Mastering Java 8 Streams: Avoiding Common Pitfalls
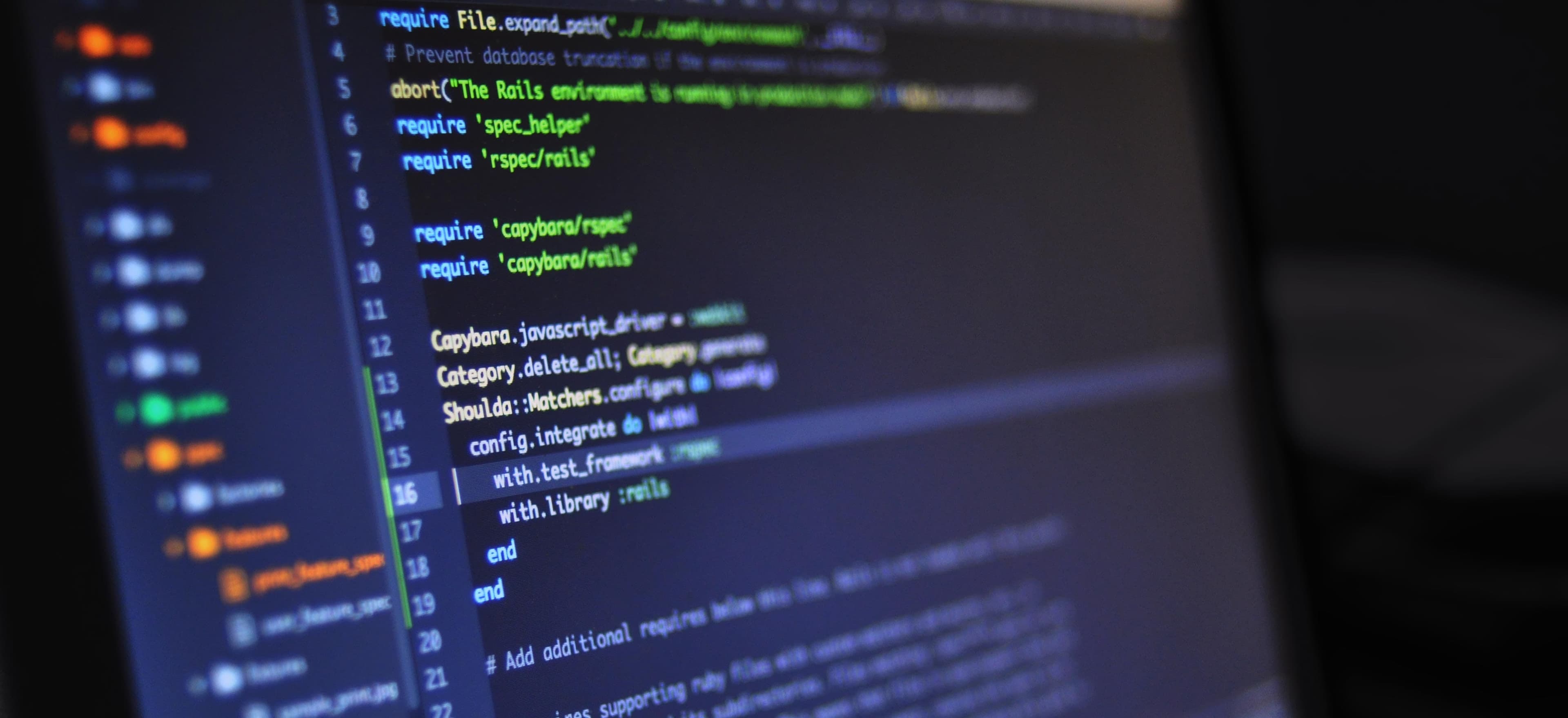
- Published on
Mastering Java 8 Streams: Avoiding Common Pitfalls
Java 8 introduced a powerful new abstraction for handling collections of data: the Stream API. This new feature allows developers to express complex data processing queries in a clear and concise manner. While the Stream API offers great benefits, it can also lead to some common pitfalls. This blog post will walk you through these pitfalls and provide guidance on how to avoid them while mastering the Stream API in Java 8.
Understanding the Basics of Streams
Before diving into common pitfalls, let's quickly summarize what a stream is. A stream represents a sequence of elements that can be processed in parallel or sequentially. Streams can be created from various data sources, including collections, arrays, or I/O channels.
Creating Streams
You can easily create a stream from a collection like so:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Stream;
public class StreamExample {
public static void main(String[] args) {
List<String> list = Arrays.asList("apple", "banana", "cherry");
Stream<String> stream = list.stream(); // Creating a stream from a list
stream.forEach(System.out::println); // Print each element
}
}
In this code snippet, we create a stream from a list of strings and print each element. This is a basic yet powerful operation you can perform with Java streams.
