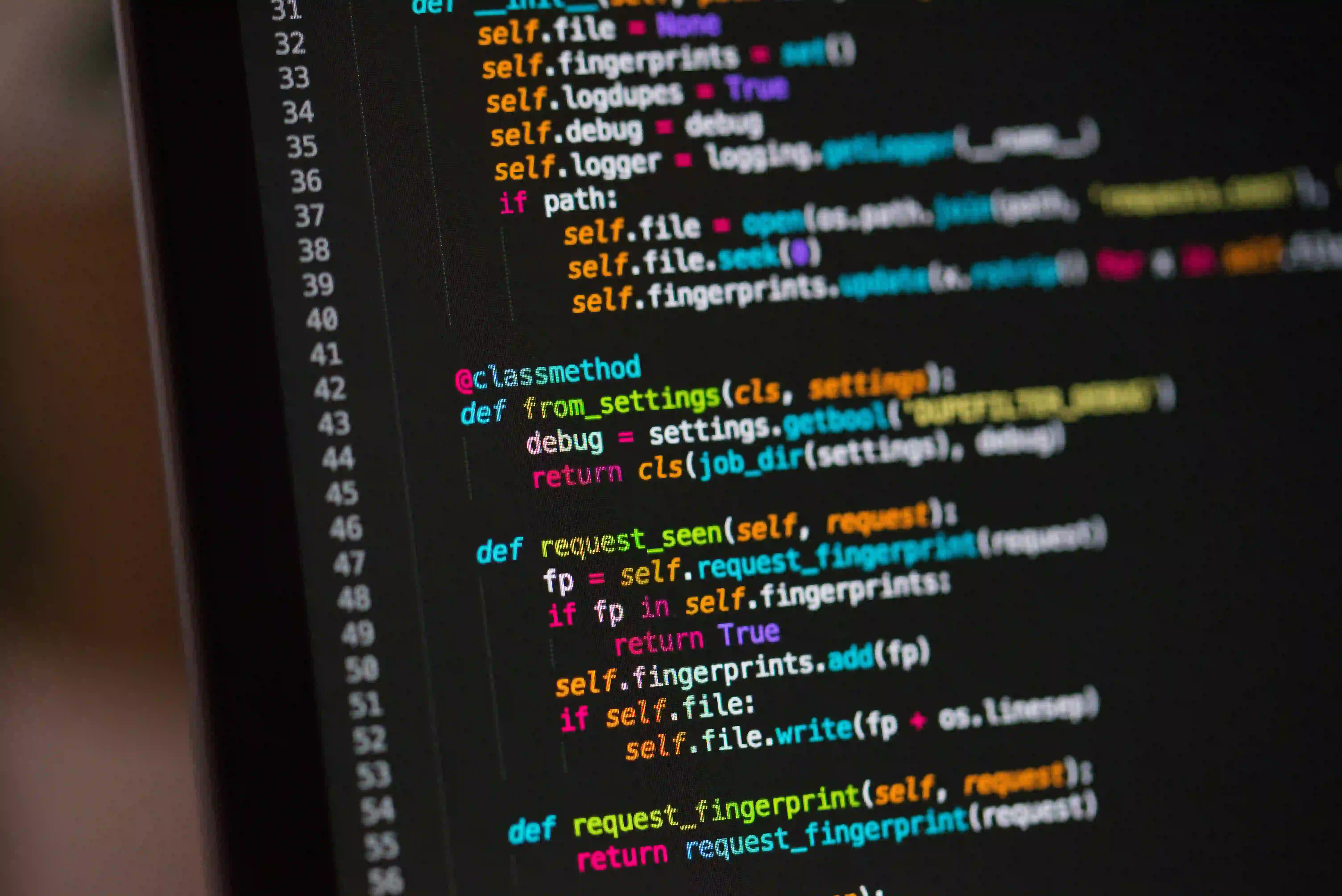
Optimizing Cache: Avoiding Stale Data Issues
Caching is an essential component of modern software architecture. It enhances performance by storing frequently accessed data for quick retrieval. However, a common pitfall is the risk of serving stale data. This not only dilutes user experience but can also lead to severe application issues. In this blog post, we'll explore strategies to optimize caching in Java applications and effectively avoid stale data problems.
