The Hidden Cost of Method Calls in Java: What You Need to Know
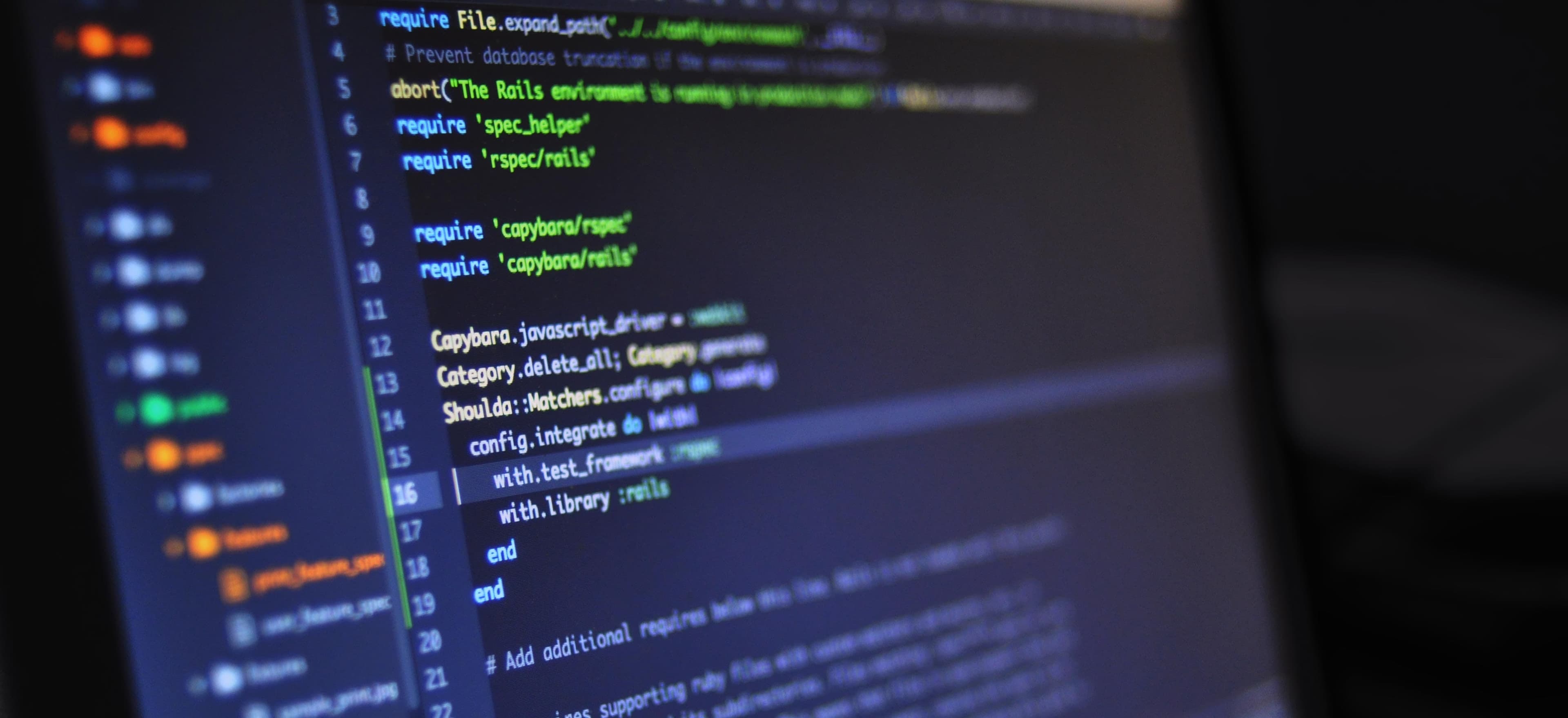
- Published on
The Hidden Cost of Method Calls in Java: What You Need to Know
Java is a powerful, high-level programming language that provides developers with a robust environment for building applications. One of the fundamental features of Java, and indeed any object-oriented programming language, is the ability to call methods. While method calls are a convenient way to structure code and promote reusability, they come with hidden performance costs that developers often overlook. In this blog post, we will explore these costs, their implications, and strategies to mitigate potential performance hits.
