Navigating Java 9's Complex New Features: A Guide
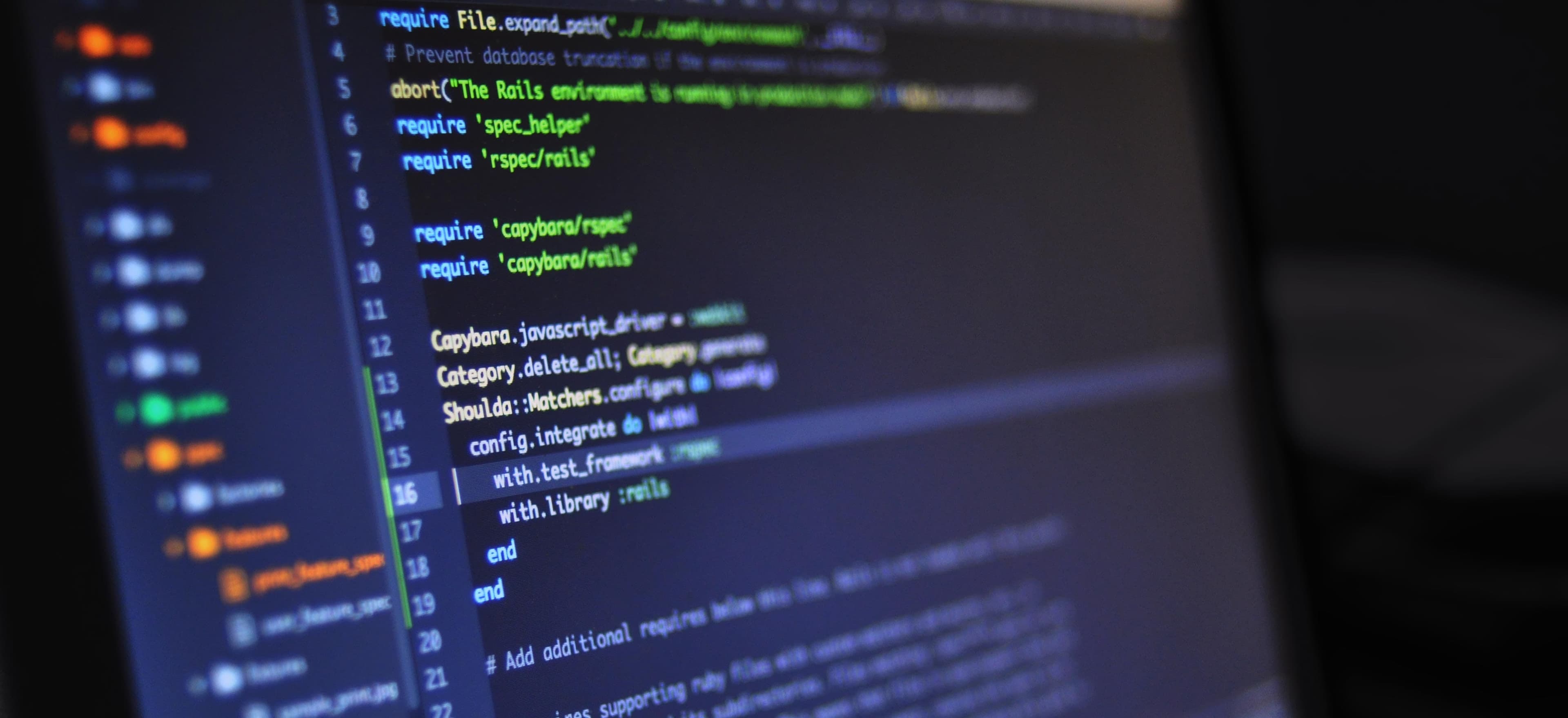
- Published on
Navigating Java 9's Complex New Features: A Guide
Java 9, released in September 2017, brought several significant changes and enhancements to the beloved programming language. From the introduction of the module system to improved APIs, Java 9 aimed to enhance performance, scalability, and usability. In this guide, we will walk through some of the most notable features of Java 9, providing code snippets for clarity and insight into why these changes matter.
Table of Contents
- The Java Platform Module System (JPMS)
- Improved Javadoc
- JShell: The Java Shell
- Stream API Enhancements
- Optional Enhancements
- Conclusion
The Java Platform Module System (JPMS)
What is JPMS?
One of the standout features of Java 9 is the introduction of the Java Platform Module System (JPMS), also known as Project Jigsaw. This module system allows developers to modularize their applications, making them easier to manage, scale, and maintain.
