Troubleshooting JSON File Writes with Moshi
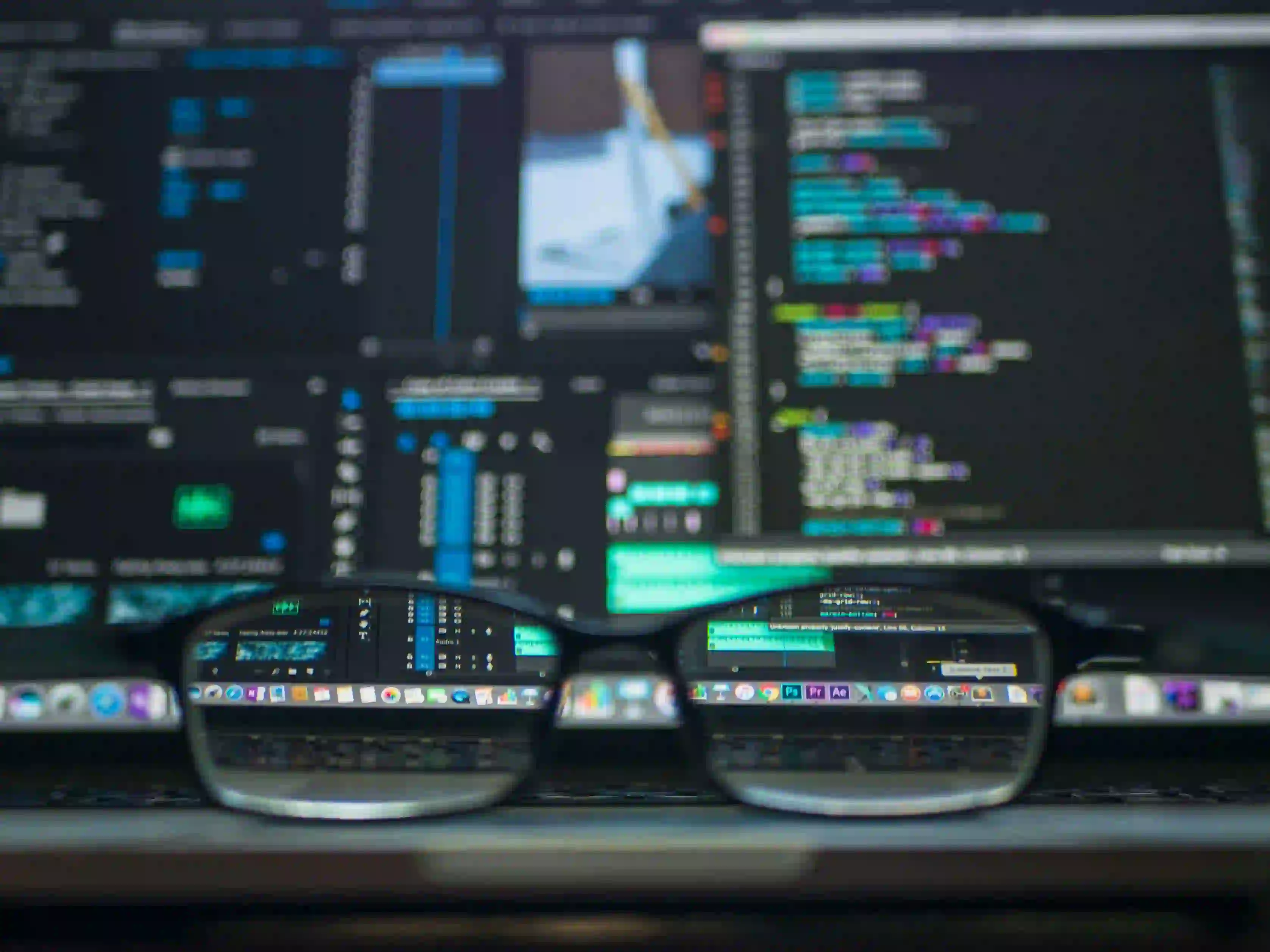
Troubleshooting JSON File Writes with Moshi in Java
In the world of Java programming, working with JSON data can often seem challenging, especially when writing and reading JSON files. This might lead to unexpected results, frustrating bugs, and a general sense of confusion. Fortunately, the Moshi library streamlines the process due to its simplicity and effectiveness. In this post, we will explore how to troubleshoot JSON file writes using Moshi, along with code snippets and practical tips.
What is Moshi?
