Unlocking Speed: Top Tips for Performance Optimization
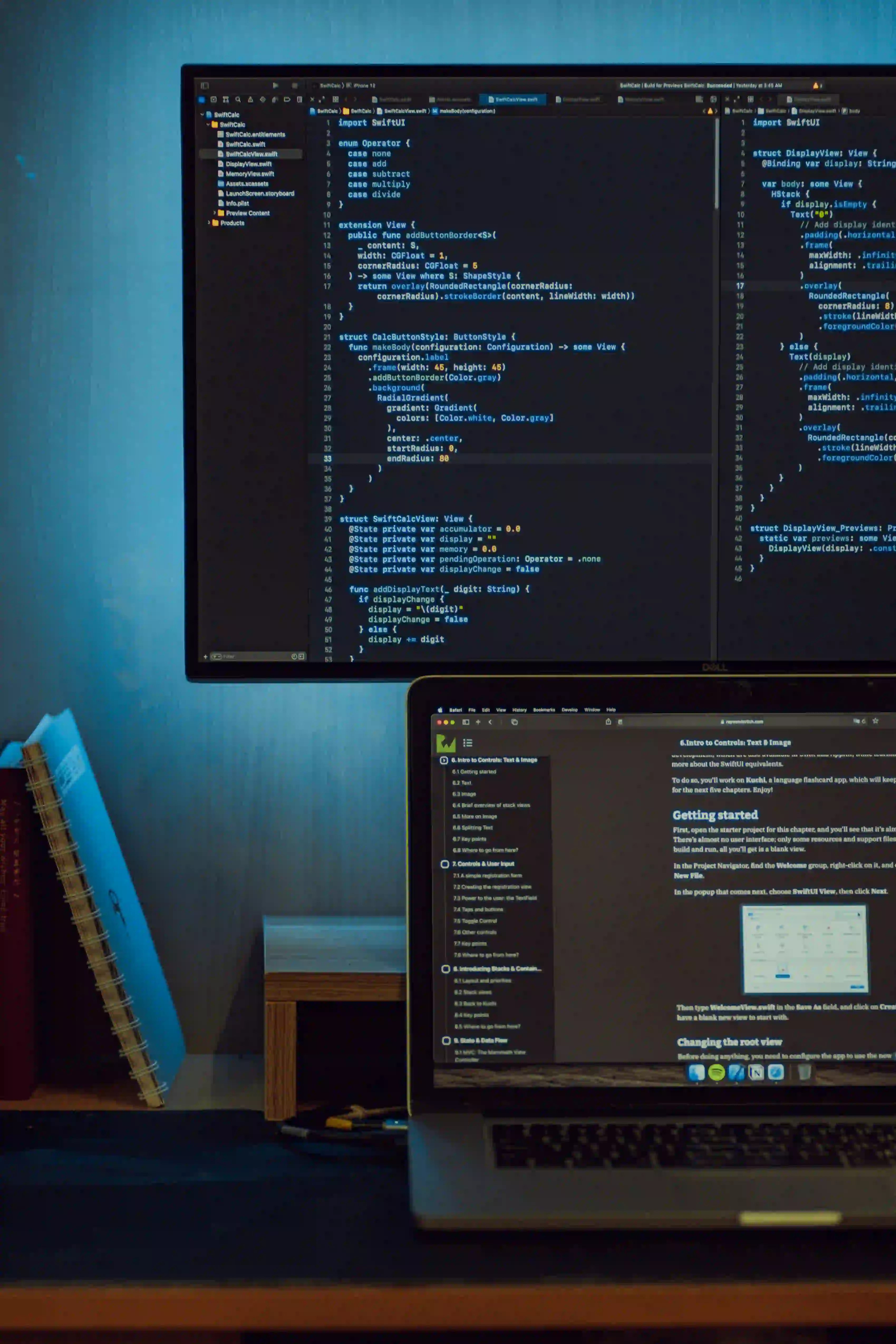
Unlocking Speed: Top Tips for Performance Optimization in Java
In the modern world, software performance is crucial for user satisfaction and operational efficiency. Particularly in Java development, where large applications can easily suffer from performance issues, optimization becomes an integral part of the development cycle. In this blog post, we will explore practical tips and techniques aimed at enhancing the performance of your Java applications.
Table of Contents
- Understanding Java Performance
- Optimizing Memory Usage
- Utilizing Proper Data Structures
- Improving I/O Operations
- Multithreading: Concurrency Matters
- Profiling and Benchmarking Your Application
- Conclusion
1. Understanding Java Performance
Java is a versatile programming language that runs on the Java Virtual Machine (JVM). Understanding how the JVM works can significantly impact the performance of your application. The JVM optimizes code execution using Just-In-Time (JIT) compilation, but there are numerous ways you can help it run more efficiently.
2. Optimizing Memory Usage
Memory management is fundamental to Java performance. Here are key strategies:
Use Primitive Types Wisely
While Java provides wrapper classes for primitive types (like Integer for int), they consume more memory.
