Migrating to Spring for Apache Hadoop: Common Pitfalls
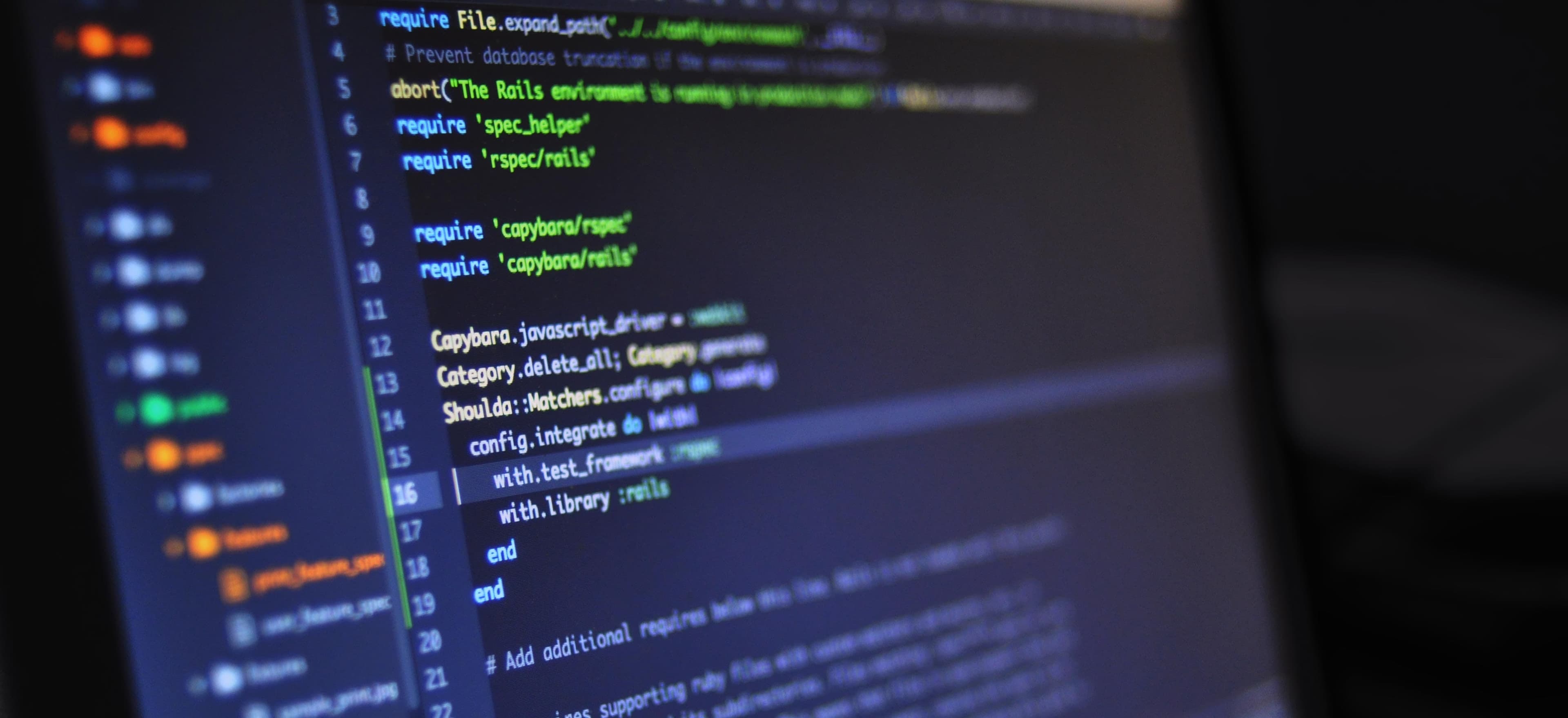
- Published on
Migrating to Spring for Apache Hadoop: Common Pitfalls
Migrating to Spring for Apache Hadoop can offer numerous benefits, including improved project organization, simplified configuration, and adherence to the principle of dependency injection (DI). However, this transition is not without its challenges. Whether you are migrating an existing application or starting fresh, it’s essential to be aware of common pitfalls to ensure a smoother process.
Why Spring for Apache Hadoop?
Before diving into the challenges, let's explore why you might consider using Spring for Apache Hadoop in the first place. Spring offers a comprehensive programming and configuration model, enabling:
- Simplified Management: Streamlined configuration through Spring’s DI container.
- Modular Components: Spring encourages modular application development, allowing for easier testing and maintenance.
- Integration Features: Out-of-the-box support to integrate various technologies, making it easier to connect with databases, messaging queues, etc.
To get started, you may want to check out the Spring for Apache Hadoop documentation.
Common Pitfalls to Avoid
1. Ignoring Compatibility
What to Watch For: One of the most common mistakes during the migration is neglecting to check the compatibility of your existing Hadoop version with Spring for Apache Hadoop.
Why It Matters: Mismatched versions can lead to unexpected behavior, bugs, and ultimately wasted time trying to troubleshoot what might be simple compatibility issues.
