Troubleshooting Common Spring 4.0 REST API Setup Issues
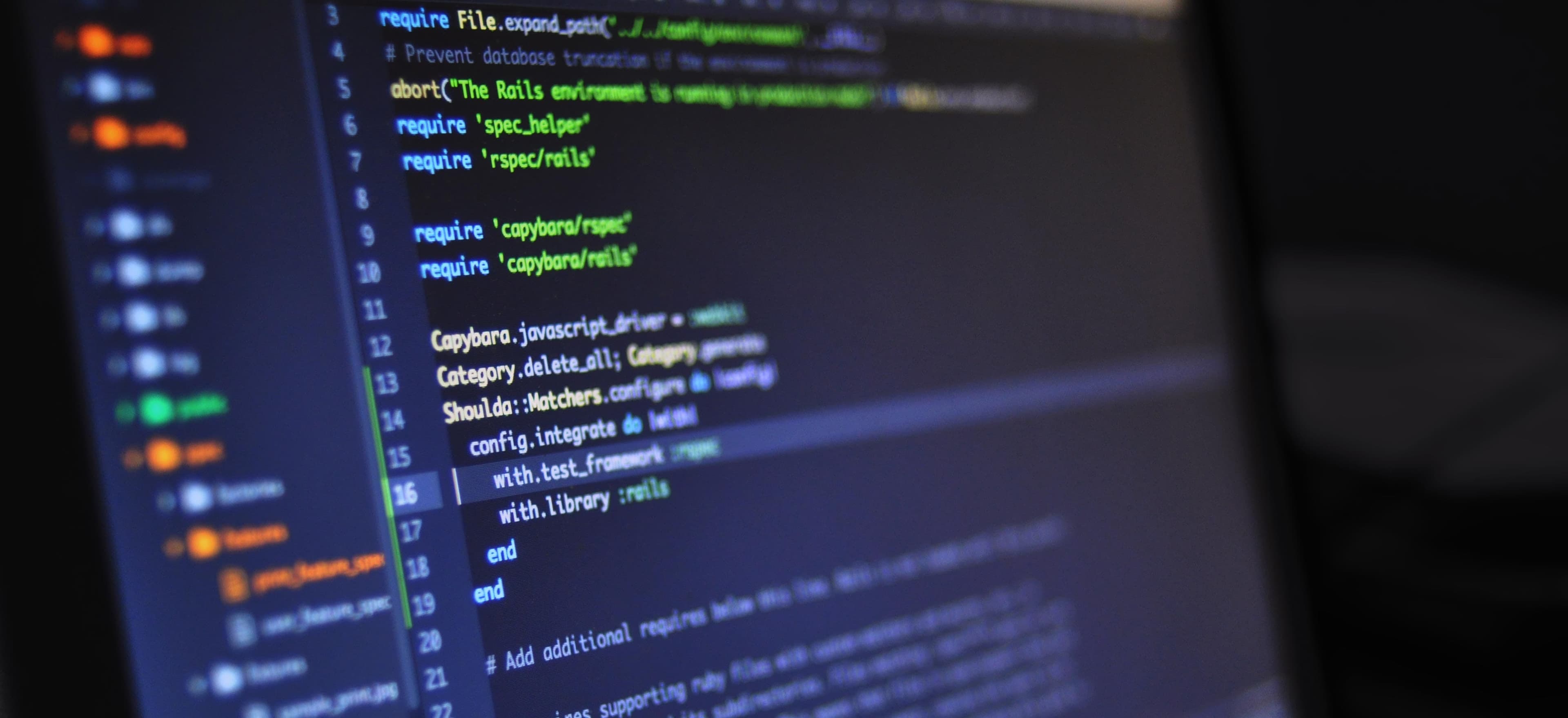
- Published on
Troubleshooting Common Spring 4.0 REST API Setup Issues
Spring Framework is a robust framework for building Java applications, particularly RESTful web services. The advent of Spring 4.0 brought several enhancements, especially with its support for REST APIs. However, setting up a REST API is not without challenges. This blog post will explore common issues developers may encounter when setting up a Spring 4.0 REST API, along with solutions and best practices.
