Common Pitfalls When Creating Your Own CDI Extension
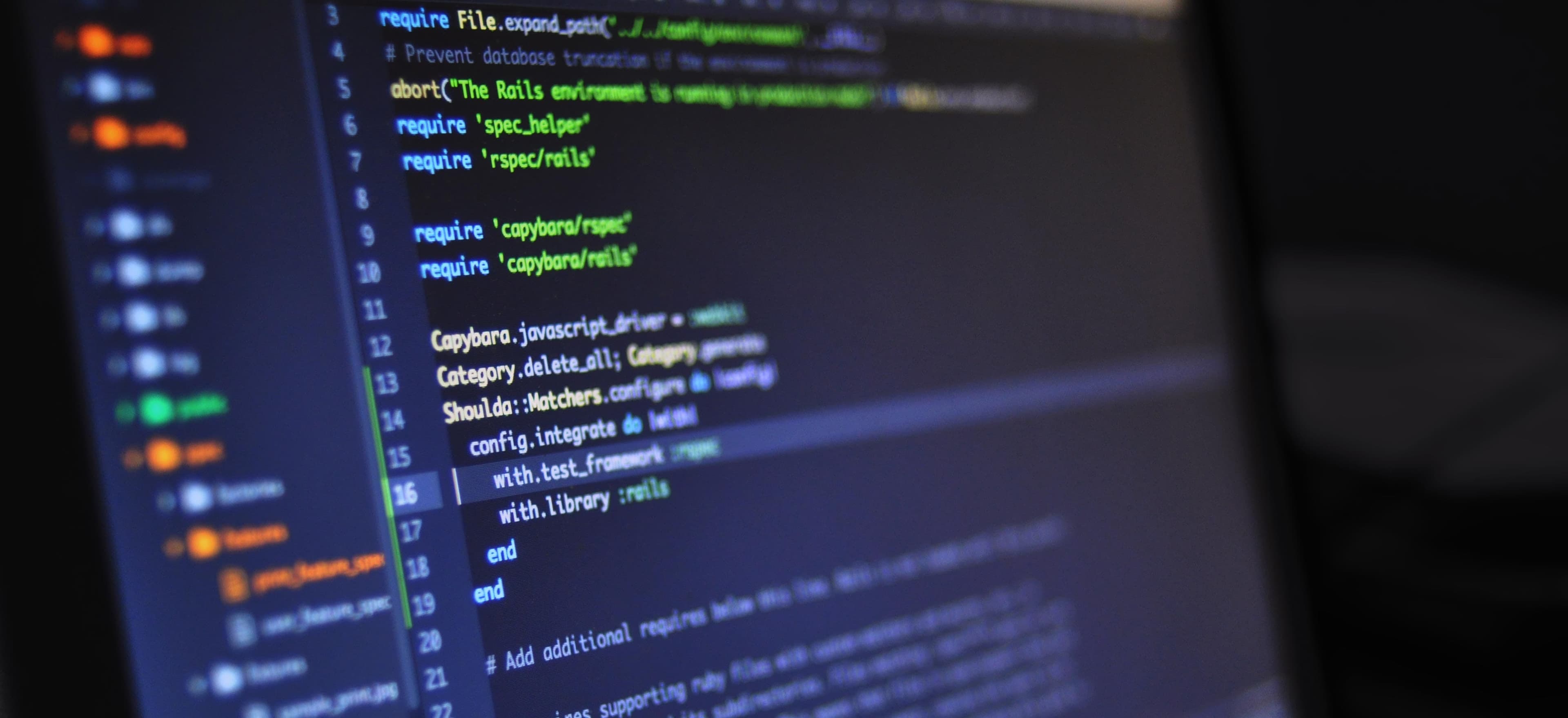
- Published on
Common Pitfalls When Creating Your Own CDI Extension
Creating a Context and Dependency Injection (CDI) extension can significantly enhance your Java EE or Jakarta EE applications. However, the journey towards building a robust and effective CDI extension can be fraught with pitfalls. In this article, we will discuss common mistakes developers make and how to avoid them. This guide is beneficial for anyone looking to integrate CDI extensions into their applications more effectively.
What is CDI?
CDI, or Contexts and Dependency Injection, is a powerful framework that manages the lifecycle of beans and their dependencies in a Java application. By utilizing CDI, developers can write cleaner, more structured code, promoting reusability and maintainability.
CDI extensions allow developers to extend the lifecycle and behavior of the CDI container. When mismanaged, these extensions can lead to performance issues, unexpected behaviors, and even runtime exceptions.
