Common Pitfalls When Using Spring Cloud Contracts with PCF
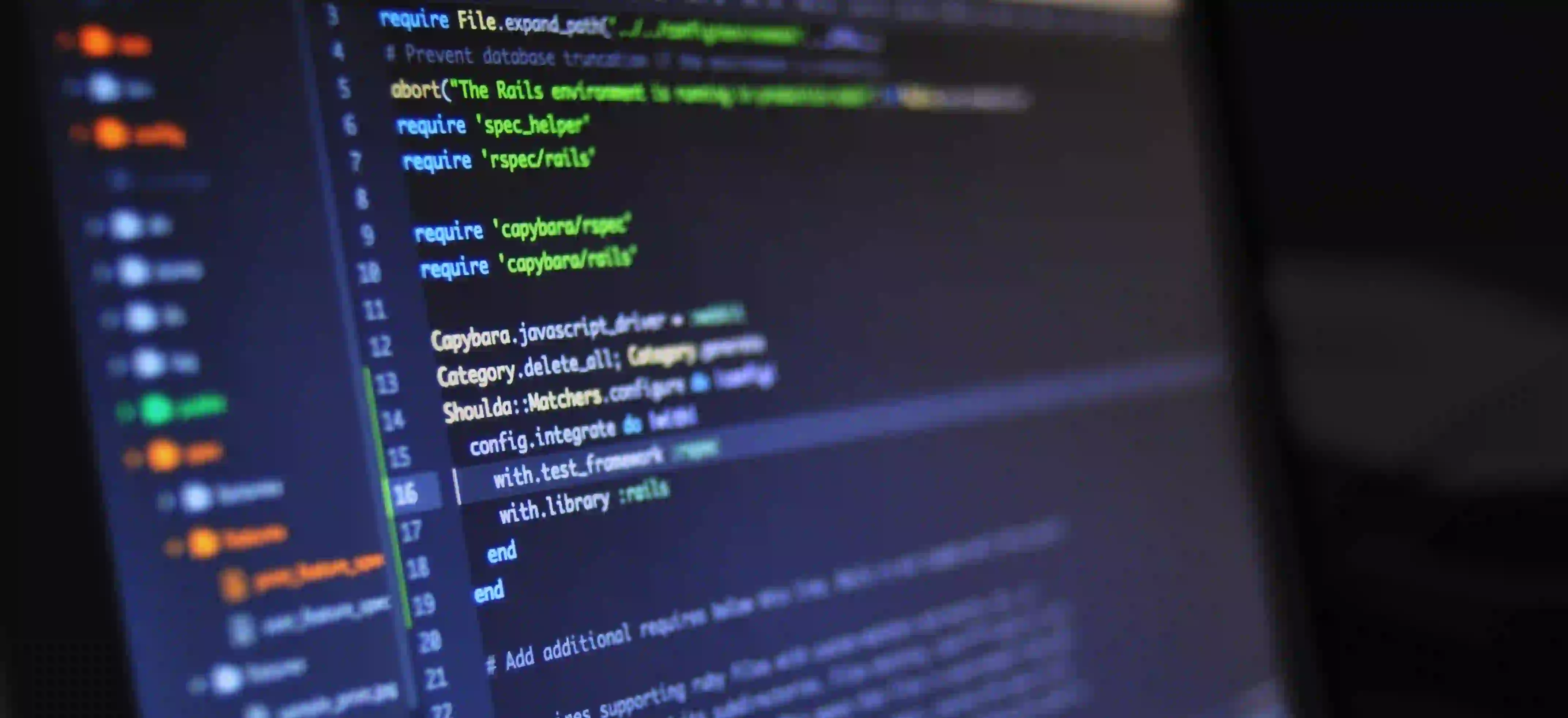
Common Pitfalls When Using Spring Cloud Contracts with PCF
In the ever-evolving landscape of microservices, ensuring that services communicate effectively is paramount. This is where Spring Cloud Contract shines, enabling the definition of contracts between service consumers and providers. When deploying applications to a cloud platform like Pivotal Cloud Foundry (PCF), however, developers may encounter certain challenges. This blog post will explore common pitfalls when using Spring Cloud Contracts with PCF, offering insights to help you navigate these issues successfully.
What is Spring Cloud Contract?
