Common Pitfalls for Beginners in Java Machine Learning
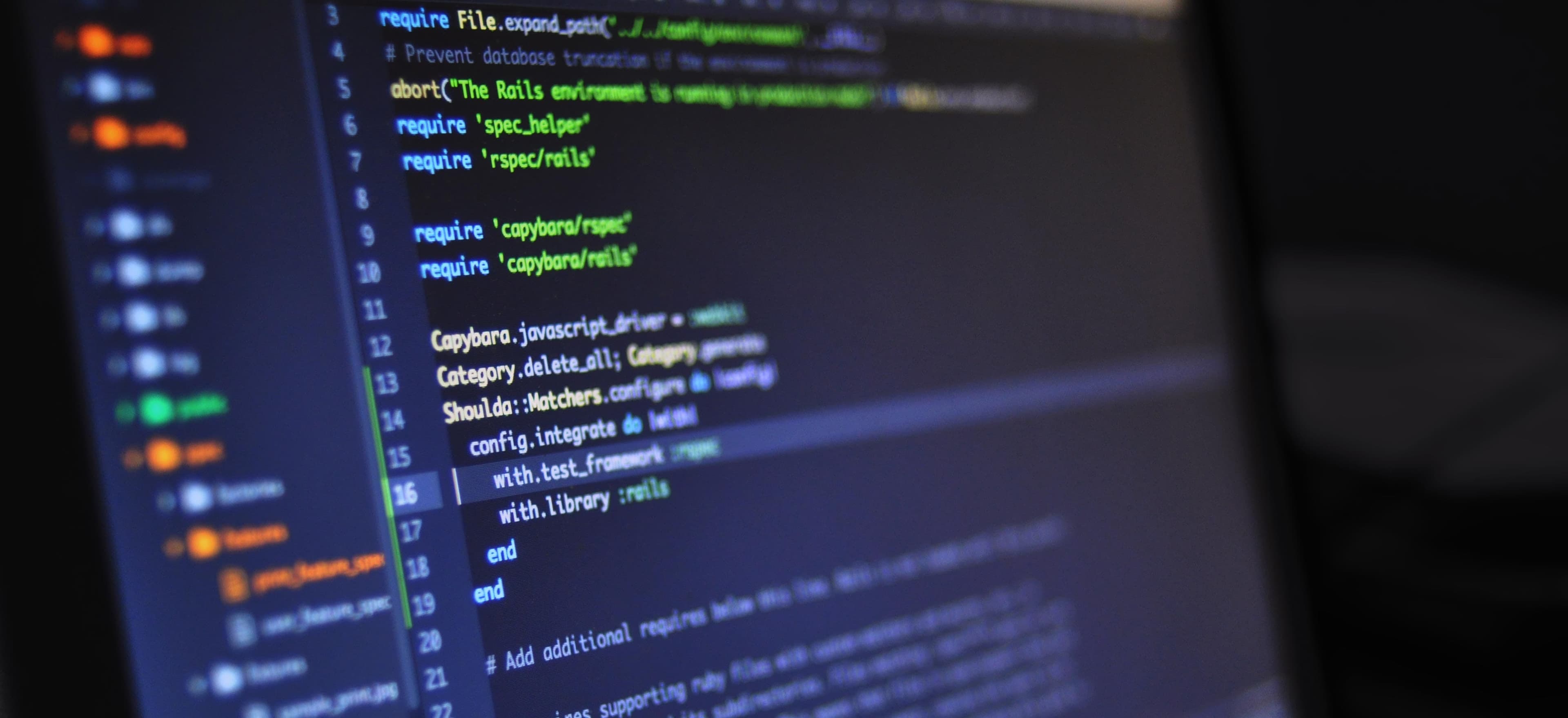
- Published on
Common Pitfalls for Beginners in Java Machine Learning
Java is a robust language that has made significant strides in the Machine Learning (ML) domain over the years. From its rich ecosystem of libraries to community support, it often serves as a great introduction for those looking to delve into ML. However, like any programming discipline, beginners often run into hurdles. Understanding these common pitfalls can significantly enhance your learning curve and help you avoid frustrations.
In this article, we will explore the most common pitfalls faced by beginners in Java Machine Learning, backed up with explanations, code snippets, and best practices.
1. Skipping the Basics of Java
Understanding Why It's Important
Many newcomers jump straight into Machine Learning without solidifying their understanding of Java fundamentals. This creates a lack of clarity in writing clean and efficient code.
Elaboration
Before diving into complex ML algorithms, ensure that you're well-versed with:
- Syntax: Getting comfortable with Java syntax will help you translate ML concepts into code more effectively.
- Data Structures: Knowledge of collections, arrays, and lists is crucial since you'll often need to manipulate data.
- Object-Oriented Programming (OOP): Most ML libraries are designed with OOP principles which are essential for creating effective ML models.
Suggestion
Make sure to brush up on your Java skills. Consider resources like Java Programming and Software Engineering Fundamentals on Coursera.
