Troubleshooting JMS Sender Connection Issues with ActiveMQ
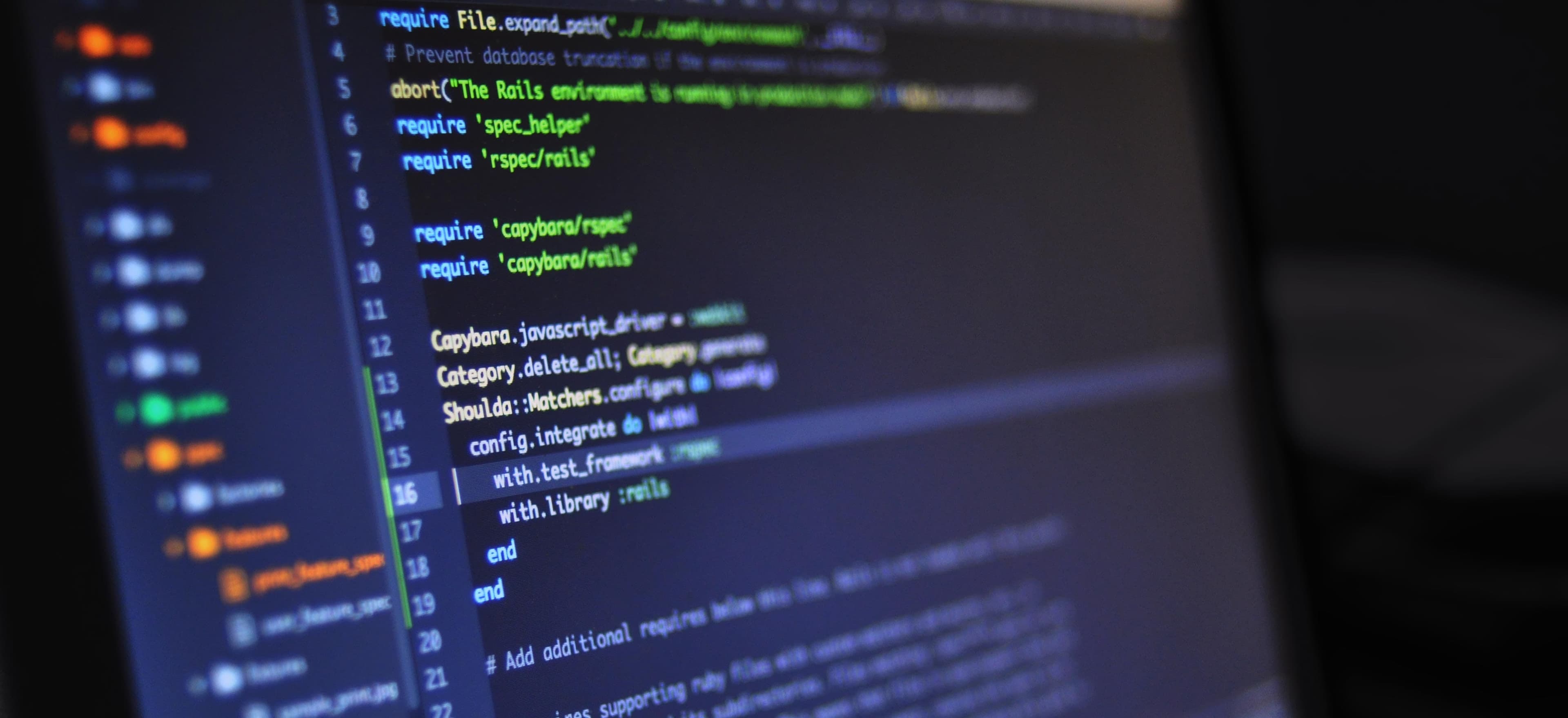
- Published on
Troubleshooting JMS Sender Connection Issues with ActiveMQ
Java Messaging Service (JMS) is a crucial component for building robust, loosely-coupled, and asynchronous applications. ActiveMQ, a popular open-source message broker written in Java, is commonly used for JMS implementations. However, while developing applications that rely on JMS with ActiveMQ, you may encounter connection issues that can be perplexing. In this blog post, we will explore common sender connection issues and how to troubleshoot them effectively.
Understanding JMS and ActiveMQ Basics
Before diving into troubleshooting, it is essential to review some underlying concepts.
- JMS: JMS is an API that allows for the exchange of messages between two or more clients. It provides two generalized messaging models: point-to-point and publish-subscribe.
- ActiveMQ: ActiveMQ acts as a middleware service that facilitates message transmission between producers (message senders) and consumers (message receivers).
Key Components of ActiveMQ
The architecture involves several key components:
- Broker: The server that routes messages.
- Destination: A queue or topic where messages are sent.
- Session: A single context for producing and consuming messages.
- ConnectionFactory: An object that provides connections to the broker.
