Overcoming Neo4j Traversal Query Timeout Issues
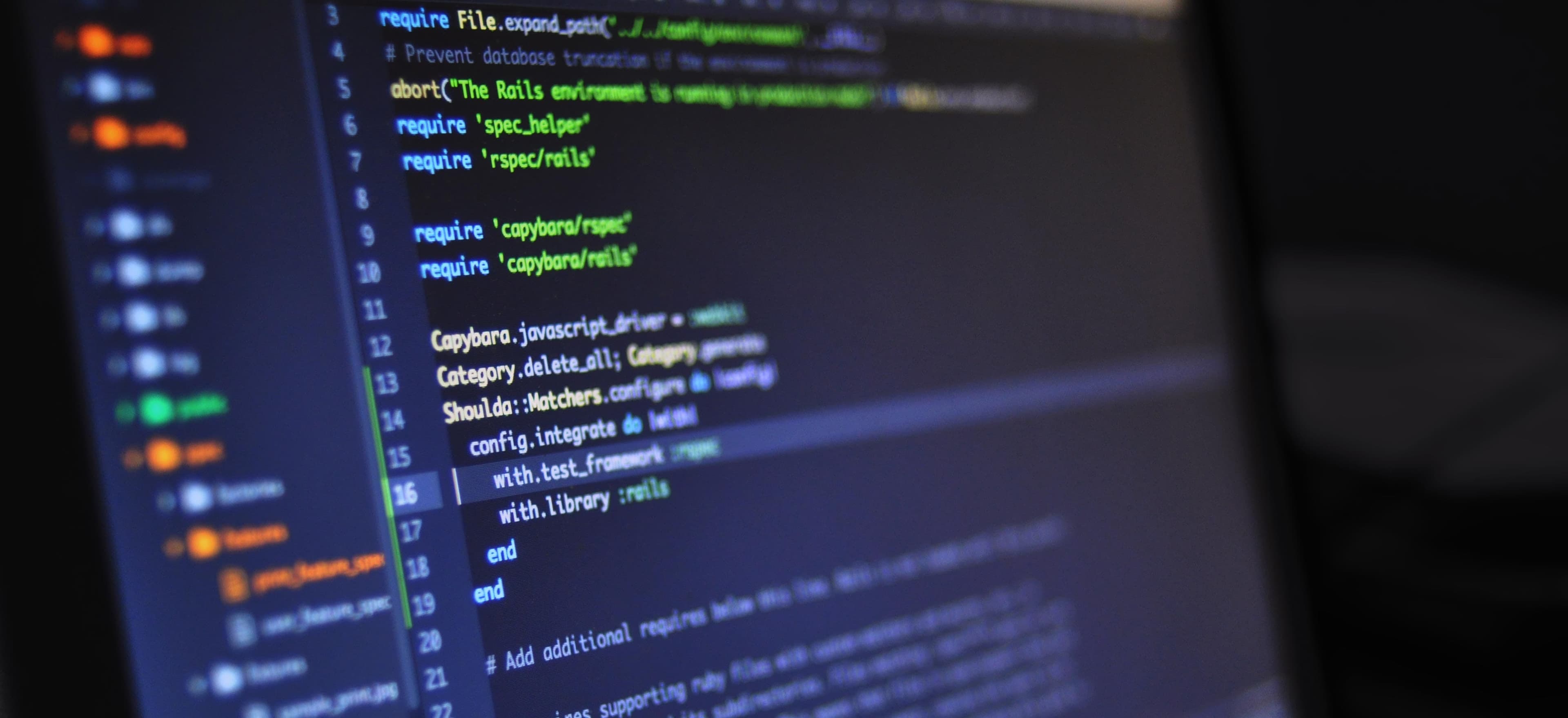
- Published on
Overcoming Neo4j Traversal Query Timeout Issues
When working with graph databases, Neo4j stands out for its flexibility and performance in querying connected data. However, developers often encounter the frustrating timeout issues when executing complex traversal queries. This blog post will explore how to identify the root causes of these timeouts, methods to prevent them, and efficient strategies to enhance query performance.
Understanding Neo4j Timeouts
Timeouts in Neo4j happen when queries take longer than a predefined duration to execute. The default timeout settings are put in place to prevent long-running queries from hogging system resources, ensuring fair allocation among users. The timeout can lead to incomplete processes and can be a source of significant inefficiencies.
Common Causes of Timeout Issues
Before diving into solutions, we need to identify the typical reasons behind these timeout problems:
- Complex Queries: Intricate traversals with a deep level of relationships tend to take longer.
- Data Volume: Large datasets can slow down query execution.
