Common Mistakes with JAX-RS Annotations and How to Fix Them
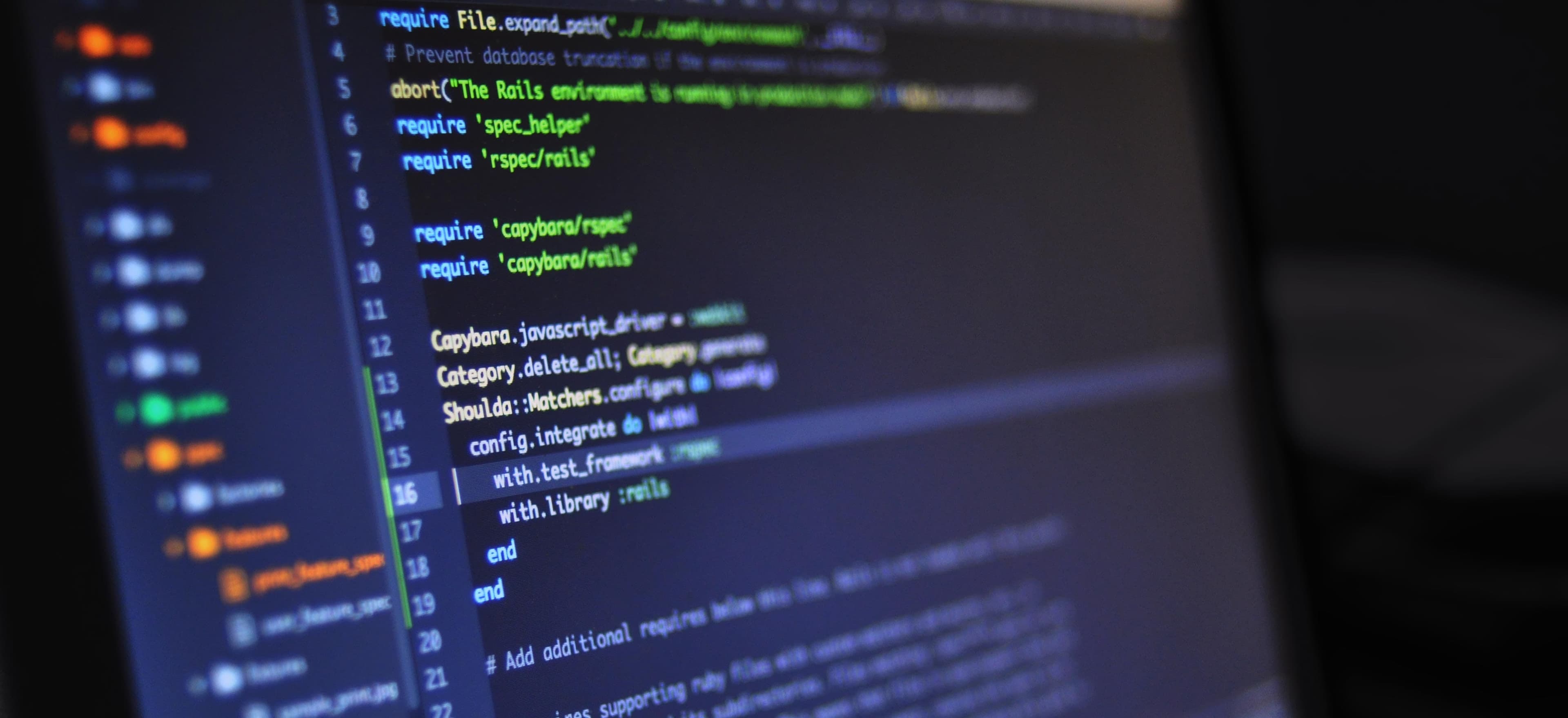
- Published on
Common Mistakes with JAX-RS Annotations and How to Fix Them
Java API for RESTful Web Services (JAX-RS) has become a cornerstone for building RESTful services in Java. While it simplifies the creation of web services, it is not without its pitfalls. Many developers encounter common mistakes when using JAX-RS annotations. In this post, we'll discuss these mistakes, explain why they occur, and how to fix them.
What is JAX-RS?
JAX-RS is part of the Java EE specifications aiming to simplify the process of developing RESTful web services in Java. It provides annotations to customize the HTTP operations easily and allows developers to focus more on the logic rather than the boilerplate code typically associated with web services.
Common Mistakes with JAX-RS Annotations
1. Not Understanding Annotations
Mistake: Many developers jump into writing services without fully understanding the various JAX-RS annotations. This often leads to accidental misconfigurations.
