Debugging Common Issues in Parameterized Integration Tests
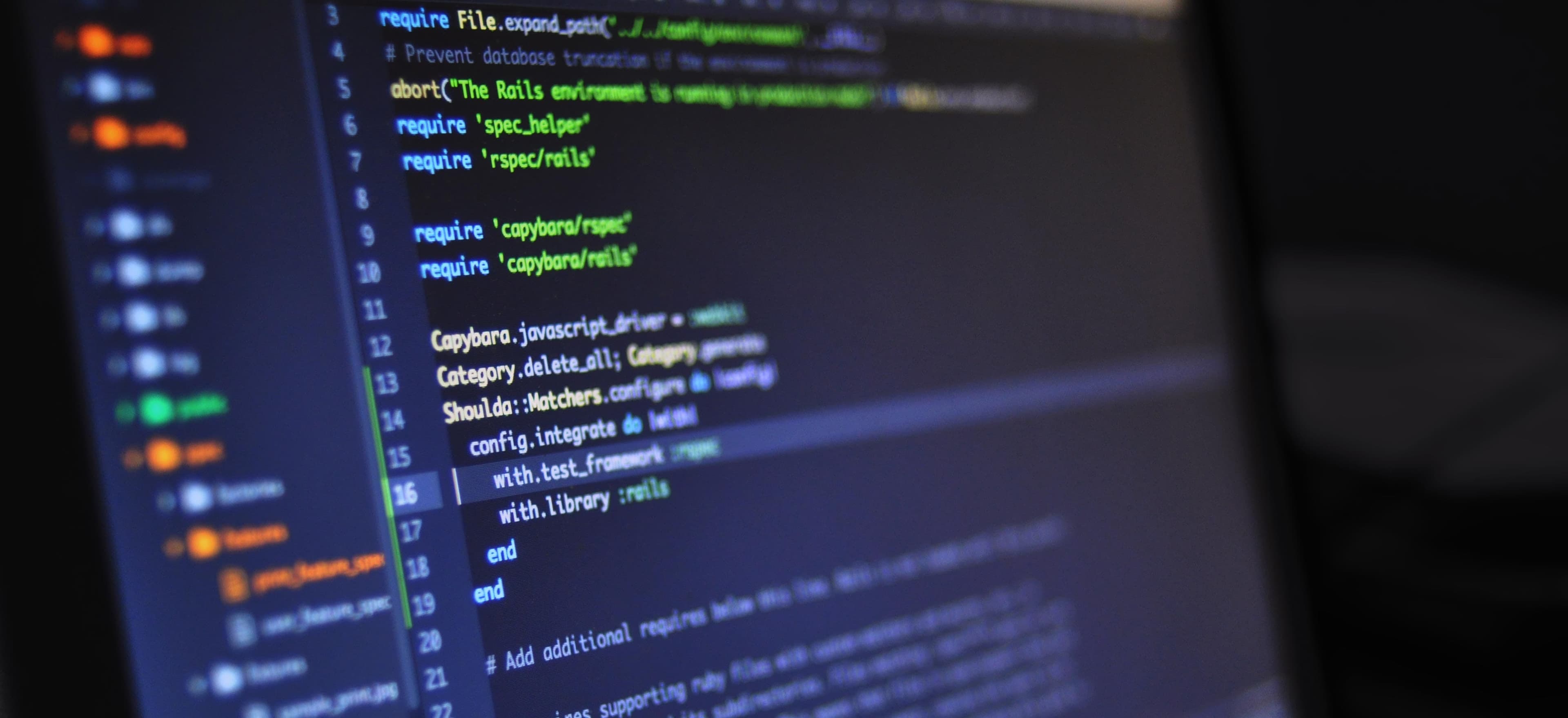
- Published on
Debugging Common Issues in Parameterized Integration Tests
In the realm of software development, integration tests play a crucial role in ensuring the components of a system work seamlessly together. When we add parameters to our integration tests, they become even more robust and flexible. However, debugging issues in parameterized integration tests can be tricky and daunting. In this post, we will explore common issues faced when dealing with parameterized integration tests, how to identify them, and effective ways to debug.
What Are Parameterized Integration Tests?
Parameterized integration tests allow developers to run the same test logic with different sets of input parameters. This capability makes it easy to validate the behavior of the application under various scenarios with minimal changes to the test code.
