Overcoming Latency Issues in Serverless Data Pipelines
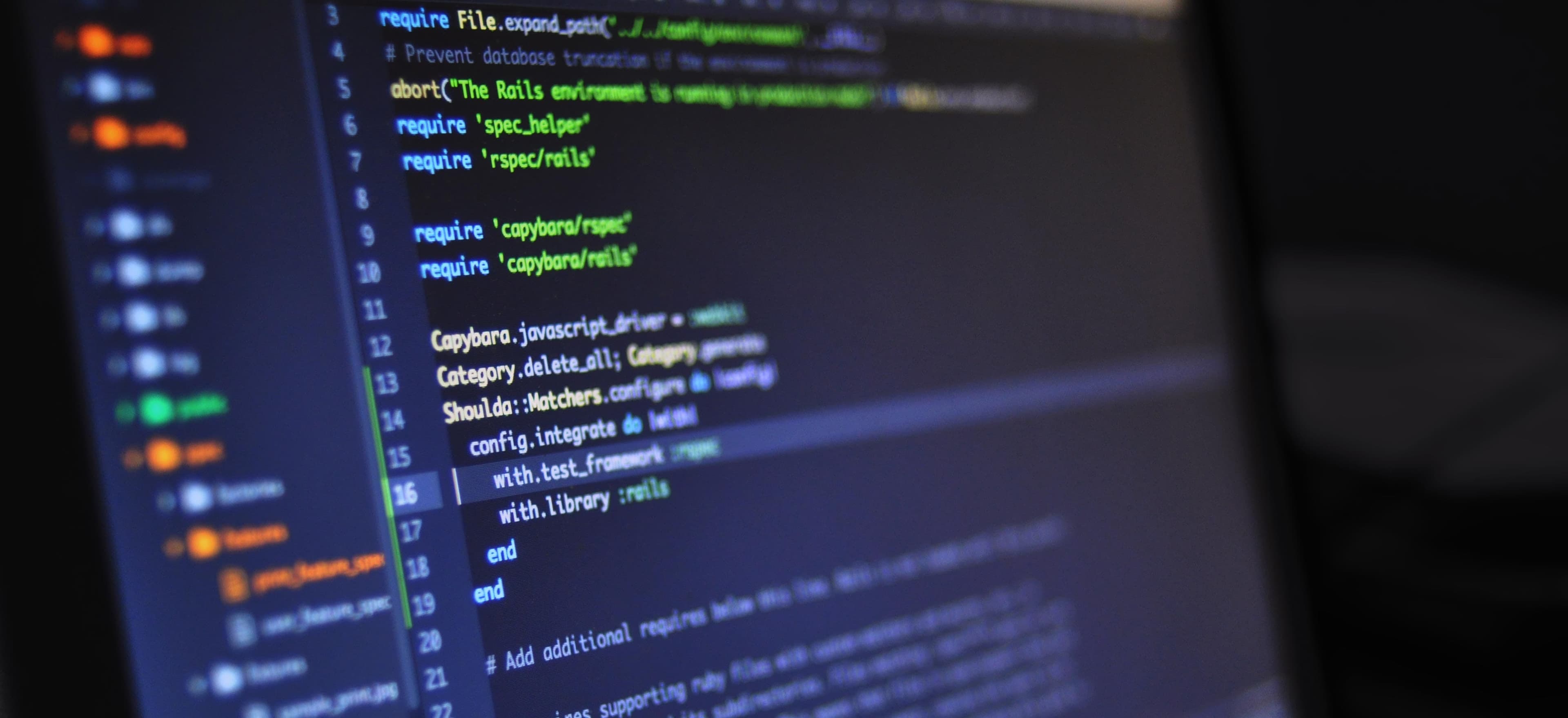
- Published on
Overcoming Latency Issues in Serverless Data Pipelines
In the rapidly evolving landscape of technology, serverless computing has emerged as a game changer for building efficient data pipelines. However, managing latency in these setups can be a daunting task, especially when dealing with vast amounts of data generated in real-time. In this post, we'll explore the nuances of latency in serverless data pipelines and present strategies to alleviate these issues effectively.
What is a Serverless Data Pipeline?
A serverless data pipeline is an architecture where cloud services handle the management of servers. Developers build and deploy applications without worrying about infrastructure management. Services like AWS Lambda, Google Cloud Functions, and Azure Functions are prime examples.
These serverless architectures enable agility and scalability, essential for processing large streams of data. However, they introduce challenges, particularly concerning latency.
Understanding Latency
Latency refers to the time it takes for a message or data packet to travel from one point to another in a system. In serverless data pipelines, latency can result from several factors, including:
- Cold Starts: The delay incurred when a function is invoked after being idle for some time.
