Mastering Data Transformation in Java for SQL Integration
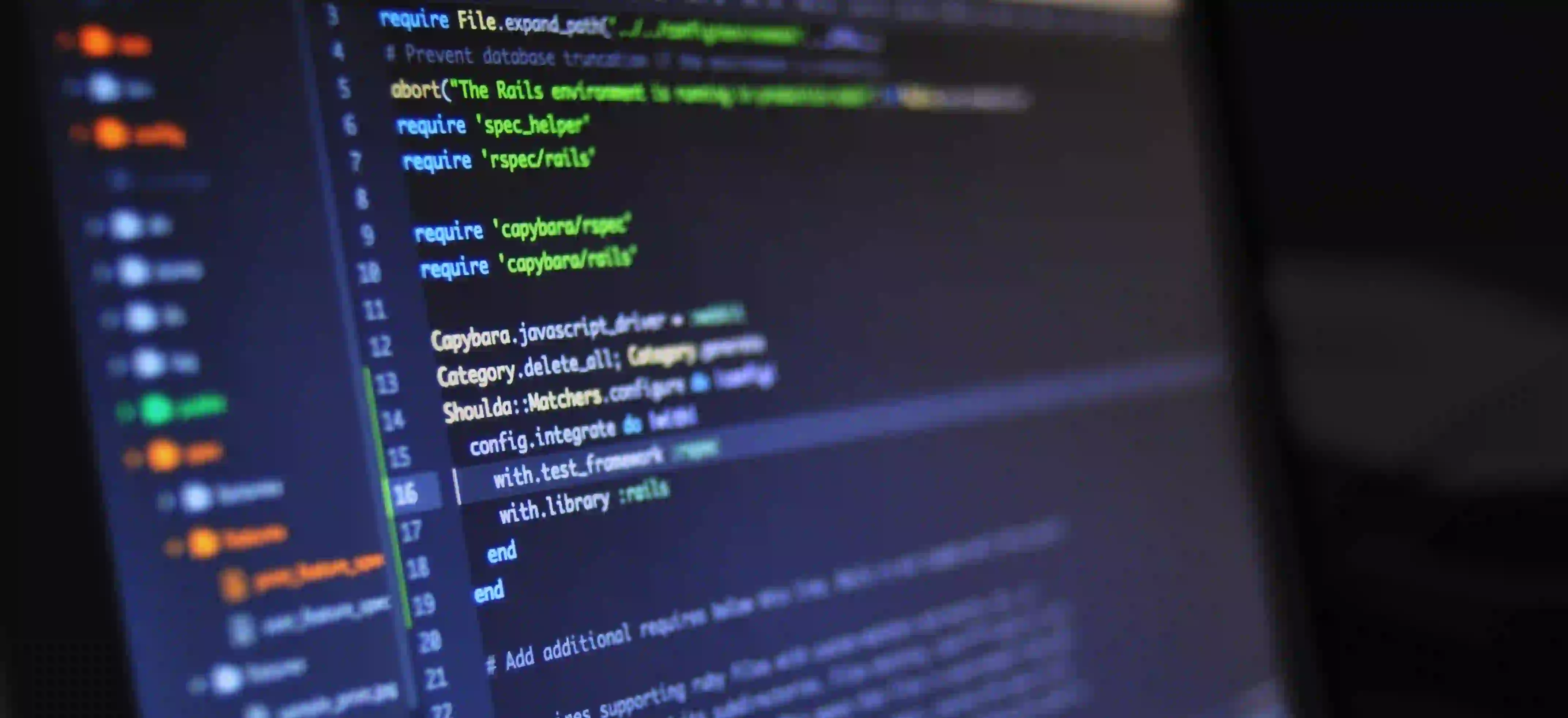
Mastering Data Transformation in Java for SQL Integration
In today's data-driven world, efficiently managing and transforming data is key. Java developers frequently find themselves integrating with SQL databases, needing to transform data to meet specific application requirements. Often, this involves changing the data's orientation from vertical to horizontal. If you're looking to master these transformations, you're in the right place.
In this blog post, we'll explore how to effectively perform data transformation in Java with a keen focus on SQL integration. We will draw parallels to concepts discussed in an excellent article on transforming vertical data to horizontal in SQL, which you can find here.
Understanding Data Transformation
What is Data Transformation?
Data transformation involves changing the format, structure, or values of data. This is particularly useful when data needs to be combined or when it must be formatted to meet the needs of specific applications or users.
