Unpacking Java Method Call Performance: The Speed Dilemma
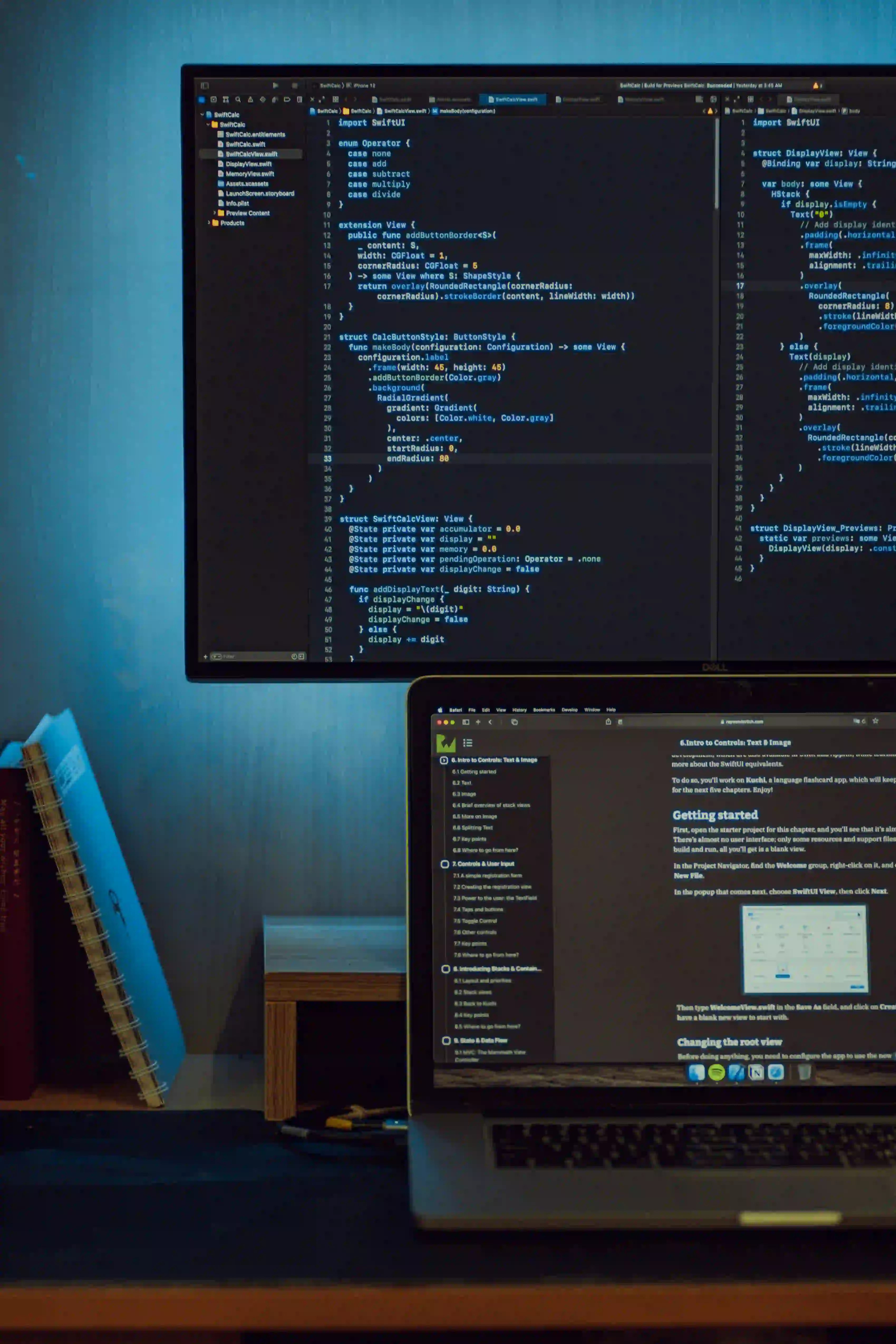
Unpacking Java Method Call Performance: The Speed Dilemma
When developing Java applications, performance can often be a major concern. Among the various factors that contribute to the performance of a Java application, method call performance stands out due to its direct impact on efficiency and speed. Understanding how Java handles method calls, what influences their performance, and how to mitigate potential bottlenecks is key to writing high-quality, responsive applications.
Understanding Java Method Calls
A method call in Java is the mechanism through which a method is invoked. This can be done in several ways: instance methods, static methods, and even through interfaces. The process involves multiple steps, including checking access permissions, handling the stack frame, and managing parameters.
