Are Nulls in Ceylon Really Handled Better Than Java?
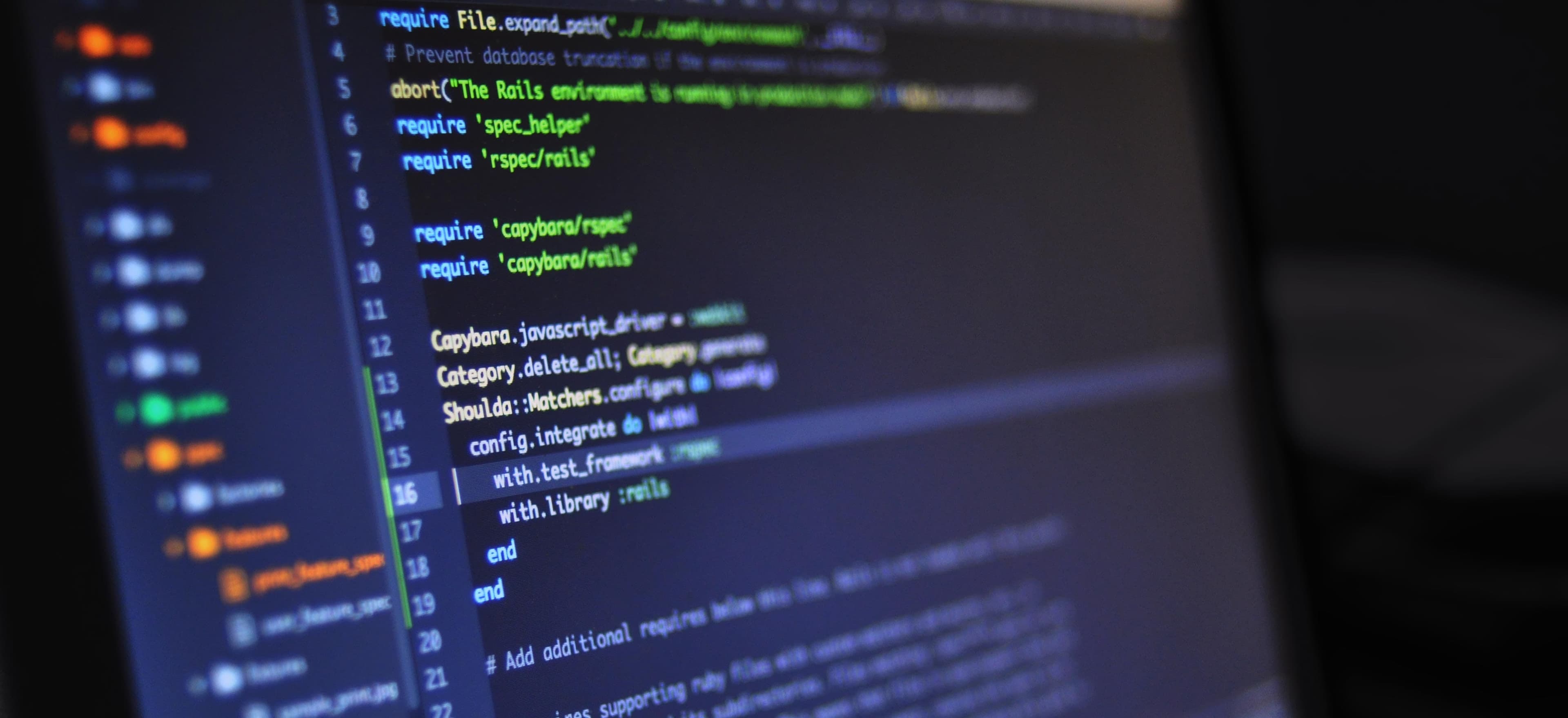
- Published on
Are Nulls in Ceylon Really Handled Better Than Java?
Null references have been the bane of many developers’ lives, causing countless bugs and runtime exceptions in codebases worldwide. In this blog post, we'll compare how Ceylon and Java handle nulls. Our aim is to clarify whether Ceylon offers a more robust solution for null reference management compared to Java.
