Streamlining JDBC ResultSets: Convert to Object Streams Easily
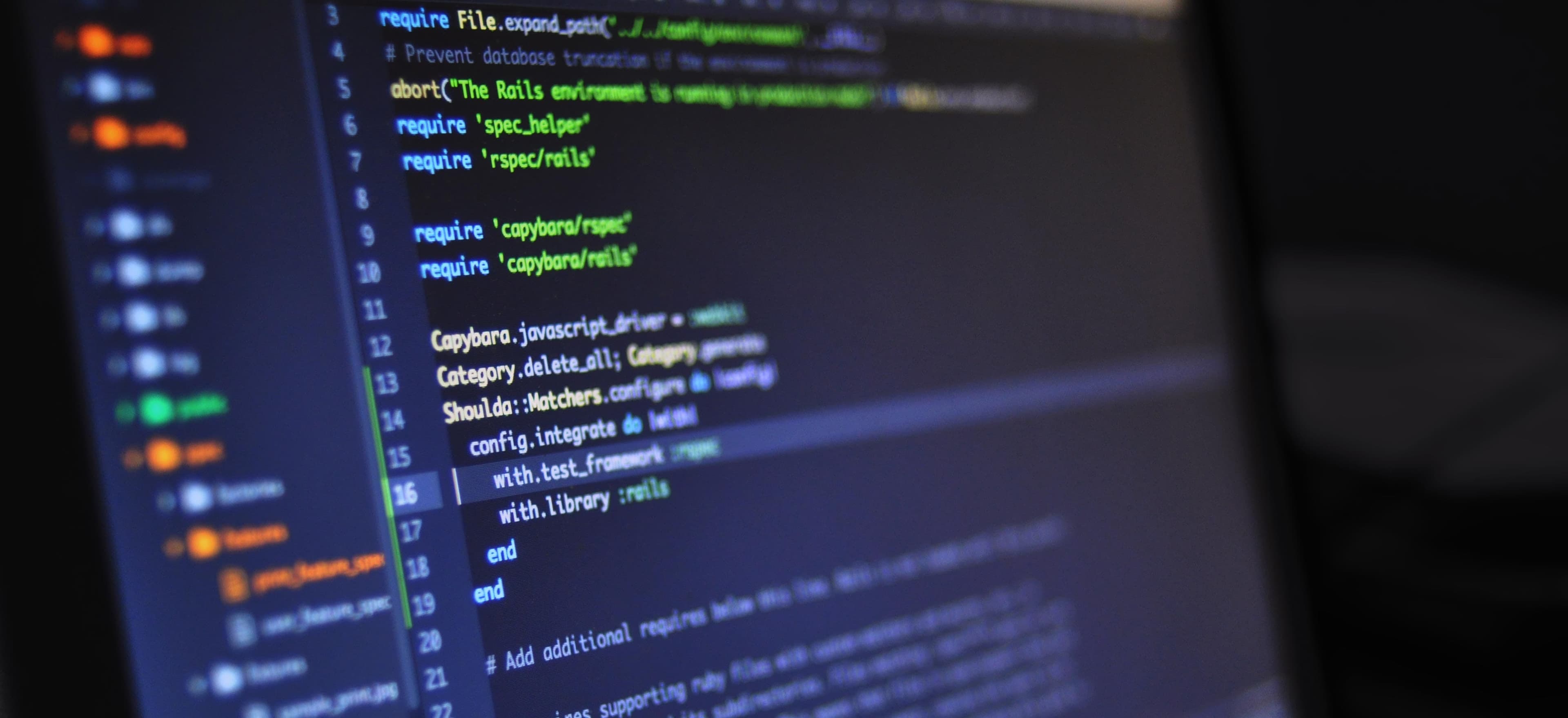
- Published on
Streamlining JDBC ResultSets: Convert to Object Streams Easily
Java Database Connectivity (JDBC) is an API that enables Java applications to interact with databases. It's a powerful tool, but specifically handling data retrieval through ResultSet
can sometimes feel cumbersome. Many developers have encountered tedious processes when extracting data and converting it into usable Java objects. In this post, we’ll explore how you can streamline your JDBC ResultSet
handling by converting it to object streams efficiently.