Moshi is a modern JSON library for Android and Java, developed by Square. It enables you to parse JSON into Java objects and serialize Java objects back into JSON format. Moshi's main advantage is its support for Kotlin’s nullable types, making it a popular choice among developers.
Setting Up Moshi
Before we dive into troubleshooting strategies, let’s ensure Moshi is set up properly in your project. You can add Moshi to your Maven or Gradle build.
For Gradle
dependencies {
implementation 'com.squareup.moshi:moshi:1.12.0'
implementation 'com.squareup.moshi:moshi-kotlin:1.12.0'
}
For Maven
<dependency>
<groupId>com.squareup.moshi</groupId>
<artifactId>moshi</artifactId>
<version>1.12.0</version>
</dependency>
<dependency>
<groupId>com.squareup.moshi</groupId>
<artifactId>moshi-kotlin</artifactId>
<version>1.12.0</version>
</dependency>
With Moshi integrated, let’s explore the process of reading and writing JSON files.
Writing JSON to a File
When writing an object to a JSON file, the process typically involves creating a Moshi JsonAdapter
, which will convert your Java object into JSON format, and then writing that formatted JSON string to a file.
Example Code
import com.squareup.moshi.Moshi;
import com.squareup.moshi.JsonAdapter;
import java.io.FileWriter;
import java.io.IOException;
public class User {
String name;
int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
}
public class JsonWriter {
public void writeUserToFile(User user, String filePath) throws IOException {
Moshi moshi = new Moshi.Builder().build();
JsonAdapter<User> jsonAdapter = moshi.adapter(User.class);
String jsonString = jsonAdapter.toJson(user);
try (FileWriter fileWriter = new FileWriter(filePath)) {
fileWriter.write(jsonString);
}
}
}
Commentary on the Code
-
Moshi Instance: The
Moshi.Builder().build()
constructs an instance of Moshi. It's a best practice to create an instance and reuse it. -
JsonAdapter: The
jsonAdapter
is the key component here. It takes care of converting your object into JSON format. -
File Writing: Using
FileWriter
allows us to handle file input and output streams effectively, particularly when combined with the try-with-resources statement for safe resource management.
Common Issues When Writing JSON Files
-
File Not Found Exception: Ensure that the specified file path is correct and accessible. Path issues often lead to runtime exceptions.
-
Serialization Errors: If the object has transient fields or circular references, Moshi may throw a
JsonDataException
. Always validate the input object before serialization. -
Malformed JSON: Ensure that your Java class structure corresponds closely to the intended JSON output. If you're unsure, you can log the JSON string before writing it.
Logging JSON String
System.out.println(jsonString); // Useful for debugging
Reading JSON from a File
Once you have written JSON data to a file, reading it back into your object is the next essential step. Here is how to do it:
Example Code
import com.squareup.moshi.Moshi;
import com.squareup.moshi.JsonAdapter;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class JsonReader {
public User readUserFromFile(String filePath) throws IOException {
Moshi moshi = new Moshi.Builder().build();
JsonAdapter<User> jsonAdapter = moshi.adapter(User.class);
try (BufferedReader bufferedReader = new BufferedReader(new FileReader(filePath))) {
return jsonAdapter.fromJson(bufferedReader);
}
}
}
Commentary on the Code
-
BufferedReader: This is ideal for reading text from a character-input stream efficiently.
-
fromJson: The
fromJson
method deserializes the JSON string into a User object. If the file is empty or malformed, it may returnnull
.
Troubleshooting File Reading
-
Incorrect JSON Structure: The file contents must match the expected structure defined in your class.
-
Null Object: If you receive a null object, it indicates that Moshi couldn’t parse the JSON. Check for typographical errors in the JSON or Java class.
-
File Not Found: Again, ensure the file path is correct. This is the most common cause of failure in file reading.
Error Handling
Always ensure proper exception handling. Wrapping your reading and writing implementation in try-catch blocks will help catch and log exceptions such as IOException
or JsonDataException
.
Example of Proper Exception Handling
public void safeWrite(User user, String filePath) {
try {
writeUserToFile(user, filePath);
} catch (IOException e) {
e.printStackTrace(); // Log your errors or rethrow
}
}
Lessons Learned
Moshi simplifies working with JSON in Java, allowing developers to efficiently serialize with minimal friction. Nonetheless, issues can arise during the writing and reading processes. By following the above guidelines, and employing logging and robust error handling practices, you can diagnose and resolve any JSON file write issues with ease.
Feel free to explore more on Moshi's GitHub page for in-depth documentation and examples. Happy coding!