Troubleshooting Common Issues with Hibernate Event Listeners
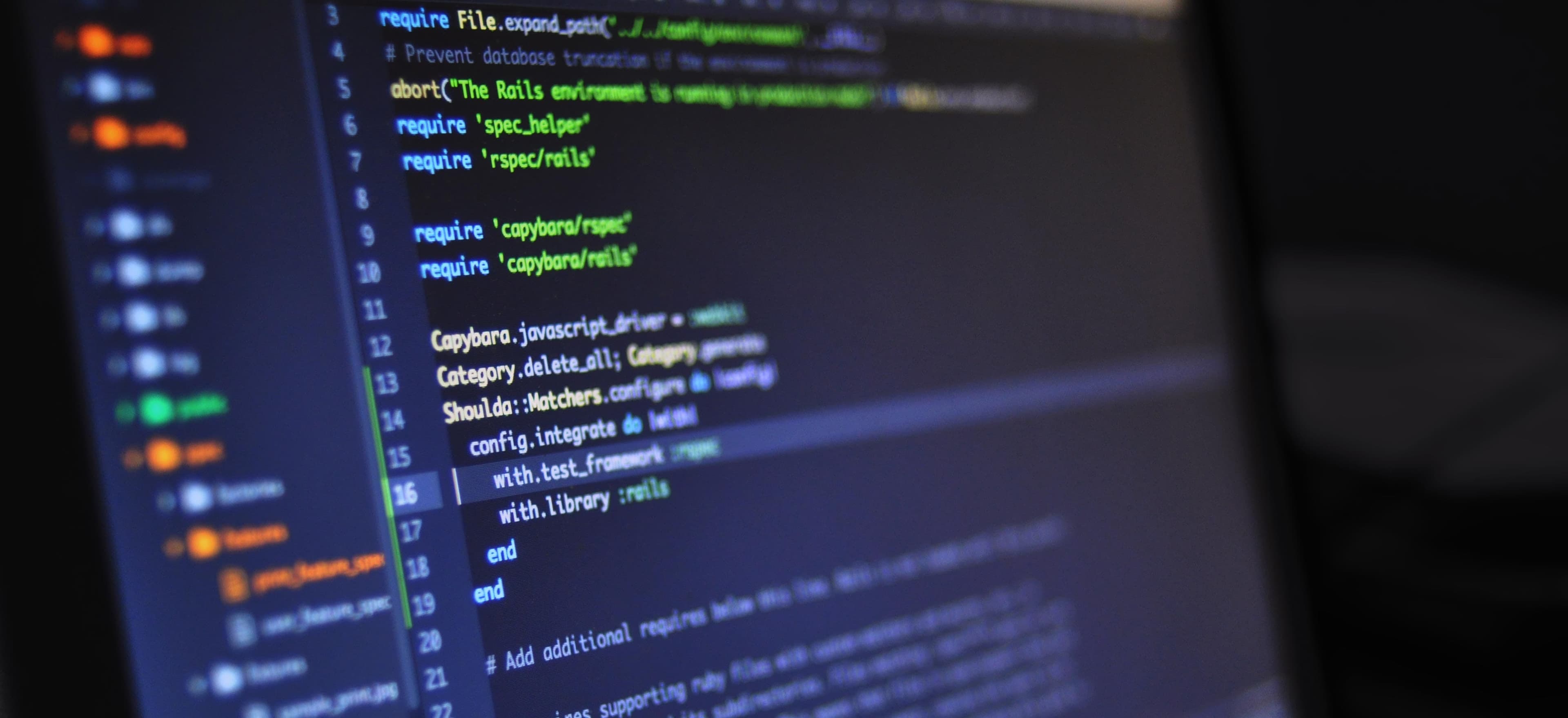
- Published on
Troubleshooting Common Issues with Hibernate Event Listeners
Hibernate is a powerful Object-Relational Mapping (ORM) framework used in Java to interact with databases seamlessly. One of its fascinating features is the concept of event listeners, which allows for the execution of custom logic during various phases of the entity lifecycle (like creation, update, deletion). However, working with Hibernate event listeners can sometimes lead to unexpected issues. In this blog post, we will explore common problems encountered while utilizing Hibernate event listeners, their solutions, and best practices to follow.
Understanding Hibernate Event Listeners
Hibernate provides several event listeners that can be implemented to react to entity lifecycle events. Some of these events include:
- PrePersist: Triggered before an entity is saved.
- PostPersist: Activated after an entity is saved.
- PreUpdate: Invoked before an entity is updated.
- PostUpdate: Triggered after an entity is updated.
Using event listeners allows developers to separate concerns and add business logic without cluttering existing entity classes.
