Common Pitfalls in Spoofax: How to Avoid Early Mistakes
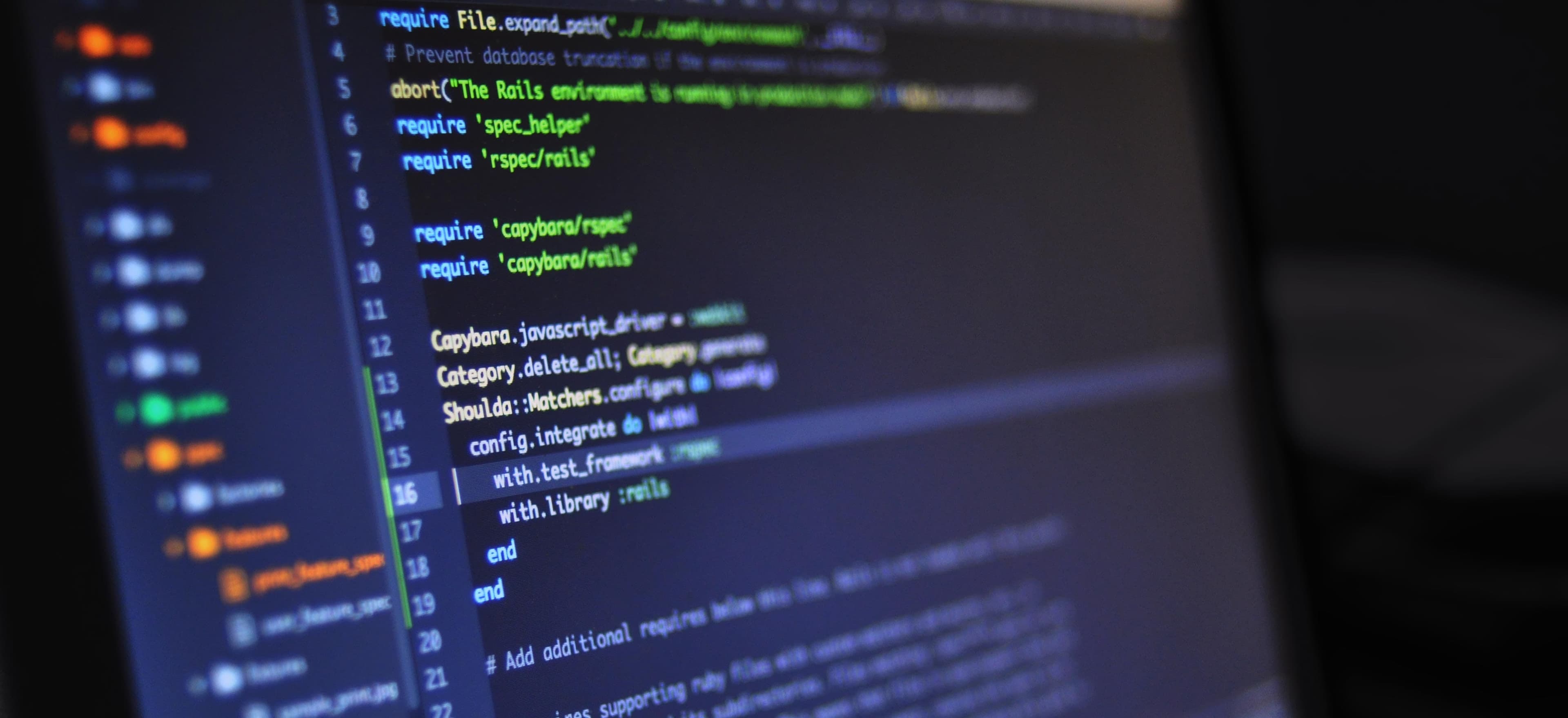
- Published on
Common Pitfalls in Spoofax: How to Avoid Early Mistakes
Spoofax is a powerful language development framework designed for creating domain-specific languages (DSLs) and language workbenches. However, newcomers often stumble upon various pitfalls that can hinder their progress and lead to poor design choices. This blog post aims to illuminate these common pitfalls and provide practical solutions to help you get started with Spoofax effectively.
What is Spoofax?
