Avoiding Pitfalls of Java 8 Default Methods in Interfaces
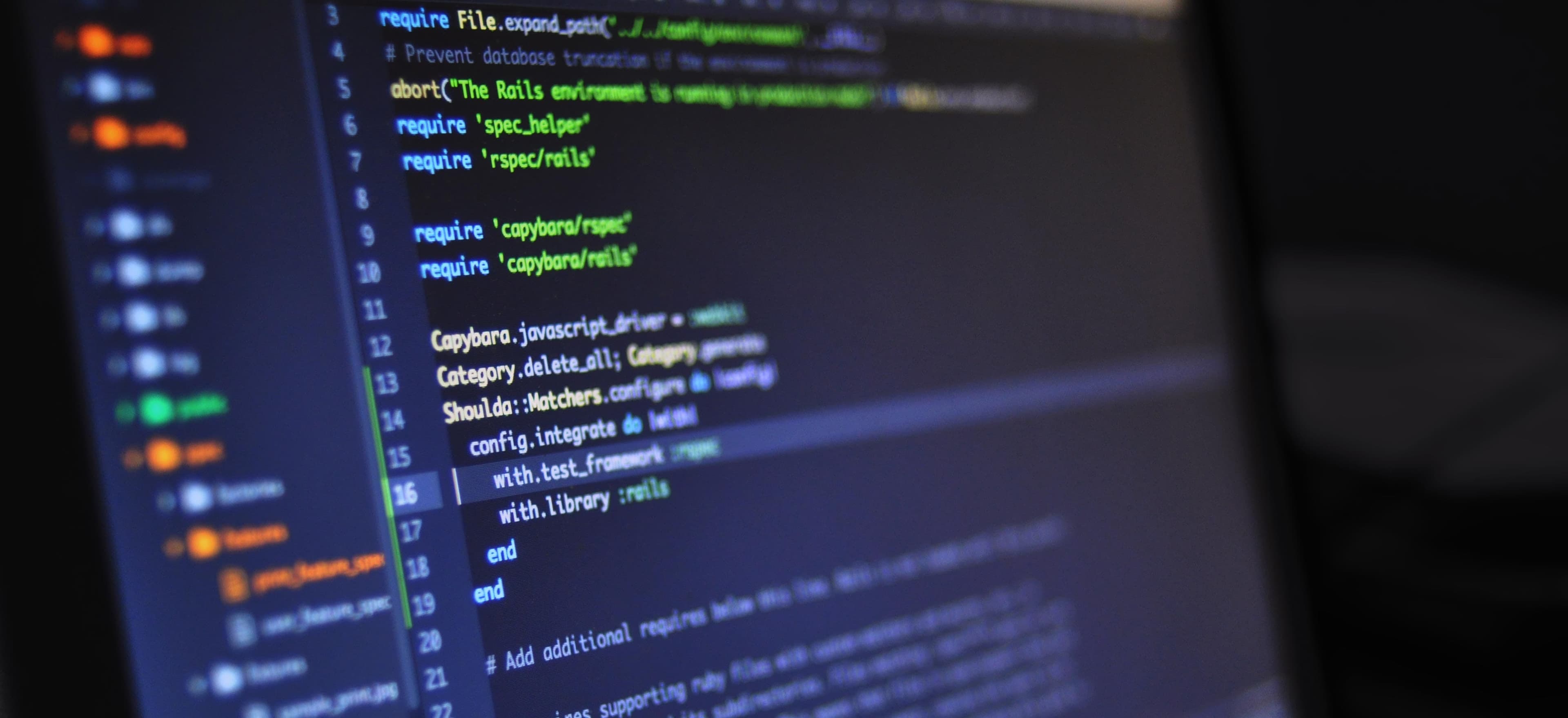
- Published on
Avoiding Pitfalls of Java 8 Default Methods in Interfaces
Java 8 introduced a significant enhancement to the Java programming language: default methods in interfaces. This feature allows developers to define methods within interfaces, providing an implementation that other classes can inherit. While this adds flexibility and reusability to the language, it also introduces potential pitfalls. In this blog post, we will explore these pitfalls and how to avoid them by following best practices.
Understanding Default Methods
Before diving into the pitfalls, it is essential to understand what default methods are and how they can be effectively utilized.