Example of an Event Listener Implementation
Here’s a basic example of a PrePersist event listener for a hypothetical User
entity:
import org.hibernate.event.spi.PrePersistEvent;
import org.hibernate.event.spi.PrePersistEventListener;
public class UserEventListener implements PrePersistEventListener {
@Override
public boolean onPrePersist(PrePersistEvent event) {
if (event.getEntity() instanceof User) {
User user = (User) event.getEntity();
// Custom logic before user is persisted
validateUser(user);
}
return false; // Return false to allow the persist to proceed
}
private void validateUser(User user) {
if (user.getUsername() == null || user.getUsername().isEmpty()) {
throw new IllegalArgumentException("Username cannot be empty");
}
}
}
Why Use Event Listeners?
Using listeners provides several benefits:
- Separation of Concerns: You can encapsulate the logic related to persistent operations, keeping your entity classes clean.
- Reusability: Listeners can be reused across different parts of the application, promoting DRY principles.
- Cross-Cutting Concerns: ECross-cutting functionalities like logging, validation, and auditing can be centralized in one place.
Common Issues Encountered with Hibernate Event Listeners
1. Listener Not Called
One of the most common issues developers face is that the event listener is not invoked. This can happen for several reasons:
- Listener Not Registered: If the listener is not added to the session factory or not included in the configuration, it won't trigger. Make sure to register it properly.
Configuration configuration = new Configuration();
configuration.setListener("pre-persist", new UserEventListener());
- Entity Not Managed: If the entity doesn’t go through Hibernate's management (e.g., it is detached), the listener won’t be triggered. Always ensure that your entities are properly managed by the session.
2. Multiple Listeners and Order of Execution
When multiple event listeners are present for the same event, it's important to understand their execution order. The order of listener execution can lead to unpredictable behavior.
- Conflict: If listeners interfere with one another (e.g., both trying to validate data), it’s essential to enforce a proper execution flow or combine functionality.
// Register multiple listeners
configuration.setListener("pre-persist", new UserEventListener());
configuration.setListener("pre-persist", new AuditEventListener());
Best Practice: Keep listeners simple and focused. If combined functionality is necessary, consider using a decorator or a mediator pattern to manage the logic without conflict.
3. Performance Issues
Event listeners can introduce performance overhead, particularly if you perform heavy operations such as network calls or database interactions.
- Minimize I/O Operations: Try to limit resource-intensive operations inside listeners. Prefer in-memory checks and validations.
Example:
@Override
public boolean onPrePersist(PrePersistEvent event) {
if (event.getEntity() instanceof User) {
User user = (User) event.getEntity();
// Avoid making external calls here
if (!isUsernameTaken(user.getUsername())) {
// Proceed
}
}
// Instead of checking the database every time, use a cached list of existing usernames when possible
}
4. Exception Handling
Unhandled exceptions can lead to transaction failures, which may not provide clear feedback on the root cause of the issue.
- Proper Logging: Ensure that exceptions are logged adequately to facilitate debugging.
- Transaction Management: Be cautious with exception handling strategies. Do not catch all exceptions; instead, allow critical errors to propagate while managing expected issues gracefully.
Example:
@Override
public boolean onPrePersist(PrePersistEvent event) {
try {
// Validation logic
} catch (IllegalArgumentException e) {
// Log error and rethrow
logger.error("Validation failed for user: {}", event.getEntity(), e);
throw e; // Let the transaction fail
}
}
Best Practices for Using Hibernate Event Listeners
-
Use Annotations for Configuration: Consider using JPA annotations to configure listeners, as they are self-documenting and easily integrated within the code.
@EntityListeners(UserEventListener.class) public class User { // entity fields }
-
Keep Logic Simple: Event listener logic should ideally be lightweight. Delegate heavier processing to background jobs or separate services.
-
Use Dependency Injection: Utilize a dependency injection framework (like Spring) to manage event listener instances. This practice promotes better lifecycle management and testing.
-
Test Extensively: Test your listeners meticulously to cover edge cases. Unforeseen interactions can lead to difficult-to-track issues.
-
Monitor Performance: Watch for performance bottlenecks post-implementation. Use profiling tools to understand the overhead your listeners may introduce.
Lessons Learned
Hibernate event listeners are powerful tools that can enhance the functionality and maintainability of your data access layer. However, they come with their own set of challenges that can affect your application if not handled correctly. By following the recommendations outlined in this post, you can build robust and efficient Hibernate event listeners that seamlessly integrate into your Java applications.
To learn more about Hibernate and event listeners, you can visit the official Hibernate Documentation for a deeper dive into their capabilities and best practices. If you're just getting started with Hibernate, it might be beneficial to read about JPA and Hibernate to grasp the foundational elements.
Happy Coding!
Checkout our other articles