Common Selenium Testing Mistakes and How to Avoid Them
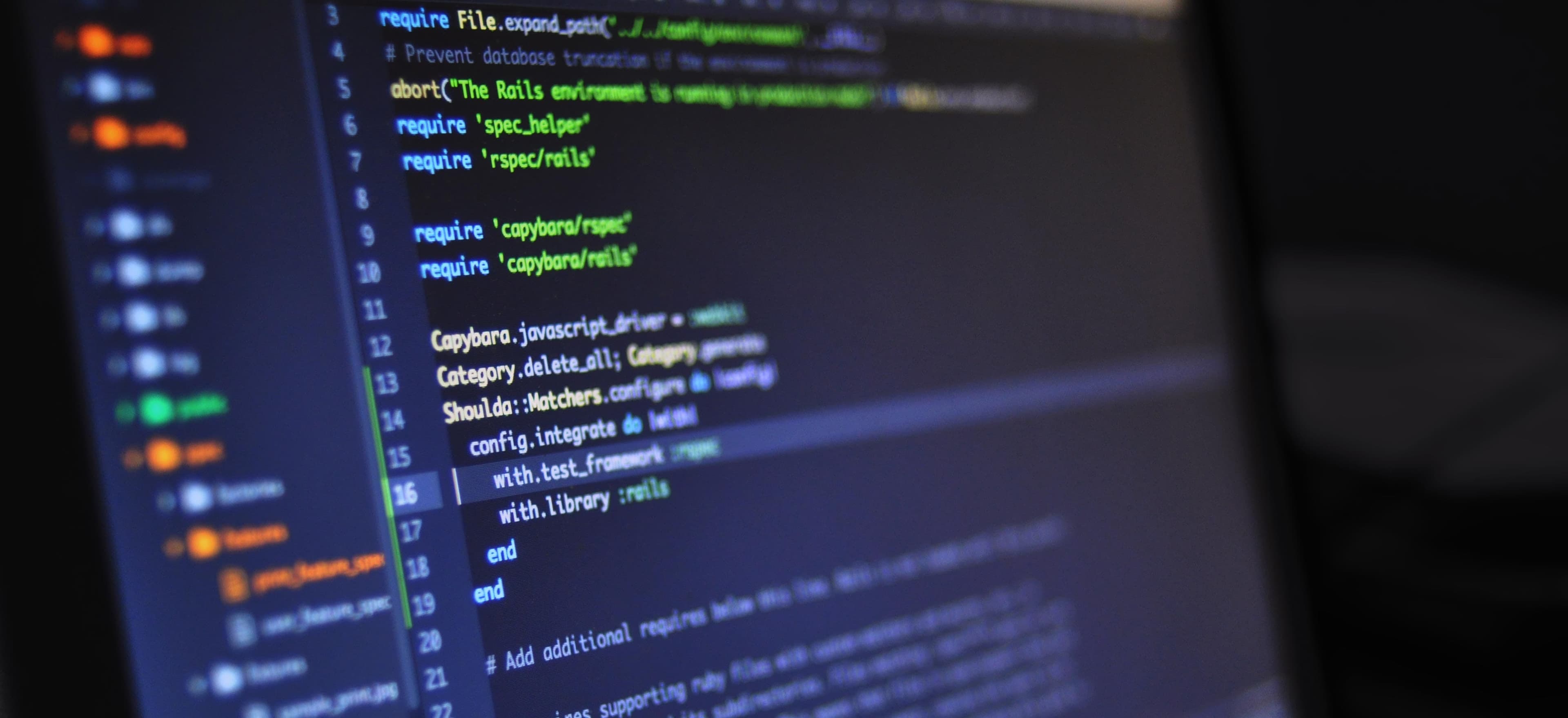
- Published on
Common Selenium Testing Mistakes and How to Avoid Them
Selenium is one of the leading frameworks for automated web application testing. Yet, many developers and testers make common mistakes that can hinder the efficiency and effectiveness of their testing efforts. Understanding these pitfalls and how to avoid them can significantly improve your Selenium test automation experience.
In this blog post, we will highlight some frequent mistakes made by Selenium users and offer solutions to help you avoid these missteps.
1. Not Implementing a Robust Test Framework
The Mistake
Many beginners jump straight into writing Selenium tests without considering the underlying structure of their test framework. This can lead to a disorganized codebase and challenges in maintaining and scaling tests.
The Right Approach
Establish a solid test framework that incorporates patterns such as Page Object Model (POM) or the Model-View-Controller (MVC) design. This structure allows for cleaner code, better maintainability, and easier scalability.
public class LoginPage {
private WebDriver driver;
private By username = By.id("username");
private By password = By.id("password");
private By submitButton = By.id("submit");
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void enterUsername(String user) {
driver.findElement(username).sendKeys(user);
}
public void enterPassword(String pass) {
driver.findElement(password).sendKeys(pass);
}
public void clickSubmit() {
driver.findElement(submitButton).click();
}
}
Why This Works: This separation of concerns allows you to keep your test scripts clean and reusable. You can easily interact with web elements through methods in your page class without cluttering your test scripts.