Here's a simple example in JUnit, a popular testing framework for Java:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
import org.junit.runners.Parameterized;
import org.junit.runners.Parameterized.Parameters;
import java.util.Arrays;
import java.util.Collection;
@Parameterized.UseParametersRunner
public class CalculatorTest {
private int input1;
private int input2;
private int expectedResult;
public CalculatorTest(int input1, int input2, int expectedResult) {
this.input1 = input1;
this.input2 = input2;
this.expectedResult = expectedResult;
}
@Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{1, 1, 2},
{2, 3, 5},
{3, 5, 8},
{6, 6, 12}
});
}
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(expectedResult, calculator.add(input1, input2));
}
}
Why Parameterized Tests Matter
Using parameterized tests can greatly enhance test coverage by varying inputs without duplicating test logic. They are not only cleaner but also promote better maintainability. As a result, these tests can help pinpoint issues in a codebase more effectively.
Common Issues in Parameterized Integration Tests
1. Incorrect Test Data
One of the most common pitfalls in parameterized tests is incorrect or inconsistent test data. If the input parameters are not set properly, the tests may not accurately reflect the scenarios you wish to evaluate.
Debugging Tip: Always log the input values as a part of your test execution. This can help you understand which set of parameters failed under what conditions.
Here’s how you might add logging:
@Test
public void testAdd() {
System.out.println("Testing addition with inputs: " + input1 + " and " + input2);
assertEquals(expectedResult, calculator.add(input1, input2));
}
2. Database State Management
For integration tests that interact with a database, managing the state is critical. If your test data is left behind from previous tests or incorrectly set up, you might encounter unexpected results.
Debugging Tip: Use a dedicated testing database that is reset before each test execution. Alternatively, consider using in-memory databases like H2 during test execution to manage the state better.
3. Environment Configuration
Integration tests often depend on various environmental setups—try running tests on different platforms or configurations. Differences in these setups can lead to null pointer exceptions, or unexpected behavior.
Debugging Tip: Ensure that the test environment matches the production environment as closely as possible. Document the environment setup in the README of your project.
4. Dependency Version Conflicts
In many cases, failing parameterized tests can be traced back to incompatible library versions. If libraries have been updated or changed over time, these might impact the way integration tests behave.
Debugging Tip: Regularly review the project dependencies. Using tools like Maven or Gradle can help you manage these versions consistently.
5. Code Logic Errors
Sometimes, the issue lies not with the test but with the production code itself. A bug in your implementation could lead to test failures that may falsely indicate an error in the parameterized tests.
Debugging Tip: Start by breaking down the function being tested. Temporarily replace the method call with simple expected values to ensure the test suite behaves as expected.
Example of a Logic Error in Code
public int add(int a, int b) {
// Potential error: returning wrong sum due to logical mistake
return a + b + 1; // This should be 'a + b'
}
When you run a parameterized test against this method, you will see incorrect results:
// Example output might show: Input: (2,3) Expected: (5) Actual: (6)
Best Practices for Debugging Parameterized Integration Tests
Use Descriptive Test Names
When your test cases are parameterized, descriptive names offer clarity. Consider giving context to your parameter set directly in the method.
@Parameters(name = "{index}: add({0}+{1})= {2}")
Leverage Assertions
Incorporate detailed assertions that tell you not only if the test passed but also why it passed or failed. Frameworks like AssertJ provide fluency that can enhance your assertions.
import static org.assertj.core.api.Assertions.assertThat;
assertThat(calculator.add(input1, input2)).isEqualTo(expectedResult);
Parallel Test Execution
Take advantage of testing frameworks that can support parallel execution of tests. While this can speed up the process, be careful of shared mutable resources leading to unpredictable test failures.
The Last Word
Though parameterized integration tests can introduce complexities, they significantly augment the robustness of your tests. By understanding common pitfalls and applying debugging approaches, you can ensure a smoother testing process. Remember, take the time to analyze the failed tests, experiment with different parameters, and verify your foundational code.
For further reading, consider exploring the following resources:
- JUnit 5 User Guide
- Effective Unit Testing: A Guide for Java Developers
By addressing these common issues, you can stay ahead in maintaining the health of your application and enhancing your integration testing strategies. Happy coding!
Checkout our other articles