Common Connection Issues
When sending messages using JMS, you might encounter issues that disrupt connectivity to ActiveMQ. Below are common problems and their respective solutions.
1. Incorrect Connection URL
One of the most frequent issues arises from an incorrect broker URL. ActiveMQ uses a specific URI format for connections.
Example Connection URL
String brokerURL = "tcp://localhost:61616"; // Default URL for ActiveMQ
Troubleshooting Steps:
- Verify that the URL is formatted correctly.
- Ensure that the rate of using
tcp://
is appropriate as per your ActiveMQ configuration.
2. Authentication Failures
When connecting to secured brokers, authentication can fail due to incorrect credentials.
Example Code
import javax.jms.*;
import org.apache.activemq.ActiveMQConnectionFactory;
public class JMSConnection {
public static void main(String[] args) {
String brokerURL = "tcp://localhost:61616";
String username = "admin"; // Change as per your configuration
String password = "admin"; // Change as per your configuration
try {
ActiveMQConnectionFactory factory =
new ActiveMQConnectionFactory(brokerURL);
Connection connection = factory.createConnection(username, password);
connection.start();
// Application logic here
} catch (JMSException e) {
e.printStackTrace();
}
}
}
Why this matters: Failing to provide correct username
and password
results in an authentication exception. Verify these credentials in the activemq.xml
configuration.
3. Firewall and Network Issues
If your application is hosted on a different machine from ActiveMQ, ensure that firewall settings allow traffic over the designated port (61616 by default).
Troubleshooting Steps:
- Run a telnet command to check connectivity:
telnet localhost 61616
- Adjust firewall settings or add rules to allow TCP traffic on the required port.
4. ActiveMQ Broker Not Running
If you’re unable to connect, ensure that the ActiveMQ broker is running.
How to check:
- You can verify this via the command line:
activemq status
- Alternatively, check the ActiveMQ Web Console by navigating to
http://localhost:8161/admin
.
5. Network Addressing Issues
Check that the host address you are using in your connection URL is reachable. Use tools like ping
to confirm connectivity.
6. Thread Limits and Resource Constraints
When working with multiple sender or consumer threads, you could run into resource constraints.
Monitoring Connections:
ActiveMQ provides management options to monitor connections. You can use JMX to observe active connections and thread usage.
// Enable JVM Heap and Thread analysis
System.setProperty("activemq.brokerName", "localhost");
System.setProperty("activemq.brokerPORT", "61616");
Why this matters: Thread exhaustion or memory issues can prevent new connections. Monitor the broker's performance appropriately.
7. Using URI Options
ActiveMQ supports URI options that help configure connection settings such as timeouts and connection retries.
Example of Defining URI Options
String brokerURL = "tcp://localhost:61616?jms.useAsyncSend=true";
Why This Matters: Asynchronous sending can improve performance when dealing with high throughput scenarios.
Debugging Tips
When you encounter connection issues, information from logs can be invaluable.
-
Check Broker Logs: ActiveMQ logs errors that can provide context about connection issues. Look in the
logs/
directory where your ActiveMQ is installed. -
Increase Log Level for Troubleshooting: Temporarily increase the logging level to DEBUG or TRACE to get more insights.
Add the following to your
log4j.properties
:log4j.logger.org.apache.activemq=DEBUG
-
JMX Monitoring: Utilize JMX interfaces to monitor broker health and connection stats.
Example Code Snippet
Here is a comprehensive example that includes error handling and connection management:
import javax.jms.*;
import org.apache.activemq.ActiveMQConnectionFactory;
public class JmsSender {
public static void main(String[] args) {
String brokerURL = "tcp://localhost:61616";
Connection connection = null;
try {
ActiveMQConnectionFactory factory = new ActiveMQConnectionFactory(brokerURL);
connection = factory.createConnection();
connection.start();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Destination destination = session.createQueue("testQueue");
MessageProducer producer = session.createProducer(destination);
TextMessage message = session.createTextMessage("Hello, ActiveMQ!");
producer.send(message);
System.out.println("Sent message: " + message.getText());
} catch (JMSException e) {
System.err.println("Connection issue: " + e.getMessage());
} finally {
try {
if (connection != null) {
connection.close();
}
} catch (JMSException e) {
e.printStackTrace();
}
}
}
}
Lessons Learned
Troubleshooting JMS sender connection issues with ActiveMQ can be challenging, but understanding the common pitfalls allows developers to remedy problems swiftly. Remember to validate configurations, confirm that components are running as expected, and monitor logs for insights. Additionally, employing best practices like setting URI options and error handling can enhance the robustness of your JMS application.
For further reading, consider exploring the following resources:
- ActiveMQ Official Documentation
- JMS Specifications
- Development Best Practices with JMS
Feel free to share your experiences or questions related to JMS and ActiveMQ in the comments below!