Common Pitfalls
1. Not Understanding Intermediate vs. Terminal Operations
One of the most common mistakes is confusing intermediate and terminal operations. Intermediate operations (like map
, filter
, and sorted
) are lazy and not executed until a terminal operation (like forEach
, collect
, or reduce
) is invoked.
Example of the Issue
import java.util.Arrays;
import java.util.List;
public class CommonPitfall {
public static void main(String[] args) {
List<String> list = Arrays.asList("apple", "banana", "cherry");
// Intermediate operations
list.stream()
.filter(s -> s.startsWith("a"))
.map(String::toUpperCase);
// No terminal operation is invoked, so nothing happens!
}
}
In the above code, the use of filter
and map
does nothing because we forgot to include a terminal operation. To fix this, we can add a terminal operation like collect
:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class CommonPitfall {
public static void main(String[] args) {
List<String> list = Arrays.asList("apple", "banana", "cherry");
List<String> result = list.stream()
.filter(s -> s.startsWith("a"))
.map(String::toUpperCase)
.collect(Collectors.toList()); // Correct usage with terminal operation
System.out.println(result); // Prints: [APPLE]
}
}
2. Modifying Collections During Stream Operations
Another common pitfall is the unsafe modification of collections during stream operations. Streams are designed to be functional and stateless, meaning you should not modify the underlying collection while processing it.
Example of the Issue
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class ModifyDuringStream {
public static void main(String[] args) {
List<String> list = new ArrayList<>(Arrays.asList("apple", "banana", "cherry"));
// Modifying the collection while streaming
list.stream()
.filter(s -> {
if (s.startsWith("a")) {
list.remove(s); // This can lead to ConcurrentModificationException
}
return true;
})
.forEach(System.out::println);
}
}
Here, attempting to remove items from the list while processing it with a stream leads to unpredictable behavior. Instead, you can create a new collection with the results you want to keep:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class ModifyDuringStream {
public static void main(String[] args) {
List<String> list = new ArrayList<>(Arrays.asList("apple", "banana", "cherry"));
// Correct way: Filter and collect to a new list
List<String> filteredList = list.stream()
.filter(s -> !s.startsWith("a")) // Remove elements starting with "a"
.collect(Collectors.toList()); // Create a new filtered list
System.out.println(filteredList); // Prints: [banana, cherry]
}
}
3. Ignoring Parallel Streams
When working with large datasets, you might be tempted to use parallel streams for better performance. However, parallel streams can introduce challenges, especially when dealing with mutable shared state. Before using them, consider whether your operation is thread-safe and does not introduce side effects.
Proper Usage of Parallel Streams
import java.util.Arrays;
import java.util.List;
public class ParallelStreamExample {
public static void main(String[] args) {
List<String> list = Arrays.asList("apple", "banana", "cherry");
// Using parallel stream for increased performance
long count = list.parallelStream()
.filter(s -> s.startsWith("b"))
.count();
System.out.println("Count of fruits starting with 'b': " + count);
}
}
In this example, a parallel stream is used to count fruit names starting with the letter 'b'. This approach can significantly improve performance for large collections but should be used carefully to avoid issues related to thread safety.
4. Returning Collections Directly from Streams
Another common mistake is returning mutable collections directly from stream operations, which can lead to unintended side effects.
Example of the Issue
import java.util.Arrays;
import java.util.List;
public class DirectReturnFromStream {
public static void main(String[] args) {
List<String> list = Arrays.asList("apple", "banana", "cherry");
List<String> result = list.stream()
.filter(s -> s.startsWith("b"))
.collect(Collectors.toList());
// Mutating the returned list
result.add("blueberry");
System.out.println(result); // This is fine, but be careful with the original list!
}
}
If you're returning a mutable list and subsequently modifying it, be aware that your changes may inadvertently affect your original collection or other parts of your code. It's often wise to return an unmodifiable collection, especially when exposing it outside your methods.
import java.util.Collections;
import java.util.List;
import java.util.Arrays;
import java.util.stream.Collectors;
public class CorrectUnmodifiableReturn {
public static List<String> getFruits() {
List<String> list = Arrays.asList("apple", "banana", "cherry");
// Return an unmodifiable list
return Collections.unmodifiableList(
list.stream()
.filter(s -> s.startsWith("b"))
.collect(Collectors.toList())
);
}
public static void main(String[] args) {
List<String> fruits = getFruits();
// This will throw UnsupportedOperationException
fruits.add("blueberry");
}
}
Final Considerations
In this article, we covered common pitfalls to avoid while working with Java 8 streams. Understanding the differences between intermediate and terminal operations, avoiding concurrent modifications, recognizing when to use parallel streams, and making wise design choices about returning collections will help you write better, more efficient code.
For more in-depth reading on Java 8 Streams, consider checking out the official Java documentation on Streams.
By mastering these concepts and avoiding these pitfalls, you will significantly enhance your Java programming skills and utilize the full power of the Java 8 Stream API.
Feel free to reach out with questions or additional topics you'd like to explore in relation to Java and the Stream API! Happy coding!
Checkout our other articles