Debunking the Myth: How Many Objects Are Actually Created?
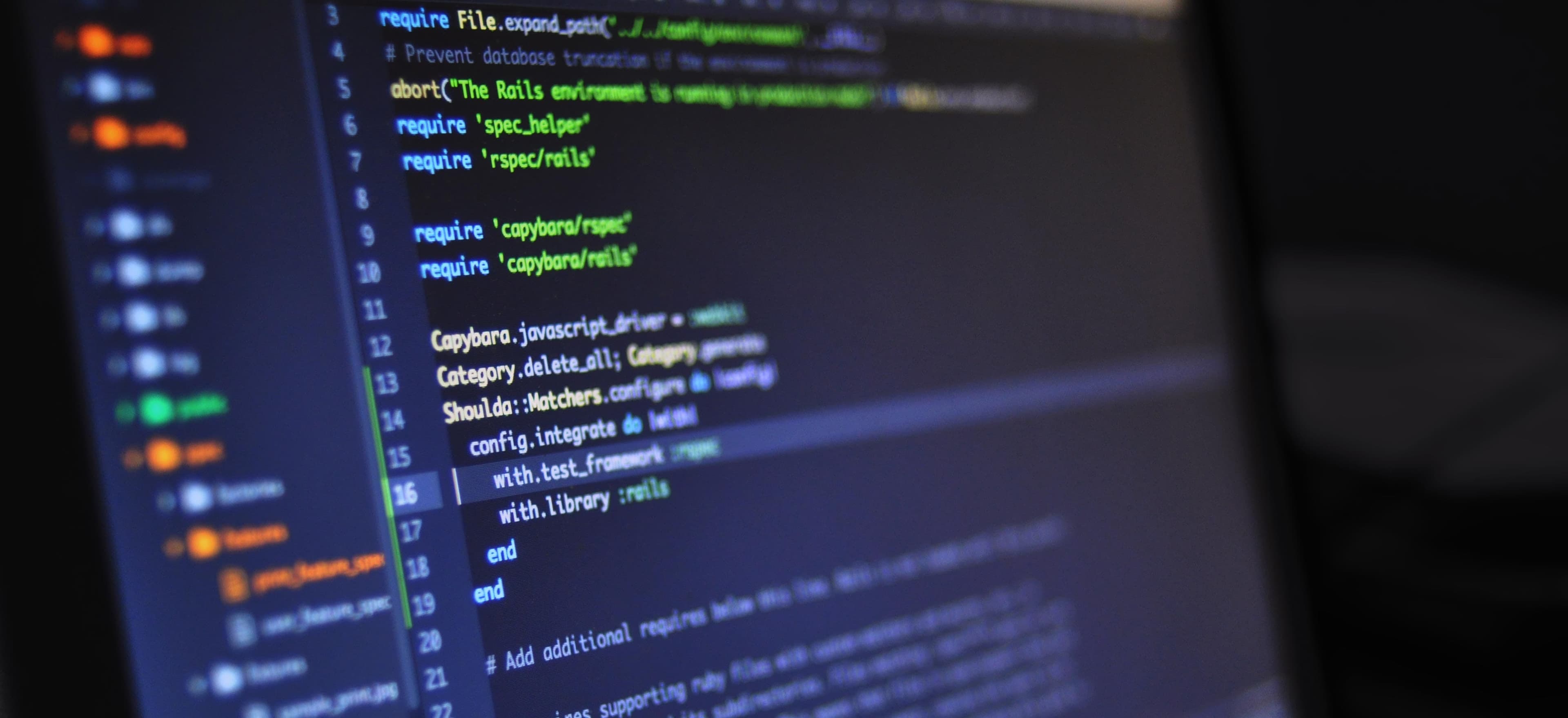
- Published on
Debunking the Myth: How Many Objects Are Actually Created in Java?
Java, a versatile and powerful programming language, is often lauded for its simplicity and robustness. However, there is a persistent myth within the Java community regarding the actual number of objects created during the execution of a program. This myth can lead to misunderstanding software performance and resource management. In this blog post, we will dissect this myth, explore how Java manages objects, and distill practical knowledge for optimizing Java applications.
Understanding Java Object Creation
In Java, everything revolves around the concept of objects. An object is essentially an instance of a class, and classes are blueprints for creating these instances. The act of creating an object in Java involves memory allocation and a constructor call.