Before diving into pitfalls, it is crucial to understand what Spring Cloud Contract (SCC) is. SCC is a framework that allows teams to define contracts for communication between service consumers and producers. By using contracts, you can create consumer-driven contracts (CDC), which ensure that the producer adheres to the expectations of the consumer.
Key Features of Spring Cloud Contract:
- Testing: Helps ensure that services are compatible.
- Documentation: Automatically generates documentation based on contracts.
- Stub Generation: Creates stubs from consumer contracts for rapid testing.
Integrating Spring Cloud Contract with PCF
When deploying microservices to PCF that utilize Spring Cloud Contract, developers often run into challenges. Below are the most common pitfalls along with solutions and best practices.
1. Misalignment of API Versioning
Pitfall: One of the primary issues developers face is not properly maintaining API versioning through contracts. Inconsistent versioning can lead to unexpected behavior in services.
Solution: Always version your contracts alongside your APIs. Use semantic versioning whenever possible to manage changes. By aligning versioning, you ensure that both consumers and producers are on the same page.
Example:
// contracts/your-service.groovy
contract {
request {
method 'GET'
urlPath('/api/v1/products')
}
response {
status 200
body([
id: 1,
name: 'Product 1'
])
headers {
contentType(applicationJson())
}
}
}
By specifying /api/v1/products
, you clarify which version of the API consumers are invoking.
2. Insufficient Mocking Configurations
Pitfall: When running tests, developers sometimes forget to configure mocks properly, leading to discrepancies between expected and actual outputs.
Solution: Define your stub repository accurately. Ensure that tests are referencing the correct MockRestServiceServer instances to avoid issues during contract verification.
Example:
@Test
public void testGetProduct() {
mockService
.expect(requestTo("/api/v1/products/1"))
.andRespond(withSuccess("{\"id\":1,\"name\":\"Product 1\"}", MediaType.APPLICATION_JSON));
// Call your service logic that retrieves the product here
}
Here, mocking the expected service response ensures that your tests align with your contracts.
3. Ignoring Environmental Differences
Pitfall: Sometimes, developers assume that a contract will work in both local and PCF environments without testing in both settings, leading to surprises in production.
Solution: Always test your contracts in an environment that closely mirrors production. Use environment variables to adapt contract behavior based on deployment specifics.
4. Overlooking Security Concerns
Pitfall: Security configurations can be overlooked when generating contracts. Missing authentication or authorization can lead to exposed endpoints.
Solution: Incorporate security contexts into your contracts. Define security configurations explicitly in your contract stubs, so there's never ambiguity about secure access.
Example:
contract {
request {
method 'GET'
urlPath('/api/v1/secure/products')
headers {
header('Authorization', 'Bearer someToken')
}
}
response {
status 200
body([...])
}
}
In this case, your contract clearly exercises security measures, setting expectations for authentication.
5. Not Using Consumer-Driven Contracts
Pitfall: Developers often create contract tests only from the provider's perspective, ignoring the consumer-driven aspects.
Solution: Work closely with your consumers to ensure that contracts meet their requirements. This collaboration prevents breaking changes and fosters better team dynamics.
6. Ignoring the Importance of Documentation
Pitfall: Some developers may neglect the documentation aspect of Spring Cloud Contracts, which can lead to confusion for both providers and consumers.
Solution: Utilize Spring Cloud Contract’s auto-documentation capabilities effectively. This feature helps clarify how services interact without needing extensive manual documentation.
# Documentation for Product API
## GET /api/v1/products
### Response
- Returns a list of products.
### Security
- Requires Authorization.
7. Backup Plans for Testing Failures
Pitfall: Depending solely on contract tests can cause delays if those tests fail. Without proper backup plans, the deployment process may be halted.
Solution: Implement fallback testing strategies and ensure that regression tests cover fundamental functionalities. This dual approach allows for flexibility in the delivery pipeline.
Lessons Learned
Integrating Spring Cloud Contracts with PCF presents its challenges, but understanding and avoiding these common pitfalls can lead to smoother deployments and more robust microservices architectures. Always focus on versioning, mock configurations, environment alignment, security, consumer collaboration, documentation, and contingency planning.
For detailed guides on Spring Cloud Contracts, check out the official Spring documentation here.
Moreover, if you face scaling issues with PCF or need to streamline your microservices, take a look at the comprehensive resources available on the Pivotal Cloud Foundry website here.
Working closely with these strategies will enhance your microservices development journey, resulting in reliable communication between services. Happy coding!