Understanding Method Calls in Java
Methods in Java enable developers to encapsulate functionality into logical blocks. This allows for modular designs and easier maintenance. For example, in a typical Java class, we might define a method like this:
public void printGreeting(String name) {
System.out.println("Hello, " + name + "!");
}
In this example, printGreeting
is a method that prints a greeting. You can call this method from anywhere in your code using:
printGreeting("Alice");
The Basics of Method Call Overhead
When a method is called, several things happen behind the scenes:
-
Stack Frame Creation: Whenever a method is invoked, a new stack frame is created. This stack frame holds data, such as local variables and the return address.
-
Parameter Passing: Parameters are passed to the method, either by value or by reference, which requires additional memory.
-
Execution Context Switch: The CPU needs to switch context, which involves multiple processor-level transitions.
These steps can introduce overhead. While for occasional calls this may not be visible, repeated or nested method calls can accumulate a significant performance cost.
The Cost of Method Calls
Performance Impact
The exact cost of a method call can vary based on several factors, including:
-
JVM optimizations: The Java Virtual Machine (JVM) performs several optimizations, such as Just-In-Time (JIT) compilation, which can reduce overhead but doesn’t eliminate it entirely.
-
Method Inlining: The JVM might inline methods, especially non-private, small methods. This can eliminate the call by substituting the method body directly, which considerably reduces overhead.
-
Call Depth: Deeper call stacks may lead to higher cumulative costs. Each frame switch contributes to the total execution time.
Example to Illustrate Overhead
Consider the following two versions of a computation:
Without Method Calls
public int computeSum(int n) {
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
With Method Calls
public int computeSum(int n) {
return calculateSum(n);
}
private int calculateSum(int n) {
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
In the second version, method calls are introduced. While this improves code readability and maintainability, it also incurs the overhead of stack frame creation and context switching.
Microbenchmarking
To quantify the performance impact, consider using a microbenchmarking framework like JMH (Java Microbenchmark Harness). Here’s a simplified benchmark example:
import org.openjdk.jmh.annotations.*;
@State(Scope.Thread)
public class MethodCallBenchmark {
@Benchmark
public int withoutMethodCall() {
return computeSum(1000);
}
@Benchmark
public int withMethodCall() {
return computeSumWithMethod(1000);
}
public int computeSum(int n) {
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
public int computeSumWithMethod(int n) {
return calculateSum(n);
}
private int calculateSum(int n) {
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
}
Using JMH allows you to get accurate results regarding method call overhead. You can analyze the performance variations under different scenarios.
For more information on microbenchmarking in Java, refer to the official JMH GitHub page.
When Method Calls Are Necessary
Despite the overhead, it's crucial to remember that method calls are not inherently bad. They play a significant role in maintaining clean, organized, and scalable code. The cost-benefit analysis often favors method calls for most applications due to:
- Ease of maintenance: Well-structured methods are easier to manage.
- Reusability: Methods can be reused, avoiding code duplication.
- Testing: Isolated methods can be unit tested independently.
Performance Optimization Techniques
To balance between code quality and performance, consider employing the following strategies:
1. Use Method Inlining Where Possible
The JVM often optimizes small methods by inlining them. While you can’t force inlining, writing smaller methods can encourage the JVM to optimize them.
2. Avoid Unnecessary Calls
If a method is called frequently without needing to be, consider inlining it manually or refactoring to avoid excessive method calls in a tight loop.
3. Use Static Methods
Static methods are often more efficient because they do not require an instance of a class to be invoked, and the JVM can optimize their invocations better.
public static int staticMethod(int x) {
return x * 2;
}
4. Profile Your Code
Regular profiling with tools like VisualVM or YourKit can help identify a method call overhead in your application. Analyze the hotspots and refactor them as needed before they become bottlenecks.
For comprehensive profiling methods, refer to the VisualVM documentation.
Final Considerations
While method calls in Java are essential for creating readable, maintainable, and reusable code, developers should be aware of their hidden costs, especially in performance-critical applications. It's crucial to strike a balance between clean architecture and execution speed.
By leveraging profilers and understanding JVM optimizations, you can make informed decisions that align with your application's performance goals. Embrace method calls, but do so with a pragmatic approach that considers their implications. After all, the goal is not just to write code, but to write efficient and effective code.
For further reading on Java performance techniques, you might find these resources helpful:
- Java Performance: The Definitive Guide
- Effective Java
Happy coding!