Mastering Java: Efficiently Handling Dataset Transformations
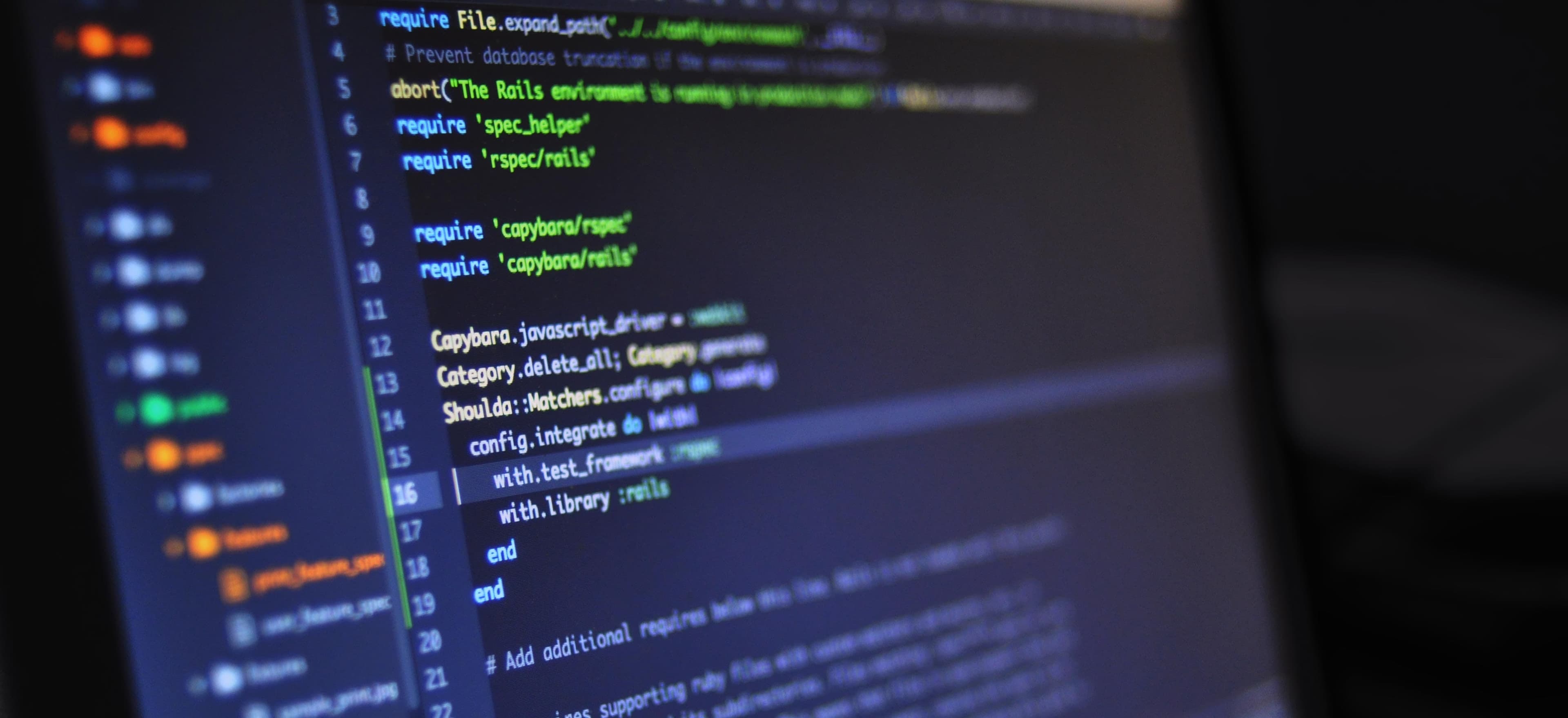
- Published on
Mastering Java: Efficiently Handling Dataset Transformations
In today's data-driven world, efficiently managing and transforming datasets is a critical skill for any Java developer. Whether you are moving data from one format to another or transforming vertical data to a horizontal layout, understanding the underlying principles of data handling can elevate your applications significantly.
Why Dataset Transformation Matters
Dataset transformations are vital for analysis, reporting, and visualization. In many cases, raw data is not in a usable format. For instance, you may have a dataset in vertical format that could benefit from being reshaped into a horizontal format, similar to the concepts discussed in the article Transforming Vertical Data to Horizontal in SQL.