Fix: Take the time to understand the purpose of each annotation. Here are some of the most commonly used ones:
@Path
: Defines the URI where the resource can be accessed.@GET
,@POST
,@PUT
,@DELETE
: Specify the HTTP method that the method handles.@Produces
and@Consumes
: Define the media types produced or consumed by the resource.
Example:
@Path("/users")
public class UserService {
@GET
@Path("/{id}")
@Produces(MediaType.APPLICATION_JSON)
public Response getUser(@PathParam("id") String id) {
// Logic to fetch user by id
return Response.ok(user).build();
}
}
In this example, the getUser
method is fully annotated to specify its path, HTTP method (GET), and response media type.
2. Incorrect Use of @PathParam
and @QueryParam
Mistake: Not using @PathParam
and @QueryParam
correctly can lead to confusion and errors in retrieving parameters from the request.
Fix: Use @PathParam
for parameters embedded in the URL path and @QueryParam
for query string parameters.
Example:
@GET
@Path("/users/{userId}")
public Response getUserById(@PathParam("userId") String userId) {
// Process the userId obtained from the path
return Response.ok(userService.fetchUserById(userId)).build();
}
Here, @PathParam
is used correctly for a path variable.
@GET
@Path("/users")
public Response getUsersByStatus(@QueryParam("status") String status) {
// Process the status query parameter
return Response.ok(userService.findUsersByStatus(status)).build();
}
In this case, @QueryParam
is used for a query string parameter.
3. Misconfigured @Produces
and @Consumes
Mistake: Failing to configure @Produces
and @Consumes
can lead to issues when sending or receiving JSON or XML data.
Fix: Always ensure these annotations are specified correctly based on the content type your service expects and produces.
Example:
@POST
@Path("/users")
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public Response createUser(User user) {
// Logic to create a new user
return Response.status(Response.Status.CREATED).entity(createdUser).build();
}
This code snippet ensures that the service consumes and produces JSON format.
4. Ignoring Error Handling
Mistake: Not implementing proper error handling can lead to vague errors being sent back to the client, making it hard to debug.
Fix: Use exception mappers to handle errors gracefully.
Example:
@Provider
public class CustomExceptionMapper implements ExceptionMapper<Throwable> {
@Override
public Response toResponse(Throwable exception) {
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity("Server error: " + exception.getMessage())
.type(MediaType.TEXT_PLAIN)
.build();
}
}
This example shows how to create a custom exception mapper that sends back structured error messages.
5. Overusing Singleton Pattern for Resources
Mistake: Many developers misuse singleton patterns with JAX-RS resources, leading to issues with state and concurrency.
Fix: JAX-RS resources should be stateless and act like services rather than single instances. This ensures thread safety and reliability in a concurrent environment.
Example:
@Path("/orders")
public class OrderService {
@GET
@Path("/{id}")
public Response getOrder(@PathParam("id") String id) {
Order order = orderService.findOrderById(id); // Stateless service
return Response.ok(order).build();
}
}
Here, OrderService
acts statelessly, preventing any concurrency issues.
6. Lack of API Documentation
Mistake: One crucial aspect often neglected is documenting the API using methods like OpenAPI Specification (OAS).
Fix: Utilize libraries like Swagger or SpringFox to generate documentation for your API automatically.
Example Using Swagger:
import io.swagger.v3.oas.annotations.Operation;
@Path("/products")
public class ProductService {
@GET
@Path("/{id}")
@Operation(summary = "Get Product by ID")
public Response getProductById(@PathParam("id") Long id) {
// Logic to get product by ID
return Response.ok(product).build();
}
}
This code enables users of your API to understand its capabilities without needing to read your code.
7. Hardcoding URIs
Mistake: Hardcoding URIs in the application can lead to challenges when modifying paths or deploying in different environments.
Fix: Use configuration files or environment variables to manage URIs dynamically.
To Wrap Things Up
Understanding and effectively using JAX-RS annotations is critical for building robust and efficient RESTful services. By avoiding these common mistakes, you can enhance the reliability, maintainability, and usability of your APIs.
For further reading on JAX-RS and best practices, consider visiting:
- Official JAX-RS Documentation
- RestEasy Documentation
Remember that while learning from mistakes helps, proactively applying best practices is key to mastering JAX-RS and creating high-quality web services.
Let’s Connect!
What mistakes have you encountered with JAX-RS? What solutions did you find effective? Share your thoughts in the comments below!