Mastering JavaScript for Enhanced CSS Battle Performance
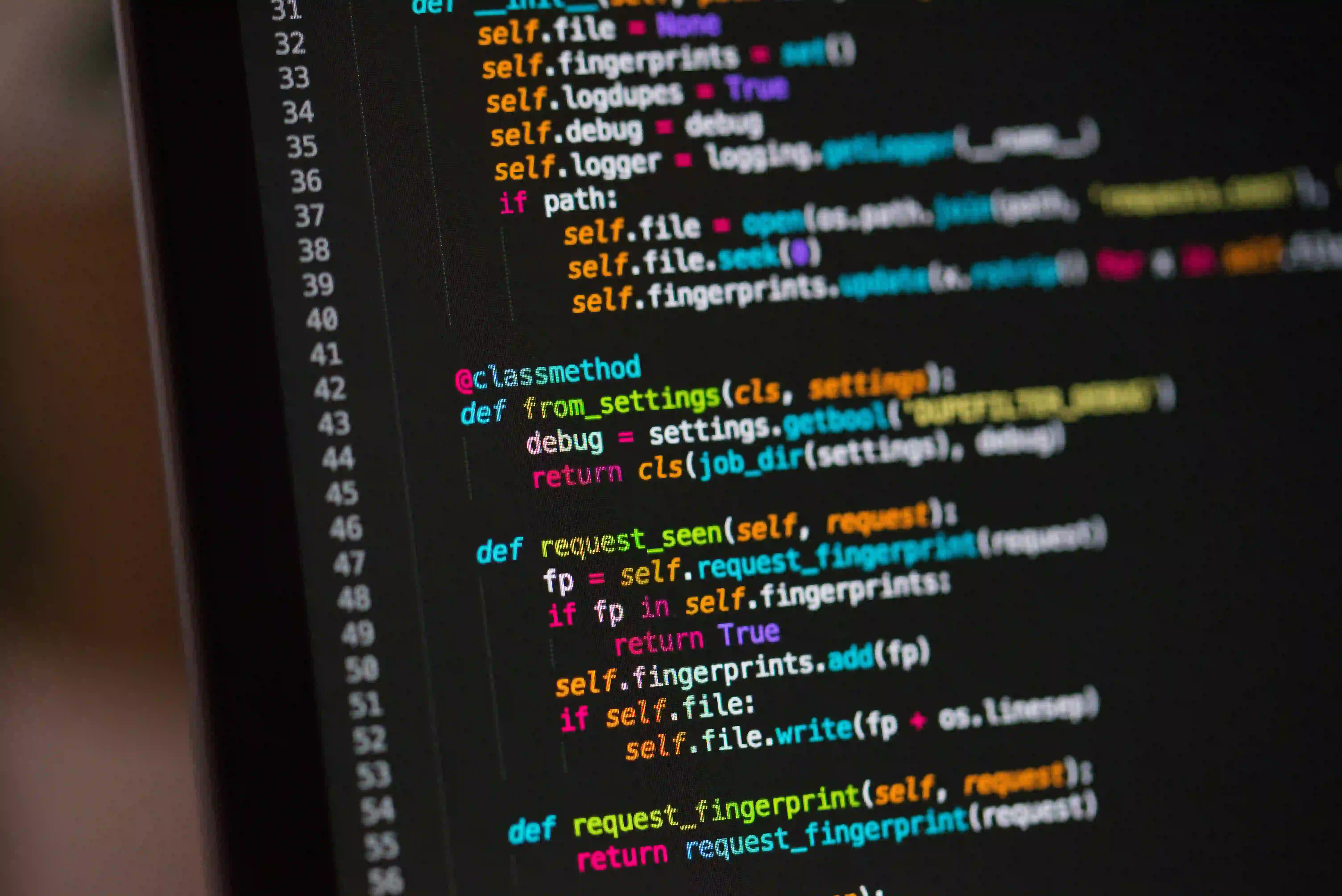
Mastering JavaScript for Enhanced CSS Battle Performance
In the world of web development, CSS Battle is an exciting platform for honing your CSS skills, allowing developers to challenge themselves and others in creating visually stunning user interfaces. However, there’s an unexpected ally that can complement your CSS prowess—JavaScript. If you learn to blend these two languages effectively, you'll not only elevate your performance but also boost your CSS Battle scores.
In this blog post, we'll explore how mastering JavaScript can enhance your performance in CSS Battle challenges. We’ll dissect several strategies, provide relevant code snippets, and conclude with some actionable tips to help you become a formidable CSS Battle competitor.
The Relationship Between JavaScript and CSS
JavaScript is an essential tool that enables dynamic interactions within web pages. While CSS is responsible for presentation and layout, JavaScript adds interactivity. Understanding how to leverage JavaScript can take your CSS skills to the next level, allowing you to create engaging, responsive designs that adapt to user actions.