The Mechanics of a Method Call
When you call a method, here’s what happens under the hood:
- Parameter Handling: Arguments are evaluated and passed as actual values to the method.
- Stack Frame Creation: A new stack frame is created within the call stack for the method. This holds local variables and a reference to the method’s bytecode.
- Execution Suspension: The execution context of the calling method is suspended, allowing for the new method's code to be executed.
- Return Value Handling: After execution, the return value is passed back to the calling method, and the stack frame is removed.
This complex process can introduce overhead. However, Java’s Just-In-Time (JIT) compiler optimizes this by compiling bytecode into native machine code at runtime, significantly improving performance.
Key Factors Influencing Method Call Performance
1. Call Type: Static vs. Instance Methods
Consider how you invoke methods. Instance methods involve creating an object, which adds a slight overhead. Static methods, on the other hand, do not require an instance of a class, leading to faster access.
Example: The difference between the two is simple yet critical.
public class SpeedDilemma {
public void instanceMethod() {
System.out.println("Called instance method");
}
public static void staticMethod() {
System.out.println("Called static method");
}
}
// Usage
public class Main {
public static void main(String[] args) {
SpeedDilemma obj = new SpeedDilemma();
obj.instanceMethod(); // Overhead of instance creation
SpeedDilemma.staticMethod(); // Direct access
}
}
In high-performance scenarios, avoiding unnecessary instance creation can lead to gains in speed.
2. Method Overloading and Overriding
Overloading allows multiple methods with the same name but different parameters. Overriding enables a subclass to provide a specific implementation of a method declared in its superclass. While these features enhance flexibility and reusability, they can also add some overhead due to the added checks performed to resolve which method to execute.
Example of Overloading:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
// Calls are resolved based on the parameter type
Example of Overriding:
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
In such cases, choosing between compile-time or run-time method resolution can affect performance.
3. Boxing and Unboxing
In Java, primitive types (like int
, char
, etc.) are stored differently than their object counterparts (like Integer
, Character
, etc.). When a primitive is converted to an object, known as boxing, it introduces overhead. Consecutively converting back to a primitive, called unboxing, can also incur a performance penalty.
Example of Boxing and Unboxing:
Integer boxed = new Integer(5); // Boxing
int unboxed = boxed.intValue(); // Unboxing
Avoiding excessive boxing and unboxing can contribute to improved method call efficiency.
4. Number of Method Calls
Simply put, the more method calls you make, the longer the execution time. It is essential to keep method calls to a minimum in performance-critical sections of your code.
Example: Direct calculations are better than multiple method calls.
// Less efficient
public int calculate(int a, int b) {
return add(a, b);
}
private int add(int a, int b) {
return a + b;
}
Improved:
// More efficient
public int calculate(int a, int b) {
return a + b; // Direct calculation
}
Profiling and Monitoring Performance
Before making any changes, it is crucial to profile your applications to identify where the bottlenecks occur. Tools like VisualVM or Java Mission Control can help you inspect method calls and their performance impacts.
Using VisualVM
To profile your application with VisualVM:
- Download and install VisualVM.
- Run your Java application.
- Open VisualVM and connect to your running Java process.
- Monitor CPU usage, memory, and method call statistics.
- Use the call tree to identify slow methods.
Code Optimization Techniques
1. Inline Methods
Inlining is a technique where the method call is replaced with the method's body. This can reduce the overhead of method calls, especially for small, frequently called methods.
Example:
// Original
public int square(int x) {
return x * x;
}
// After inlining
public int calculateArea(int radius) {
return radius * radius; // Inlined calculation
}
2. Use Interfaces Judiciously
While interfaces enable flexibility, excessive use can lead to increased overhead. In performance-critical sections of code, prefer direct class usage where possible.
3. Short-Lived Objects
Creating short-lived objects can lead to memory pressure and frequent garbage collection pauses. Consider reusing objects when feasible, especially in loops.
A Final Look
The performance of Java method calls is impacted by various factors, from the type of methods being called to the overhead introduced by boxing and unboxing. Understanding these aspects can lead developers to make more informed choices when writing code. Regular profiling is essential to identify performance bottlenecks effectively.
By considering these techniques and adjustments, you'll be able to enhance the speed and efficiency of your Java applications. Performance should always be balanced with maintainability and readability, but with careful optimization, significant gains can be achieved.
For further reading, check out these resources:
- Java Performance Tuning Guide
- Understanding Java Method Performance
By thoughtfully navigating the nuances of Java method calls, you can create applications that are not only efficient but also robust and maintainable. Happy coding!