Troubleshooting Common Smack XMPP Connection Issues
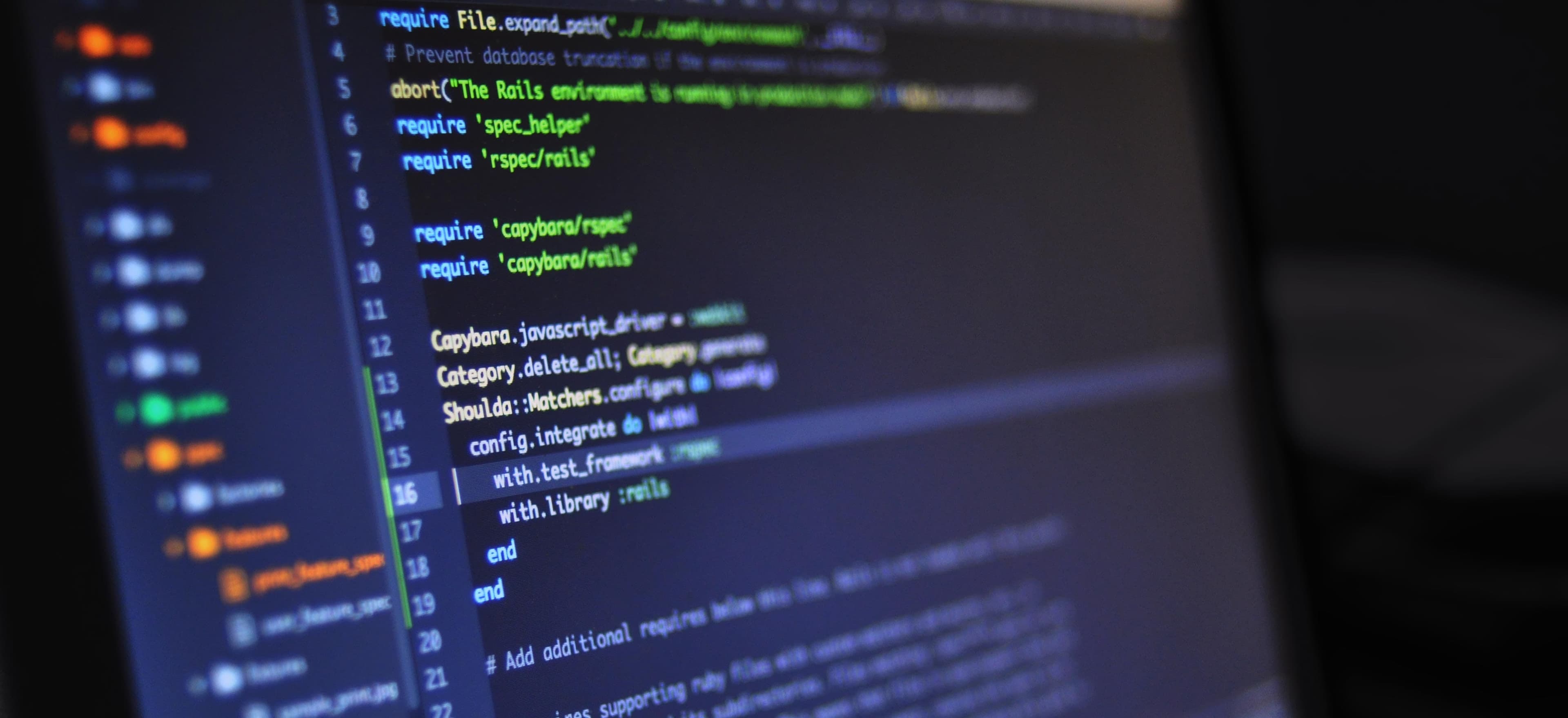
- Published on
Troubleshooting Common Smack XMPP Connection Issues
The eXtensible Messaging and Presence Protocol (XMPP) is a widely-used communication protocol for real-time messaging. If you are developing applications with XMPP, you might have heard of Smack, one of the most popular libraries for working with this protocol in Java. While Smack simplifies the process of connecting to XMPP servers, developers often encounter connection issues that can hinder their applications' functionality. In this blog post, we will explore common Smack XMPP connection problems and how to troubleshoot them effectively.
Understanding Smack and XMPP
Smack is an open-source XMPP client library written in Java. It provides an easy-to-use API for connecting to and interacting with XMPP servers. You can read more about Smack's architecture and features on the official Smack Documentation.
Before diving into troubleshooting strategies, it’s essential to understand the foundational concepts of XMPP, including XMPP servers, clients, and the client-server communication process.
