Why Unit Testing Standards Fail in QA Learning
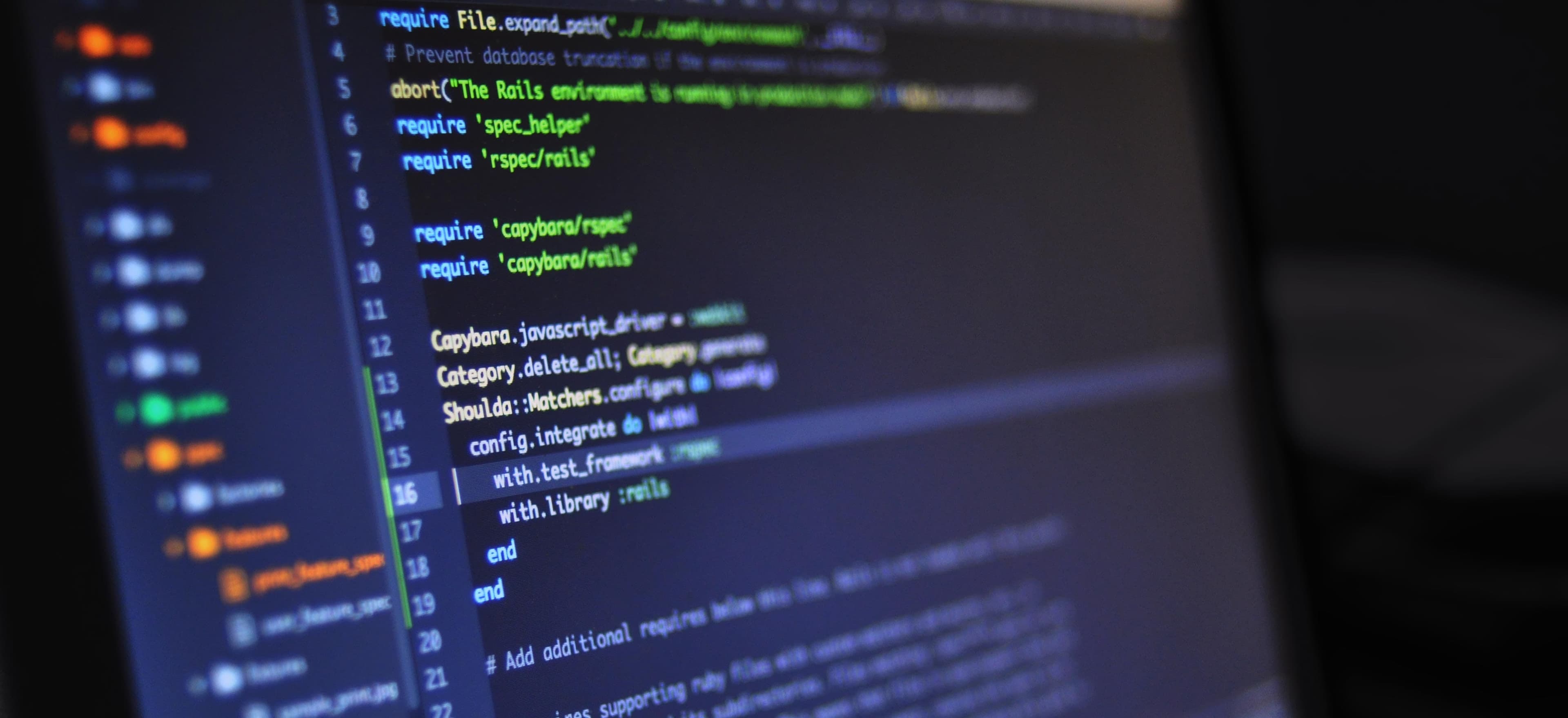
- Published on
Why Unit Testing Standards Fail in QA Learning
Unit testing is an essential practice in quality assurance (QA) that ensures individual components of software function correctly. Despite its importance, many organizations struggle with implementing effective unit testing standards. This blog post delves into the reasons behind the failure of unit testing standards in QA learning and suggests ways to improve this crucial aspect of software development.
Understanding Unit Testing
Before we explore the failures in unit testing standards, let's define what unit testing is. According to JUnit, unit testing involves testing the smallest testable parts of an application. These tests are typically automated and executed by developers during the development phase, allowing for continuous feedback and improvements.