Key Pitfalls to Avoid
-
Insufficient Understanding of CDI Concepts
Before diving into creating extensions, it's crucial to have a solid grasp of CDI concepts like Observers, Producers, and Scopes. Skipping this foundational knowledge may lead to misconceptions and faulty implementations.
Tip: Read the official CDI documentation to solidify your understanding of its core concepts.
-
Overcomplicating the Extension Logic
Simplicity is paramount. Overly complicated logic in CDI extensions may lead to obscure behaviors in bean management and performance issues.
Here's a simplified example of a producer method:
public class MyProducer { @Produces public MyBean createMyBean() { // Simple instantiation without complex logic return new MyBean(); } }
Why: This code avoids unnecessary complexity, enabling clear and maintainable producer methods.
-
Neglecting Bean Scoping
One common oversight is mishandling the scoping of beans. Failing to specify the correct scope can lead to resource leaks or inconsistent states.
For instance, using a
@SessionScoped
bean within a singleton context can cause unintended behavior:@Singleton public class ApplicationBean { @Inject private MySessionScopedBean sessionScopedBean; // Incorrect }
Why: This code snippet demonstrates an incorrect operation, where a session-scoped bean is injected into a singleton bean, leading to unexpected lifecycle issues.
-
Ignoring the Importance of Decorators and Interceptors
Some developers overlook the purpose and utility of decorators and interceptors. Utilizing these can significantly improve cross-cutting concerns like logging or transaction management.
Consider this simple example of an interceptor:
@InterceptorBinding @Target({ METHOD, TYPE }) @Retention(RUNTIME) public @interface Logged {} @Logged @Interceptor public class LoggingInterceptor { @AroundInvoke public Object logMethod(InvocationContext context) throws Exception { System.out.println("Executing: " + context.getMethod().getName()); return context.proceed(); } }
Why: This code allows for very clean cross-cutting concerns where methods can be intercepted for logging without cluttering the business logic.
-
Lack of Testing
Testing your CDI extension is essential. Developers often treat extensions as secondary features and neglect testing, leading to bugs in production.
Utilize framework tools like Arquillian or JUnit for effective testing. For instance, here’s a simple unit test for a producer:
@RunWith(Arquillian.class) public class MyProducerTest { @Inject private MyBean myBean; @Deployment public static Archive<?> createDeployment() { return ShrinkWrap.create(JavaArchive.class) .addClass(MyProducer.class) .addClass(MyBean.class) .addAsManifestResource(BeanDiscoveryMode.ALL); } @Test public void testMyBeanNotNull() { assertNotNull(myBean); } }
Why: This ensures that your CDI extension behaves as expected and integrates well within the application context.
-
Neglecting Performance Implications
Extensions can lead to performance overhead if not carefully managed. Ensure that introduction of new features does not impede the CDI container's performance.
For instance, too many observers can cause excessive processing time during events. Mitigate these issues by batching or deferring work when possible.
-
Relying on Specific Implementations
When developing CDI extensions, it's tempting to rely on specific container implementations. This may limit your extension's portability.
Instead, aim to adhere to the CDI specification to ensure maximum compatibility:
@Alternative public class MyAlternativeBean implements MyInterface { // implementation details }
Why: Using alternatives allows you to switch implementations without making substantial code changes in your consumers.
Best Practices for Building CDI Extensions
-
Documentation and Clarity
Make sure to document your extension. Provide clear instructions on its setup, usage, and limitations. A well-documented extension is far easier to adopt.
-
Use CDI Annotations Regularly
Leverage CDI annotations effectively. This ensures any developer can see the intentions and contexts of your beans.
-
Follow SOLID Principles
By adhering to the SOLID principles of object-oriented design, you ensure that your extension is both maintainable and extensible.
-
Monitor Performance
Implement performance logging and monitoring to catch potential slowdowns early. Tools like VisualVM can help identify bottlenecks in your CDI extension.
The Last Word
Creating your own CDI extension can empower your Java application with additional functionality and streamlined processes. However, avoiding common pitfalls is critical to ensure success. By understanding CDI fundamentals, maintaining simplicity, utilizing decorators and interceptors, testing thoroughly, and heeding performance considerations, you can create robust, effective extensions.
For further reading, consider delving into resources such as the CDI 2.0 Specification or related tutorials on how to enhance your Java ecosystem with dependency injection patterns.
With these guidelines and insights, you can confidently embark on your journey to develop powerful CDI extensions, boosting the maintainability and scalability of your applications while avoiding common errors.
Happy coding!