Tip: Always review the compatibility matrix available in the Spring for Apache Hadoop GitHub repository before you begin the migration.
2. Over-Complicating Configuration
Spring's configuration capabilities can sometimes lead developers to over-complicate settings when moving to Spring for Apache Hadoop.
What to Watch For: Using XML configurations where Java-based or annotation-driven configurations would suffice.
Why It Matters: Clean and minimal configuration enhances readability and maintainability. Excessive XML can become difficult to manage, especially in larger projects.
Example Code Snippet:
@Configuration
@EnableHadoop
public class HadoopConfig {
@Bean
public Configuration hadoopConfiguration() {
Configuration configuration = new Configuration();
configuration.set("fs.defaultFS", "hdfs://localhost:9000");
return configuration;
}
}
In this example, we use Java-based configuration instead of XML, offering better readability and ease of modification.
3. Neglecting Dependency Management
What to Watch For: Not fully appreciating the significance of managing dependencies when moving to Spring for Apache Hadoop.
Why It Matters: Missing dependencies can lead to runtime errors that are hard to diagnose.
Tip: Utilize a build automation tool like Maven or Gradle to handle dependencies effectively. The basic POM file might look like this:
<dependencies>
<dependency>
<groupId>org.springframework.hadoop</groupId>
<artifactId>spring-hadoop-core</artifactId>
<version>2.0.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-core</artifactId>
<version>2.7.3</version>
</dependency>
<!-- Add other necessary dependencies -->
</dependencies>
This approach helps manage version conflicts and keeps your build streamlined.
4. Leaving Out Testing
What to Watch For: Skipping automated testing of your Spring components can be tempting but is a significant pitfall.
Why It Matters: In complex systems, testing isn't just beneficial; it's essential. A lack of rigorous testing can lead to issues that surface only in production.
Tip: Leverage Spring’s testing support to facilitate unit and integration tests.
Example Code Snippet:
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = HadoopConfig.class)
public class HadoopIntegrationTest {
@Autowired
private Configuration hadoopConfig;
@Test
public void testHadoopConnection() {
assertNotNull(hadoopConfig);
assertNotNull(hadoopConfig.get("fs.defaultFS"));
}
}
In this example, we ensure the Hadoop configuration is loaded and functional.
5. Skipping Documentation and Best Practices
What to Watch For: Neglecting to document your migration process leaves future maintainers in the dark.
Why It Matters: Clear documentation ensures continuity, especially in larger teams or when onboarding new members.
Tip: Create a detailed migration plan, inclusive of both technical steps and the rationale behind design choices. Using platforms like Confluence or a README.md file can provide a structured format.
6. Ignoring Security Configurations
What to Watch For: Underestimating the importance of securing your Hadoop ecosystem post-migration to Spring.
Why It Matters: Hadoop systems are often exposed to sensitive data, and without robust security configurations, you leave yourself vulnerable.
Tip: Utilize Spring Security combined with Hadoop’s security features to enforce authentication and authorization.
<security:http auto-config="true" use-expressions="true">
<security:intercept-url pattern="/admin/**" access="hasRole('ROLE_ADMIN')" />
</security:http>
This snippet demonstrates basic security rules within a Spring-managed Hadoop application.
7. Failing to Use Spring Batch Properly
Spring Batch provides robust solutions for batch processing but can be underutilized or misconfigured in a Hadoop context.
What to Watch For: Not leveraging the full capabilities of Spring Batch can limit your application's efficiency.
Why It Matters: You lose out on optimized performance and scalability when you don't utilize features like job repository, partitioning, and chunk processing.
Example Code Snippet:
@EnableBatchProcessing
@Configuration
public class BatchConfig {
@Bean
public Job sampleJob(JobBuilderFactory jobBuilderFactory, StepBuilderFactory stepBuilderFactory) {
Step step1 = stepBuilderFactory.get("step1")
.tasklet((contribution, chunkContext) -> {
System.out.println("Executing step 1");
return RepeatStatus.FINISHED;
}).build();
return jobBuilderFactory.get("sampleJob")
.incrementer(new RunIdIncrementer())
.flow(step1)
.end()
.build();
}
}
In this case, the configurations allow for easy adjustments to processing logic as needed, promoting scalability and manageability.
Wrapping Up
Migrating to Spring for Apache Hadoop can be a rewarding yet challenging task. Awareness of common pitfalls—such as compatibility issues, overly complex configurations, dependency mismanagement, lack of testing, insufficient documentation, overlooked security measures, and underutilized Spring Batch capabilities—can help you mitigate risks during the migration process.
To ensure a successful transition:
- Take advantage of Spring's documentation.
- Embrace community forums to ask questions.
- Conduct thorough testing to cover edge cases.
By following best practices, you'll set a solid foundation for your Spring-managed Hadoop application. For further information, exploring the Spring for Apache Hadoop project can provide valuable insights to enhance your knowledge.
Whether you’re a novice or a seasoned developer, adapting to these methodologies will surely enhance your Hadoop applications’ robustness and maintainability. Happy coding!