Common Connection Issues
While developing XMPP applications with Smack, there are several common connection issues you may encounter:
- Invalid Credentials
- Network Issues
- Server Configuration Errors
- Timeout Errors
- TLS/SSL Issues
Let's delve into each of these issues individually.
1. Invalid Credentials
One of the most common reasons for connection failure is invalid credentials. An attempt to authenticate with incorrect username or password will lead to an authentication error.
XMPPTCPConnectionConfiguration config = XMPPTCPConnectionConfiguration.builder()
.setXmppDomain("example.com")
.setHost("chat.example.com")
.setPort(5222)
.setDebuggerEnabled(true)
.setSecurityMode(SecurityMode.disabled)
.build();
AbstractXMPPConnection connection = new XMPPTCPConnection(config);
try {
connection.connect();
connection.login("username", "wrongpassword"); // This will generate an error
} catch (SmackException | IOException | InterruptedException e) {
System.out.println("Authentication failed: " + e.getMessage());
}
Why it Matters: Always ensure that your credentials are correct. If there are special characters in your password, ensure they are properly encoded.
2. Network Issues
Network-related issues can also occur when trying to connect to the XMPP server. Firewalls, VPNs, or even unstable networks can disrupt connectivity.
Network Check
Test connectivity to the server using tools such as ping
or telnet
.
ping chat.example.com
telnet chat.example.com 5222
If you do not get a response, there is a network issue preventing the connection.
Code Snippet: Handling Network Issues
try {
connection.connect();
} catch (SmackException.NoResponseException e) {
System.out.println("No response from server: " + e.getMessage());
} catch (IOException e) {
System.out.println("IO Exception occurred: " + e.getMessage());
} catch (InterruptedException e) {
System.out.println("Connection thread interrupted: " + e.getMessage());
}
Why it Matters: Document any network problems, as they may require action such as whitelisting the connection or updating firewall settings.
3. Server Configuration Errors
Improper configuration of the XMPP server could also lead to connection issues. For example, if the server is not set to accept non-encrypted connections, any attempts to connect without TLS will fail.
Configuring Connection Settings
XMPPTCPConnectionConfiguration config = XMPPTCPConnectionConfiguration.builder()
.setXmppDomain("example.com")
.setResource("smack")
.setHost("chat.example.com")
.setPort(5222)
.setSecurityMode(SecurityMode.required) // Adjust this according to server settings
.build();
Why it Matters: Understand the server’s configuration to set up your client correctly. Always check the server documentation for the required settings.
4. Timeout Errors
Connection attempts may time out if the server takes too long to respond. This can occur due to a high server load or a slow network connection.
Code Snippet: Setting Timeout
XMPPTCPConnectionConfiguration config = XMPPTCPConnectionConfiguration.builder()
.setXmppDomain("example.com")
.setHost("chat.example.com")
.setPort(5222)
.setConnectionListener(new XMPPTCPConnection.ConnectionListener() {
@Override
public void connected(AbstractXMPPConnection connection) {
System.out.println("Connected successfully.");
}
@Override
public void connectionClosed() {
System.out.println("Connection closed.");
}
})
.setSocketFactory(new CustomSocketFactory(5000)) // 5 seconds timeout
.build();
Why it Matters: Be aware of your network and server conditions to adjust timeout settings accordingly, ensuring a smoother connection.
5. TLS/SSL Issues
XMPP commonly uses TLS/SSL encryption to secure connections. If the server mandates SSL and the client attempts to connect without it, the connection will fail.
XMPPTCPConnectionConfiguration config = XMPPTCPConnectionConfiguration.builder()
.setXmppDomain("example.com")
.setHost("chat.example.com")
.setPort(5222)
.setSecurityMode(SecurityMode.required) // Must be required for SSL/TLS
.build();
Why it Matters: Failing to use the correct security settings can expose security risks or result in connection errors. Always implement the recommended security measures specified by the server.
Additional Resources
For developers interested in further enhancing their understanding of XMPP protocols, consider exploring the official XMPP Standards Foundation website. This site contains many resources regarding XMPP Server configurations, server-client interactions, and best practices.
In Conclusion, Here is What Matters
Troubleshooting connection issues in Smack involves careful attention to detail and understanding how both the client and server work together. By following the steps outlined above and leveraging proper debugging techniques, you can resolve most connection issues effectively.
If your issue persists despite following these guidelines, consider reaching out to community forums or resources, such as Stack Overflow or the Smack community. Engaging with experienced developers can often expedite finding solutions to challenging problems.
Through diligence and a methodical approach, you can build robust XMPP applications that leverage the Smack library effectively. Embrace the challenges, and happy coding!
Checkout our other articles