Unlocking Java 8 Optional: Beyond Just Null Handling
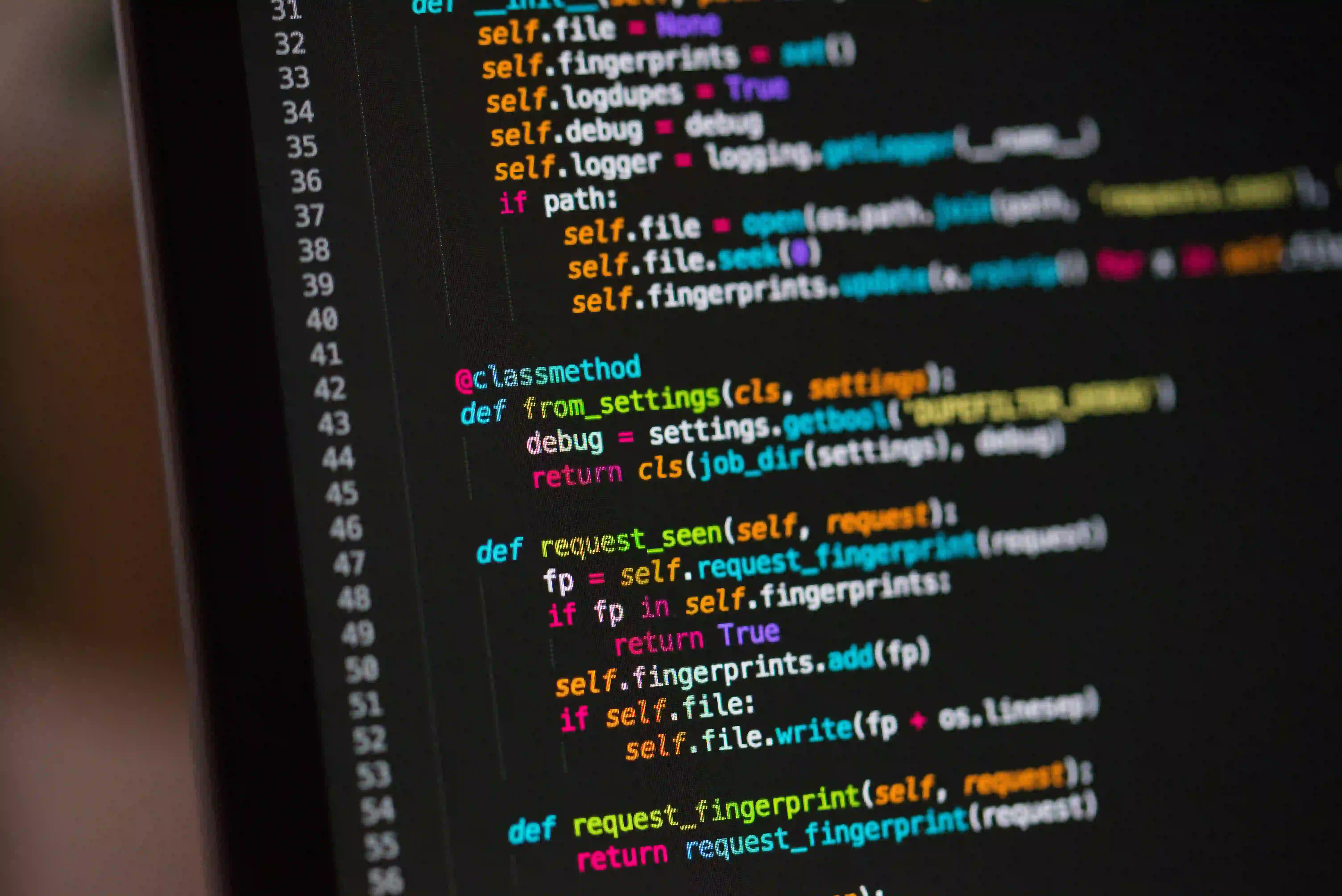
Unlocking Java 8 Optional: Beyond Just Null Handling
Java has long been criticized for its handling of null references. The infamous NullPointerException
has caused countless headaches for developers. With the introduction of Java 8, a new solution emerged: Optional
. While many developers initially employed Optional
as a way to avoid null references, its capabilities extend far beyond basic null handling. In this article, we'll explore the full potential of Optional
, its role in functional programming, best practices, and examples that illustrate its effectiveness.
What is Optional
?
Optional
is a container object which may or may not contain a non-null value. It's a way to represent optionality in your code, promoting better error handling and improving readability. Rather than returning null
, methods can now return an instance of Optional
. This encourages developers to explicitly handle the absence of a value.
