Mastering Java Strings: Efficiently Split by Nth Character
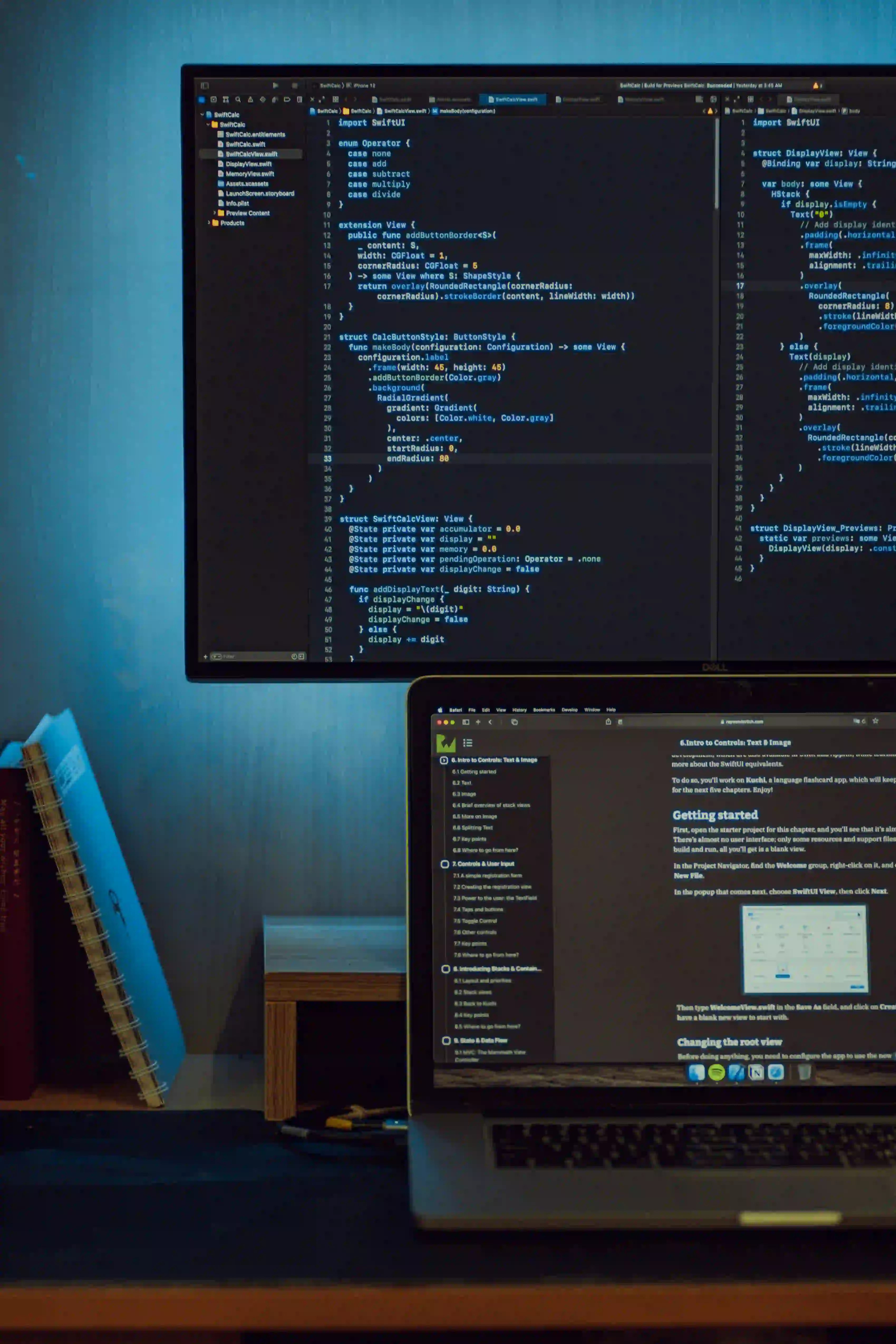
Mastering Java Strings: Efficiently Split by Nth Character
Java is a versatile programming language known for its robustness and agility. Among its many functionalities, string manipulation stands out as an essential skill for any developer. Splitting strings, particularly by the Nth character, is a common task that can streamline data processing and enhance code efficiency.
In this blog post, we will dive deep into how to efficiently split strings by the Nth character in Java. This will involve examining various methods, pros and cons of each, and illustrative code snippets that highlight the "why" behind our chosen implementations.
