Mastering New Features in Effective Java Third Edition
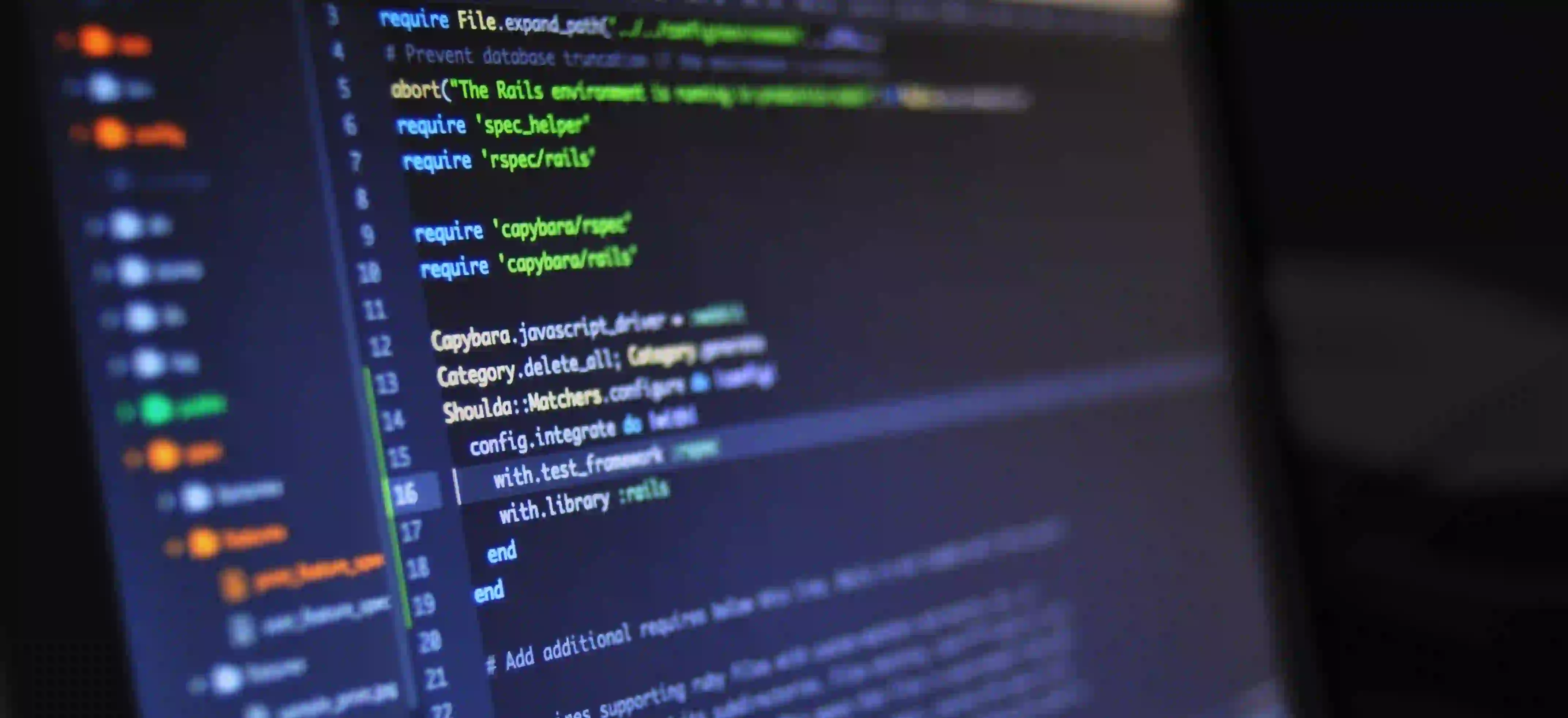
Mastering New Features in Effective Java Third Edition
In the world of software development, Java remains one of the top programming languages used across various industries. With the release of "Effective Java," Joshua Bloch has enlightened developers on best practices to write efficient, maintainable, and high-quality Java code. The third edition of this seminal work introduces several newer concepts relevant to modern development. In this blog post, we will dive deep into the new features of Effective Java, Third Edition and explore their practical applications.
1. The Power of Static Factory Methods
One of the most significant features highlighted in the new edition is the use of static factory methods over constructors. While constructors have been a staple in Java, static factory methods offer developers enhanced flexibility, readability, and control.