// Using int vs. Integer
int primitiveInt = 10; // 4 bytes
Integer wrapperInt = Integer.valueOf(10); // 16 bytes
Why? Primitive types are significantly less memory-intensive than their wrapper counterparts. Opt for primitives whenever you can to lighten the heap usage.
Take Advantage of String Interning
Strings in Java are immutable, meaning that numerous identical strings can consume excess memory. String interning helps alleviate this issue.
String regularString = new String("Hello");
String internedString = regularString.intern();
Why? By calling intern()
, you're ensuring that duplicate strings will reference the same memory address, thereby optimizing memory usage.
3. Utilizing Proper Data Structures
The choice of data structures can significantly affect the performance of your Java application.
Choosing the Right Collection
Using the right Java collection can improve performance drastically. For example:
- Use
ArrayList
for fast random access. - Use
LinkedList
for frequent insertions and deletions.
Here's a brief comparison:
List<Integer> arrayList = new ArrayList<>();
List<Integer> linkedList = new LinkedList<>();
// Adding elements to both
arrayList.add(1); // O(1)
linkedList.add(1); // O(1)
arrayList.remove(0); // O(n)
linkedList.remove(0); // O(1)
Why? Understanding the operational costs (time complexity) of various data structures allows you to choose one that optimally serves your application’s needs.
4. Improving I/O Operations
Input/Output operations can become bottlenecks in your application. Here are suggestions to optimize these:
Buffered Streams
Using buffered streams can improve performance when reading or writing large amounts of data.
try (BufferedReader reader = new BufferedReader(new FileReader("input.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
Why? Buffered streams read and write data in larger chunks, reducing the number of I/O operations, hence improving performance.
Asynchronous File I/O
In scenarios involving multiple I/O tasks, asynchronous I/O can be a game-changer:
AsynchronousFileChannel fileChannel = AsynchronousFileChannel.open(Paths.get("file.txt"), StandardOpenOption.READ);
ByteBuffer buffer = ByteBuffer.allocate(1024);
fileChannel.read(buffer, 0, null, new CompletionHandler<Integer, Object>() {
@Override
public void completed(Integer result, Object attachment) {
System.out.println("Read " + result + " bytes");
}
@Override
public void failed(Throwable exc, Object attachment) {
exc.printStackTrace();
}
});
Why? Asynchronous operations allow your application to perform other tasks while waiting for I/O operations to complete, thus improving responsiveness and overall throughput.
5. Multithreading: Concurrency Matters
In Java, taking advantage of concurrency can lead to substantial performance gains, particularly in CPU-bound operations.
Leveraging Executor Framework
Instead of managing threads manually, use the Executor framework:
ExecutorService executor = Executors.newFixedThreadPool(4);
executor.submit(() -> {
// Your task here
});
executor.shutdown();
Why? The executor framework simplifies thread management and helps prevent the pitfalls of thread lifecycle management and resource leaks.
Use Concurrency Utilities
Java provides several utilities in the java.util.concurrent
package, such as CountDownLatch
and Semaphore
. These can help coordinate tasks.
CountDownLatch latch = new CountDownLatch(2);
// Create multiple threads
new Thread(() -> {
// Task
latch.countDown();
}).start();
new Thread(() -> {
// Another Task
latch.countDown();
}).start();
latch.await(); // Will wait until both threads complete
Why? These utilities make it easier to handle complex thread coordination without excessive overhead.
6. Profiling and Benchmarking Your Application
Optimization is an iterative process. Employ profiling and benchmarking tools to identify areas for improvement.
Using Java Flight Recorder
Java Flight Recorder is a powerful tool integrated in the JVM that can help you analyze performance. You can generate recordings and analyze CPU usage, memory consumption, and other metrics.
java -XX:StartFlightRecording=duration=60s,filename=recording.jfr -jar YourApp.jar
Why? Profiling provides granular insight into what parts of your application are slowing it down, allowing you to make informed optimizations.
Microbenchmarking with JMH
The Java Microbenchmark Harness (JMH) is essential for accurately benchmarking small sections of code.
import org.openjdk.jmh.annotations.Benchmark;
public class MyBenchmark {
@Benchmark
public void testMethod() {
// Code to test
}
}
Why? JMH takes care of JVM warm-up, measurement accuracy, and multiple iterations, producing reliable results for your benchmarks.
In Conclusion, Here is What Matters
Optimizing Java applications is a multifaceted process that combines understanding of Java internals, appropriate data structures, efficient memory management, and effective use of concurrency. By applying these tips and techniques, you can significantly enhance the performance of your Java applications.
Performance optimization is an ongoing task. Profile frequently, analyze your results, and don’t shy away from revisiting previously optimized code as new Java versions introduce better tools and methodologies.
For further reading, consider examining resources like Java Performance by Scott Oaks and Effective Java by Joshua Bloch to deepen your knowledge in performance optimization.
By employing these methodologies, you can ensure your Java applications run as efficiently as possible. Happy coding!