Example Concept: Dynamic Style Manipulation
One of the primary uses of JavaScript in conjunction with CSS is dynamic style manipulation. With JavaScript, you can change CSS properties on the fly. This technique is particularly beneficial in CSS competitions when aiming to create responsive designs.
Here’s a quick example:
// Select the element by its ID
const box = document.getElementById('colorBox');
// Function to change background color
function changeColor(color) {
box.style.backgroundColor = color; // Dynamically changes the color
}
// Event listener for mouseover
box.addEventListener('mouseover', () => {
changeColor('#ff5733'); // Changes to a specific color on hover
});
// Event listener for mouseout
box.addEventListener('mouseout', () => {
changeColor('#34eb7e'); // Changes back on mouse out
});
Why Use Dynamic Style Manipulation?
Using dynamic style manipulation fosters an interactive environment for users. Instead of static designs, achieving fluid transitions and color changes can give your CSS designs the edge they need to stand out in CSS Battle competitions.
Enhancing User Experience (UX)
Improving user experience is another area where JavaScript shines. By incorporating interactive elements, you can make your designs not only visually appealing but also functional. Consider adding animations, transitions, or real-time updates based on user input.
Example Concept: Using setInterval for Animation
You can employ JavaScript's setInterval
function to create continuous animations or changes that keep users engaged.
// Select element
const animateDiv = document.getElementById('animate');
// Start animation
let position = 0;
const intervalId = setInterval(() => {
if (position >= 100) {
clearInterval(intervalId); // Stop the animation
} else {
position++;
animateDiv.style.transform = `translateX(${position}px)`; // Move element to the right
}
}, 20); // Runs every 20 milliseconds
Why Include Animation in Your Designs?
Animations lead to a more dynamic web experience, enticing users to engage with the content. This increases the likelihood of achieving higher scores in CSS Battle, showcasing your ability to integrate motion seamlessly into your designs.
Leveraging JavaScript Libraries
For CSS Battles, utilizing JavaScript libraries can enhance both your design capabilities and coding efficiency. Libraries such as jQuery or GSAP provide High-level functions that simplify tasks that would otherwise take considerable amount of lines in vanilla JavaScript.
Here's a brief example using jQuery to change CSS properties:
$(document).ready(function() {
$('#animationBox').hover(
function() {
$(this).css('background-color', '#ff5733'); // On hover
},
function() {
$(this).css('background-color', '#34eb7e'); // On mouse out
}
);
});
Why Use JavaScript Libraries?
These libraries can often shorten your code and increase readability. In a competition setting, simplicity and clarity can be just as essential as functionality. Plus, knowing how certain libraries operate can give you an edge during rapid development. For those looking to dive deeper into strategies for improving CSS performance, you might find useful insights in this existing article: Boost Your CSS Battle Scores: 4 Proven Strategies.
Asynchronous Programming
Another powerful aspect of JavaScript is its asynchronous nature, allowing for fetching data or responding to events without blocking the main execution thread. In the context of CSS Battle, you could use asynchronous code to load styles or configurations dynamically.
Here's a simple example that fetches a JSON object and applies styles from it:
async function loadStyles() {
const response = await fetch('styles.json');
const styles = await response.json(); // Assume it returns an object with CSS properties
const element = document.getElementById('dynamicStyle');
for (const [property, value] of Object.entries(styles)) {
element.style[property] = value; // Apply each style
}
}
loadStyles();
Why Asynchronous Programming Is Important?
In CSS Battle scenarios, loading styles or configurations on-demand can enhance performance and allow you to adapt your design based on user preference or external data. It gives you more flexibility to manage resources efficiently.
Keeping Your Code Clean and Maintainable
While it's tempting to write complex JavaScript to achieve eye-catching CSS, maintaining clarity and cleanliness is vital. Use comments effectively and maintain a coherent style throughout your code. It not only demonstrates professionalism but aids in troubleshooting during competitions.
Here’s a concise way to comment your code:
// Change the background color based on user interaction
document.getElementById('box').addEventListener('click', function() {
this.style.backgroundColor = '#ffeb3b'; // Change color to yellow on click
});
Best Practices for Clean Code
- Consistent Naming Conventions: Use clear and descriptive names for functions and variables.
- Comment Strategically: Keep comments relevant and succinct, explaining 'why' rather than 'what'.
- Limit Complexity: Break down complicated functions into smaller, manageable ones.
The Closing Argument: Bringing It All Together
Mastering JavaScript is a secret weapon in your CSS Battle arsenal. By incorporating dynamic interactions, leveraging libraries, utilizing asynchronous programming, and maintaining clean code, you pave the way for higher scores and a more engaging user experience.
By understanding the synergy between JavaScript and CSS, you can develop innovative and interactive designs that captivate users and judges alike. Remember, it’s not just about executing code; it’s about creating an experience. The tips and strategies discussed herein are stepping stones to elevate your skills bravely.
For more tips on CSS strategies, ensure to check out Boost Your CSS Battle Scores: 4 Proven Strategies to continue your journey toward mastery! Happy coding!