- Inefficient Indexing: Queries that do not utilize indexes effectively will suffer.
- Resource Constraints: Limited CPU and memory resources can lead to performance bottlenecks.
- Network Latency: High latency in distributed setups may contribute to timeouts.
Configuring Timeout Settings
One of the first steps to consider is adjusting timeout settings. In Neo4j, you can change these configurations in the Neo4j configuration file (neo4j.conf
) or at runtime. The parameter to modify is:
dbms.transaction.timeout=5s
This line sets the global transaction timeout to 5 seconds. Adjust this value according to your application requirements. However, increasing the timeout does not solve the root issue; it only mitigates the symptoms temporarily.
Optimizing Your Queries
When working with complex queries, optimization becomes critical. Here are several strategies that can significantly reduce execution times:
1. Use Indexes Effectively
Indexing is one of the most potent tools in optimizing queries. When you traverse nodes, ensure that you use indexes where possible.
For example, consider the following query:
MATCH (p:Person)-[:FRIENDS_WITH]->(f:Person)
WHERE p.name = 'John'
RETURN f.name
If you have a designated index on the Person
labels for the name
property, this query will run much faster.
You can create an index using:
CREATE INDEX ON :Person(name)
Why: The index allows Neo4j to quickly locate nodes based on the name
property, drastically reducing the search space.
2. Avoid Cartesian Products
Cartesian products occur when queries unintentionally return combinations of rows from different parts. This can significantly slow down query performance.
Suppose you modify the previous query to include a condition on f
:
MATCH (p:Person)-[:FRIENDS_WITH]->(f:Person)
MATCH (f)-[:LIKES]->(l:Location)
WHERE p.name = 'John'
RETURN f.name, l.name
To avoid the Cartesian product, restructure the query:
MATCH (p:Person { name: 'John' })-[:FRIENDS_WITH]->(f:Person)-[:LIKES]->(l:Location)
RETURN f.name, l.name
Why: This adjustment ensures that related nodes are matched in a single pass, preventing unnecessary combinations.
3. Limit Results
When dealing with extensive datasets, always use pagination or LIMIT to control the number of results returned by your queries.
MATCH (p:Person)-[:FRIENDS_WITH]->(f:Person)
WHERE p.name = 'John'
RETURN f.name
LIMIT 10
Why: Limiting the number of results helps reduce the data load on the database engine.
4. Utilize Query Profiling
Neo4j provides tools to help analyze your query performance. Use the PROFILE
keyword to output a graphical representation of how your query is executed.
For instance:
PROFILE MATCH (p:Person)-[:FRIENDS_WITH]->(f:Person)
WHERE p.name = 'John'
RETURN f.name
Why: Profiling provides insights into how the query is executed, helping you to identify bottlenecks.
5. Use APOC Procedures
The APOC (Awesome Procedures On Cypher) library enhances Neo4j's capabilities. It provides numerous utility functions for optimization and should be included in your Neo4j setup.
For traversing large datasets, consider using APOC procedures like apoc.periodic.iterate
. As an example:
CALL apoc.periodic.iterate(
"MATCH (p:Person) RETURN p",
"MATCH (p)-[:FRIENDS_WITH]->(f:Person) RETURN f",
{ batchSize: 1000, parallel: true }
)
Why: This allows for more efficient processing of large sets of data without stalling due to timeout issues.
Monitoring and Resource Management
In addition to optimizing queries, it is crucial to manage database resources effectively.
1. Adjust Memory Allocation
Review your Neo4j configurations regarding memory allocation settings. If sufficient resources aren't allocated, timeouts may become prevalent. Fine-tune settings such as:
dbms.memory.heap.initial_size=512m
dbms.memory.heap.max_size=8g
Why: Proper memory allocation allows the database engine to perform more efficiently when executing queries.
2. Scale Your Database
Evaluate whether your current Neo4j setup can handle the data load efficiently. If you're using Neo4j in a distributed setup, ensure you have adequate instances to share the workload.
3. Upgrade Neo4j
Keep your Neo4j installation up to date. Each new release often includes performance improvements, bug fixes, and optimizations.
The Last Word
Neo4j traversal query timeouts can be obtrusive but are manageable through proper query optimization, configuration adjustments, and effective resource management. By understanding the causes and employing the aforementioned strategies, you can not only overcome timeout issues but also enhance the overall performance of your Neo4j database.
For further reading:
- Neo4j Documentation
- APOC Documentation
- Indexing in Neo4j
By adopting these best practices, you can ensure that your Neo4j database operates seamlessly, even under heavy loads. Happy coding!
Checkout our other articles