Common Pitfalls in Android Voice Recognition You Must Avoid
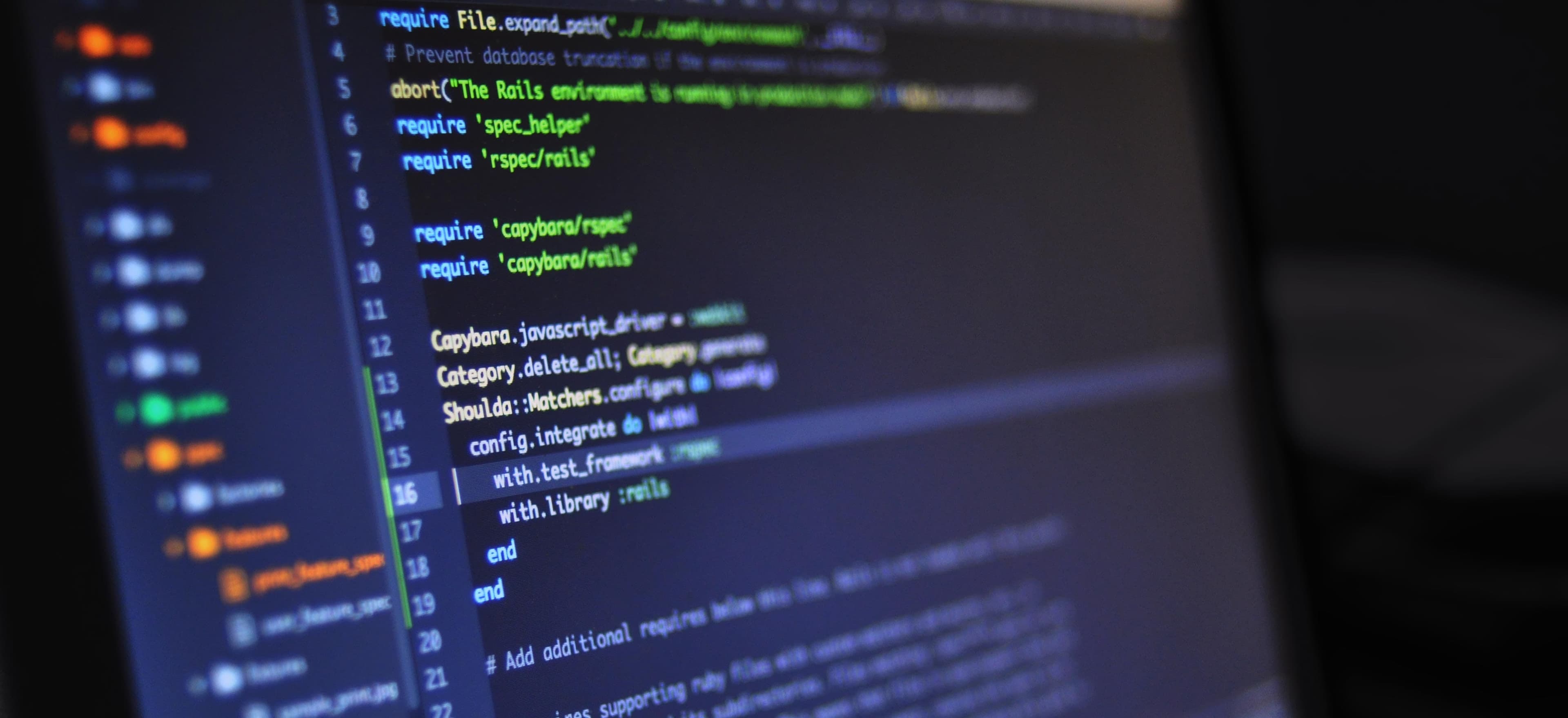
- Published on
Common Pitfalls in Android Voice Recognition You Must Avoid
Voice recognition technology is becoming increasingly prevalent in today's mobile applications. As an Android developer, incorporating voice recognition can enhance user experience, streamline operations, and facilitate accessibility. However, while developing applications with speech recognition features, you might encounter a variety of challenges. In this blog post, we will explore common pitfalls in Android voice recognition and provide essential strategies to help you avoid them.
Understanding Android Voice Recognition
Voice recognition on Android is primarily powered by the Google Speech API. It allows applications to process spoken words and convert them into text format, enabling functionalities like voice commands, voice-to-text input, and much more.
For more insight on how voice recognition works in Android, you can refer to Google’s Official Documentation.
Key Pitfalls in Android Voice Recognition
-
Inadequate Testing in Varied Environments
One of the most significant pitfalls is failing to adequately test your voice recognition application in different noise environments. Voice recognition systems thrive on clarity, and background noise can interfere significantly.
Solution: Always conduct tests in a variety of environments—quiet rooms, busy streets, cafés, etc. This practice will inform you about how much noise the app can handle and how accurately it can recognize voice commands.
