Unlocking Java String Manipulation: The Nth Split Solution
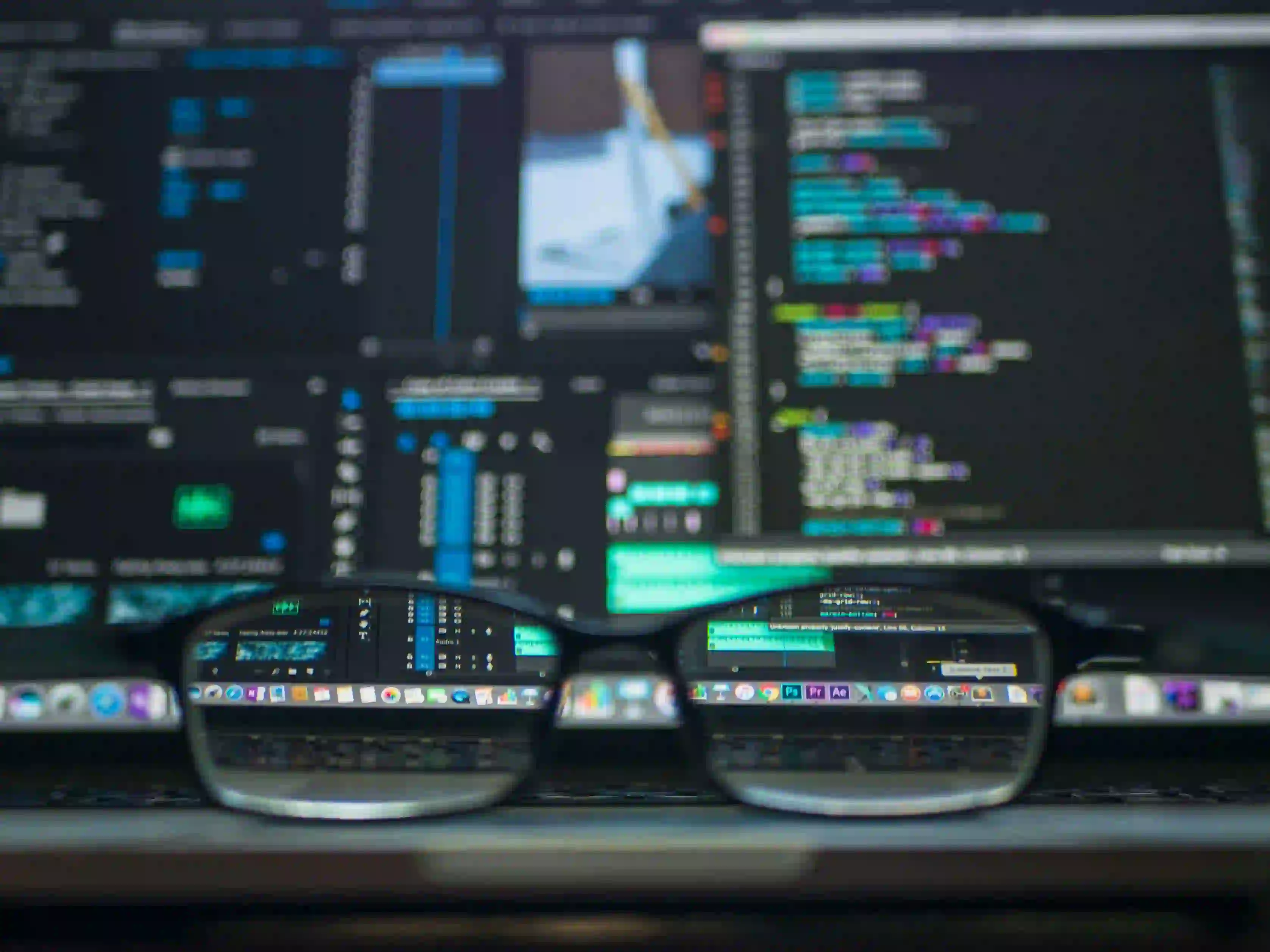
Unlocking Java String Manipulation: The Nth Split Solution
In the realm of Java programming, string manipulation is a fundamental skill that developers must master. From processing user inputs to generating dynamic outputs, strings are ever-present in every application. In this blog post, we will delve deep into an engaging challenge: splitting strings at the Nth occurrence of a specified delimiter. This concept isn't just a coding exercise; it's a necessary tool in your programming toolbox.
