Common Pitfalls When Configuring Quartz with JDBC JobStore
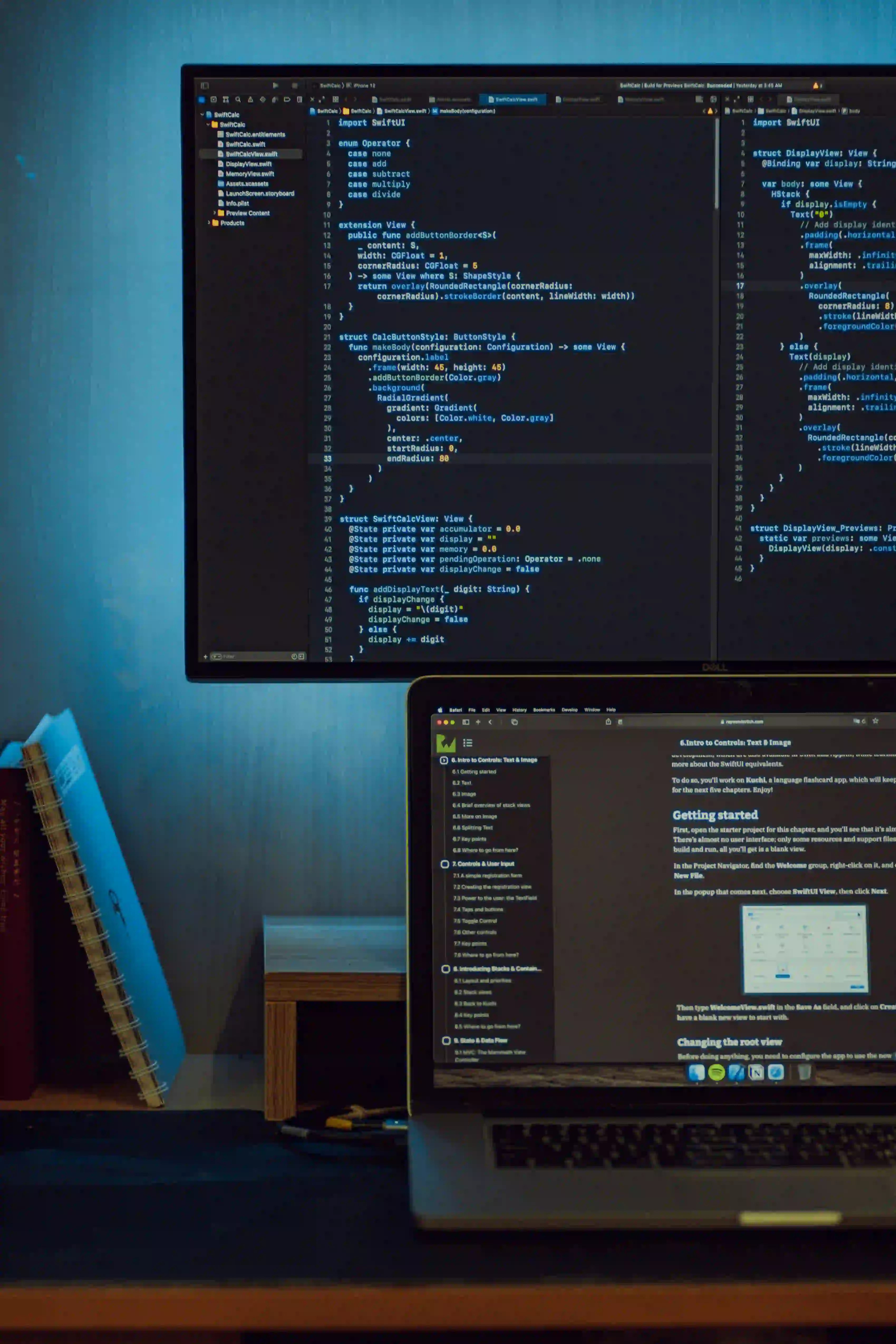
Common Pitfalls When Configuring Quartz with JDBC JobStore
Quartz is a powerful Java scheduling library used for running scheduled tasks in applications. One of the most flexible and powerful ways to store job data in Quartz is through JDBC JobStore. This method allows you to persist your jobs in a relational database, enabling support for distributed job scheduling and job recovery. However, with great power comes great responsibility, and configuring Quartz with JDBC JobStore can lead to common pitfalls if not done properly.
In this blog post, we will discuss these common pitfalls, explain their implications, and provide solutions for each. Let’s dive deep into the world of Quartz and JDBC JobStore.
Understanding JDBC JobStore
Before addressing the pitfalls, it's important to understand what JDBC JobStore is. Quartz has native job stores that allow you to schedule jobs in memory or persist them in a relational database using JDBC. The JDBC JobStore supports clustering and allows persistent job recovery, which means that failed jobs can be rescheduled if they fail during execution.