Before diving into the pitfalls, it is crucial to understand what Spoofax offers. Spoofax provides tools for language specifications, parsers, interpreters, and pretty printers. It is built on top of the Language Server Protocol, making it a flexible solution for developing languages that need features like syntax highlighting, auto-completion, and error-checking.
Common Pitfalls in Spoofax
1. Ignoring Documentation
The Importance of Reading the Docs
One of the biggest mistakes new Spoofax users make is neglecting the documentation. Spoofax has thorough documentation that covers various aspects, including setup, language design, and tooling. Not leveraging this valuable resource can lead to confusion and unnecessary rework.
Solution
Make it a habit to refer to the Spoofax Documentation regularly. Familiarize yourself with its structure and features. If you're unsure about something, there's a good chance the answer is already documented.
2. Skipping Examples
Concrete Implementations Matter
Another common pitfall is the inclination to jump straight into development without looking at practical examples. Spoofax provides numerous examples that demonstrate its capabilities and best practices.
Solution
Before creating your own DSL, review the examples provided in the Spoofax GitHub Repository. Pay attention to how others have structured their languages, defined their syntax, and implemented features. This can save you time and help you learn effective design patterns.
3. Overcomplicating Grammar Definitions
Keep It Simple
It's easy to get carried away and create overly complex grammar definitions. While Spoofax supports powerful language features, simplicity often leads to better maintainability and clarity.
Solution
Start with a minimal grammar. Here’s a simple example of a language definition:
module mylang
syntax MyLang = expression*
expression = term (('+' | '-') term)*
term = factor ( ('*' | '/') factor)*
factor = number | '(' expression ')'
number = [0-9]+
Why This Matters: By breaking down your grammar into manageable pieces, you can focus on the essential functionalities of your language. This will make debugging easier and your grammar more understandable.
4. Not Utilizing the Spoofax Language Workbench
Lack of Tooling
Many developers are unaware of the full capabilities of the Spoofax Language Workbench. They may neglect features like error highlighting, code formatting, or lacking efficient debugging tools.
Solution
Familiarize yourself with the integrated tooling features. For example, use the error messages and suggestions:
// Spoofax Java code snippet demonstrating a simple analysis
public class SimpleAnalyzer implements Analysis {
@Override
public void analyze(Document document) {
if (document == null || document.isEmpty()) {
addError("Document is empty");
} else {
// Analysis logic here
}
}
}
Why This Matters: Utilizing these tools enhances your language development process by catching errors early, improving code quality, and increasing productivity.
5. Lack of Testing
Testing is Essential
One of the most neglected aspects of language development is testing. Developers often assume their code works without adequate tests in place.
Solution
Integrate unit testing as part of your development process from the start. Spoofax supports unit tests via its testing framework. Here is an example of a simple test:
@Test
public void testInitialValue() {
MyLangParser parser = new MyLangParser();
ParseResult result = parser.parse("1 + 2");
assertTrue(result.isSuccessful());
assertEquals(3, result.getValue());
}
Why This Matters: Testing your language ensures that each component behaves as expected. It helps catch regressions early when modifications are made.
6. Poor Error Handling
Graceful Failures
Failing to implement meaningful error messages can leave users perplexed when issues arise. Poor error handling can lead to frustration and lower adoption rates of your DSL.
Solution
Provide clear and informative error messages, guiding users to correct their inputs. Use Spoofax's built-in mechanisms to offer specific feedback:
syntax Expression = "E" (uda{message}: "Invalid expression. Syntax error." )
Why This Matters: Users appreciate clear guidance when errors occur. This can significantly improve the learning curve and user experience for your language.
Final Considerations
Developing a language with Spoofax can be an enriching experience, but it is not without its pitfalls. By consciously avoiding these common mistakes, you can create a more robust and user-friendly DSL. Always keep documentation close, examine examples, start simple, embrace testing, and handle errors gracefully.
Remember, the goal is not just to build a language but to foster an experience that is both intuitive and powerful for users. By adhering to these guidelines, you will set yourself on a path to successfully harnessing the potential of Spoofax.
For more insights and updates, consider following Spoofax's community forums and joining discussions on relevant platforms. Happy coding!
Are you looking for more detailed information on aspects of Spoofax? Don’t hesitate to check out the official Spoofax GitHub for a wealth of resources.
Checkout our other articles