Transforming Twitter4J Events into RxJava Observables
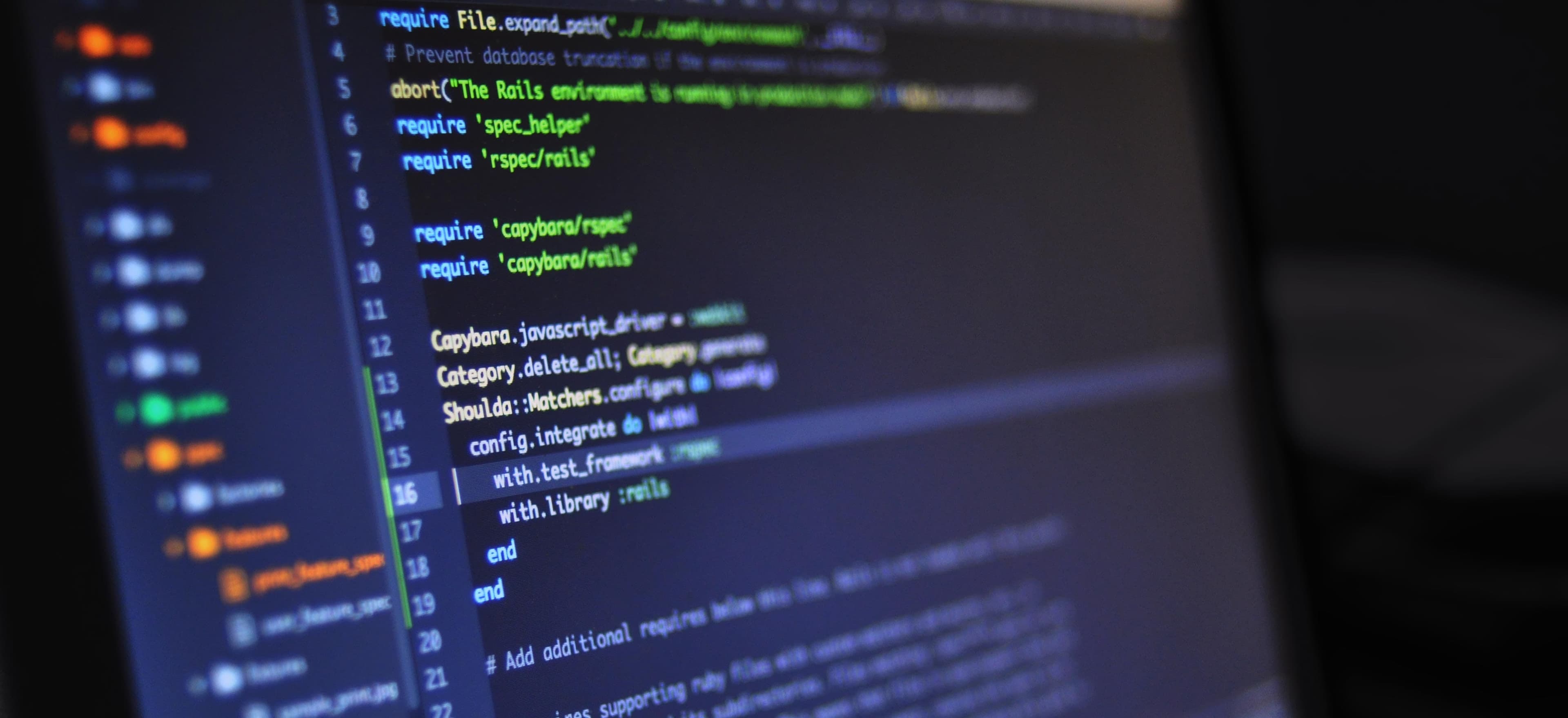
- Published on
Transforming Twitter4J Events into RxJava Observables
In the world of software development, the ability to handle real-time data streams has become vital. Twitter4J, a popular library for accessing the Twitter API, enables developers to consume Twitter events efficiently. Combined with RxJava, a powerful tool for reactive programming, we can create elegant and scalable applications that respond to Twitter events asynchronously.
In this blog post, we'll explore how to transform Twitter4J events into RxJava Observables. We will start by providing a brief overview of Twitter4J and RxJava, followed by a hands-on approach to building our event-driven solution. By the end of this post, you'll have a solid understanding of how to implement this transformation and how it can enhance your application.
What is Twitter4J?
Twitter4J is an unofficial Twitter API library written in Java. It allows you to interact with Twitter's RESTful API as well as the streaming API, making it easy for Java developers to send and receive data from Twitter. By providing a simple interface, Twitter4J helps developers manage tweets, user profiles, trends, and more.
Key Features of Twitter4J:
- Access to the REST API and Streaming API.
- Support for various data formats, including JSON.
- Easy handling of Twitter's rate limits and errors.
For more information on Twitter4J, visit its official documentation.
What is RxJava?
RxJava is a reactive programming library for Java, designed to simplify asynchronous programming. By allowing developers to compose asynchronous and event-based programs using observable sequences, RxJava makes it easier to deal with data as it arrives in real time.