Understanding JDBC ResultSets
Before diving into conversion techniques, it’s critical to understand the fundamentals of JDBC ResultSet
. When you execute a SQL query using JDBC, the database returns the result in a ResultSet
. The ResultSet
essentially acts like a cursor that points to the rows of the data returned. However, working directly with ResultSet
can lead to verbosity and readability issues.
Example of Basic JDBC ResultSet Usage
Here’s a fundamental example of retrieving data from a database using JDBC:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class SimpleJDBCExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
try {
Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT id, name FROM students");
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
System.out.println("Student ID: " + id + ", Name: " + name);
}
resultSet.close();
statement.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, we're connecting to a MySQL database, executing a query, and then iterating through the ResultSet
to print student names. But as your application scales, repeating this code can quickly become cumbersome. Thus, we seek a more elegant solution by converting ResultSet
objects into a stream of Java objects.
The Need for Conversion
Converting ResultSet
into Java objects can significantly enhance the readability and maintainability of your code. Rather than working with cursor-like ResultSet
objects, you can treat data as elements of a collection. This method aligns well with the object-oriented principles that Java prides itself on.
Benefits of Using Object Streams
- Clarity: Processing is clearer when working with objects rather than arrays or lists of primitive data types.
- Maintainability: Maintaining code becomes easier since objects can encapsulate behaviors as well as data.
- Stream Processing: Leveraging Java Streams allows you to take advantage of functional programming paradigms. This can lead to more readable and expressive operations.
The Java Object Stream Approach
Here's a structured way of converting a JDBC ResultSet
into a collection of objects using Java Streams.
Step 1: Create Your Model Class
First, define what a student looks like. We’ll create a simple Java class:
public class Student {
private int id;
private String name;
public Student(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
@Override
public String toString() {
return "Student{" + "id=" + id + ", name='" + name + '\'' + '}';
}
}
Step 2: Implement ResultSet to Stream Conversion
Next, we’ll create a utility method that takes a ResultSet
and converts it into a stream of Student
objects:
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class ResultSetUtil {
public static List<Student> convertToStudentList(ResultSet resultSet) throws SQLException {
List<Student> students = new ArrayList<>();
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
students.add(new Student(id, name));
}
return students;
}
}
In this method, we iterate through the ResultSet
, creating new Student
objects and adding them to a list. The method returns this list, simplifying the downstream processing.
Step 3: Stream the Results
To use the new conversion method, we can modify our original code as follows:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
try (Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement()) {
ResultSet resultSet = statement.executeQuery("SELECT id, name FROM students");
List<Student> students = ResultSetUtil.convertToStudentList(resultSet);
students.forEach(System.out::println);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Understanding the Code
In the modified example:
- We maintain cleaner code by using a separate utility class to handle
ResultSet
conversion. - Using a list of
Student
objects allows us to utilize Java Streams and other operations like filtering, mapping, and reducing easily.
Leveraging Streams Further
Now that you have a clean list of Student
objects, let’s apply some powerful stream operations. Suppose you want to filter students by name and collect them into a new list:
List<Student> filteredStudents = students.stream()
.filter(student -> student.getName().startsWith("A"))
.collect(Collectors.toList());
filteredStudents.forEach(System.out::println);
This snippet filters out students whose names start with 'A' and prints them in a more functional style.
The Last Word
By converting ResultSet
objects into Java object streams, you improve code clarity and reduce redundancy. Through the steps outlined in this post, we can seamlessly integrate database interactions into our object-oriented programming paradigm.
Final Thoughts
Adopting these practices not only makes your code more maintainable but also sets a solid ground for further enhancements, such as implementing design patterns or integrating with frameworks like Spring. Remember that code quality is essential in maintaining efficient collaboration among development teams.
For more resources on JDBC and streams, consider checking out the official Java Documentation on JDBC and Java Stream API Guide. Happy coding!
Additional References
This guide presents an essential approach towards better database handling in Java using JDBC, ensuring your projects remain scalable and maintainable.
Checkout our other articles