Table of Contents
Understanding Spring REST
Spring provides a foundation for building robust and easily maintainable REST APIs. It leverages annotations to simplify configuration and enhances the flexibility of data handling. The following annotations are instrumental:
- @RestController: Combines @Controller and @ResponseBody, indicating that the methods return data directly rather than a view.
- @RequestMapping: Maps web requests to specific handler methods.
- @GetMapping, @PostMapping, etc.: Specialized versions of @RequestMapping for handling specific HTTP methods.
To illustrate, here's a simple example of a REST controller:
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users")
public List<User> getAllUsers() {
// Normally you'd retrieve users from a database
return Arrays.asList(new User("John"), new User("Jane"));
}
}
In this example, a GET request to /api/users
will return a list of users in JSON format.
Common Setup Issues
Issue 1: Dependency Management
Incorrect or incomplete dependencies can often lead to runtime issues. Ensure you include the necessary dependencies in your pom.xml
if you are using Maven:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</dependency>
</dependencies>
Why This Matters: Spring Boot Starter for web includes Spring MVC and the Jackson library for JSON serialization and deserialization. Missing these libraries can lead to class-not-found exceptions and serialization errors.
Issue 2: Incorrect Configuration
Spring's configuration can sometimes be the source of frustrating error messages. Make sure you have configured the DispatcherServlet
correctly in your web.xml
, if you're using a standard servlet container:
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/api/*</url-pattern>
</servlet-mapping>
Why This Matters: Failing to map the servlet properly can result in your endpoints being unreachable, leading to 404 Not Found errors. Always double-check window mappings and path specifics.
Issue 3: CORS Issues
Cross-Origin Resource Sharing (CORS) is a security feature implemented in web browsers. You may encounter issues if your API is accessed from a different origin. You can enable CORS in Spring using:
import org.springframework.web.bind.annotation.CrossOrigin;
@RestController
@RequestMapping("/api")
@CrossOrigin(origins = "http://localhost:3000") // Your frontend URL
public class UserController {
// Your methods here
}
Why This Matters: CORS issues are prevalent in frontend-backend setups. By specifying the origins, you allow only trusted sources to interact with your API. Otherwise, you might face 'CORS policy' errors.
Issue 4: Serialization Problems
Serialization issues arise when trying to convert Java objects to JSON format. This might occur if you have circular references or unrecognized types. A common solution is to use the @JsonIgnore
annotation to prevent the serialization of specific fields.
public class User {
private String name;
@JsonIgnore
private List<Friend> friends; // Circular reference
// Getters and Setters
}
Why This Matters: Without proper handling, serialization can yield stack overflow errors. Ignoring fields that lead to circular references ensures smooth JSON generation.
Best Practices for Setting Up REST APIs
-
Use Spring Boot: Simplify your project setup and dependency management. Spring Boot’s auto-configuration feature reduces boilerplate code.
-
Follow REST Principles: Adhere to RESTful conventions. Use appropriate HTTP methods, and ensure you follow proper URL structuring.
-
Document Your API: Utilize tools like Swagger or Spring Rest Docs to create interactive API documentation. This aids both in development and provides an easy reference for consumers of your API.
-
Employ Exception Handling: Use
@ControllerAdvice
for global exception handling, providing informative error responses.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<Object> handleNotFound(ResourceNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
}
- Version Your API: As your application evolves, v1, v2 routing allows clients to access specific versions of your APIs. This prevents breaking changes from affecting users.
The Last Word
Setting up a Spring 4.0 REST API can be straightforward or riddled with issues, depending on your awareness of common pitfalls. By being cautious of dependency management, configuration details, CORS settings, and serialization intricacies, you can streamline your API development process. Additionally, following best practices ensures your API remains robust and ready for future extensions.
For more information on Spring and RESTful services, check out the Spring Framework documentation. Happy coding!
This structured approach highlights issues and solutions while considering reader engagement. Feel free to adapt this article according to your needs!