Why Use Optional
?
The primary reasons for using Optional
include:
- Clearer Intent: An
Optional
clearly indicates that a value may not be present, improving the readability of your code. - Avoiding NullPointerExceptions: By using
Optional
, you can significantly reduce the occurrences ofNullPointerException
. - Functional Style:
Optional
integrates neatly into Java’s functional programming constructs, allowing for more expressive code.
Basic Usage of Optional
Here's a quick look at how to create an Optional
, check for value presence, and extract values safely.
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
// Create an Optional object
Optional<String> optionalString = Optional.of("Hello, Java 8!");
// Check if value is present
if(optionalString.isPresent()) {
System.out.println(optionalString.get()); // "Hello, Java 8!"
}
}
}
Commentary on the Code
In the above code, Optional.of()
is used to create a non-empty Optional
. The isPresent()
method checks for the existence of a value before calling get()
. This distinction demonstrates how Optional
encourages developers to handle scenarios where a value might be absent.
Advanced Features of Optional
1. Alternative Value with orElse()
Sometimes you may want to provide a default value if the Optional
is empty. The orElse()
method allows you to do just that.
public class OptionalExample {
public static void main(String[] args) {
Optional<String> absentValue = Optional.empty();
String result = absentValue.orElse("Default Value");
System.out.println(result); // Output: "Default Value"
}
}
Commentary on the Code
In this example, Optional.empty()
generates an empty Optional
. The subsequent call to orElse()
provides a default value when absentValue
does not contain a non-null result.
2. Using orElseGet()
Unlike orElse()
, which takes a value, orElseGet()
takes a supplier—which can be beneficial for performance in certain scenarios.
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
Optional<String> absentValue = Optional.empty();
String result = absentValue.orElseGet(() -> {
// Some expensive computation
return "Computed Default Value";
});
System.out.println(result); // Output: "Computed Default Value"
}
}
Commentary on the Code
Using orElseGet()
, we can defer the execution of the computation until it's determined that the Optional
is indeed empty. This is significant when the computation is resource-intensive.
3. Chaining with map()
Optional
supports functional programming paradigms, allowing for method chaining through map()
. This is particularly useful for transforming values in a safe, concise manner.
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
Optional<String> name = Optional.of("Java");
Optional<String> uppercasedName = name.map(String::toUpperCase);
System.out.println(uppercasedName.get()); // Output: "JAVA"
}
}
Commentary on the Code
Here, map()
transforms the contained value into its uppercase equivalent. If name
were empty, uppercasedName
would also be an empty Optional
, preventing any exceptions.
4. Filtering with filter()
Yet another powerful functionality of Optional
is the ability to filter values based on predicates.
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
Optional<String> name = Optional.of("Java");
// Filter based on condition
Optional<String> filteredName = name.filter(n -> n.length() > 4);
System.out.println(filteredName.get()); // Output: "Java"
}
}
Commentary on the Code
filter()
checks if the name length is greater than four characters. If it is, the Optional
continues to hold the value; otherwise, it becomes empty. This makes it easy to avoid unnecessary checks or exceptions in your code.
Common Mistakes When Using Optional
1. Overusing Optional
While Optional
is a robust solution, it is not a panacea. It’s designed for use cases where the absence of a value is a legitimate scenario. Consider the examples where it may make more sense to use exceptions or simply return primitive types.
2. Confusing Optional
with Containers
It is crucial to remember that Optional
is not a replacement for collections. Using Optional
when a list or map is needed can add unnecessary complexity to your code.
Best Practices for Using Optional
- Prefer Returning Optional: When a method might not have a value, return an
Optional
instead of null. - Avoid
Optional
in Fields: UsingOptional
in class fields can introduce unnecessary overhead. Stick to method return types. - Combine Functional Style: Leverage
map()
,flatMap()
, andfilter()
for a more functional approach, reducing the need for multiple null checks.
In Conclusion, Here is What Matters
Optional
represents a significant evolution in Java programming, providing a clear and effective paradigm for dealing with potential absent values. Its rich set of features allows developers to write cleaner, more maintainable code. By shifting the focus from null checks to a more functional approach, you can unlock the full potential of Java 8's Optional
.
To dive deeper into Java 8 features, consider checking out the official Java Documentation.
Incorporating Optional
into your coding practices isn't just about avoiding null references—it's about embracing a way of thinking that promotes clearer, more expressive code. Start unlocking Java's potential and happy coding!
This blog post has provided a comprehensive overview of the Java 8 Optional
. The combination of code snippets and their reasoning gives a rounded perspective that can benefit both novice and seasoned developers.