Unlocking the Mystery: Managing ActiveMQ Message Priorities
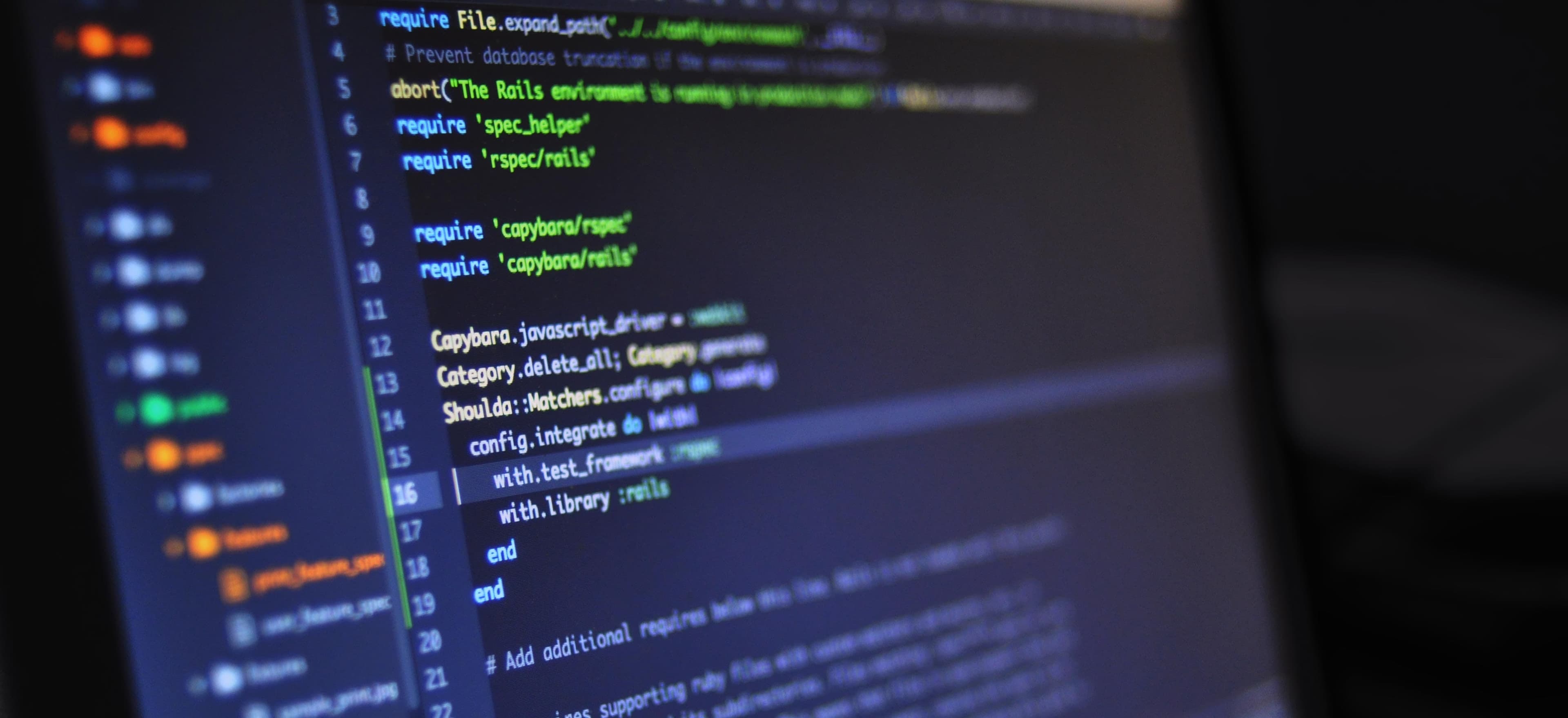
- Published on
Unlocking the Mystery: Managing ActiveMQ Message Priorities
Apache ActiveMQ is a popular message broker that allows applications to communicate through messages. One essential feature that can significantly impact messaging performance is the priority system. In this blog post, we will explore how message priorities work in ActiveMQ, why they are important, and provide code examples to help you manage message priorities effectively.
Table of Contents
- What Are Message Priorities?
- Why Use Message Priorities?
- Setting Message Priorities in ActiveMQ
- A Code Example
- Best Practices for Handling Priorities
- Conclusion
What Are Message Priorities?
Message priorities in ActiveMQ allow messages to be processed in a certain order based on their importance. ActiveMQ supports a range of priorities between 0 (lowest) and 9 (highest). When a consumer retrieves messages from the queue, it will first process messages with higher priority before those with lower priority.
Key Concepts
- Priority Level: Each message can have a priority value between 0 and 9.
