Understanding the Impact of Full GC on Java Performance
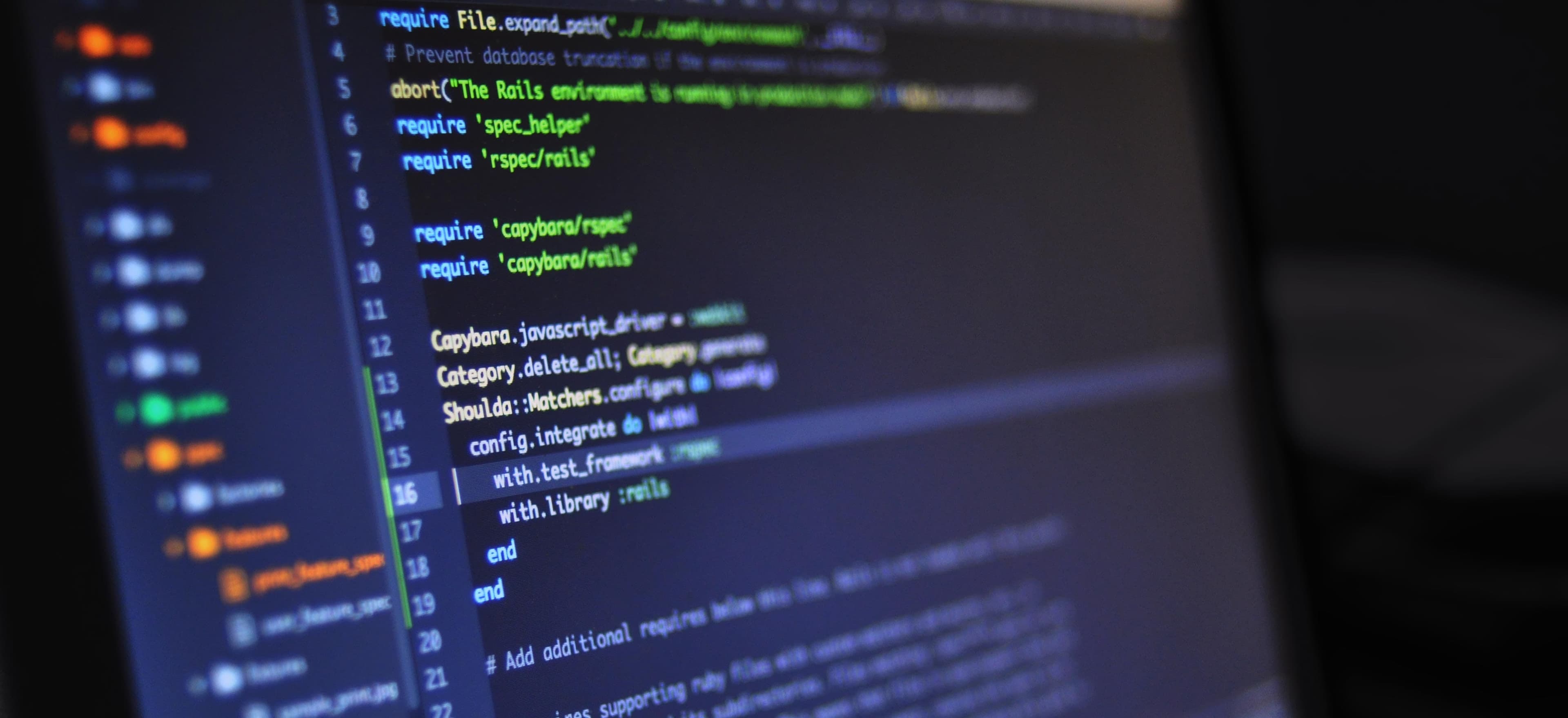
- Published on
Understanding the Impact of Full GC on Java Performance
Java, one of the most widely-used programming languages today, relies heavily on automatic memory management through its garbage collection (GC) system. While Java developers enjoy the luxury of not having to handle memory allocation and deallocation manually, they must understand the implications of garbage collection, particularly Full GC. This blog post delves into Full GC's role in memory management, its impact on performance, and strategies for optimization.
What is Garbage Collection?
Garbage Collection (GC) is a process by which Java automatically releases memory that is no longer in use, effectively preventing memory leaks. In Java, this process is divided mainly into two types: Minor GC and Full GC (or Major GC).
- Minor GC: Primarily cleans up the young generation space, where newly created objects reside. It's typically efficient and occurs frequently.
- Full GC: Targets both the young generation and the old generation (where long-lived objects are allocated). It involves a more comprehensive and time-consuming process to clean up memory.