-
Ignoring Multilingual Speech Recognition
Many applications cater to users across different linguistic backgrounds. Neglecting to implement multilingual support can alienate a significant portion of your user base.
Solution: Utilize the
getSupportedLanguages()
function provided by the SpeechRecognizer class to determine available languages:private void checkSupportedLanguages() { List<String> languages = SpeechRecognizer.getAvailableLanguages(); for (String language : languages) { Log.d("Supported Language", language); } }
Always provide options for users to select their preferred language, and ensure your recognition logic accounts for this selection.
-
Faulty Error Handling
When it comes to voice recognition, users should be aware of what went wrong if a command fails. Relying solely on a generic error message can frustrate users.
Solution: Implement comprehensive error handling using
RecognitionListener
. Ensure to provide meaningful feedback based on the type of error encountered.recognitionListener = new RecognitionListener() { @Override public void onError(int error) { switch (error) { case SpeechRecognizer.ERROR_AUDIO: Log.e("Voice Recognition", "Audio recording error"); break; case SpeechRecognizer.ERROR_NETWORK: Log.e("Voice Recognition", "Network error"); break; default: Log.e("Voice Recognition", "Unknown error occurred"); } } };
This feedback will improve user trust and experience with your application.
-
Lack of User Confirmation for Actions Based on Voice Commands
Implementing decisive actions triggered by voice commands without user confirmation can lead to unintended consequences. Imagine a scenario where a user says "Delete my file" and the file gets deleted without any warning.
Solution: Always use confirmation dialogs for critical actions. You can employ the following example:
private void confirmAction() { new AlertDialog.Builder(this) .setTitle("Confirm Action") .setMessage("Are you sure you want to delete the file?") .setPositiveButton(android.R.string.yes, (dialog, which) -> deleteFile()) .setNegativeButton(android.R.string.no, null) .show(); }
It reassures users and provides an added layer of security.
-
Underestimating the Importance of User Training
Users may not be familiar with the voice recognition features of your application. Assuming they will know how to use it effectively can result in poor user engagement.
Solution: Include a guide or tutorial explaining how to interact with the voice recognition feature effectively. This can mitigate confusion and improve the overall user experience.
-
Overlooking Accessibility Features
Accessibility features are crucial for providing an inclusive user experience for individuals with disabilities. Failing to consider these users can result in negative feedback and decreased application adoption.
Solution: Ensure your voice recognition feature conforms to accessibility standards. Utilize Android’s
AccessibilityManager
to check and handle accessibility-related events.AccessibilityManager am = (AccessibilityManager) getSystemService(ACCESSIBILITY_SERVICE); if (am.isEnabled()) { // Notify users about voice recognition feature }
-
Relying Heavily on System Voice Recognition
While the built-in voice recognition features provided by Android are highly effective, they come with some limitations. Not every application can fully leverage these without customization.
Solution: Consider integrating third-party libraries or building a custom solution tailored to your application's needs. Libraries like PocketSphinx allow customization based on specific vocabularies and commands, which can significantly enhance voice recognition in specialized applications.
-
Ignoring Privacy Concerns
Voice data is sensitive. Users are often cautious about how their voice data is stored, shared, or utilized. Ignoring user privacy can lead to trust issues and potential legal complications.
Solution: Always inform users about data usage policies. Obtain explicit permission when you collect voice data, and ensure you comply with regulations such as GDPR and CCPA.
private void requestPermissions() {
if (ContextCompat.checkSelfPermission(this, Manifest.permission.RECORD_AUDIO) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.RECORD_AUDIO}, REQUEST_RECORD_AUDIO);
}
}
-
Failing to Optimize for Device Variations
With numerous Android devices available, variations in hardware, screen size, and operating systems can affect performance.
Solution: Adapt your application to different screen sizes and configurations through responsive design principles and testing on multiple device types. Remember to analyze how microphone quality can vary across devices, affecting the performance of voice recognition.
-
Not Keeping Up-to-Date With Android Upgrades
Android continually evolves, and the speech recognition components might undergo significant changes. Neglecting to keep your application updated can lead to compatibility issues.
Solution: Regularly check for updates on the Android release notes and adapt your application accordingly. Keeping libraries and dependencies up-to-date ensures access to the latest features and security improvements.
Final Considerations
Integrating voice recognition in your Android applications can significantly enhance user experience, but it requires careful consideration to avoid common pitfalls. By conducting thorough testing, managing errors effectively, and consistently prioritizing user engagement and privacy, you can ensure a robust and user-friendly application.
This guide highlights some essential best practices for developing voice-enabled applications in Android. To give your users an effective and enjoyable experience, always take the time to understand their needs and application contexts.
For further reading, check out these resources:
By following these guidelines, you'll be better equipped to create Android applications that leverage the exciting capabilities of voice recognition without running into troublesome pitfalls. Happy coding!
Checkout our other articles