Why Modules?
Prior to Java 9, the Java class path was often chaotic. Developers would typically bundle all dependencies into a single executable JAR file. JPMS changes that by allowing you to define modules explicitly. This provides strong encapsulation, ensuring that the internal implementation of a module is hidden.
Code Example
Below is an example showing how to define a simple module:
// In module-info.java
module com.example.helloworld {
exports com.example.helloworld;
}
This module-info.java
file is essential because it declares the module's name (com.example.helloworld
) and specifies which packages are accessible outside the module.
Benefits of JPMS
- Encapsulation: Only the parts of the module that need to be accessed publicly are exposed.
- Versioning: Modules can be versioned independently.
- Reduced Complexity: It reduces the complexity of external libraries being misused through better organization.
For a deep dive into JPMS, visit the official Java documentation here.
Improved Javadoc
Java 9 also significantly improved Javadoc, making it more user-friendly and easier to use.
Why This Matters
Javadoc is vital for developer collaboration and understanding. The enhancements in Java 9 include:
- Search Functionality: A search box in the generated documentation allows developers to find classes quickly.
- HTML5 Support: Javadoc now supports HTML5, making it more visually appealing and modern.
Code Example
Here’s how you might generate Javadoc for a simple class:
javadoc -d doc -sourcepath src -subpackages com.example
This command will generate documentation in the doc
directory for all files under the com.example
package. Creating clear, accessible documentation helps maintain a clean codebase and aids future contributors.
For a full breakdown of the new Javadoc features, check out the official changes here.
JShell: The Java Shell
Introducing JShell
JShell is a new command-line tool introduced in Java 9, acting as a REPL (Read-Eval-Print Loop) for Java. It allows developers to test snippets of code in real-time, expediting the learning process.
Why JShell?
JShell is incredibly useful for testing code solutions quickly. Whether you’re prototyping or experimenting with new APIs, it simplifies the process.
Code Example
You can open JShell and immediately start coding:
jshell> int x = 5;
jshell> int y = 10;
jshell> x + y
This would output 15
, demonstrating quick calculations without the need to set up an entire project.
For a detailed introduction to JShell, visit the tutorial here.
Stream API Enhancements
New Features in Stream API
Java 9 introduces several new methods to the Stream API, enhancing its functionality and usability. Some examples include takeWhile
, dropWhile
, and ofNullable
.
Why Stream API Enhancements?
These new methods simplify complex data handling tasks, providing more robust processing capabilities.
Code Example
Here’s a practical example using takeWhile
and dropWhile
to filter collections:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Take while the condition holds true
List<Integer> lessThanFour = numbers.stream()
.takeWhile(n -> n < 4)
.collect(Collectors.toList());
// Drop while the condition holds true
List<Integer> greaterThanTwo = numbers.stream()
.dropWhile(n -> n <= 2)
.collect(Collectors.toList());
System.out.println(lessThanFour); // Output: [1, 2, 3]
System.out.println(greaterThanTwo); // Output: [3, 4, 5]
Benefits of Enhanced Stream API
These enhancements make it more intuitive to manipulate collections, leading to cleaner and more readable code. You can access more comprehensive coverage of these enhancements here.
Optional Enhancements
What’s New with Optional?
Java 9 introduced several useful methods for Optional
, such as ifPresentOrElse
and or
.
Why the Enhancements?
These enhancements streamline handling optional values, reducing boilerplate code and improving readability.
Code Example
This example showcases the use of ifPresentOrElse
:
Optional<String> optionalName = Optional.ofNullable(getNameFromService());
optionalName.ifPresentOrElse(
name -> System.out.println("Name found: " + name),
() -> System.out.println("No name found")
);
Here, ifPresentOrElse
neatly handles both cases: whether a value is present or not.
More on Optional Enhancements
The enhancements make using optional values easier and more expressive. To learn more, explore the official documentation here.
My Closing Thoughts on the Matter
Java 9 presented a range of new features that improve code organization, documentation, and usability. The Java Platform Module System (JPMS) redefined how we structure our applications, while enhancements to Javadoc, JShell, Stream API, and Optional have made our coding experience smoother and more intuitive.
By understanding and leveraging these features, developers can build more efficient and maintainable Java applications. For those looking to elevate their Java skills, embracing Java 9's capabilities is certainly a step in the right direction.
Further Reading
Embrace the journey of Java 9, and let its new features empower your coding!
Checkout our other articles