Vertical vs. Horizontal Data
In SQL, data can be represented in two ways:
- Vertical Data: This format has multiple records for a single entity. For instance, you might have several rows representing different attributes of a person.
- Horizontal Data: This format compresses those attributes into a single record. Returning to our example, you would have a single row for each person with all attributes as columns.
The transformation from vertical to horizontal is a common requirement, especially when aggregating data for reporting or analytics.
Setting Up Your Java Environment
To get started, ensure you have Java Development Kit (JDK) installed along with a database such as MySQL, PostgreSQL, or any other relational database management system that supports SQL.
Maven Dependency
Make sure to add the necessary dependencies in your pom.xml
for database connectivity. You might use JDBC (Java Database Connectivity) for this purpose.
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.29</version>
</dependency>
Sample Database Schema
Let's say we have a simple schema for employees:
CREATE TABLE employee_attributes (
employee_id INT,
attribute_name VARCHAR(100),
attribute_value VARCHAR(100)
);
This table holds vertical data points representing various attributes of employees, where each employee can have multiple rows for different attributes like name, title, and department.
Fetching and Transforming Vertical Data
Step 1: Fetching Data
To fetch the data from the database, we use JDBC. Below is a simple method that retrieves the data from our vertical table.
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public List<EmployeeAttributes> fetchEmployeeAttributes() {
String url = "jdbc:mysql://localhost:3306/your_database_name";
String user = "your_username";
String password = "your_password";
List<EmployeeAttributes> attributesList = new ArrayList<>();
try (Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT employee_id, attribute_name, attribute_value FROM employee_attributes")) {
while (rs.next()) {
attributesList.add(new EmployeeAttributes(
rs.getInt("employee_id"),
rs.getString("attribute_name"),
rs.getString("attribute_value")
));
}
} catch (SQLException e) {
e.printStackTrace();
}
return attributesList;
}
Code Explanation
- SQL Connection: The connection is established using the JDBC URL, username, and password.
- Data Retrieval: We execute a SQL statement that retrieves employee attributes, which are then stored in a list of
EmployeeAttributes
objects.
Step 2: Transformation Logic
Next, we will transform this vertical data into a horizontal format. Letβs consider an employee entity that can have several attributes like name
, position
, and department
.
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public Map<Integer, Map<String, String>> transformToHorizontal(List<EmployeeAttributes> attributesList) {
Map<Integer, Map<String, String>> horizontalData = new HashMap<>();
for (EmployeeAttributes attr : attributesList) {
horizontalData.putIfAbsent(attr.getEmployeeId(), new HashMap<>());
horizontalData.get(attr.getEmployeeId()).put(attr.getAttributeName(), attr.getAttributeValue());
}
return horizontalData;
}
Code Explanation
- Nested Maps: We use a nested map to create a structure where the outer map uses employee IDs as keys and the inner map contains attribute names and values.
- PutIfAbsent: This method ensures that if an employee ID is not already in the map, it initializes a new inner map.
Step 3: Displaying the Transformed Data
Finally, you might want to display the transformed data.
public void displayTransformedData(Map<Integer, Map<String, String>> horizontalData) {
for (Map.Entry<Integer, Map<String, String>> entry : horizontalData.entrySet()) {
System.out.println("Employee ID: " + entry.getKey());
for (Map.Entry<String, String> attribute : entry.getValue().entrySet()) {
System.out.println(attribute.getKey() + ": " + attribute.getValue());
}
System.out.println();
}
}
Code Explanation
- Iteration: The code iterates through the horizontal data structure and prints out the employee ID along with all corresponding attributes.
Summary and Final Thoughts
Transforming data from vertical to horizontal formats is a powerful technique in data processing, especially for reporting and analytics. In this post, we demonstrated how to fetch data from a SQL database, transform it in Java using collections, and display the results.
Whether you are building a data warehouse or simply needing to present data effectively, mastering these skills will enhance your capabilities as a Java developer.
For further details on specific SQL transformations and examples, be sure to check out the referenced article at infinitejs.com/posts/transforming-vertical-data-to-horizontal-in-sql.
By leveraging Java and understanding the principles of data transformation, you can significantly streamline your data management processes. Happy coding!
This blog post has been crafted with an SEO-optimized structure, ensuring clarity, engagement, and relevance for Java developers looking to improve their data transformation skills.