Understanding the Basics of String Splitting
Before we jump into the code, it's crucial to grasp the fundamental concept of splitting a string. When we talk about splitting by the Nth character, we are essentially dividing a string into substrings at defined intervals or positions.
Why Split a String?
- Data Parsing: When dealing with CSV files or structured data, splitting by characters like commas or semicolons can help us process and organize that data effectively.
- Text Processing: Applications that require word or character analysis benefit significantly from efficient string splitting.
- Improved Readability: Breaking down complex strings into smaller components enhances readability and manageability in the code.
Method 1: Using Regular Expressions
One of the most versatile tools in Java for string manipulation is regular expressions. The String.split()
method can be combined with a regex to split by Nth character.
Example Code: Using String.split()
public class RegExSplitter {
public static String[] splitStringByNthCharacter(String text, int n) {
// Validate the input
if (n <= 0) {
throw new IllegalArgumentException("n must be greater than 0");
}
// Creating a regex based on the Nth character
String regex = ".{1," + n + "}"; // Matches groups of N characters
// Split the string using the regex
return text.split(regex);
}
public static void main(String[] args) {
String input = "HelloWorld";
String[] result = splitStringByNthCharacter(input, 3);
for (String str : result) {
System.out.println(str);
}
}
}
Explanation
In the code snippet above:
.{1,n}
is a regex that matches any character one or more times, up to N characters.- We are using
String.split()
to split the string based on that pattern.
This approach is powerful, but there are performance considerations when handling large strings. Regular expressions can be relatively slow due to their complexity.
Method 2: Using StringBuilder
If performance is critical, especially with larger strings or numerous splits, consider using StringBuilder
combined with a conventional loop. This method avoids regex overhead.
Example Code: Using StringBuilder
import java.util.ArrayList;
import java.util.List;
public class StringBuilderSplitter {
public static List<String> splitStringByNthCharacter(String text, int n) {
List<String> result = new ArrayList<>();
// Validate the input
if (n <= 0) {
throw new IllegalArgumentException("n must be greater than 0");
}
StringBuilder sb = new StringBuilder();
for (int i = 0; i < text.length(); i++) {
sb.append(text.charAt(i));
// Check if we reached the Nth character
if ((i + 1) % n == 0) {
result.add(sb.toString());
sb.setLength(0); // Reset StringBuilder
}
}
// Add any remaining characters
if (sb.length() > 0) {
result.add(sb.toString());
}
return result;
}
public static void main(String[] args) {
String input = "HelloWorld";
List<String> result = splitStringByNthCharacter(input, 3);
for (String str : result) {
System.out.println(str);
}
}
}
Explanation
In this code:
- We use a
StringBuilder
to efficiently construct substrings as we iterate through the input string. - The modulo operation (
(i + 1) % n == 0
) helps us identify when we've reached the Nth character. - After collecting substrings, we reset the
StringBuilder
to prepare for the next segment.
This method is generally faster than regex-based solutions, especially for long strings, since it avoids regex compilation and engine overhead.
Method 3: Using Streams (Java 8 and Above)
If you are using Java 8 or later, you can leverage the Stream API to create a more functional approach to string splitting.
Example Code: Using Java Streams
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
public class StreamSplitter {
public static List<String> splitStringByNthCharacter(String text, int n) {
// Validate the input
if (n <= 0) {
throw new IllegalArgumentException("n must be greater than 0");
}
return IntStream.range(0, text.length())
.boxed()
.collect(Collectors.groupingBy(i -> i / n, Collectors.mapping(text::charAt, Collectors.toList())))
.values()
.stream()
.map(chars -> chars.stream().map(String::valueOf).collect(Collectors.joining()))
.collect(Collectors.toList());
}
public static void main(String[] args) {
String input = "HelloWorld";
List<String> result = splitStringByNthCharacter(input, 3);
for (String str : result) {
System.out.println(str);
}
}
}
Explanation
This version:
- Utilizes
IntStream.range()
to create a stream of indices. - Groups characters according to their positions divided by N.
- Collects the grouped characters into a list of strings.
While functional programming offers clean and elegant solutions, it may come at a cost in terms of readability for those unfamiliar with Java Streams.
A Final Look
Learning to split strings efficiently is an invaluable skill in Java programming. We examined three strategies: using regular expressions, StringBuilder
, and Java Streams. Each method has its own advantages and scenarios where it excels.
For additional insights into string manipulation challenges, check out Mastering String Splitting: Tackle the Nth Character Challenge!.
As you continue to develop your skills in Java, experimenting with these different methods will help you choose the right tool for your specific needs. Happy coding!