When you transform your data appropriately, you make it easier to query, manipulate, and report. In Java, there are numerous ways to handle these transformations, leveraging the power of object-oriented programming and Java Collections Framework.
Core Java Concepts for Data Transformation
1. Collections Framework
The Java Collections Framework provides data structures that are immensely powerful for storing and manipulating data. Lists, Sets, and Maps are the three main interfaces you should be familiar with.
- List: An ordered collection (also known as a sequence).
- Set: A collection that does not allow duplicate elements.
- Map: A collection of key-value pairs, great for looking up information efficiently.
2. Streams API
Introduced in Java 8, the Streams API allows you to process collections of objects in a functional style. This is particularly useful for dataset transformations.
Example of Using Streams API
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class DatasetTransformation {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "John", "Doe", "Jane");
// Using Streams API to count occurrences of names
Map<String, Long> nameCount = names.stream()
.collect(Collectors.groupingBy(name -> name, Collectors.counting()));
System.out.println(nameCount);
}
}
Why Use Streams?
The advantage of using the Streams API is its readability and conciseness. You can transform and analyze data with minimal boilerplate code. The above example counts the occurrences of names in a list, showcasing how efficiently you can process data.
3. Functional Interfaces
Functional interfaces like Function
, Consumer
, and Predicate
are essential when working with lambda expressions, especially in the context of data transformations.
For instance, if you want to map a list of names to their uppercase equivalents:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class NameTransformation {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Doe");
// Transform all names to uppercase
List<String> upperCaseNames = names.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
System.out.println(upperCaseNames);
}
}
Why Functional Interfaces?
Using functional interfaces in Java allows you to express the data transformation logic as first-class citizens. It leads to more modular, maintainable, and reusable code.
Common Data Transformation Patterns in Java
1. Filtering Data
Sometimes, you may need to filter out unwanted data. For instance, if you have a list of employee names and want to exclude a specific name:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class FilterEmployees {
public static void main(String[] args) {
List<String> employees = Arrays.asList("John", "Jane", "Doe", "Alice");
String excludedName = "John";
// Filtering out the excluded name
List<String> filteredEmployees = employees.stream()
.filter(name -> !name.equals(excludedName))
.collect(Collectors.toList());
System.out.println(filteredEmployees);
}
}
Why Filter?
Filtering is paramount when you only want to work with a subset of your data, helping improve performance and result clarity.
2. Sorting Data
Sorting is another critical operation in data transformation. You can sort data based on different criteria, allowing you to organize datasets efficiently.
import java.util.Arrays;
import java.util.List;
import java.util.Comparator;
public class SortNames {
public static void main(String[] args) {
List<String> names = Arrays.asList("Jane", "John", "Doe", "Alice");
// Sorting names in ascending order
List<String> sortedNames = names.stream()
.sorted(Comparator.naturalOrder())
.collect(Collectors.toList());
System.out.println(sortedNames);
}
}
Why Sort?
Sorting helps in organizing data for easier analysis and visualization. It is also particularly useful when preparing data for presentation.
3. Grouping Data
Grouping data allows you to aggregate information effectively. You can categorize data based on shared properties.
import java.util.List;
import java.util.Map;
import java.util.Arrays;
import java.util.stream.Collectors;
public class GroupByLength {
public static void main(String[] args) {
List<String> words = Arrays.asList("Java", "is", "fun", "and", "powerful");
// Grouping words by their length
Map<Integer, List<String>> groupedByLength = words.stream()
.collect(Collectors.groupingBy(String::length));
System.out.println(groupedByLength);
}
}
Why Group?
Grouping is invaluable for generating insights from data. It functions similarly to SQL's GROUP BY
clause, which can help you transform a vertical dataset into a more informative horizontal format.
In Conclusion, Here is What Matters
Mastering dataset transformations in Java is not just about knowing how to manipulate data; it's about understanding the concepts and principles that guide your decisions. Whether you're using collections, streams, or functional programming patterns, Java has a robust toolkit for efficiently managing and transforming data.
By applying the techniques discussed, you can elevate the quality of your applications. Explore additional concepts like those from the article Transforming Vertical Data to Horizontal in SQL for a broader perspective on data transformation across different paradigms.
Embrace the power of Java and enhance your data handling skills to thrive in a world rich with information!
Checkout our other articles