Common Pitfalls When Using Simple Java SSH Client
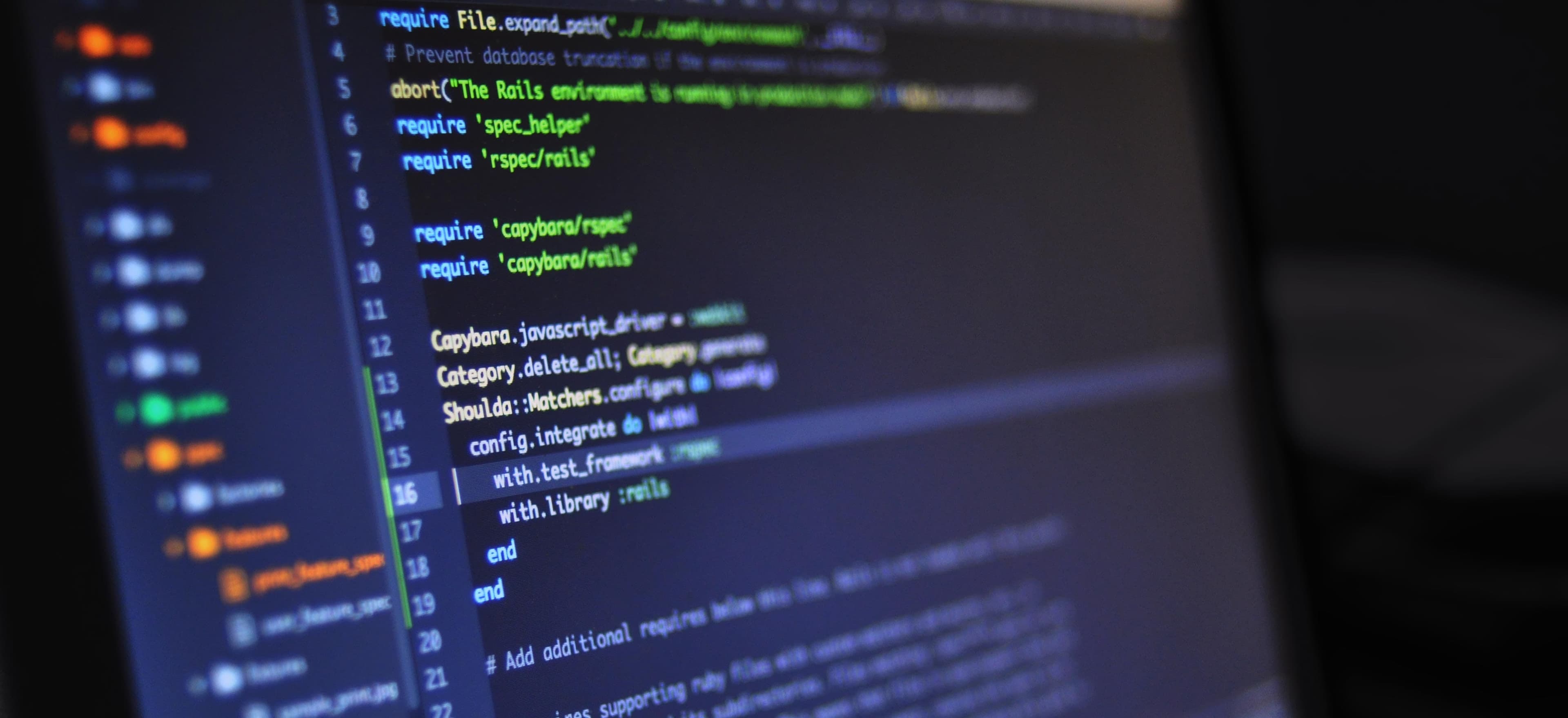
- Published on
Common Pitfalls When Using a Simple Java SSH Client
Java is a powerful language, widely used for developing various applications, including networking tools. One such tool is the SSH client, which allows secure remote connections to servers. While utilizing a simple SSH client in Java can seem straightforward, developers often stumble upon several common pitfalls. This post aims to provide insights into these challenges while offering strategies for overcoming them. By doing so, we'll ensure a smoother development experience for your next SSH project.
Understanding SSH in Java
SSH (Secure Shell) is a cryptographic network protocol that provides a secure way to access a computer over an unsecured network. Java developers typically utilize libraries such as JSch or Apache MINA SSHD for SSH functionalities.