2. Ignoring Synchronization Issues
The Mistake
Synchronization problems are common when using Selenium. Users often forget to wait for elements to be present, leading to NoSuchElementException
or ElementNotInteractableException
.
The Right Approach
Use Selenium's built-in waiting strategies. These include implicit waits, explicit waits, and Fluent waits.
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("submit")));
element.click();
Why This Works: By waiting explicitly for a condition to become true, you reduce the chances of your tests failing due to timing issues. It leads to more stable and reliable tests.
3. Making Tests Too Fragile
The Mistake
Tests that rely heavily on the structure of the web application can break easily with minor changes to the web page layout or element attributes.
The Right Approach
Design tests that prioritize stability. Avoid using static properties like xpath
that might change with UI updates. Instead, rely on attributes like IDs or data attributes specifically designed for testing.
WebElement submitButton = driver.findElement(By.cssSelector("[data-testid='submit']"));
submitButton.click();
Why This Works: Using stable selectors reduces the chances of test failures due to page alterations. It promotes a more resilient testing process.
4. Not Following Best Practices for Element Identification
The Mistake
Many testers use overly complex locators like long XPath expressions, which can be hard to read and maintain.
The Right Approach
Prioritize simpler locators such as IDs or CSS selectors, which are usually faster and more reliable. Sometimes, simplifying your locator can significantly improve your test execution time.
// Prefer this
WebElement searchBox = driver.findElement(By.id("search"));
// Instead of this
WebElement searchBox = driver.findElement(By.xpath("//input[@type='text' and @name='search']"));
Why This Works: Simpler locators execute faster and are easier to read, reducing complexity and improving test maintainability.
5. Neglecting Browser Compatibility
The Mistake
Not testing across different browsers can lead to unverified behaviors in production. A feature might work well in Chrome but fail in Firefox or Safari.
The Right Approach
Utilize tools like Selenium Grid or cloud services like BrowserStack to run tests across multiple environments. This helps in identifying browser-specific issues early on.
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
WebDriver driver = new RemoteWebDriver(new URL("http://localhost:4444/wd/hub"), capabilities);
Why This Works: Testing across browsers ensures a consistent user experience and mitigates issues before they reach production.
6. Poor Test Design
The Mistake
Tests can become fragile and difficult to understand if they are not designed with best practices in mind. This includes hardcoding values and omitting necessary assertions.
The Right Approach
Focus on writing readable test cases that are easy to understand. Invest in using meaningful names for your tests and include assertions to verify the correctness of the application under test.
@Test
public void testUserLogin() {
LoginPage loginPage = new LoginPage(driver);
loginPage.enterUsername("user");
loginPage.enterPassword("password");
loginPage.clickSubmit();
Assert.assertTrue(driver.getCurrentUrl().contains("dashboard"));
}
Why This Works: Clear test names and assertions lead to better maintainability and quicker identification of test failures.
7. Not Keeping Tests Independent
The Mistake
Some users create tests that depend on the outcomes of previous tests, leading to situations where one failing test can cause others to fail.
The Right Approach
Design your tests to be independent of one another. Each test should set up its own state and clean up after itself if necessary.
@BeforeMethod
public void setUp() {
driver = new ChromeDriver();
driver.get("http://example.com");
}
@AfterMethod
public void tearDown() {
driver.quit();
}
Why This Works: Independent tests are more reliable. If one fails, it doesn't affect others, making troubleshooting easier.
8. Overlooking Reporting and Logging
The Mistake
Failing to implement proper logging and reporting can make it difficult to trace issues when tests fail.
The Right Approach
Incorporate logging through frameworks like Log4j or integrate reporting tools like Allure or ExtentReports, which provide rich and understandable outputs for your test results.
import org.apache.log4j.Logger;
Logger logger = Logger.getLogger(YourTest.class);
logger.info("Starting login test");
Why This Works: Detailed logs and elements enhance traceability and make it easier to pinpoint where something went wrong during the test execution.
The Last Word
Automating tests with Selenium can greatly improve the efficiency of your software development life cycle, but it also comes with its challenges. By avoiding common mistakes and embracing best practices, you can create a robust, maintainable, and efficient test suite.
For more resources on Selenium best practices, consider visiting SeleniumHQ for the official documentation, and don't hesitate to gain insights from testing blogs or forums like Ministry of Testing.
Incorporating these practices into your Selenium tests will not only improve the quality of your automated tests but also enhance your overall development workflow. Remember, effective testing is a cornerstone of quality software development! Happy testing!
Checkout our other articles