Common Pitfalls in Secure Software Design You Must Avoid
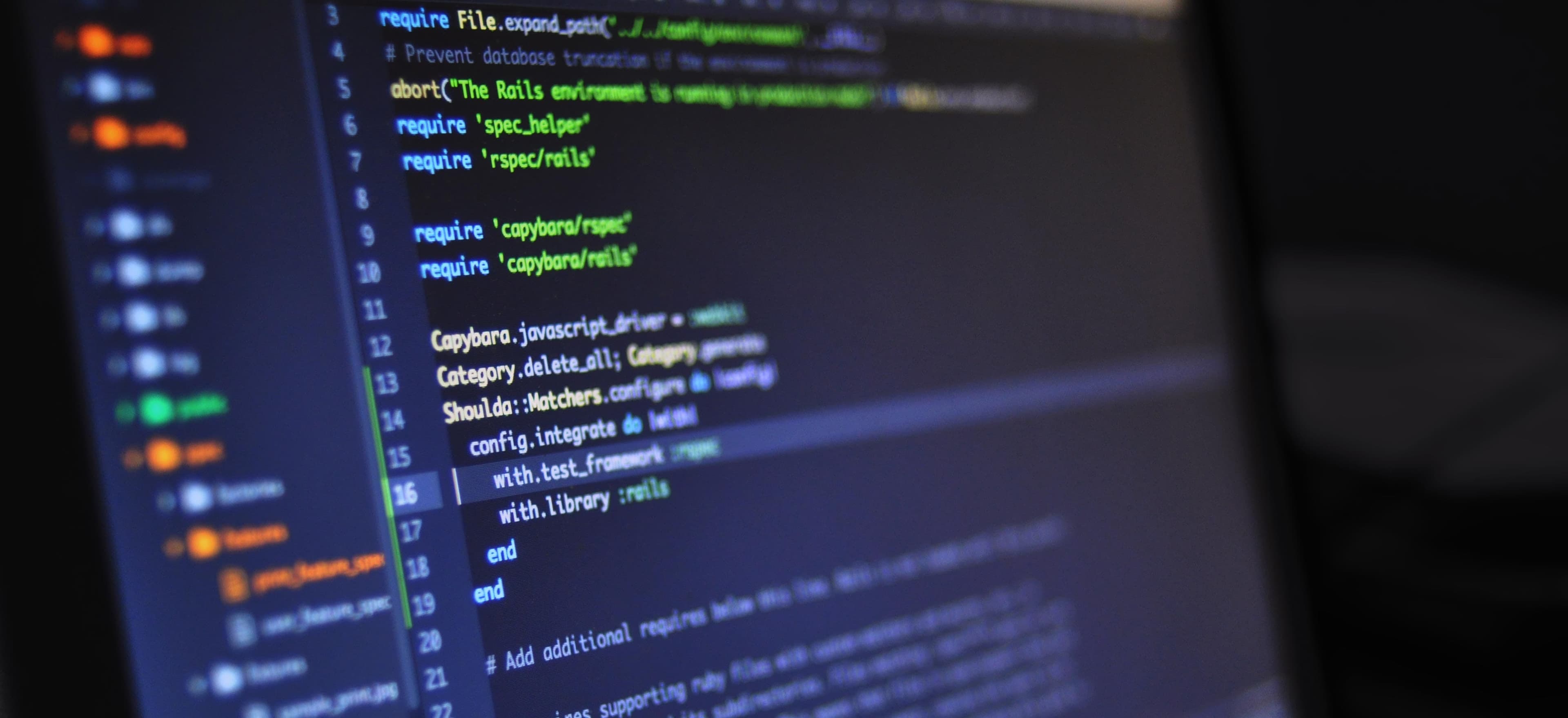
- Published on
Common Pitfalls in Secure Software Design You Must Avoid
In today's digital age, secure software design is more crucial than ever. As cyber threats evolve, the stakes for developing secure applications also heighten. Poorly designed software can lead to significant vulnerabilities, which can be exploited by malicious actors. This blog post will discuss common pitfalls in secure software design and how to avoid them, ensuring that your applications are resilient and robust against attacks.
Understanding Secure Software Design
Before diving into the pitfalls, it's essential to clarify what secure software design entails. Secure software design incorporates principles and practices that aim to create systems resistant to unauthorized access, data breaches, and other security threats. It involves considering security implications throughout the software development lifecycle (SDLC), from design and development to deployment and maintenance.
Common Pitfalls in Secure Software Design
1. Neglecting Threat Modeling
Why It Matters: Threat modeling is a structured approach to identifying potential threats, vulnerabilities, and the likelihood of exploitation. By neglecting this process, developers can overlook critical security flaws.