2. Ignoring Data Preprocessing
Preparing Your Data
In Machine Learning, the quality of data is everything. Beginners often ignore the preprocessing stage, leading to poor model performance.
Elaboration
Data preprocessing includes:
- Normalization: Ensuring that your data is on a similar scale.
- Cleaning: Handling missing values and removing outliers.
- Encoding: Converting categorical variables into numerical form.
Code Snippet: Data Normalization
public class DataNormalization {
public static double[] normalize(double[] data) {
double min = Double.MAX_VALUE;
double max = Double.MIN_VALUE;
for (double value : data) {
if (value < min) min = value;
if (value > max) max = value;
}
double[] normalizedData = new double[data.length];
for (int i = 0; i < data.length; i++) {
normalizedData[i] = (data[i] - min) / (max - min);
}
return normalizedData;
}
}
Why This Matters
Normalization is crucial because it helps the model to converge quickly during the optimization process. It's particularly important when using algorithms that are sensitive to the scale of the data.
3. Underestimating the Importance of Libraries
Proficiency in Using Libraries
Java provides numerous ML libraries such as Weka, Deeplearning4j, and Apache Spark ML. Beginners often either fail to use them optimally or don't leverage them at all.
Elaboration
Libraries abstract a lot of complexity and allow you to focus on building and tuning ML models rather than worrying about the underlying mathematics.
Code Snippet: Using Weka Library
import weka.classifiers.Classifier;
import weka.classifiers.trees.J48;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class WekaExample {
public static void main(String[] args) throws Exception {
DataSource source = new DataSource("dataset.arff");
Instances data = source.getDataSet();
data.setClassIndex(data.numAttributes() - 1); // Set the last attribute as the class
Classifier cls = new J48(); // Create a J48 decision tree classifier
cls.buildClassifier(data); // Build the classifier
System.out.println(cls);
}
}
Why This Matters
Using established libraries allows you to quickly implement and iterate through different models, enabling you to understand what works best for your dataset.
4. Not Tuning Hyperparameters
Beyond Initial Model Building
Once you've built a model, it's easy to assume that your job is done. However, hyperparameters can significantly affect model performance.
Elaboration
Hyperparameter tuning involves adjusting parameters like:
- Learning rate
- Depth of the trees (in tree-based algorithms)
- Regularization parameters
Code Snippet: Grid Search for Hyperparameter Tuning
import weka.classifiers.Classifier;
import weka.classifiers.trees.J48;
import weka.classifiers.meta.GridSearch;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class HyperparameterTuning {
public static void main(String[] args) throws Exception {
DataSource source = new DataSource("dataset.arff");
Instances data = source.getDataSet();
data.setClassIndex(data.numAttributes() - 1);
GridSearch grid = new GridSearch();
grid.setClassifier(new J48());
grid.setEvaluationMeasure("accuracy");
// Set ranges for hyperparameters
grid.setOptions(new String[] {"-C", "0.25", "-M", "2"});
grid.buildClassifier(data); // Perform grid search
System.out.println("Best model: " + grid.getBestClassifier());
}
}
Why This Matters
Improper hyperparameters can lead to overfitting or underfitting. A tailored model for your data can significantly improve results.
5. Failing to Evaluate the Model
Evaluating Your Work
A model might perform well on training data but may not generalize effectively to unseen data. Beginners often overlook this crucial evaluation stage.
Elaboration
You should:
- Split your dataset into training and testing sets.
- Use cross-validation to validate model robustness.
- Employ metrics like accuracy, precision, recall, and F1-score.
Code Snippet: Model Evaluation
import weka.classifiers.Classifier;
import weka.classifiers.Evaluation;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class ModelEvaluation {
public static void main(String[] args) throws Exception {
DataSource source = new DataSource("dataset.arff");
Instances data = source.getDataSet();
data.setClassIndex(data.numAttributes() - 1);
Classifier cls = new J48();
cls.buildClassifier(data);
Evaluation eval = new Evaluation(data);
eval.crossValidateModel(cls, data, 10, new java.util.Random(1));
System.out.println(eval.toSummaryString());
}
}
Why This Matters
A good evaluation will help ensure that your model not only learns but also performs well on unseen data — a fundamental requirement in machine learning.
The Closing Argument
Stepping into Java Machine Learning is an exciting journey filled with opportunities for growth and learning. However, by being aware of common pitfalls such as skipping Java fundamentals, ignoring data preprocessing, underestimating library usage, overlooking hyperparameter tuning, and failing to evaluate models, you can set yourself on a successful path.
Remember, effective learning is not just about knowing the right algorithms but understanding the entire pipeline from data collection to model evaluation. Keep experimenting, iterate, and seek feedback.
For a deep dive into the various machine learning algorithms available in Java, consider exploring Java Machine Learning by example, which provides practical insights into the realm of ML with Java.
Happy coding!
Checkout our other articles