Common Mockito Pitfalls: Avoid These Beginner Mistakes!
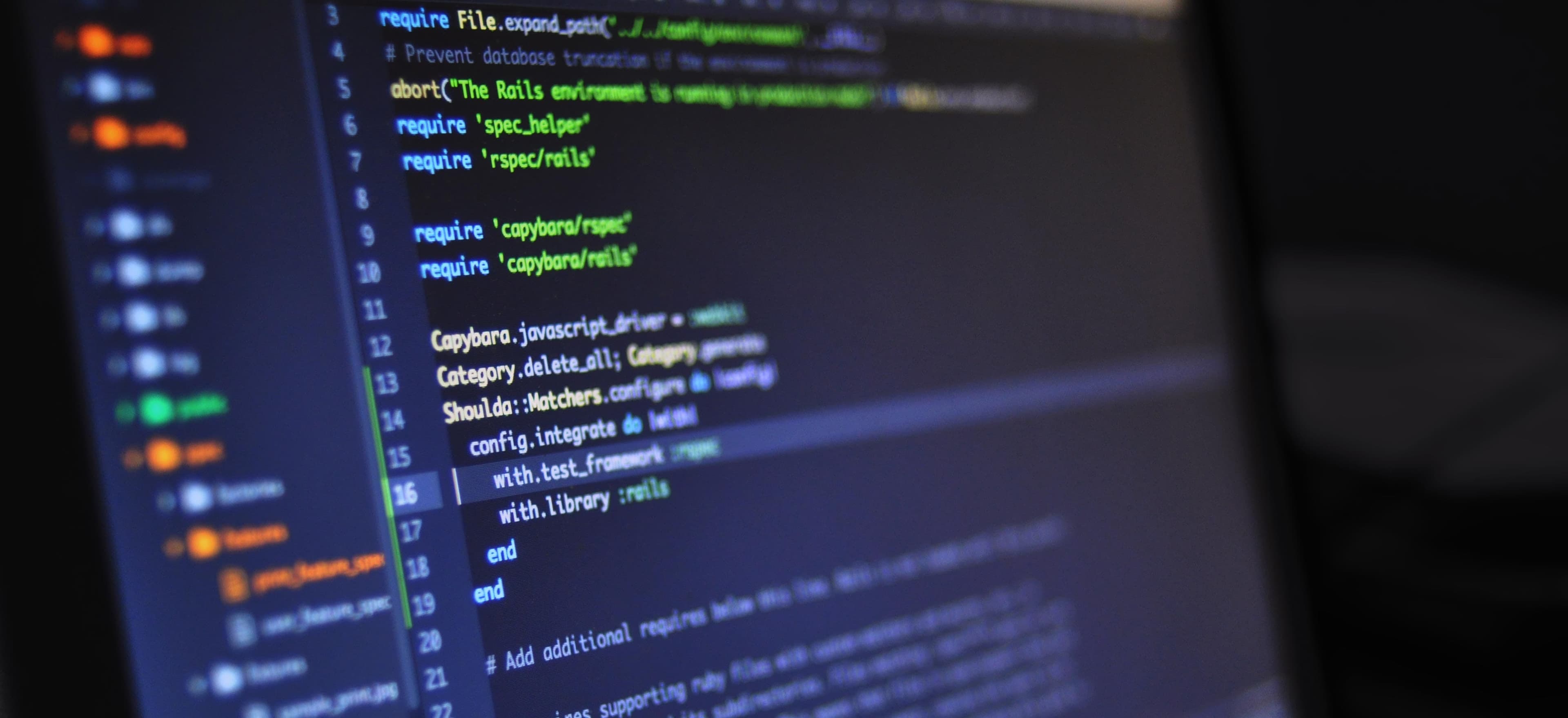
- Published on
Common Mockito Pitfalls: Avoid These Beginner Mistakes!
Mockito is one of the most popular and powerful testing frameworks in the Java ecosystem. It simplifies the process of creating mock objects for unit tests, allowing developers to focus on testing functionality rather than worrying about external dependencies. However, despite its usefulness, many newcomers fall into common pitfalls when using Mockito. In this blog post, we will explore these pitfalls and provide insights on how to avoid them, thereby making your testing process smoother and more effective.
1. Not Using @Mock Annotations Correctly
The Mistake
One of the first things beginners often overlook is the correct use of the @Mock
annotation. Failing to initialize mocks correctly can lead to NullPointerException
during testing.
Solution
Always ensure that you initialize mocks either using MockitoAnnotations.openMocks(this)
in your setup method or rely on the MockitoJUnitRunner
or MockitoExtension
for JUnit 5.