Example Configuration
Here's a sample Quartz configuration using JDBC JobStore:
# Quartz Scheduler Configuration
org.quartz.scheduler.instanceName = MyScheduler
org.quartz.scheduler.instanceId = AUTO
# JDBC JobStore settings
org.quartz.jobStore.class = org.quartz.impl.jdbcjobstore.JobStoreTX
org.quartz.jobStore.driverDelegateClass = org.quartz.impl.jdbcjobstore.StdJDBCDelegate
org.quartz.jobStore.dataSource = myDS
org.quartz.jobStore.isClustered = true
org.quartz.jobStore.clusterCheckinInterval = 20000
# DataSource configuration
org.quartz.dataSource.myDS.driver = com.mysql.cj.jdbc.Driver
org.quartz.dataSource.myDS.URL = jdbc:mysql://localhost:3306/quartz
org.quartz.dataSource.myDS.user = root
org.quartz.dataSource.myDS.password = password
org.quartz.dataSource.myDS.maxConnections = 5
Here we see the configuration of a Quartz Scheduler that interacts with a MySQL database using JDBC.
Common Pitfalls
1. Ignoring Database Compatibility
Every database has its nuances and characteristics. Not every feature available in Quartz is compatible with all databases.
Why this is a problem?
Ignoring this can lead to unexpected behavior, especially with SQL queries that are optimized for specific databases.
Solution:
Always refer to the Quartz documentation for information on database compatibility. For instance, if you're using PostgreSQL, you might need to customize certain SQL commands.
-- Example of a PostgreSQL specific SQL statement
CREATE TABLE IF NOT EXISTS QRTZ_JOB_DETAILS (
SCHED_NAME VARCHAR(120) NOT NULL,
JOB_NAME VARCHAR(200) NOT NULL,
JOB_GROUP VARCHAR(200) NOT NULL,
DESCRIPTION VARCHAR(250) NULL,
IS_DURABLE VARCHAR(1) NOT NULL,
... -- Additional fields
PRIMARY KEY (SCHED_NAME,JOB_NAME,JOB_GROUP)
);
2. Not Ensuring Proper Connection Pooling
When dealing with JDBC JobStore, efficient connection management is critical. Poorly managed connections can lead to database exhaustion and application failures.
Why this is a problem?
If all connections in your pool are consumed, Quartz will fail to execute any jobs that require a database connection.
Solution:
Optimize your connection pool settings. Ensure that you configure the maxConnections
parameter appropriately based on your load requirements.
org.quartz.dataSource.myDS.maxConnections = 10
Monitor your application and adjust as necessary, considering the job load.
3. Failing to Handle Transaction Management
Quartz uses transactions to manage job execution and state changes. If transactions aren't managed properly, jobs may not get executed as expected.
Why this is a problem?
If a transaction isn't committed or rolled back properly, it can lead to jobs being skipped or executing multiple times.
Solution:
Always wrap job execution logic in transactions and ensure proper error handling. Use scan for failed jobs and retry mechanisms as necessary.
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
TransactionTemplate txTemplate = new TransactionTemplate(transactionManager);
txTemplate.execute(status -> {
// Perform job logic here
// commit/rollback handled by TransactionTemplate
return null;
});
}
4. Not Configuring Clustering Properly
Quartz supports clustered setups that can improve the reliability and scalability of job execution. However, misconfiguration can lead to jobs being executed multiple times or not at all.
Why this is a problem?
Properly configured clustering can ensure load balancing and failover. If not configured correctly, it could undermine these benefits.
Solution:
Ensure that the isClustered
property is set to true
and that all nodes in the cluster share the same database with a common configuration.
org.quartz.jobStore.isClustered = true
It's also vital to define the clusterCheckinInterval
to a sensible value that matches the needs of your job scheduling.
5. Neglecting Job Recovery Mechanisms
Job recovery in Quartz is crucial, especially when jobs are long-running or need to persist data after failures.
Why this is a problem?
If job recovery isn’t properly set up, there may be data loss, with jobs never running again after a failure.
Solution:
Always implement Quartz's job recovery capabilities. Use durable jobs and specify recovery options in your job configuration.
JobDetail jobDetail = JobBuilder.newJob(MyJob.class)
.withIdentity("myJob", "group1")
.storeDurably()
.requestRecovery() // Enables recovery
.build();
A Final Look
Configuring Quartz with JDBC JobStore is a powerful solution for job scheduling in Java applications, but it requires careful consideration of various factors to avoid common pitfalls. By paying attention to database compatibility, ensuring optimal connection management, managing transactions, configuring clustering properly, and enabling job recovery, you can harness the full potential of the Quartz Scheduler.
For further reading and detailed examples, consider checking out the official Quartz documentation. Whether you're new or an experienced developer, understanding these common pitfalls will help you effectively implement scheduled job management in your applications.
Additional Resources
- Quartz JobStore Configuration
- Transaction Management in Spring
- Understanding JDBC Connection Pools
By paying careful attention to these areas and following best practices, you can build a robust and scalable scheduling solution for your Java applications. Happy scheduling!