Why Java's Performance Issues Are Holding You Back
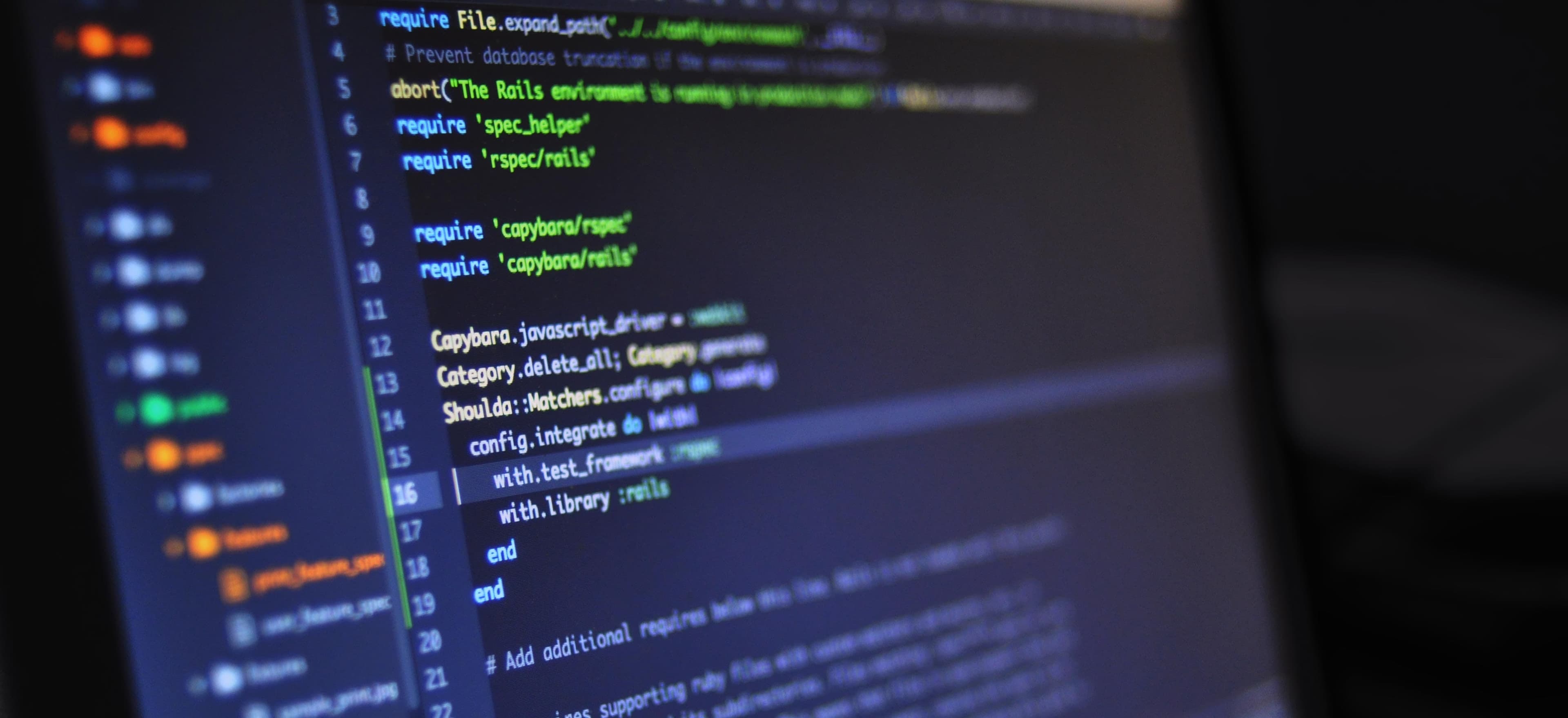
- Published on
Why Java's Performance Issues Are Holding You Back
Java has long been a staple in the world of programming languages, widely used for enterprise applications, mobile development, and server-side applications. However, performance issues often plague Java-based systems, holding developers back from achieving the levels of speed and efficiency they desire. In this blog post, we will dive into common performance issues in Java, exploring their causes and providing practical solutions to enhance your application's performance.
The Java Ecosystem: A Double-Edged Sword
Java’s extensive ecosystem, with frameworks like Spring and Hibernate, and its robustness make it an attractive choice for developers. However, these advantages can sometimes lead to slower performance due to additional layers of abstraction and configuration overhead. Understanding these trade-offs is the first step towards optimizing your Java application.
Memory Management and Garbage Collection
One of the most notable performance issues in Java comes from its automatic memory management system, particularly the Garbage Collector (GC). While GC simplifies memory handling and reduces memory leaks, it can introduce latency in your application.
What Happens During Garbage Collection?
When the GC runs, it stops application threads to reclaim memory, which can lead to performance bottlenecks.
public void createLargeObject() {
// Creating a large object within a method scope
int[] largeArray = new int[1000000];
// Simulate processing
for (int i = 0; i < largeArray.length; i++) {
largeArray[i] = i;
}
// largeArray will be eligible for GC once this method finishes
}
In this snippet, the large array consumes a considerable amount of memory, which can affect GC performance. The more transient objects you create, the more frequently the GC activates, causing pauses in your application. Managing object creation and scope can mitigate this issue.