Getting Started to Null References
A null reference is essentially a pointer that doesn't point to any object. Java has long used null references, leading to famously dreaded NullPointerExceptions
(NPEs) during runtime. To mitigate this, developers often introduce checks or use "Optional" types, but these are not foolproof methods.
Java and Nulls
Java has several strategies for managing nulls, though it is far from perfect. The introduction of the Optional
class in Java 8 was a significant step toward safer null handling. Here is how it works:
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> optionalValue = Optional.ofNullable(getData());
// Using ifPresent to avoid null checks
optionalValue.ifPresent(value -> System.out.println("Value is: " + value));
// fallback using orElse
String result = optionalValue.orElse("Default Value");
System.out.println("Result: " + result);
}
private static String getData() {
return null; // Simulating a data retrieval that could return null
}
}
Commentary on Java’s Approach
In this code snippet, we encapsulate the result of getData()
in an Optional
. By using ifPresent
, we eliminate the need for null checks. The orElse
method allows for a default return value if the Optional
is empty. However, this does require recognizing when to use Optional
, and it's not automatically a default behavior for all reference types.
The Shortcomings of Java Null Handling
- Implicit Nulls: Java doesn’t prevent the assignment of null values to types unless they are encapsulated in an
Optional
. - Retrofit: You need to refactor code for existing null checks throughout.
- Performance Overheads: Using
Optional
can introduce additional overhead due to its object creation and boxing/unboxing.
To explore more on Java's handling of nulls, you can check Java's Optional Documentation.
Ceylon: A Different Approach
Ceylon was designed with a more modern and type-safe feature set, including the treatment of nulls. One of its notable features is the null
types system which succinctly separates non-nullable types from nullable types.
Ceylon's Handling of Nulls
In Ceylon, you explicitly declare whether a variable can hold a null reference or not. This is in sharp contrast to Java's more permissive allowance of nulls. Here is how this is implemented:
shared void main() {
// Explicitly declaring a nullable String
String? nullableString = null;
// Non-nullable String
String nonNullableString = "Ceylon";
if (nullableString != null) {
print(nullableString);
}
// This would throw a compile-time error
// nonNullableString = null; // Uncommenting this line will result in a compile-time error
}
Commentary on Ceylon’s Approach
Here, using the type declaration String?
denotes that nullableString
can hold null values, while nonNullableString
cannot. This compile-time enforcement means that potential null reference issues are caught during development rather than at runtime.
Advantages of Ceylon's Null Handling
- Compile-Time Safety: Developers are explicitly informed of whether a variable can be null.
- Cleaner Code: Since you have to indicate nullability, the code becomes cleaner without various null checks.
- Improved Readability: It's immediately clear which variables could potentially be null.
For more on Ceylon's type system, you can refer to the Ceylon Language Documentation.
Comparison: Ceylon vs. Java
| Feature | Java | Ceylon |
|---------------------|---------------------------|---------------------------|
| Null Representation | null
| Nullable types (Type?
) |
| Safety | Runtime exceptions (NPEs) | Compile-time checking |
| Performance | Overhead with Optionals | Type checks at compile time|
| Syntax Simplicity | Optional required | Inline nullability declared |
As we can see from the comparison, Ceylon's handling of nulls stands out as a more proactive and type-safe strategy.
Final Considerations
Java's handling of nulls, while flexible, often leads to ambiguous code that can lead to bugs. Ceylon's robust type system addresses this by enforcing nullability at compile-time, substantially improving code quality and reducing runtime exceptions.
While Ceylon is not as widely adopted as Java, it introduces best practices worth considering for any serious software developer, especially in new projects.
In summary, if you are looking for a language that inherently avoids the pitfalls tied to null references, Ceylon appears to be a better choice. Nonetheless, understanding Java's shortcomings allows developers to adopt safer practices even within its ecosystem.
Final Thoughts
The debate between these two languages is not merely technical; it's about how developers think about and manage null references in their applications. As you define your next project or refactor existing code, it’s worth considering how best to handle nulls, be it through existing strategies in Java or exploring new languages like Ceylon.