- Data Transfer Delays: Time taken to move data between services.
- Processing Time: The time taken by the function to execute its logic.
Understanding these factors is crucial for optimizing latency in your serverless data pipelines.
Strategies to Overcome Latency
1. Optimize Cold Start Latency
Cold starts occur when a serverless function is invoked after a period of inactivity. To mitigate the impact of cold start delays, consider the following:
Pre-Warm Functions
One approach is to keep functions warm by invoking them at regular intervals. Here is an exemplary snippet using AWS SDK to invoke a Lambda function.
import com.amazonaws.services.lambda.AWSLambda;
import com.amazonaws.services.lambda.AWSLambdaClientBuilder;
import com.amazonaws.services.lambda.model.InvokeRequest;
public class PreWarmLambda {
public static void main(String[] args) {
AWSLambda lambdaClient = AWSLambdaClientBuilder.defaultClient();
String functionName = "YourLambdaFunction";
InvokeRequest invokeRequest = new InvokeRequest()
.withFunctionName(functionName)
.withPayload("{\"key\":\"value\"}");
lambdaClient.invoke(invokeRequest);
}
}
Why? This code snippet ensures that the Lambda function gets invoked periodically. By keeping the function warm, you minimize cold start latency, leading to improved performance.
2. Employ Asynchronous Processing
By decoupling services and processing requests asynchronously, latency can be significantly reduced. This can be achieved using message queues like AWS SQS or managed services like AWS EventBridge.
Example of Asynchronous Invocation with AWS SDK
import com.amazonaws.services.sqs.AmazonSQS;
import com.amazonaws.services.sqs.AmazonSQSClientBuilder;
import com.amazonaws.services.sqs.model.SendMessageRequest;
public class AsyncMessageSender {
private static final String QUEUE_URL = "your-queue-url";
public static void main(String[] args) {
AmazonSQS sqs = AmazonSQSClientBuilder.defaultClient();
SendMessageRequest sendMsgRequest = new SendMessageRequest()
.withQueueUrl(QUEUE_URL)
.withMessageBody("Hello, your async message!")
.withDelaySeconds(5);
sqs.sendMessage(sendMsgRequest);
}
}
Why? The above code demonstrates how to send messages to SQS. By processing data asynchronously, the system can handle requests more efficiently and avoid unnecessary waits, reducing overall latency.
3. Use Faster Data Transfer Protocols
Choosing the right data transfer protocol can significantly impact latency. For example, leveraging gRPC can lead to faster serialization and deserialization compared to traditional REST APIs.
Example of a gRPC Service Definition
syntax = "proto3";
service DataPipeline {
rpc StreamData(StreamRequest) returns (StreamResponse);
}
message StreamRequest {
string data = 1;
}
message StreamResponse {
bool acknowledged = 1;
}
Why? Using gRPC allows for more efficient data transfer between services. This efficiency leads to lower latency and improved performance in data processing scenarios.
4. Implement Data Caching
Caching values that don’t change often or are expensive to compute can dramatically reduce data retrieval times. AWS provides services like ElastiCache for Redis, which can be integrated within serverless applications.
Example of Using AWS ElastiCache
import redis.clients.jedis.Jedis;
public class CacheUtil {
private static final String CACHE_HOST = "your-cache-host";
public static void main(String[] args) {
try (Jedis jedis = new Jedis(CACHE_HOST)) {
jedis.set("key", "value");
String value = jedis.get("key");
System.out.println("Cached Value: " + value);
}
}
}
Why? This code snippet demonstrates connecting to and using a Redis cache. By storing frequently accessed data, the need to repeatedly invoke server-side processing can be minimized, significantly enhancing throughput and reducing latency.
5. Optimize Function Code
Streamlining your functions can have a considerable impact on execution speed. Here are general optimization techniques:
- Eliminate unnecessary dependencies.
- Use efficient algorithms and data structures.
- Start processing as soon as sufficient data is available (e.g., batch processing).
Example of Optimizing a Lambda Function
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class ExampleLambda implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
// Efficient processing logic
return "Processed: " + input;
}
}
Why? By focusing on efficient logic within your function, you reduce processing time, which directly decreases latency. Tuning function memory settings can also lead to improved performance.
Lessons Learned
Addressing latency in serverless data pipelines is not a one-size-fits-all solution but rather requires a combination of strategies. From keeping functions warm to leveraging asynchronous processing and employing caching, optimizing these aspects can lead to a significant decrease in latency.
As you build and refine your serverless data pipelines, continually assess your performance metrics. Keep learning and adjusting your architecture based on the requirements and challenges you face.
For further reading on related topics, be sure to check out:
Implementing these strategies will pave the way for more efficient and responsive data pipelines, harnessing the full power of serverless computing. Happy coding!