Default methods are declared using the default
keyword in an interface. They allow developers to add new methods to interfaces without breaking the existing implementations.
Example of a Default Method
public interface Vehicle {
void start();
default void stop() {
System.out.println("Vehicle is stopping.");
}
}
In the above example, the Vehicle
interface has a start
method that must be implemented by any class that implements the interface. However, the stop
method has a default implementation, meaning implementing classes can use this version directly or override it if desired.
The Benefits of Default Methods
- Backward Compatibility: You can add new methods to interfaces without breaking existing implementations.
- Ease of Use: Default methods provide a common base implementation that can be shared.
- Multiple Inheritance: Default methods allow you to inherit implementations from multiple interfaces.
Common Pitfalls of Default Methods
Despite their advantages, default methods can lead to several issues if not handled carefully. Here are some common pitfalls and how to avoid them:
1. Ambiguity in Multiple Inheritance
When a class implements multiple interfaces with the same default method, ambiguity arises. This can lead to compile-time errors, as the compiler will not know which default method to invoke.
Example:
public interface Engine {
default void start() {
System.out.println("Engine starting.");
}
}
public interface Electric {
default void start() {
System.out.println("Electric starting.");
}
}
public class ElectricCar implements Engine, Electric {
public void start() {
Engine.super.start(); // Explicitly calling Engine's start method
Electric.super.start(); // Explicitly calling Electric's start method
}
}
In this example, the ElectricCar
class implements both Engine
and Electric
, both of which have a start
method. By providing an explicit implementation, we can resolve the ambiguity.
Tips to Avoid Ambiguity:
- Keep method names consistent across different interfaces.
- Limit the use of default methods that share names across interfaces.
2. Overriding Default Methods
While overriding a default method can be a way to customize behavior, it can also lead to confusion if not documented correctly. If a method is overridden, the original intent may get lost, leading to maintenance challenges.
Example:
public class SportsCar implements Vehicle {
@Override
public void stop() {
System.out.println("SportsCar is stopping rapidly!");
}
}
Here, the SportsCar
class overrides the stop
method. While this is perfectly valid, it diverges from the default behavior of the base interface.
Tips for Documentation:
- Clearly document the purpose and the behavior of overridden methods.
- Use meaningful names for overridden implementations to distinguish from defaults.
3. Code Spread and Abuse
Using default methods can lead to a situation where business logic starts spreading across interfaces instead of remaining in the service or implementation classes. This may lead to an interface-heavy design that lacks clarity.
Solution:
Encourage the use of default methods for utility and common functionality rather than complex business logic. If a method requires intricate logic, it should likely be part of a concrete class or a separate utility class.
4. Testing Default Methods
Testing default methods can sometimes be tricky since they introduce behavior that is not inherently part of the implementing class. It requires careful consideration during the unit testing phase.
Solution:
Always include unit tests for the default methods in the interfaces themselves. This way, you ensure that the default implementations work as expected regardless of the implementations that choose to inherit them.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class VehicleTest {
@Test
public void testDefaultStop() {
Vehicle vehicle = new Vehicle() {
@Override
public void start() {
// No implementation required for this test
}
};
vehicle.stop(); // Should print "Vehicle is stopping."
}
}
Best Practices for Using Default Methods
- Use Sparingly: Only use default methods when they add real value.
- Favor Interface Segregation: Ensure interfaces remain lean and focused, adhering to the Interface Segregation Principle (ISP).
- Document Behavior: Clearly document the behavior of all default methods, especially if they are likely to be overridden.
- Test Rigorously: Implement effective unit tests for default methods to ensure reliability.
Final Thoughts
Java 8 default methods bring both opportunity and responsibility. They offer API designers a powerful tool to enhance flexibility and maintainability while enabling a seamless evolution of interfaces. However, developers must be cautious of the pitfalls such as ambiguity, maintenance challenges, and potential misuse.
By adhering to best practices, including thorough documentation and rigorous testing, we can harness the full potential of default methods while mitigating their risks. For more detailed information on Java interfaces and default methods, you can refer to the Java documentation.
As you continue to explore Java and its powerful features, remember to keep questioning how best to structure your code, ensuring simplicity, clarity, and robust functionality. Happy coding!