Avoiding the Pitfall: Implement formal threat modeling sessions during the design phase. Use methodologies such as STRIDE or PASTA to systematically assess and prioritize threats.
Code Example: Basic Threat Modeling
public void threatModeling(String component) {
// Example of identifying a potential threat
if (component.equals("UserAuthentication")) {
System.out.println("Potential Threat: Brute Force Attack");
} else if (component.equals("DataTransmission")) {
System.out.println("Potential Threat: Eavesdropping");
}
}
Commentary: This code snippet simplifies the threat modeling process. Identifying threats by component helps developers focus on the necessary security measures.
2. Hardcoding Secrets
Why It Matters: Storing sensitive data such as API keys, passwords, or private keys directly in the codebase exposes them to anyone who can access the source code.
Avoiding the Pitfall: Utilize environment variables or secure vault services to manage sensitive information. This keeps secrets out of the codebase and reduces the risk of accidental exposure.
Code Example: Accessing Environment Variables
public String getDatabasePassword() {
return System.getenv("DB_PASSWORD");
}
Commentary: This approach retrieves the database password from an environment variable, promoting secure secret handling.
3. Ignoring Input Validation
Why It Matters: Failing to validate input can lead to various attacks such as SQL Injection, Cross-Site Scripting (XSS), or Buffer Overflows.
Avoiding the Pitfall: All user inputs must be validated, sanitized, and encoded. Using libraries that handle these tasks can significantly lower the risk of exploits.
Code Example: Input Validation
public boolean isValidEmail(String email) {
String emailRegex = "^[a-zA-Z0-9_+&*-]+(?:\\.[a-zA-Z0-9_+&*-]+)*@(?:[a-zA-Z0-9-]+\\.)+[a-zA-Z]{2,7}$";
return email.matches(emailRegex);
}
Commentary: This code checks email address formats before accepting them. Implementing such validations helps ensure only legitimate data proceeds through the system.
4. Using Outdated Libraries
Why It Matters: Libraries and frameworks often release updates to patch security vulnerabilities. Ignoring these updates leaves applications exposed to known threats.
Avoiding the Pitfall: Regularly update dependencies and monitor them for vulnerabilities. Use tools like OWASP Dependency-Check to identify outdated libraries.
Code Example: Dependency Management
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.4</version> <!-- Check for the latest version -->
</dependency>
Commentary: Manage dependencies carefully in your pom.xml
. Always review and update dependencies to the latest versions to mitigate security risks.
5. Overlooking Error Handling
Why It Matters: Poor error handling can expose sensitive information or lead to denial-of-service attacks.
Avoiding the Pitfall: Ensure that error messages do not disclose sensitive information. Implement proper logging mechanisms to capture critical information while keeping user-facing messages vague.
Code Example: Error Handling
public void handleError(Exception e) {
// Log the full error internally
logger.error("An error occurred: ", e);
// Send a generic message to the user
System.out.println("An unexpected error occurred. Please try again later.");
}
Commentary: This snippet demonstrates a balance between logging errors for internal diagnostics and providing a secure, non-specific error message to the user.
6. Failing to Implement Least Privilege
Why It Matters: Users and applications should have only the minimum necessary access they require to perform their functions. Failing to enforce this principle can lead to unauthorized access.
Avoiding the Pitfall: Conduct regular audits of permissions and roles. Ensure that users' access levels are continuously reviewed and adjusted based on their current needs.
Code Example: Role-Based Access Control
public void accessResource(User user) {
if (!user.hasRole("ADMIN")) {
throw new SecurityException("Access denied: Insufficient permissions.");
}
// Proceed to access resource
}
Commentary: This simple access control mechanism ensures that only users with the correct role can access certain resources.
7. Neglecting Security Testing
Why It Matters: Security testing is often an afterthought, leading to the identification of vulnerabilities late in the development process or, worse, after deployment.
Avoiding the Pitfall: Integrate security testing into the regular testing pipeline, using tools like static analysis, penetration testing, and dynamic analysis.
Code Example: Static Code Analysis Example
spotbugs -effort:max -output SpotBugs.xml /path/to/your/class/files
Commentary: Running static analysis tools can help identify vulnerabilities before they reach production. Automating this in the CI/CD process ensures continuous security checks.
Wrapping Up
Building secure software is an ongoing commitment that demands diligence and attention to detail. By understanding and avoiding these common pitfalls, developers can enhance the security posture of their applications and contribute to a safer digital landscape.
To delve deeper into secure software design practices, consider exploring OWASP's secure coding guidelines and Microsoft's secure development lifecycle.
Take these lessons to heart and implement robust security measures in your development process. The effort not only protects your users but also strengthens the integrity and reputation of your software. Embrace security as a foundational aspect of your design and development strategy.
Checkout our other articles