Example: Static Factory Method
public class Complex {
private final double real;
private final double imaginary;
private Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public static Complex of(double real, double imaginary) {
return new Complex(real, imaginary);
}
}
In this code, the of
static method replaces a traditional constructor. The key benefits are:
- Descriptive Names: You can give the static factory method any name that helps convey its purpose, improving code readability.
- Controlled Instantiation: You can add logic to the static factory method to control the number of instances created, enhancing memory management.
For a deeper dive into the advantages of static factories, you can refer to this article.
2. Enhancing Enum Usages
Enums in Java have evolved significantly, and the new edition provides insights into leveraging their power fully. Enums are ideal for representing fixed sets of constants but can also encapsulate complex behavior.
Example: Enum with Behavior
public enum Operation {
PLUS("+") {
@Override
public double apply(double x, double y) {
return x + y;
}
},
MINUS("-") {
@Override
public double apply(double x, double y) {
return x - y;
}
};
private final String symbol;
Operation(String symbol) {
this.symbol = symbol;
}
public abstract double apply(double x, double y);
@Override
public String toString() {
return symbol;
}
}
In this example, the Operation
enum defines two operations, PLUS and MINUS, each implementing their logic in the apply
method. This approach leverages polymorphism, allowing us to keep related data and methods tied to the enum entries.
Why Use Enums This Way?
Using enums with behavior encapsulated within them increases modularity and provides clear and explicit control over each type’s implementation logic. Additionally, enums make the code easier to read and debug.
3. Use of Optional to Avoid Nulls
Null references are often termed as the "billion-dollar mistake." They can lead to pesky NullPointerExceptions if not handled appropriately. The introduction of the Optional
class in Java 8 provides a powerful way to avoid these pitfalls.
Example: Using Optional
import java.util.Optional;
public class User {
private Optional<Address> address;
public User(Optional<Address> address) {
this.address = address;
}
public String getCity() {
return address.map(Address::getCity).orElse("Unknown");
}
}
Here, by encapsulating Address
in an Optional
, we can avoid a null reference. The map
and orElse
methods enable a more fluent and expressive way to handle potential nulls.
Why Use Optional?
Using Optional
enhances code clarity. Instead of checking for null explicitly, developers can chain their operations—making the code succinct and expressive. For more information on how to effectively use Optional
, check out this guide on ServiceStack.
4. Varargs with Generics
Another feature given emphasis is the proper usage of varargs in conjunction with generics, which is often misunderstood. Varargs can lead to type safety issues, especially when they work with generic types.
Example: Varargs Method
import java.util.Arrays;
public class VarargsExample {
@SafeVarargs
public static <T> void printAll(T... elements) {
Arrays.stream(elements).forEach(System.out::println);
}
public static void main(String[] args) {
printAll("Hello", "World", "Varargs", "Java");
}
}
With the above code using the @SafeVarargs
annotation, we ensure that the method remains type-safe. This declaration is useful when you use varargs with generic types, reducing the risk of heap pollution.
Why Is This Important?
Understanding the nuances of varargs with generics allows for cleaner code design and avoids the common pitfalls associated with type safety. The @SafeVarargs
annotation signals to others (and the compiler) that you're aware of the implications, leading to better maintainability.
5. Leveraging Stream API
The Stream API, introduced in Java 8, offers a new way to process data efficiently. Utilizing this API can lead to more succinct code compared to traditional iterations.
Example: Using Streams
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.stream()
.filter(name -> name.startsWith("A"))
.map(String::toUpperCase)
.forEach(System.out::println);
}
}
In the above code, we filter names starting with "A," convert them to uppercase, and print them—all in a few concise lines. This method promotes a functional programming style and enhances clarity.
Why Use Streams?
The Stream API enables developers to express complex data processing sequences in a much more readable manner while allowing for optimizations like parallel processing. For more in-depth concepts on the Stream API, you can visit the official Oracle documentation.
The Last Word
Mastering the new features in Effective Java Third Edition is crucial for any Java developer who aims to write modern, efficient, and maintainable code. Embracing static factory methods, thoughtful enum usage, the Optional
type, safe varargs, and the Stream API can drastically improve your programming approach.
By investing time in understanding these practices, you'll be well on your way to becoming a more proficient Java developer. Happy coding, and may your journey through the vast world of Java be both enlightening and rewarding!
Incorporating these new ideas not only aligns you with contemporary Java practices but also enhances the robustness and readability of your code. The techniques mentioned here provide the foundation for creating high-quality Java applications, and mastering them will position you as a valuable asset in any software development team.