The Importance of String Manipulation
String manipulation is essential in various applications like data parsing, logging, and text processing. When you understand how to effectively work with strings, you can quickly extract and format data as needed. One specific problem in this domain is splitting strings at specific occurrences, which often proves more complex than it seems at first glance.
Problem Overview: Nth Character Challenge
Before we jump into the solution, let's explore the context of our problem. We are inspired by an existing article titled Mastering String Splitting: Tackle the Nth Character Challenge. The challenge presented is to split a given string at the Nth occurrence of a specific delimiter and return the results.
Example Walkthrough
Consider the string "apple-orange-banana-grape"
and the delimiter "-"
. If we want to split this string at the 2nd occurrence of the delimiter, the desired outcome would be:
- Before the split:
"apple-orange"
- After the split:
"banana-grape"
Creating the Nth Split Function
Let's create a reusable Java method that accomplishes this task. We'll write a method called splitAtNthOccurrence
, which takes three parameters: the input string, the delimiter, and the N value indicating which occurrence to split at.
Code Snippet
public class StringSplitter {
public static String[] splitAtNthOccurrence(String input, String delimiter, int n) {
// Check for invalid input values to avoid indices out of bounds
if (input == null || delimiter == null || n < 1) {
throw new IllegalArgumentException("Invalid input values");
}
// Split the string using the specified delimiter
String[] parts = input.split(delimiter, -1);
// Check if the Nth occurrence exists
if (n > parts.length - 1) {
return new String[]{input}; // Return the whole string if N is too large
}
// Join the first N parts together and return with the remaining parts
String beforeSplit = String.join(delimiter, Arrays.copyOf(parts, n));
String afterSplit = String.join(delimiter, Arrays.copyOfRange(parts, n, parts.length));
return new String[]{beforeSplit, afterSplit};
}
}
Explanation
-
Input Validation: At the very start, we check if the input string or delimiter is null or if N is less than one. This ensures that our function responds appropriately to invalid inputs.
-
String Splitting: We utilize the
split
method on strings. The-1
parameter allows for trailing empty strings to be included, which is essential when our delimiter appears at the end of the input. -
Existence Check: Before we work with the splits, we see if the Nth occurrence exists. If N is greater than the parts we've split, we simply return the original string wrapped in an array.
-
Joining Strings: We use
String.join
to concatenate our parts back together before and after the split point.
Testing the Function
Now that we have our method, it’s prudent to test it to ensure it performs as expected.
Example Test Cases
public static void main(String[] args) {
String input = "apple-orange-banana-grape";
String delimiter = "-";
int n = 2;
String[] splitResult = StringSplitter.splitAtNthOccurrence(input, delimiter, n);
System.out.println("Before split: " + splitResult[0]); // Output: "apple-orange"
System.out.println("After split: " + splitResult[1]); // Output: "banana-grape"
}
Running this test should yield the expected outcome, confirming that our function works correctly.
Additional Considerations
Edge Cases
Always consider edge cases when dealing with string manipulations:
- Empty Strings: Ensure your function handles empty strings without throwing an error.
- Single Occurrence: If there's only one occurrence of the delimiter and N is greater than one, the function should return the full string as is.
- Non-Existent Delimiter: If the specified delimiter does not exist in the string, the output should simply return the original string.
Performance
The given approach is efficient for moderate string sizes. However, if you're working with significantly large strings or a high frequency of calls, you may need to consider performance implications.
Final Thoughts
String manipulation is more than just a set of functions; it’s a language's capability to interact with data. The Nth split approach is just one example of how we can flexibly handle strings in Java. For more interesting concepts, like efficiently splitting strings and handling edge cases, be sure to check out the article Mastering String Splitting: Tackle the Nth Character Challenge.
Further Resources
For more advanced techniques in string manipulation, consider checking out:
Your Turn
Now it’s your turn! Try implementing your version of the Nth split function and test it against various string inputs. String manipulation is a skill that improves with practice, so don’t shy away from challenges!
Happy coding!