These libraries abstract much of the complexity involved in SSH communications, making it easy for developers to establish secure connections, execute commands, and transfer files. However, this abstraction can sometimes lead to assumptions that can cause problems if not understood correctly.
Common Pitfalls
1. Ignoring Key Management
When establishing an SSH connection, key management is paramount. An oversight in configuring SSH keys may lead to authentication failures.
Example:
import com.jcraft.jsch.*;
public class SshConnection {
public static void main(String[] args) {
String host = "example.com";
String user = "user";
String privateKey = "/path/to/private/key";
try {
JSch jsch = new JSch();
jsch.addIdentity(privateKey);
Session session = jsch.getSession(user, host, 22);
// Setting StrictHostKeyChecking config
session.setConfig("StrictHostKeyChecking", "yes");
session.connect();
System.out.println("Connected successfully!");
session.disconnect();
} catch (JSchException e) {
e.printStackTrace();
}
}
}
Commentary:
In the example above, we are using addIdentity
to specify the private key for authentication. Not only is this a critical step, but the line that enables StrictHostKeyChecking
is equally important. This ensures that your client checks whether the host's key is known, helping protect against man-in-the-middle attacks.
2. Not Handling Exceptions Properly
Network communication comes with plenty of uncertainties. If exceptions are not handled properly, they could lead to application crashes.
In Java, it is essential to catch exceptions effectively and log meaningful messages. Here's how you can add better error handling to your SSH connection:
try {
session.connect();
// Execute commands after establishing the connection
} catch (JSchException e) {
System.err.println("Connection failed: " + e.getMessage());
e.printStackTrace();
}
3. Timeouts and Networking Issues
Many developers overlook setting timeouts when establishing connections. Failing to do so may cause your application to hang indefinitely if a connection cannot be established.
Setting Connection Timeout:
session.connect(5000); // Connect timeout of 5 seconds
Adding a connection timeout like in the snippet above can prevent application hangs. Always opt for a reasonable timeout value depending on your network conditions.
4. Misconfiguring Port Forwarding
Port forwarding is a powerful feature in SSH, allowing you to route connections through your SSH client. However, misconfiguration can lead to unexpected behaviors or security vulnerabilities.
Basic Command Forwarding:
session.setPortForwardingL(localPort, remoteHost, remotePort);
Commentary:
Using setPortForwardingL
, you should verify the ports you are connecting to. Incorrect configurations can expose sensitive data or undermine the very security the SSH protocol offers.
5. Forgetting to Clean Up Resources
It’s easy to forget to close your sessions and channels. Failing to do so can lead to memory leaks and resource exhaustion over time.
Example of Closing Sessions:
try {
// Your SSH commands
} finally {
if (session != null && session.isConnected()) {
session.disconnect();
}
}
This finally block ensures that the session is cleaned up after it has been used, which is crucial for maintaining performance and stability.
Best Practices for Java SSH Clients
To avoid falling into these pitfalls, it's wise to follow some best practices:
-
Use Connection Pools: If your application frequently opens and closes SSH connections, consider using connection pooling to optimize resource use and performance.
-
Log Everything: Implement logging for all your SSH activities. If something goes wrong, having detailed logs can help you figure out the issue quickly.
-
Security First: Always validate the authenticity of the SSH keys you are working with. Never hard-code sensitive details directly into your code.
-
Test Extensively: Implement error handling and tests. Try to simulate timeout conditions, failed logins, and malformed commands. This early testing can save you future headaches.
-
Stay Updated: Libraries evolve. Aim to keep your SSH libraries updated to benefit from the latest security patches and feature enhancements.
Additional Resources
For detailed documentation and advanced use cases, consider checking out:
The Last Word
Creating a simple Java SSH client offers a fascinating insight into network programming. However, understanding the common pitfalls associated with the implementation is key to crafting an effective and secure application. By applying the tips and techniques discussed in this post, you can minimize errors and optimize your SSH client experience.
With careful consideration of key management, exception handling, and resource cleanup, your SSH connections can be secure and stable. Do not overlook testing and security practices, and always keep your libraries up-to-date to adapt to new standards and security requirements.
Armed with this knowledge, you should be well-prepared to build efficient Java SSH clients that not only function well but also adhere to best practices in security and resource management. Happy coding!