- Ordering: Messages with higher priority are delivered first.
Why Use Message Priorities?
Utilizing message priorities brings several advantages:
- Resource Optimization: By processing high-priority messages first, you can make better use of system resources.
- Improved User Experience: Critical messages can be acted on faster, leading to timely responses in user-facing applications.
- Better Control Over Processing: You can exert more control over how messages are handled within your application, which can be vital for mission-critical systems.
Setting Message Priorities in ActiveMQ
Setting up priorities in ActiveMQ is straightforward. When a message is created, you can assign it a specific priority level. In code, this process might look as follows:
Sample Code
import org.apache.activemq.ActiveMQConnectionFactory;
import javax.jms.*;
public class PriorityMessageSender {
public static void main(String[] args) throws JMSException {
// Create a connection to the ActiveMQ broker
ConnectionFactory connectionFactory = new ActiveMQConnectionFactory("tcp://localhost:61616");
Connection connection = connectionFactory.createConnection();
connection.start();
// Create a Session
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
// Create Destination (Queue)
Destination destination = session.createQueue("SampleQueue");
// Create a MessageProducer
MessageProducer producer = session.createProducer(destination);
// Create a high-priority message
TextMessage highPriorityMessage = session.createTextMessage("High Priority Message");
producer.send(highPriorityMessage, DeliveryMode.NOT_PERSISTENT, 9, 0); // Priority: 9
// Create a low-priority message
TextMessage lowPriorityMessage = session.createTextMessage("Low Priority Message");
producer.send(lowPriorityMessage, DeliveryMode.NOT_PERSISTENT, 1, 0); // Priority: 1
// Clean up
producer.close();
session.close();
connection.close();
}
}
Commentary on the Code
- ActiveMQConnectionFactory: This establishes a connection to the ActiveMQ broker. The URL specifies the broker's address.
- Priority Levels: Notice how the
send
method includes parameters for delivery mode, priority, and time-to-live. Higher numbers in the priority field ensure that critical messages are consumed first. - Message Types: TextMessage is used here, but ActiveMQ supports other message types such as ObjectMessage and BytesMessage.
A Code Example
Let's further explore how the consumer side behaves with these messages. The following code demonstrates how a consumer receives messages based on their priorities:
Sample Consumer Code
import org.apache.activemq.ActiveMQConnectionFactory;
import javax.jms.*;
public class PriorityMessageConsumer {
public static void main(String[] args) throws JMSException {
// Create a connection
ConnectionFactory connectionFactory = new ActiveMQConnectionFactory("tcp://localhost:61616");
Connection connection = connectionFactory.createConnection();
connection.start();
// Create a session
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
// Create Destination (Queue)
Destination destination = session.createQueue("SampleQueue");
// Create a MessageConsumer
MessageConsumer consumer = session.createConsumer(destination);
// Receive messages
while (true) {
Message message = consumer.receive();
if (message instanceof TextMessage) {
TextMessage textMessage = (TextMessage) message;
System.out.println("Received: " + textMessage.getText());
}
}
// Clean up
// consumer.close();
// session.close();
// connection.close();
}
}
Commentary on the Consumer Code
- Continuous Listening: The
while (true)
loop allows the consumer to continuously receive messages. This is crucial in scenarios requiring real-time processing. - Type Checking: The consumer checks if the message is of type
TextMessage
for proper handling. - Priority Processing: Here, the consumer will always pick higher-priority messages from the queue first before others, thanks to the priority mechanism in ActiveMQ.
Best Practices for Handling Priorities
To effectively manage message priorities in ActiveMQ, consider the following best practices:
-
Avoid Overuse of High Priorities: Always use the priority levels judiciously. If all messages are set to high priority, it negates the benefit. This can lead to a situation known as priority inversion.
-
Monitor Performance: Regularly monitor the performance of your message-processing application. Adjust priorities based on insights gathered. Tools like ActiveMQ Web Console can aid in monitoring.
-
Consider Alternative Approaches: In specific cases, consider using different queues for different priority levels. For example, have a separate low-priority queue to allow better management and separation.
-
Testing: Before deploying changes to production, rigorously test different priority settings in a staging environment. This helps ensure that the application behaves as expected.
Wrapping Up
Managing message priorities in ActiveMQ can enhance your application's performance and reliability. By understanding how to set and retrieve message priorities, minimizing the risks associated with improper usage, and following best practices, you can ensure that critical messages are prioritized correctly.
For additional reading, visit Apache ActiveMQ Documentation for further insights into specific configuration settings and extended use cases.
Happy coding!
Checkout our other articles