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.mockito.Mock;
import org.mockito.junit.jupiter.MockitoExtension;
@ExtendWith(MockitoExtension.class)
public class MyServiceTest {
@Mock
private MyDependency myDependency;
private MyService myService;
@BeforeEach
public void setUp() {
myService = new MyService(myDependency);
}
@Test
public void testServiceLogic() {
// Test logic here
}
}
In this example, the @ExtendWith(MockitoExtension.class)
annotation automatically initializes the @Mock
fields, ensuring a clean and error-free setup for your tests.
2. Stubbing Return Values Incorrectly
The Mistake
Another common error is improperly stubbing methods. Beginners might forget to specify the return value, leading to unexpected behavior during the test.
Solution
Use the when(...).thenReturn(...)
syntax to explicitly define what the mock should return.
import static org.mockito.Mockito.*;
@Test
public void testGetData() {
// Arrange
when(myDependency.getData()).thenReturn("Mocked Data");
// Act
String result = myService.getData();
// Assert
assertEquals("Mocked Data", result);
}
Here, we specify the behavior of the getData
method on the mock myDependency
. This ensures that when this method is called in our myService
, it returns "Mocked Data" as expected.
3. Ignoring the Order of Operations
The Mistake
Students of unit testing often miss the importance of the order of operations when it comes to interactions with mocks. This can lead to false positives and unclear test outcomes.
Solution
Always make sure your mock interactions match the expected order of method calls. Consider using InOrder
in Mockito for this purpose.
import static org.mockito.Mockito.inOrder;
@Test
public void testOrderOfOperations() {
// Arrange
MyDependency dependency1 = mock(MyDependency.class);
MyDependency dependency2 = mock(MyDependency.class);
MyService service = new MyService(dependency1, dependency2);
// Act
service.process();
// Assert
InOrder inOrder = inOrder(dependency1, dependency2);
inOrder.verify(dependency1).doFirst();
inOrder.verify(dependency2).doSecond();
}
By using InOrder
, you ensure that the interactions happen in the specified sequence, enhancing the credibility of your test.
4. Overusing Mocks
The Mistake
New developers might develop a tendency to mock everything, including simple objects that could just be instantiated. This can lead to convoluted tests that are difficult to read and maintain.
Solution
Only mock objects that have external dependencies or are costly to instantiate. For simple data objects or utility classes, prefer to use real instances.
@Test
public void testSimpleFunction() {
int result = myService.add(5, 10);
assertEquals(15, result);
}
In this case, there is no need to mock myService
because the logic is simple and straightforward. Simplified tests are easier to understand and maintain.
5. Failing to Verify Interactions
The Mistake
Verification is a critical part of testing with mocks. Beginners often forget to verify if the expected interactions with mock objects took place, leading to incomplete tests.
Solution
Utilize verify(...)
for checking interactions post-execution.
@Test
public void testSendNotification() {
// Act
myService.sendNotification("Hello!");
// Assert
verify(myDependency).notify("Hello!");
}
Adding a verification step ensures that your mocked dependencies were interacted with correctly, reflecting the intended use of the service being tested.
6. Mocking Final Classes and Static Methods
The Mistake
Mockito, by default, does not support mocking final classes and static methods. This limitation can confuse beginners who may expect their mocks to work as intended.
Solution
You can use Mockito's inline mock maker or other libraries like PowerMockito to achieve this, but do so sparingly. Always strive for cleaner architectures that reduce the need for such practices, such as Dependency Injection.
For example, if you have a final class:
final class FinalClass {
static String staticMethod() {
return "Static Method Output";
}
}
Instead of mocking, try to design your classes in such a way that you can inject dependencies to avoid mocking statics.
7. Creating Unnecessary Complex Mocks
The Mistake
Creating overly complex mock setups can lead to confusion and increase the likelihood of future errors. Beginners sometimes feel that a mock needs to be an intricate simulation of the real object.
Solution
Keep your mocks as simple and minimal as possible. Focus on what you need for your test and don't add complexity unless absolutely necessary.
// Too complex
MyDependency myDependency = mock(MyDependency.class);
// Simpler version
MyDependency myDependency = new MyDependency();
When you simplify your mocks, not only do you enhance readability, but you also make it easier for others (and future you) to understand the intention behind the tests.
In Conclusion, Here is What Matters
While Mockito is an incredibly powerful tool for unit testing in Java, it is essential to use it wisely. By being aware of these common pitfalls and implementing the solutions provided in this post, you can streamline your testing process and avoid potential roadblocks.
For more resources on Mockito, check out the official documentation and become proficient in writing robust unit tests. Happy testing!
Checkout our other articles