Avoiding Data Duplication: The Simple vs Easy Dilemma
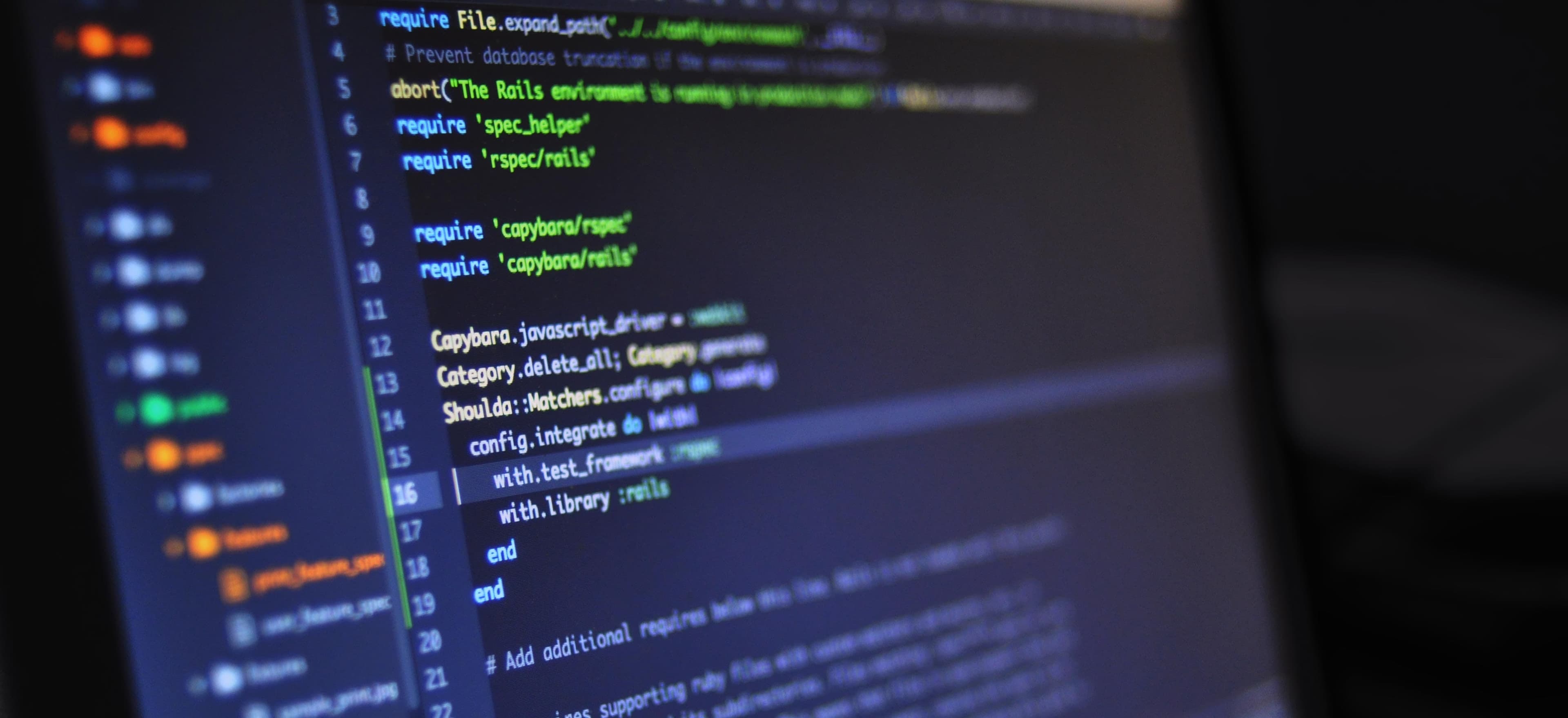
- Published on
Avoiding Data Duplication: The Simple vs Easy Dilemma
In software development, particularly in Java applications, data duplication is an ever-present challenge. It can lead to performance issues, difficulty in maintaining data integrity, and increased storage costs. Developers often find themselves in a dilemma: opt for simple solutions or choose what may seem easier but is ultimately more complex. In this blog post, we will explore how to avoid data duplication in Java applications, examining the simple versus easy dilemma along the way.