Understanding Cache and Stale Data
What is Cache?
Cache is a temporary storage layer that saves copies of data or computed results to reduce latency in data access. It exists at various levels - from web browsers to distributed systems.
What is Stale Data?
Stale data refers to cached information that has become outdated or incorrect, typically due to underlying data changes that are not reflected in the cache. For instance, if a user's profile information is updated in a database, but the cached version of that profile is not refreshed, any access to the cache will yield outdated data.
Why Caching is Crucial
- Performance Improvement: Speeding up data retrieval results in faster application performance.
- Reduced Load on Databases: Caching helps mitigate traffic to the database, reducing operational costs.
- Enhanced User Experience: Quick data access can significantly improve user engagement and satisfaction.
Strategies to Avoid Stale Data
To effectively manage cache and avoid stale data, consider the following strategies:
1. Cache Expiration Policies
Setting expiration times enables caches to become invalidated after a specific duration. This reduces the chance of serving stale data.
Example Code
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
import java.util.concurrent.TimeUnit;
public class CacheWithExpiration {
private Cache<String, String> cache;
public CacheWithExpiration() {
cache = Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES) // Cache expires after 10 minutes
.maximumSize(100)
.build();
}
public void putValue(String key, String value) {
cache.put(key, value);
}
public String getValue(String key) {
return cache.getIfPresent(key);
}
}
Why this matters: Setting an expiration time allows the cache to clear out old data automatically. This reduces the likelihood of stale data being served. You'll want to balance the expiration time with how often data is updated.
2. Cache Invalidation
In some applications, especially those with frequent updates, it is crucial to invalidate the cache immediately when underlying data changes.
Example Code
public void updateUserProfile(User updatedUser) {
database.update(updatedUser);
cache.invalidate(updatedUser.getId()); // Immediately invalidate the cache
}
Why this matters: By invalidating the cache right after data modification, we ensure that the next data access fetches fresh data from the underlying source, preventing stale information from getting served.
3. Read-Through Caching
In a read-through cache implementation, whenever data isn't found in the cache, the application fetches it from the database, stores it in the cache, and then returns it to the user.
Example Code
public User getUserById(String userId) {
return cache.get(userId, key -> database.findUserById(key)); // Fetches and caches data on miss
}
Why this matters: This technique bridges cache and data access efficiently, ensuring users get the most up-to-date data while keeping cache usage optimal.
4. Use Versioning
When updating data in your cache, versioning can help track changes to avoid serving stale data. Each data structure associated with the cached object has a version number that increments with each update.
Example Code
class VersionedData {
private String data;
private int version;
public VersionedData(String data, int version) {
this.data = data;
this.version = version;
}
// getters and setters omitted for brevity
}
Why this matters: Versioning allows you to determine whether the cache should be updated based on data versions. This method can be intricate but is highly effective in systems with constant updates.
5. Hybrid Caching Strategies
Some applications benefit from combining different caching strategies. Using both cache expiration and invalidation can significantly reduce stale data issues.
Best Practices for Cache Optimization
- Monitor Cache Hit Ratio: Regularly analyze the cache hit-to-miss ratio. A low hit ratio indicates problems with cache effectiveness.
- Fine-tune Expiration Times: Tailor expiration based on specific data access patterns and update frequency.
- Test Caching Mechanisms: Incorporate load testing to identify potential stale data issues before they escalate in production.
Tools & Libraries for Java Caching
-
Caffeine: This Java library provides an efficient in-memory caching solution with easy-to-configure policies.
-
EHCache: A widely used, mature caching library for Java.
Closing Remarks
Optimizing cache to avoid stale data issues is vital for building fast, reliable Java applications. Implementing strategies like cache expiration, invalidation, read-through caching, and versioning can substantially minimize the risk of serving outdated information. Remember to continually monitor your caching mechanisms and adapt them as your application grows and evolves. You want to ensure your caching strategy aligns with your data access patterns.
By following these principles, not only can you enhance your application’s performance, but you can also provide a better experience for your users. Consider experimenting with the different strategies outlined and assess their impact on your systems. Happy coding!