Why Full GC is Triggered
Full GC can be triggered by several factors, including:
- Insufficient Memory: When the old generation has reached its limit, Full GC runs to reclaim memory.
- Explicit Call: Methods like
System.gc()
can trigger Full GC, though its efficacy is backgrounded by JVM implementation. - Long-lived Objects: If a significant number of long-lived objects are created and memory is allocated inefficiently, a Full GC may result.
Performance Impact of Full GC
The consequences of Full GC can be dire for Java applications. Here are several critical ways it can impact performance:
1. Increased Latency
Full GC pauses all application threads to reclaim memory, resulting in "stop-the-world" (STW) events. A long pause can lead to noticeable latency, making the application feel sluggish or unresponsive. This behavior is particularly damaging in real-time systems or performance-sensitive applications.
2. Increased CPU Usage
Despite being a memory recovery method, Full GC consumes CPU resources. Heavy CPU cycles are required to traverse and manage objects in memory, which can lead to overall performance degradation. This might even affect user experience if the application cannot respond seamlessly.
3. Non-linear Performance Degradation
Frequent Full GC events can lead to heap fragmentation. As memory is continuously allocated and deallocated, the usable memory becomes split into smaller chunks, making it harder for the JVM to allocate memory efficiently. This condition may lead to increased GC events, creating a vicious cycle that deteriorates performance.
Example: Monitoring GC Performance
Using tools like VisualVM, you can monitor and visualize GC behavior. Below is an example of how to enable GC logging in a Java application:
java -Xlog:gc*:file=gc.log:time,level,tags -jar YourApplication.jar
This command will generate a gc.log
file containing detailed information about garbage collection occurrences, helping you diagnose issues related to Full GC.
Best Practices to Optimize Full GC Impact
To mitigate the performance issues caused by Full GC, consider the following best practices:
1. Increase Heap Size
One of the primary solutions is to increase the heap size, which delays the necessity for Full GC. You can specify the maximum heap size using:
java -Xmx1024m -jar YourApplication.jar
However, be cautious: allocating too much memory may lead to longer garbage collection pauses.
2. Optimize Object Creation
Excessive object creation leads to frequent GC cycles. Here are strategies to optimize object creation:
-
Object Pooling: Recycle objects instead of creating new ones, especially for frequently used objects.
public class ObjectPool { private final List<MyObject> pool = new ArrayList<>(); public MyObject borrowObject() { return pool.isEmpty() ? new MyObject() : pool.remove(pool.size() - 1); } public void returnObject(MyObject obj) { pool.add(obj); } }
-
Immutable Objects: Encourage the use of immutable classes. They help reduce the need for copies and, consequently, allocations.
3. Use the Right GC Algorithm
Java offers multiple garbage collection algorithms, each with its pros and cons. The default algorithm might not suit your application's needs. Evaluate and select an appropriate one:
- G1 Garbage Collector: Suitable for applications requiring low latency.
- CMS (Concurrent Mark-Sweep): Designed to minimize pauses caused by GC.
For example, to switch to G1, use:
java -XX:+UseG1GC -jar YourApplication.jar
4. Tune JVM Parameters
Optimizing JVM parameters can also reduce the frequency and duration of Full GCs. Some parameters to consider:
- New Size (
-Xmn
): Controls the size of the young generation, which can affect the frequency of Minor GC and, consequently, Full GC. - Survivor Ratio: Adjust the ratio between the Eden space and Survivor space.
5. Monitor Application Performance
A proactive approach is crucial. Continuously monitor your application's performance, paying close attention to GC metrics. Use tools like Prometheus, Grafana, or Java Management Extensions (JMX) for real-time monitoring.
Example of JMX to Monitor GC Activity
Adding the following JVM options enables JMX monitoring:
java -Dcom.sun.management.jmxremote -Dcom.sun.management.jmxremote.port=12345 -Dcom.sun.management.jmxremote.authenticate=false -Dcom.sun.management.jmxremote.ssl=false -jar YourApplication.jar
This will allow you to connect and visualize GC trends over time.
Wrapping Up
Understanding the impact of Full GC on Java application performance is essential for building efficient and responsive systems. By recognizing the signs of overly aggressive garbage collection and taking appropriate action, you can tremendously bolster your application's performance and reliability.
Consider applying some of the strategies discussed above, whether it’s optimizing object allocation, choosing the right GC algorithm, or consistently monitoring GC logs.
For more information on Java's garbage collection, check out these resources:
- Java Garbage Collection - Oracle Documentation
- Understanding Java's G1 Garbage Collector
The road to optimal performance is a cycle of monitoring, adjusting, and testing. Embrace the nuances of garbage collection, and your Java applications will shine!
Checkout our other articles