Understanding Data Duplication
Data duplication occurs when the same piece of data is stored in multiple places. This can happen due to various reasons, such as poor database design, a lack of proper data validation, or the absence of a centralized data management strategy. Duplicate data can result in inconsistencies, making it difficult to trust the data being processed.
Why is Data Duplication a Problem?
- Increased Storage Costs: Every duplicated entry takes up valuable storage space.
- Data Inconsistency: Different copies of the same data may get updated at different times, leading to confusion and error-prone applications.
- Poor Performance: Queries that have to sift through duplicated records can become slower and less efficient.
- Complexity in Maintenance: Fixing bugs or improving features in an environment with data duplication can be difficult since the same data might need to be changed in multiple places.
The Simple vs Easy Dilemma
The "Simple" Approach
The simple approach entails thorough planning and the use of best practices in application design. This often requires more upfront work but can save significant time and resources in the long run.
Example Strategy:
- Database Normalization: Reducing data redundancy through normalization is a well-established practice that organizes data within a database efficiently.
// A SQL example for creating a normalized database table
CREATE TABLE Users (
user_id SERIAL PRIMARY KEY,
username VARCHAR(50) UNIQUE NOT NULL,
email VARCHAR(100) UNIQUE NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
This structure ensures that each user entry is unique and prevents duplication. The use of UNIQUE
constraints guarantees that the same username or email cannot be added twice.
The "Easy" Approach
On the other hand, the easy approach often focuses on quick fixes or workarounds. While this may seem more convenient at first, it frequently introduces complexity later on, especially if data duplication issues recur.
Example Strategy:
- Basic Checks in Application Logic: Using conditions in your Java application to check for duplicates before adding records.
public void addUser(String username, String email) {
// Assuming we have a method to query if a user exists
if (!userExists(username, email)) {
// Proceed to add user
// addUserToDatabase(username, email);
} else {
// Log a warning or notify the user
System.out.println("User already exists.");
}
}
public boolean userExists(String username, String email) {
// Query logic to check for existence
}
While this may appear to solve the problems, it can easily become complicated as business logic grows. As your application scale, maintaining checks in every place where data is manipulated can lead to fragile code that is difficult to debug.
Best Practices to Avoid Data Duplication
1. Normalize Your Database
As previously mentioned, database normalization is critical. It involves organizing the columns and tables of a database to ensure that dependencies are properly enforced, reducing redundancy.
2. Use Unique Constraints
Always define unique constraints on columns where duplication should not occur. This will prevent duplicate entries at the database level.
3. Employ Transaction Management
Utilizing transactions ensures that your operations either complete successfully or fail completely, maintaining data integrity. When working in a multi-user environment where concurrent modifications may occur, transaction management becomes crucial.
try (Connection conn = DriverManager.getConnection(DB_URL)) {
conn.setAutoCommit(false);
// Execute queries here
conn.commit();
} catch (SQLException e) {
// Handle error, rollback transaction
}
In this code, we utilize a transaction block to ensure that if any part of the operation fails, the entire transaction is rolled back, preserving database integrity.
4. Implement Application Logic for Checking Duplicates
In scenarios where unique constraints may not suffice, leveraging application logic to check for duplicates before data insertion can act as an additional safeguard.
5. Stay Consistent
Maintain a single source of truth for each piece of data. This often translates to having a primary database that serves all applications interacting with the same data.
6. Regular Cleanup
Periodically scanning for and removing duplicate records can help maintain data integrity. Deduplication scripts may be run as part of maintenance tasks.
The Bottom Line: Simple is More Effective Than Easy
In the context of avoiding data duplication in Java applications, simplicity often leads to effectiveness. Simple approaches call for structured methodologies, best practices, and proactive measures, while easy solutions may offer temporary fixes that complicate matters down the line.
As data management becomes more significant in our tech-driven world, understanding the implications of data duplication and actively choosing simpler methodologies will pay dividends in effectiveness and efficiency. For further reading on best practices in database design, you might want to check out Database Normalization Basics and Data Integrity in Java Applications.
By adopting simple strategies and being aware of potential pitfalls, developers can create robust applications that uphold data integrity, scalability, and performance without falling into the trap of easy but convoluted solutions.
Happy coding!
Checkout our other articles