Example: Writing a Simple Unit Test in Java
Let's illustrate unit testing with a simple Java example:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3)); // Here we expect the sum of 2 and 3 to equal 5
}
}
In this snippet, we define a CalculatorTest
class to test a Calculator
class. The testAdd
method checks whether the add
method returns the correct sum.
Reasons Why Unit Testing Standards Fail
-
Lack of Understanding Among Stakeholders
Many stakeholders in software development—from product managers to developers—do not fully understand the nuances of unit testing. This misunderstanding leads to unrealistic expectations, resulting in poorly defined testing standards.
To overcome this challenge, it's crucial to invest time in educating all stakeholders on the benefits and methodologies of unit testing. Regular workshops and training can keep everyone aligned.
-
Inconsistent Testing Practices
Organizations often fail to establish a consistent approach to unit testing. Different teams may employ various frameworks, methodologies, and structures, making it difficult to maintain a standard.
A solution is to adopt a unified unit testing framework, such as JUnit or TestNG. These frameworks provide structure and consistency, promoting best practices across all teams.
-
Insufficient Test Coverage
One common pitfall is focusing solely on code coverage metrics. While high code coverage can indicate a well-tested application, it doesn't guarantee that the tests are meaningful or effective.
Aim for a balance between quantity and quality. For example, consider scenarios in which code could fail and create tests that specifically target those situations.
@Test public void testDivide() { Calculator calculator = new Calculator(); assertThrows(ArithmeticException.class, () -> { calculator.divide(5, 0); // Testing expected exception when dividing by zero }); }
-
Neglecting Code Maintenance
Unit tests are often written at the same time as the code but can be ignored during maintenance or refactoring. When developers change the main code, they might forget to update or remove the corresponding tests.
Establish good practices for maintaining tests. Every code review should include a check for corresponding unit tests. Using version control systems, like Git, can also help track changes and ensure tests are keeping pace.
-
Time Constraints and Productivity Pressures
Developers often face pushback on writing unit tests due to time constraints. They are pressured to deliver features quickly, leading to skipped tests and ignored best practices.
Encourage a culture where unit testing is valued as a part of development that can reduce future debugging and long-term maintenance costs. Consider employing practices such as “Test-Driven Development” (TDD), which integrates testing into the development process.
-
Over-reliance on Manual Testing
In many organizations, there is a misconception that unit testing can be fully replaced by manual QA processes. Relying solely on manual testing often leads to incomplete test coverage and missed defects.
While manual testing plays a vital role, it should complement rather than replace automated unit tests. Automated tests provide faster feedback and are more reliable at catching regressions.
Improving Unit Testing Standards
-
Establish Clear Guidelines
Create a comprehensive documentation that outlines the unit testing standards your organization should follow. This documentation should cover naming conventions, testing frameworks, and expectations for test coverage.
-
Emphasize the Importance of Testing
Foster an environment where unit testing is seen as a non-negotiable aspect of software development. Recognize team members who exemplify good testing practices, encouraging others to follow suit.
-
Implement Pair Programming
Pair programming can help programmers learn from each other, including how to write effective unit tests. By working together, developers can share insights and experiences, improving the overall quality of both the code and the tests.
-
Review Tests Regularly
Establish a routine process for reviewing unit tests. The review could involve QA and development teams where they assess the relevance, effectiveness, and coverage of existing tests.
-
Measure and Adjust
Use metrics such as code coverage, test pass/fail rates, and defect rates to evaluate the effectiveness of your unit testing standards. Make adjustments based on these measurements, aligning them with the goals of your organization.
The Bottom Line
Unit testing is a critical facet of software quality assurance, yet many organizations struggle to implement effective unit testing standards. By identifying and addressing the reasons these standards fail, companies can foster a culture of testing that enhances code quality and overall project success.
If you want to delve deeper into improving your testing practices, consider exploring the resources from the Ministry of Testing or Test Automation University. These platforms provide valuable insights and learning resources for software testing and QA professionals.
With the right approach, unit testing can evolve from a mere checkbox in the development process to a robust framework that ensures the delivery of high-quality software products. Embrace the change, invest in training, and watch as your team's effectiveness in unit testing flourishes.