Key Concepts of RxJava:
- Observables: They send notifications (or emissions) to subscribers.
- Observers: They react to the data emitted by observables.
- Schedulers: Used to control the execution of observables and observers in different threads.
For a comprehensive introduction to RxJava, you can refer to its official documentation.
Why Combine Twitter4J with RxJava?
By merging Twitter4J and RxJava, we can efficiently manage asynchronous event streams. Twitter4J can produce events for tweets, retweets, and more, while RxJava can help us handle these events in a responsive manner without blocking the main thread.
Setting Up Your Project
-
Add Dependencies: To use Twitter4J and RxJava, you need to add their dependencies to your project. If you're using Maven, include the following in your
pom.xml
:<dependency> <groupId>org.twitter4j</groupId> <artifactId>twitter4j-core</artifactId> <version>4.0.7</version> <!-- Update to the latest version --> </dependency> <dependency> <groupId>io.reactivex.rxjava2</groupId> <artifactId>rxjava</artifactId> <version>2.2.20</version> <!-- Update to the latest version --> </dependency>
-
Configuration: Before you can receive events, you need to set up your Twitter API credentials. Create a
twitter4j.properties
file in your resources directory with the following format:oauth.consumerKey=YOUR_CONSUMER_KEY oauth.consumerSecret=YOUR_CONSUMER_SECRET oauth.accessToken=YOUR_ACCESS_TOKEN oauth.accessTokenSecret=YOUR_ACCESS_TOKEN_SECRET
Creating an Observable for Twitter Events
Let's dive into the code and create an Observable that listens for Twitter events.
Step 1: Setting Up the Twitter Stream
Create a TwitterStream
instance to listen for events. Here is an example implementation:
import twitter4j.*;
import io.reactivex.Observable;
import io.reactivex.subjects.PublishSubject;
public class TwitterEventStream {
private final TwitterStream twitterStream = new TwitterStreamFactory().getInstance();
private final PublishSubject<Status> tweetSubject = PublishSubject.create();
public TwitterEventStream() {
twitterStream.addListener(new StatusListener() {
@Override
public void onStatus(Status status) {
tweetSubject.onNext(status); // Emit the received status
}
// Other overridden methods are omitted for brevity
});
}
public Observable<Status> getObservable() {
return tweetSubject; // Return the observable to subscribers
}
public void startStreaming() {
twitterStream.sample(); // Start listening to the sample stream
}
public void stopStreaming() {
twitterStream.shutdown(); // Stop the stream
}
}
Explanation
- TwitterStream Instance: We create an instance of
TwitterStream
that connects to Twitter's streaming API. - PublishSubject: We leverage
PublishSubject
from RxJava to emitStatus
objects (tweets) whenever we receive them. - StatusListener: We implement the
StatusListener
interface to define what happens when we receive a tweet. TheonStatus
method is where we emit the tweet via our subject.
Step 2: Consuming the Observable
Now that we have our TwitterEventStream
class set up, we can subscribe to its observable:
public class Main {
public static void main(String[] args) {
TwitterEventStream twitterStream = new TwitterEventStream();
twitterStream.getObservable()
.subscribe(
tweet -> System.out.println("Received tweet: " + tweet.getText()),
throwable -> System.err.println("Error: " + throwable)
);
twitterStream.startStreaming();
}
}
Explanation
- Subscription: We subscribe to the observable and provide a lambda function to handle the emitted
Status
objects. In this case, we simply print the tweet text to the console. We can also log any errors in the subscription with the appropriate error handling method.
Handling Different Types of Events
The above example focuses solely on Status
updates (tweets). However, Twitter4J provides various events like onException
, onDeletionNotice
, and others that can be useful in real-time applications.
You can easily enhance your TwitterEventStream
class to emit these events by extending your observable to include additional subjects for each type of event.
Example of Handling User Events
twitterStream.addListener(new UserListListener() {
@Override
public void onUserListMemberAddition(UserList userList, User user) {
userListSubject.onNext(user); // Emit user event
}
// Other overridden methods are omitted for brevity
});
This approach enables your application to handle various types of Twitter events, making your application robust and event-driven.
In Conclusion, Here is What Matters
Combining Twitter4J and RxJava allows developers to create responsive applications that handle Twitter events asynchronously. By transforming Twitter4J events into RxJava observables, we can simplify the management of real-time data streams, enabling us to build powerful features based on Twitter data.
In this post, we've covered the essentials: from setting up your project to building an event stream that reacts to Twitter events. For more advanced use cases, consider integrating multiple event types and enhancing the error handling capabilities of your observables.
Further Reading
- Explore more about Reactive Programming.
- Dive deeper into Twitter4J features.
- Check out the RxJava tutorial for practical examples and best practices.
If you have any questions or want to share your experiences with Twitter4J and RxJava, feel free to leave a comment below! Happy coding!
This blog post serves as a guide to effectively leveraging Twitter4J events in a reactive context, providing a clear framework for developers looking to adopt these technologies in their Java applications.