Proactive Garbage Collection Strategies
-
Object Pooling: Instead of creating and destroying objects, maintain a pool from which your application can borrow and return objects.
public class ObjectPool { private List<ReusableObject> available = new ArrayList<>(); private List<ReusableObject> inUse = new ArrayList<>(); public ReusableObject borrowObject() { if (available.isEmpty()) { available.add(new ReusableObject()); } ReusableObject obj = available.remove(available.size() - 1); inUse.add(obj); return obj; } public void returnObject(ReusableObject obj) { inUse.remove(obj); available.add(obj); } }
-
Tuning the Garbage Collector: Use Java's command-line options to adjust the GC's behavior based on your application’s requirements. For example:
-Xms512m -Xmx2048m -XX:+UseG1GC
This adjusts the starting and maximum heap size while enabling the G1 Garbage Collector, engineered for large heap sizes and low pause times.
Slow Frameworks and Libraries
It's not only Java itself that can cause performance issues; many popular frameworks may introduce latency due to heavy abstractions or inefficient processes.
The Cost of Abstraction
Frameworks like Hibernate simplify database interactions but can introduce performance overhead. For instance, lazy loading can be convenient but might lead to the "N+1 Select Problem," where multiple database calls lead to slower queries.
// Example of N+1 Select Problem
List<User> users = userRepository.findAll();
for (User user : users) {
List<Order> orders = user.getOrders(); // Each call fetches orders separately
}
Effective Solutions for Framework Performance
-
Batch Fetching: Instead of fetching individual records, use joins or fetch groups to minimize database round trips.
@Query("SELECT u FROM User u JOIN FETCH u.orders") List<User> findAllWithOrders();
-
Caching: Leverage first-level and second-level caching strategies to store frequently accessed data and reduce database hits.
@Cacheable("users") public User findUserById(Long id) { return userRepository.findById(id); }
Multithreading and Concurrency Issues
Java's built-in concurrency utilities can either enhance performance or hinder it based on how they are implemented. Issues like thread contention, context switching, and shared resource access can lead to inefficient execution.
Understanding Thread Contention
Thread contention occurs when multiple threads attempt to access the same resource simultaneously, causing overhead due to locking mechanisms.
public synchronized void addToCollection(Object item) {
collection.add(item);
}
Using the synchronized
keyword guarantees thread safety but can slow down performance.
Optimizing Multithreading
-
Reduce Scope of Synchronization: The less time a thread spends holding a lock, the better.
public void addToCollection(Object item) { synchronized (this) { collection.add(item); } }
-
Use Concurrent Collections: Java provides alternatives like
ConcurrentHashMap
designed to handle concurrent operations efficiently.ConcurrentHashMap<String, String> concurrentMap = new ConcurrentHashMap<>(); concurrentMap.put("key", "value");
-
Fork/Join Framework: For tasks that can be broken into smaller subtasks, consider using the Fork/Join framework to harness multiple processors effectively.
public class Fibonacci extends RecursiveTask<Integer> {
private final int n;
public Fibonacci(int n) {
this.n = n;
}
@Override
protected Integer compute() {
if (n <= 1) return n;
Fibonacci f1 = new Fibonacci(n - 1);
f1.fork();
Fibonacci f2 = new Fibonacci(n - 2);
return f2.compute() + f1.join();
}
}
I/O Performance
Another significant area where Java applications can lag is I/O operations. File reading/writing, network communication, and database access can collectively introduce latency.
Enhancing I/O Operations
-
Use NIO (Non-blocking I/O): Java's NIO package allows for asynchronous operations that improve performance in applications needing simultaneous I/O operations.
Path path = Paths.get("data.txt"); try (AsynchronousFileChannel fileChannel = AsynchronousFileChannel.open(path, StandardOpenOption.READ)) { ByteBuffer buffer = ByteBuffer.allocate(1024); Future<Integer> result = fileChannel.read(buffer, 0); }
-
Buffering: Utilizing buffered streams improves efficiency by minimizing the number of I/O operations.
try (BufferedWriter writer = new BufferedWriter(new FileWriter("output.txt"))) { writer.write("Hello, World!"); }
Closing Remarks
Java has its performance pitfalls, but with a comprehensive understanding of its various performance issues, developers can significantly enhance their applications’ efficiency. Employing strategies like managing garbage collection, optimizing frameworks, improving concurrency, and performance tuning I/O can elevate your Java applications to new heights.
Take charge of your Java performance today! Embrace these optimization techniques, and transform your application from sluggish to speedy.
For more insights on Java performance optimization, check out Java Performance Tuning Guide or dive deeper into Java Concurrency.
By incorporating the above practices, you're not just addressing performance issues; you're investing in the long-term viability and scalability of your Java applications. Start cultivating a performance mindset today!