To clarify this, consider the following simple example:
class Dog {
String name;
Dog(String name) {
this.name = name;
}
}
public class Main {
public static void main(String[] args) {
Dog dog1 = new Dog("Buddy");
Dog dog2 = new Dog("Max");
}
}
In this snippet, two objects of type Dog
are created, each with a unique name. This leads us to an important question: How many objects does the Java Virtual Machine (JVM) actually create?
The Real Count: Beyond Instantiated Objects
At first glance, one might assume that only the objects explicitly instantiated are counted. However, Java includes various complexities that inflate this number significantly.
Autoboxing
One practical example is autoboxing. When using wrapper classes like Integer
, the JVM automatically converts primitive data types into their corresponding wrapper objects. For instance:
public class Example {
public static void main(String[] args) {
Integer num = 5; // Autoboxing occurs here
}
}
In this case, although you only wrote one line, the JVM actually creates an Integer
object due to autoboxing.
String Interning
Moreover, consider strings. Java uses a special memory pool for string literals known as the String pool. Objects that share the same literal are reused rather than recreated:
String str1 = "Hello";
String str2 = "Hello"; // Same object reused
Here, str1
and str2
point to the same object in memory, contrary to popular belief that each assignment generates a new object.
Object Pooling
Another common concept in Java object management is object pooling. This design pattern creates a set of initialized objects that can be reused, aiming to save on creation and destruction time. For example, a pool of database connections allows for efficient resource management.
Java Garbage Collection
Understanding object creation in Java also involves understanding garbage collection (GC). The GC is responsible for reclaiming memory by deleting objects that are no longer in use. But how does it determine whether an object can be destroyed?
Java uses reference counting, among other methods, to track object usage. An object is retained as long as one or more references point to it. Once it becomes unreachable, the garbage collector will eventually free the memory.
Consider the following code:
public class GarbageCollectionExample {
public static void main(String[] args) {
Dog dog = new Dog("Buddy");
dog = null; // Now the Dog object can be garbage collected
}
}
In this scenario, when dog
is set to null
, the Dog
object is eligible for garbage collection, reducing the count of existing objects.
Profiling Object Creation with Tools
To measure how many objects are truly being created in a Java application, you can make use of profiling tools. Libraries like VisualVM, YourKit, or Java Mission Control can help identify object creation hotspots in your application.
Example Using VisualVM
- Install VisualVM from the official site.
- Connect to your application.
- Enable the "Monitor" tab to observe memory usage and object counts over time.
This real-time monitoring provides precise insights into your application's performance, informing how many objects are created and how effectively memory is managed.
Practical Strategies for Managing Object Creation
Understanding how Java manages objects can lead to better software design and optimization. Here are some practical strategies you can implement:
1. Use Primitive Types When Possible
Whenever possible, opt for primitive types instead of their boxed counterparts. Since primitives do not incur the overhead of object creation, they are far more efficient.
// Prefer int over Integer whenever possible
int count = 10; // Efficient
2. Leverage StringBuilder
When building strings dynamically, use StringBuilder
instead of concatenating strings with the +
operator. This reduces the number of intermediate String
objects created, which optimizes memory usage.
StringBuilder sb = new StringBuilder();
sb.append("Hello").append(" ").append("World");
String finalString = sb.toString();
3. Implement Object Pools
For frequently created and destroyed objects, consider using object pools. This technique can significantly reduce instantiation overhead by reusing existing instances.
class ObjectPool {
private List<Dog> availableDogs;
public ObjectPool() {
availableDogs = new ArrayList<>();
}
public Dog acquireDog() {
if (availableDogs.isEmpty()) {
return new Dog("New Dog");
} else {
return availableDogs.remove(availableDogs.size() - 1);
}
}
public void releaseDog(Dog dog) {
availableDogs.add(dog);
}
}
The Bottom Line
Understanding how many objects are created in Java is crucial for optimizing application performance. The common belief that only explicitly instantiated objects count is misleading. Factors like autoboxing, string interning, and dynamic object creation all contribute to an inflated object count.
Utilizing profiling tools can shine a light on your application's memory usage patterns, while practices like using primitive types, StringBuilder
, and object pooling can lead to more efficient memory management.
Thus, debunking the myth about Java object creation empowers developers to write more efficient, performant code. Apply these insights today, and watch your Java applications thrive in terms of performance!
Additional Resources
- Effective Java by Joshua Bloch
- The Java™ Tutorials
- Understanding Java Garbage Collection
Happy coding!
Checkout our other articles